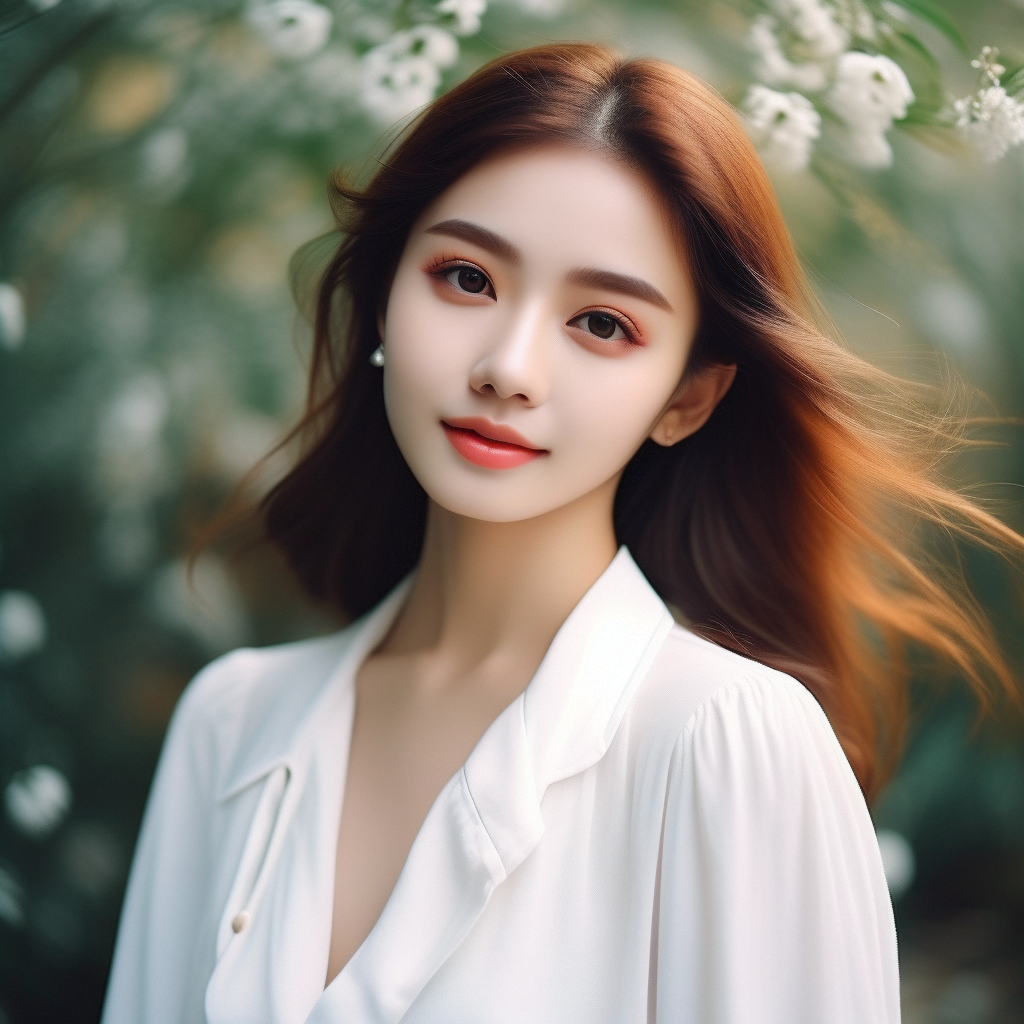
js实现贪吃蛇游戏
<!DOCTYPE html><html lang="en"><head><meta charset="UTF-8"><meta name="viewport" content="width=device-width, initial-scale=1.0"><title>贪吃蛇游戏</title><style
·
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>贪吃蛇游戏</title>
<style>
.game-container {
width: 300px;
height: 300px;
border: 1px solid #000;
position: relative;
}
.snake {
width: 20px;
height: 20px;
background-color: green;
position: absolute;
}
.food {
width: 20px;
height: 20px;
background-color: red;
position: absolute;
}
button {
display: block;
margin-top: 10px;
}
</style>
</head>
<body>
<div class="game-container">
<div class="snake" id="snake"></div>
<div class="food" id="food"></div>
</div>
<button id="start">开始游戏</button>
<script>
const gameContainer = document.querySelector(".game-container");
const snakeElement = document.getElementById("snake");
const foodElement = document.getElementById("food");
const startButton = document.getElementById("start");
let snake = [{ x: 10, y: 10 }];
let food = { x: 5, y: 5 };
let direction = "right";
let isGameRunning = false;
function moveSnake() {
if (!isGameRunning) return;
const head = { ...snake[0] };
switch (direction) {
case "up":
head.y--;
break;
case "down":
head.y++;
break;
case "left":
head.x--;
break;
case "right":
head.x++;
break;
}
snake.unshift(head);
if (head.x === food.x && head.y === food.y) {
// 吃到食物
food = {
x: Math.floor(Math.random() * 15),
y: Math.floor(Math.random() * 15),
};
} else {
snake.pop();
}
// 检查碰撞
if (
head.x < 0 ||
head.x >= 15 ||
head.y < 0 ||
head.y >= 15 ||
snake.some((segment, index) => index !== 0 && segment.x === head.x && segment.y === head.y)
) {
isGameRunning = false;
alert("游戏结束!");
return;
}
updateGameBoard();
setTimeout(moveSnake, 200);
}
function updateGameBoard() {
snakeElement.innerHTML = "";
snake.forEach((segment) => {
const segmentElement = document.createElement("div");
segmentElement.className = "snake";
segmentElement.style.left = segment.x * 20 + "px";
segmentElement.style.top = segment.y * 20 + "px";
snakeElement.appendChild(segmentElement);
});
foodElement.style.left = food.x * 20 + "px";
foodElement.style.top = food.y * 20 + "px";
}
startButton.addEventListener("click", () => {
if (!isGameRunning) {
snake = [{ x: 10, y: 10 }];
direction = "right";
isGameRunning = true;
moveSnake();
}
});
document.addEventListener("keydown", (event) => {
switch (event.key) {
case "ArrowUp":
if (direction !== "down") direction = "up";
break;
case "ArrowDown":
if (direction !== "up") direction = "down";
break;
case "ArrowLeft":
if (direction !== "right") direction = "left";
break;
case "ArrowRight":
if (direction !== "left") direction = "right";
break;
}
});
updateGameBoard();
</script>
</body>
</html>
更多推荐
所有评论(0)