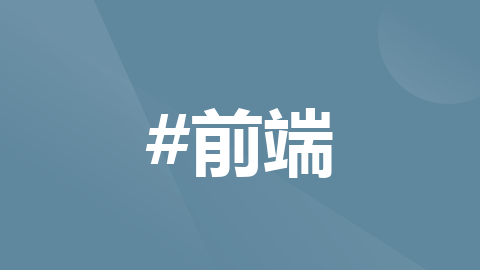
前端在线预览从服务器获取的Excel(.xlsx)文件 ,Pdf(.pdf),Word(.docx)文件
首先先要安装对应的预览插件。
·
首先先要安装对应的预览插件
npm install docx-preview
npm install xlsx
npm install vue-pdf
<template>
<div class="preview-container" v-loading="loading">
<div ref="docx" v-if="fileType == 'docx'"></div>
<div v-else-if="fileType == 'pdf'">
<pdf v-for="p in pdfInfo.pageCount" :key="p" :src="pdfInfo.src" :page="p"
style="display: inline-block; width: 100%"></pdf>
</div>
<div v-else-if="fileType == 'xlsx'">
<el-button-group>
<el-button size="mini" type="primary" v-for="(item, index) in workbook.SheetNames" @click="getTable(item)"
:key="index">{{ item }}</el-button>
</el-button-group>
<el-table :data="excelData" size="mini" :stripe="false" :border="true" :fit="false">
<el-table-column v-for="(value, key, index) in excelData[2]" :key="index" :prop="key"
:label="key"></el-table-column>
</el-table>
</div>
</div>
</template>
<script>
import axios from 'axios';
import pdf from 'vue-pdf';
// import { mapGetters } from 'vuex'
import * as XLSX from 'xlsx';
import { pptToPdf } from '@/api/chapter/info';
const docx = require('docx-preview');
/**
* @description 根据文件名获取文件后缀
* @param {String} path 文件路径
*/
function getFileType(path) {
if (!path) return '';
else {
path = path.toLocaleLowerCase();
let index = path.lastIndexOf('.');
if (index == -1) return ''; // 未知类型
return path.substring(index + 1);
}
}
export default {
data() {
return {
fileType: '',
pdfInfo: {
pageCount: 1,
page: 1,
src: ''
},
excelData: [],
workbook: {},
loading: false
}
},
props: {
previewFileInfo: {
default: {}
}
},
components: {
pdf
},
created() {
let fileType = getFileType(this.previewFileInfo.url)
this.fileType = fileType
switch (fileType) {
case 'docx':
this.readExcelFromRemoteFile(this.previewFileInfo.url)
break;
case 'pdf':
this.loadPdf(this.previewFileInfo.url)
break;
case 'xlsx':
this.loadExcel(this.previewFileInfo.url)
break;
default:
break;
}
},
methods: {
// 渲染word文档
readExcelFromRemoteFile(url) {
var vm = this;
this.loading = true;
axios({
method: 'get',
url: url,
responseType: 'blob',
})
.then(response => {
vm.loading = false;
docx
.renderAsync(response.data, vm.$refs.docx)
.then(x => console.log('加载文档完毕'))
.catch(err => {
vm.$message.error('加载文档失败,' + err.toString());
});
})
.catch(err => {
this.loading = false;
});
},
// 渲染pdf
loadPdf(url) {
this.loading = true;
this.$set(this.pdfInfo, 'src', pdf.createLoadingTask(url));
this.pdfInfo.src.promise
.then(pdf => {
this.$set(this.pdfInfo, 'pageCount', pdf.numPages);
this.loading = false;
})
.catch(err => {
this.$message.error('加载PDF文件失败。' + err.toString());
this.loading = false;
});
},
// 渲染xslx文件
loadExcel(url) {
this.loading = true;
axios({
method: 'get',
url: url,
responseType: 'arraybuffer'
})
.then(res => {
var data = new Uint8Array(res.data)
this.workbook = XLSX.read(data, { type: 'array' });
this.getTable(this.workbook.SheetNames[0]);
})
.catch(err => {
this.loading = false;
this.$message.error('加载文档失败,' + err.toString());
});
},
// 加载表格数据
getTable(sheetName) {
var worksheet = this.workbook.Sheets[sheetName];
this.excelData = XLSX.utils.sheet_to_json(worksheet);
this.loading = false;
},
}
}
</script>
更多推荐
所有评论(0)