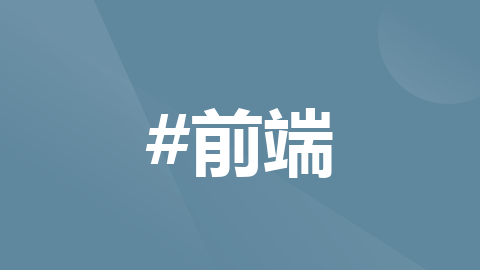
前端 单例模式新建一个类js vue
单例模式的目标是确保一个类只有一个实例,并提供一个全局访问点来访问该实例。
·
单例模式的目标是确保一个类只有一个实例,并提供一个全局访问点来访问该实例。
advMaster.js
export const advManager = new (class {
constructor() {
this.sourceList = [];
this.pendingPromise = null;
}
initialize(sources) {
this.sourceList = sources;
}
requestAd(id) {
return new Promise((resolve, reject) => {
if (this.pendingPromise) {
this.pendingPromise.then((result) => resolve(this.findAd(result, id)));
} else {
this.pendingPromise = advMasterApi.getBannerInfos(
this.sourceList,
config.env === 'development' ? 'staging' : config.env,
{ systemCode: getSystemCode() },
)
.then((adxData = {}) => {
const { adm = [] } = adxData;
if (adm && adm.length > 0) {
resolve(this.findAd(adm, id));
return adm;
}
reject(new Error('No advertising data available'));
return [];
})
.catch(reject);
}
});
}
findAd(adList, id) {
return (adList || []).find((ad) => ad.impid === id);
}
})();
index.vue
const adResources = ['123', '456'];
advManager.initialize(adResources);
banner.vue
advManager.requestAd(bannerID).then((result) => {
if (result) {
this.bannerContent = result;
this.showBanner = true;
}
});
解析-单例模式
advMaster.js中,通过const advInstall = new (class {…})()实现单例。
单例模式的目标是确保一个类只有一个实例,并提供一个全局访问点来访问该实例。
这个代码片段是一个立即执行的类表达式,会在代码加载时执行一次。
这意味着它会创建一个实例并赋值给 advInstall 变量,然后这个实例就会一直存在于应用程序的内存中。
普通类创建的方法例子
调用可反复创建实例,不是同一个。
export class AdvInstall {
constructor() {
this.sourceList = [];
this.pendingPromise = null;
}
initialize(sources) {
this.sourceList = sources;
}
requestAd(id) {
return new Promise((resolve, reject) => {
// Your implementation here...
});
}
findAd(adList, id) {
return (adList || []).find((ad) => ad.impid === id);
}
}
const resources1 = ['123', '456'];
const advInstall1 = new AdvInstall();
advInstall1.initialize(resources1);
advInstall1.requestAd(id);
const resources2 = ['789', '101'];
const advInstall2 = new AdvInstall();
advInstall2.initialize(resources2);
advInstall2.requestAd(id);
更多推荐
所有评论(0)