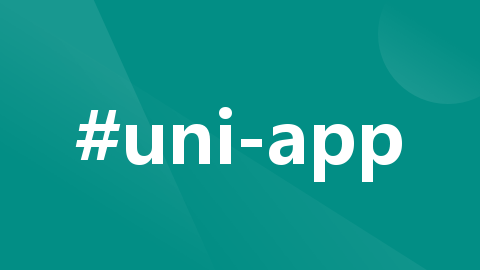
uniapp中的数据缓存
本地缓存功能是html5的新特性,uniapp中同样提供了数据的本地化操作,其中包括存储、获取、删除、清空等四个方面。数据缓存分为异步和同步,而他们的区别在于,同步请求需要有一个等待请求成功的过程,而异步请求不会有这个过程,同学们有的就疑问了,为什么不直接都用异步请求呢,不用等待,多好,但是,在实际开发中,有的些时候有必要使用同步请求的,大多数还是异步请求。
文章目录
-
- uni.setStorage(OBJECT) AND uni.setStorageSync(KEY,DATA)
- uni.getStorage(OBJECT) AND uni.getStorageSync(KEY)
- uni.getStorageInfo(OBJECT) AND uni.getStorageInfoSync()
- uni.removeStorage(OBJECT) AND uni.removeStorageSync(KEY)
- uni.clearStorage() AND uni.clearStorageSync()
前言
本地缓存功能是html5的新特性,uniapp中同样提供了数据的本地化操作,其中包括存储、获取、删除、清空等四个方面。
数据缓存分为异步和同步,而他们的区别在于,同步请求需要有一个等待请求成功的过程,而异步请求不会有这个过程,同学们有的就疑问了,为什么不直接都用异步请求呢,不用等待,多好,但是,在实际开发中,有的些时候有必要使用同步请求的,大多数还是异步请求
内容
一、uni.setStorage(OBJECT) AND uni.setStorageSync(KEY,DATA)
(1)uni.setStorage(OBJECT) 将数据存储在本地缓存中指定的 key 中,会覆盖掉原来该 key 对应的内容,这是一个异步接口。
uni.setStorage({
key: 'storage_key',
data: 'hello',
success: function () {
console.log('success');
}
});
(2) uni.setStorageSync(KEY,DATA) 将 data 存储在本地缓存中指定的 key 中,会覆盖掉原来该 key 对应的内容,这是一个同步接口。
try {
uni.setStorageSync('storage_key', 'hello');
} catch (e) {
// error
}
二、uni.getStorage(OBJECT) AND uni.getStorageSync(KEY)
(1)uni.getStorage(OBJECT) 从本地缓存中异步获取指定 key 对应的内容。
uni.getStorage({
key: 'storage_key',
success: function (res) {
console.log(res.data);
}
});
(2)uni.getStorageSync(KEY) 从本地缓存中同步获取指定 key 对应的内容。
try {
const value = uni.getStorageSync('storage_key');
if (value) {
console.log(value);
}
} catch (e) {
// error
}
三、uni.getStorageInfo(OBJECT) AND uni.getStorageInfoSync()
(1)uni.getStorageInfo(OBJECT) 异步获取当前 storage (key的属性名)的相关信息。
uni.getStorageInfo({
success: function (res) {
console.log(res.keys);
console.log(res.currentSize);
console.log(res.limitSize);
}
});
(2)uni.getStorageInfoSync() 同步获取当前 storage(key的属性名)的相关信息。
try {
const res = uni.getStorageInfoSync();
console.log(res.keys);
console.log(res.currentSize);
console.log(res.limitSize);
} catch (e) {
// error
}
四、uni.removeStorage(OBJECT) AND uni.removeStorageSync(KEY)
(1)uni.removeStorage(OBJECT) 从本地缓存中异步移除指定 key。
uni.removeStorage({
key: 'storage_key',
success: function (res) {
console.log('success');
}
});
(2)uni.removeStorageSync(KEY) 从本地缓存中同步移除指定 key。
try {
uni.removeStorageSync('storage_key');
} catch (e) {
// error
}
五、uni.clearStorage() AND uni.clearStorageSync()
(1)uni.clearStorage() 异步清理本地数据缓存。
uni.clearStorage();
(2)uni.clearStorageSync() 同步清理本地数据缓存。
try {
uni.clearStorageSync();
} catch (e) {
// error
}
小结
uni-app的缓存虽然方法有些多,但是总结一下也就是有五个,
添加,取值,查看key,删除某一个,删除全部,
只是在这些基础上添加了异步和同步
大家更具实际情况选着即可
练习
这里为大家写了一个练习,大家可以看着理解一下,结果在Console控制台
下面是代码
<template>
<view class="box">
<button type="primary" @click="setname">异步缓存一个name</button>
<button type="primary" @click="setage">同步缓存一个age</button>
<button type="primary" @click="getname">异步得到key为name的data</button>
<button type="primary" @click="getage">同步得到key为age的data</button>
<button type="primary" @click="getall">异步获取当前storage相关信息(Key的名字)</button>
<button type="primary" @click="gettall">同步获取当前storage相关信息(Key的名字)</button>
<button type="primary" @click="removevalue">从本地缓存中异步移除指定(name) key</button>
<button type="primary" @click="removeTvalue">从本地缓存中同步移除指定(age) key</button>
<button type="primary" @click="clearall">清理本地数据缓存(异步)</button>
<button type="primary" @click="cleartall">同步清理本地数据缓存</button>
</view>
</template>
<script>
export default {
data() {
return {
setname() {
uni.setStorage({
key: "name",
data: "郭向前",
success: () => {
console.log("success")
}
})
},
setage() {
try {
uni.setStorageSync("age", "18")
} catch (e) {
//TODO handle the exception
}
},
getname() {
uni.getStorage({
key:"name",
success:function(res){
console.log(res.data)
}
})
},
getage() {
try {
const value = uni.getStorageSync("age")
if (value) {
console.log(value)
} else {
console.log("null")
}
} catch (e) {
//TODO handle the exception
}
},
getall() {
uni.getStorageInfo({
success: function(res) {
console.log(res.keys)//获取key的每一项
console.log('当前占用:'+res.currentSize);//当前占用的空间大小, 单位:kb
console.log("限制:"+res.limitSize);//限制的空间大小, 单位:kb
}
})
},
gettall(){
try{
const res = uni.getStorageInfoSync()
console.log(res.keys)//获取key的每一项
console.log('当前占用:'+res.currentSize);//当前占用的空间大小, 单位:kb
console.log("限制:"+res.limitSize);//限制的空间大小, 单位:kb
}catch(e){
//TODO handle the exception
}
},
removevalue(){
uni.removeStorage({
key:"name",
success: function (res) {
console.log('success');
}
})
},
removeTvalue(){
try{
uni.removeStorageSync("age");
}catch(e){
//TODO handle the exception
}
},
clearall(){
uni.clearStorage();
},
cleartall(){
try{
uni.clearStorageSync()
}catch(e){
//TODO handle the exception
}
}
}
}
}
</script>
<style>
</style>
更多推荐
所有评论(0)