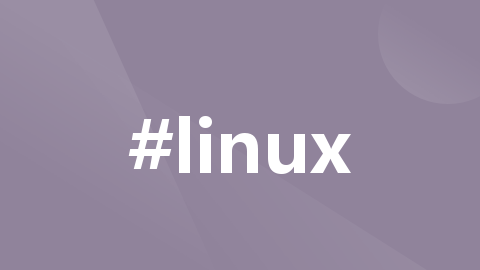
linux 中串口操作之中断 读取
1.windows端使用虚拟串口,通过串口工具发送消息。工具:vspd ,eclipse,串口调试助手。2.linux端,使用此代码读取。
·
#include "pthread.h"
#include <sys/msg.h>
#include <sys/types.h>
#include <stdio.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
#include <string.h>
#include <stdlib.h>
#include <stddef.h>
#include <fcntl.h>
#include <netinet/in.h>
#include <sys/shm.h>
#include <sys/socket.h>
#include <sys/ipc.h>
/*信号相关*/
#include<sys/signal.h>
/*信号量相关*/
#include <semaphore.h>
/*串口相关*/
#include <sys/stat.h>
#include <unistd.h>
#include <termios.h>
int wait_flag =1;
int stop = 1;
unsigned char buf[255] = {0};
int serial_fd;
/******************************************
信号处理函数,设备wait_flag=FASLE
******************************************************/
void signal_handler_IO(int status)
{
printf("received SIGIO signale.\n");
wait_flag = 0;
}
void ReceiveTaskHandler(void *argv)
{
int res;
while(1)
{
usleep(100);
/* after receving SIGIO ,wait_flag = FALSE,input is availabe and can be read*/
if(wait_flag == 0)
{
memset(buf,0,255);
res = read(serial_fd,buf,255);
printf("nread=%d\n",res);
for(int i=0 ;i <res; i++)
{
printf("%02x",buf[i]);
}
wait_flag = 1; /*wait for new input*/
}
}
}
int main()
{
struct sigaction saio;
struct termios old_cfg;
struct termios new_cfg;
//1.打开串口设备文件
serial_fd = open("/dev/ttyS0", O_RDWR | O_NOCTTY | O_NDELAY);
if (serial_fd == -1)
{
perror("open");
exit(-1);
}
//2.备份现有的串口配置
if (-1 == tcgetattr(serial_fd, &old_cfg))
{
perror("tcgetattr");
exit(-1);
}
//3.原始模式
new_cfg = old_cfg;
cfmakeraw(&new_cfg);
//4.配置波特率
cfsetispeed(&new_cfg, B115200);
cfsetospeed(&new_cfg, B115200);
//5.设置控制标志
new_cfg.c_cflag |= CREAD | CLOCAL; //使能数据接收和本地模式
//6.设置帧结构
new_cfg.c_cflag &= ~CSTOPB; //1位停止位
new_cfg.c_cflag &= ~CSIZE; //去掉数据位屏蔽
new_cfg.c_cflag |= CS8; //8位数据位
new_cfg.c_cflag &= ~PARENB; //无校验
//7.设置阻塞模式
tcflush(serial_fd, TCIOFLUSH);
//无阻塞
new_cfg.c_cc[VTIME] = 0;
new_cfg.c_cc[VMIN] = 0;
tcflush(serial_fd, TCIOFLUSH);
/*注册信号*/
saio.sa_handler = signal_handler_IO;
sigemptyset(&saio.sa_mask);
saio.sa_flags = 0;
saio.sa_restorer = NULL;
sigaction(SIGIO, &saio, NULL);
//allow the process to receive SIGIO
fcntl(serial_fd, F_SETOWN, getpid());
//make the file descriptor asynchronous
fcntl(serial_fd, F_SETFL, FASYNC);
//8.使能配置生效
if (-1 == tcsetattr(serial_fd, TCSANOW, &new_cfg))
{
perror("tcgetattr");
exit(-1);
}
pthread_t id;
pthread_create(&id, NULL, (void *)ReceiveTaskHandler, NULL);
pthread_join(id,NULL);
close(serial_fd);
return 0;
}
1.windows端使用虚拟串口,通过串口工具发送消息
2.linux端,使用此代码读取
工具:vspd ,eclipse,串口调试助手
window端
更多推荐
所有评论(0)