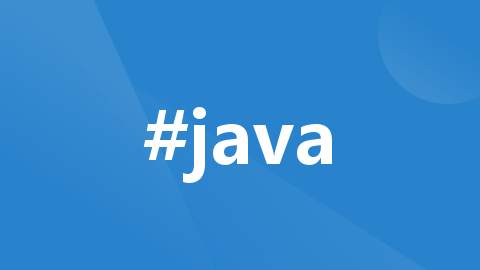
java中线程安全的list
在Java中,有多种线程安全的List实现可用于多线程环境中。

一键AI生成摘要,助你高效阅读
问答
·
文章目录
在Java中,有多种线程安全的List实现可用于多线程环境中。以下是一些常见的线程安全的List实现,以及它们的详细特性和用法:
1. CopyOnWriteArrayList
- 特性:CopyOnWriteArrayList是线程安全的List实现,通过对底层数组进行复制来实现线程安全。读操作不会阻塞,而写操作会创建一个新的数组进行修改,确保写操作不影响读操作。
- 用法:CopyOnWriteArrayList适用于读操作频繁、写操作较少的场景,例如缓存或只读数据。它提供了线程安全的遍历,但写操作的开销较高。
import java.util.concurrent.CopyOnWriteArrayList;
public class CopyOnWriteArrayListExample {
private CopyOnWriteArrayList<String> list = new CopyOnWriteArrayList<>();
public void addElement(String element) {
list.add(element);
}
public String getElement(int index) {
return list.get(index);
}
}
2. ConcurrentLinkedDeque
- 特性:ConcurrentLinkedDeque是线程安全的双向队列实现,基于无锁的并发算法实现。它提供了高效的并发操作,支持无界容量。
- 用法:ConcurrentLinkedDeque适用于需要高并发性能且无需固定容量的场景。它具有常见队列操作的线程安全性,如添加、删除和获取元素等。
import java.util.concurrent.ConcurrentLinkedDeque;
public class ConcurrentLinkedDequeExample {
private ConcurrentLinkedDeque<String> deque = new ConcurrentLinkedDeque<>();
public void addFirst(String element) {
deque.addFirst(element);
}
public String pollLast() {
return deque.pollLast();
}
}
3. ConcurrentLinkedQueue
- 特性:ConcurrentLinkedQueue是线程安全的队列实现,基于无锁的并发算法实现。它提供了高效的并发操作,支持无界容量。
- 用法:ConcurrentLinkedQueue适用于需要高并发性能且无需固定容量的场景。它适合作为任务队列或事件队列使用。
import java.util.concurrent.ConcurrentLinkedQueue;
public class ConcurrentLinkedQueueExample {
private ConcurrentLinkedQueue<String> queue = new ConcurrentLinkedQueue<>();
public void enqueue(String element) {
queue.add(element);
}
public String dequeue() {
return queue.poll();
}
}
4.ConcurrentSkipListSet
- 特性:ConcurrentSkipListSet是线程安全的有序集合实现,基于跳表(Skip List)数据结构实现。它提供了高并发性能,支持有序性和去重。
- 用法:ConcurrentSkipListSet适用于需要高并发性能且有序的集合操作。它提供了类似于TreeSet的操作,并且线程安全。
import java.util.concurrent.ConcurrentSkipListSet;
public class ConcurrentSkipListSetExample {
private ConcurrentSkipListSet<String> set = new ConcurrentSkipListSet<>();
public void addElement(String element) {
set.add(element);
}
public boolean containsElement(String element) {
return set.contains(element);
}
}
5. Vector
- 特性:Vector是Java早期的线程安全List实现,采用同步方法(synchronized)实现线程安全。它支持随机访问,具有固定容量和自动扩容机制。
- 用法:Vector适用于需要线程安全的动态数组操作。但是,由于使用了同步方法,它在高并发环境下的性能相对较低,通常不推荐在新的代码中使用。
import java.util.Vector;
public class VectorExample {
private Vector<String> vector = new Vector<>();
public void addElement(String element) {
vector.add(element);
}
public String getElement(int index) {
return vector.get(index);
}
}
常见的面试题
这些线程安全List实现相关的主题可能包括:
-
什么是线程安全的List?为什么在多线程环境中使用线程安全的List?
- 解析:线程安全的List是可以在多线程环境下安全访问和修改的List实现。在多线程环境中使用线程安全的List可以防止并发冲突和数据不一致性的问题,确保数据的一致性和正确性。
-
CopyOnWriteArrayList和ArrayList的区别是什么?在什么场景下使用CopyOnWriteArrayList?
- 解析:CopyOnWriteArrayList和ArrayList的主要区别在于线程安全性和写操作的开销。CopyOnWriteArrayList是线程安全的,适用于读操作频繁、写操作较少的场景。ArrayList不是线程安全的,适用于单线程环境或通过外部同步机制实现线程安全。
-
ConcurrentLinkedDeque和ConcurrentLinkedQueue有什么区别?在什么场景下使用它们?
- 解析:ConcurrentLinkedDeque和ConcurrentLinkedQueue都是线程安全的队列实现,区别在于它们的数据结构和操作方式。ConcurrentLinkedDeque是双向队列,支持在队列头尾进行添加、删除和获取元素。ConcurrentLinkedQueue是单向队列,只支持在队列尾进行添加和在队列头进行删除和获取元素。根据需求选择合适的操作方式和数据结构。
-
ConcurrentSkipListSet和HashSet的区别是什么?在什么场景下使用ConcurrentSkipListSet?
- 解析:ConcurrentSkipListSet和HashSet的主要区别在于线程安全性和有序性。ConcurrentSkipListSet是线程安全的有序集合,基于跳表实现。HashSet不是线程安全的无序集合。ConcurrentSkipListSet适用于需要高并发性能和有序集合操作的场景。
-
Vector相对于ArrayList的优缺点是什么?为什么不推荐在新的代码中使用Vector?
- 解析:Vector和ArrayList都是动态数组实现,但Vector是线程安全的,而ArrayList不是。Vector的优点是线程安全,支持随机访问和自动扩容。然而,Vector在高并发环境下性能较低,因为它使用了同步方法。在新的代码中,推荐使用ArrayList并通过其他方式(例如使用同步控制)实现线程安全。
更多推荐
所有评论(0)