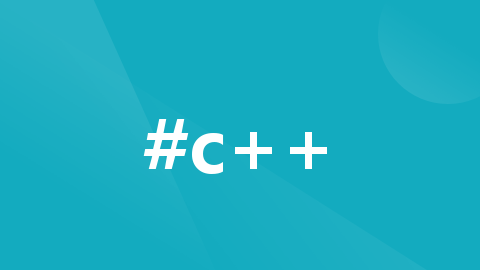
C++ string 基本用法
C++string函数的基本使用方法

目录
一、C++ string 的创建方式
1、string 的头文件
#include <iostream>//等价于C语言中的#include<stdio.h>
#include <cstring>
#include <string.h>
//以上两种都是C语言的string头文件
#include <string>//真正的C++头文件
TIP:
在C++中,#include<string>才是真正的C++头文件!
2、常见的创建 string 方式
int main()
{
string str1 = "str1";
cout << str1 << endl;//调用构造函数
string str2;
str2 = "str2";
cout << str2 << endl;//调用拷贝构造函数
string str3 = ("str3");
cout << str3 << endl;
return 0;
}
输出结果:
3、少见的初始化方式
string str4(4,'x);
用4个字符初始化字符串,输出结果就是xxxx
string str5("iloveyou",1,4);
表示用字符串从下标1开始的4位初始化str5(在C++中,字符串的下标也是从0开始的,跟C语言的数组一样)
所以输出结果就是love
二、C++ string 与C语言 char* 的区别
1、最本质的区别
在于C++ string 定义的字符串没有 ' \0 ' !
char arr[]="iloveyou";
string str5="iloveyou";
cout<<str5.size()<<endl;
cout<<str5.length()<<endl;
C++中两种输出字符串的函数——length(),size()
上面用char类型定义的一共有8+1个字符,而下面用string定义的输出结果就是8个字符。
2、如何访问 string 定义的字符串?
注意string类型可以直接用数组形式【】中括号访问,并且下标也是从1开始!
首先不能用C语言中的printf(%s)函数访问,因为string类型本质上并不是字符串类型。
若强行访问,输出结果就是乱码!
C++ string为我们提供访问字符串的接口——data(),c_str();
这两个函数将string类型转换为字符串类型,方便我们用printf函数打印。
int main()
{
string str1 = "str1";
cout << str1 << endl;
printf("%s\n", str1);
printf("%s\n", str1.data());
printf("%s\n", str1.c_str());
return 0;
}
输出结果
三、C++ string 的基本操作
1、字符串的比较
在C++中,字符串比较的规则与C语言相同。
(1)、字符串如何比较
问:“123”和“13”相比,哪个字符串大?
答:“13”更大,因为从左到右比较,字符按照ASCII码值比较,第一个字符都是‘1’,第二个字符‘3’比‘2’大,所以“13”比“123”大。
在C++中,可以cout直接输出判断字符串的比较
(2)cout比较实操
int main()
{
string first = "123";
string second = "13";
cout << (first < second) << endl;
cout << (first != second) << endl;
cout << (first > second) << endl;
return 0;
}
注意事项
- 因为返回值的问题,所以要用()把比较的式子括起来
- 返回值是bool类型,若是真,返回1,假就返回0。
(3)调用比较成员函数
cout << first.compare(second) << endl;
返回值为1:first-second>0 ------> first>second
返回值为-1:first-second<0 ------> first<second
返回值为0:first-second=0 ------> first=second
2、字符串连接
(1)cout直接法
int main()
{
string first = "i love you,";
string second = "i miss you";
cout << first + second << endl;
return 0;
}
想要把first和second两个字符串进行相加,直接在cout里面使用加法 + !
输出结果就是:i love you,i miss you
(2)通过append()函数连接
因为上述cout直接+,只能将两个完整的字符串进行相加,太笨重,而append()函数更加灵活,可以决定连接第几位开始,且数量可以自己定。
int main()
{
string first = "i love you,";
string second = "i miss you";
cout << first + second << endl;
first.append(second);
cout << first << endl;
first.append(second, 1, 5);
cout << first << endl;
first.append(4, '1');
cout << first << endl;
first.append("i miss you", 1, 5);
cout << first << endl;
return 0;
}
输出结果:
3、字符/字符串的查找
前言:
string查找类型的函数,如果查找不到,就返回-1。
因此在查找时,一般需要判断返回值是否等于string::nops(值就是-1)
(1)常用的find函数和rfind函数查找
find函数从左往右查找字符串或者字符出现的位置。
rfind函数从右往左查找字符串或者字符出现的位置。
int main()
{
string first = "i love you,";
string second = "i miss you";
if (first.find('o') != -1)
{
cout << first.find('o') << endl;
}
if (first.find("love") != -1)
{
cout << first.find("love")<< endl;
}
return 0;
}
4、字符串的替换(replace函数)
replace函数的参数一般开始是原始想要替换的下标,之后是替换字符的个数。
int main()
{
string first = "iloveyou,";
string second = "imissyou";
first.replace(1, 4,second,1,4);
cout << first << endl;
return 0;
}
输出结果就是 imissyou
5、字符串的删除(erase函数)
int main()
{
string first = "iloveyou,";
string second = "imissyou";
first.erase(0);
cout << first << endl;
second.erase(1, 4);
cout << second << endl;
return 0;
}
第一种使用方法是删除下标0,及以后的字符(注意是后面的字符全部干掉!)
第二种是用方法是删除下标1,之后的4个字符(自定义删除的个数)
6、字符串的遍历(iterator容器遍历)
iterator容器遍历适用于任何stl库,我们只需要掌握一个方法,其他依葫芦画瓢即可。
iterator使用方法像是指针,但跟指针有区别,后续会持续更新~
更多推荐
所有评论(0)