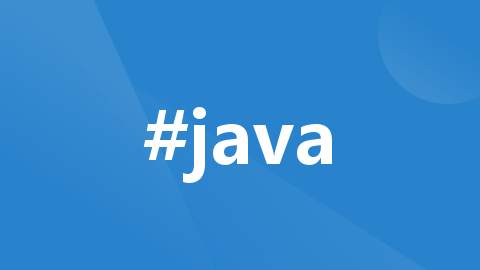
String.format()格式化输出
@[TOC](文章目录)

文章目录
String.format() 方法是 Java 的一个格式化输出方法。它可将不同类型的数据格式化为指定格式的字符串,并将结果存储在字符串中。
方法参数
String format(String format, Object... args)
- format:要返回的字符串格式
- args:要在格式中替换的值
format中可以包含若干个占位符,占位符由百分号%跟随一个格式类型字符组成。
常用占位符
占位符 | 类型 | 描述 |
---|---|---|
s | 字符串 | 通常用于字符串类型和任意对象,输出任意对象的toString()方法返回值 |
d | 整数 | 通常用于整数类型和 byte、short、int、long 等原始类型 |
f | 浮点数 | 通常用于浮点数类型和 float 和 double 等原始类型 |
c | 字符 | 通常用于字符类型和 char 等原始类型 |
b | 布尔 | 通常用于布尔类型和 boolean等原始类型 |
t | 日期/时间 | 通常用于日期和时间类型 |
e | 科学计数法 | 通常用于将浮点数字格式化为科学计数法。 |
占位符使用
- %s:字符串
String format1 = String.format("姓名:%s,性别:%s", "李哈哈", "男");
System.out.println(format1);
姓名:李哈哈,性别:男
- %d:整数
String format2 = String.format("年龄:%d", 28);
System.out.println(format2);
年龄:28
- %f:浮点数
String format3 = String.format("语文:%f,数学:%f", 95.5, 99.5);
System.out.println(format3);
语文:95.500000,数学:99.500000
- %c:字符
String format4 = String.format("语文:%c,数学:%c", 'A', 'B');
System.out.println(format4);
语文:A,数学:B
- %b:布尔
String format5 = String.format("真:%b,假:%b,真:%b,真:%b,真:%b", true, false, "", 0, -1);
System.out.println(format5);
真:true,假:false,真:true,真:true,真:true
注意:除了false,其他值都输出为true。
- %t:日期/时间
String format6 = String.format("今天是:%tF", new Date());
System.out.println(format6);
今天是:2023-06-15
注意:%t不能单独直接使用,必须跟随其他字母来指定时间格式
- %e:科学计数法
String format7 = String.format("%e", 950000000.1);
System.out.println(format7);
9.500000e+08
特殊使用方式
- 指定最小宽度
在 % 符号和占位符之间可以添加一个数字,该数字表示占位符的最小宽度。
- 如果需要填充该宽度的字符数量不足,则会自动右对齐,前面加-则号左对齐
- 如果超过该宽度,则保留所有字符。
String s1 = String.format("|%20s|", "Hello");
String s2 = String.format("|%-20s|", "Hello");
String s3 = String.format("|%20c|", 'A');
String s4 = String.format("|%20c|", 'A');
String s5 = String.format("|%20d|", 1);
String s6 = String.format("|%-20d|", 1);
String s7 = String.format("|%20f|", 1.0);
String s8 = String.format("|%-20f|", 1.0);
String s9 = String.format("|%-20b|", true);
String s10 = String.format("|%-20b|", true);
String s11 = String.format("|%-20tF|", new Date());
String s12 = String.format("|%-20tF|", new Date());
System.out.println(s1);
System.out.println(s2);
System.out.println(s3);
System.out.println(s4);
System.out.println(s5);
System.out.println(s6);
System.out.println(s7);
System.out.println(s8);
System.out.println(s9);
System.out.println(s10);
System.out.println(s11);
System.out.println(s12);
| Hello|
|Hello |
| A|
| A|
| 1|
|1 |
| 1.000000|
|1.000000 |
|true |
|true |
|2023-06-15 |
|2023-06-15 |
- 指定填充字符
可以在% 符号和占位符之间添加填充字符来指定要使用的字符。默认的填充字符为空格(上面指定最小宽度的示例就是填充的空格),支持的填充字:
- 空格:宽度不够时默认使用空格填充
- 0:宽度不够时可以使用0填充
- +:为正数或负数添加符号
- -:左对齐(默认是右对齐)
- ,:以组分隔符形式指定的数字(每 3 个数字一个逗号)
- #:对于二进制、八进制、十六进制等具有可选前缀的数字,会显示前缀
- .:精度或字符串截断点
- $:按顺序引用参数
String s1 = String.format("%10s", "hello");
String s2 = String.format("%010d", 42);
String s3 = String.format("%+d", 42);
String s4 = String.format("%-10s", "hello");
String s5 = String.format("%,d", 1234567890);
String s6 = String.format("%#x", 42);
String s7 = String.format("%.2f", 3.1415926);
String s8 = String.format("%1$s %2$s", "hello", "world");
System.out.println(s1);
System.out.println(s2);
System.out.println(s3);
System.out.println(s4);
System.out.println(s5);
System.out.println(s6);
System.out.println(s7);
System.out.println(s8);
hello
0000000042
+42
hello
1,234,567,890
0x2a
3.14
hello world
- 确定精度
对于浮点数类型和其它数字类型,可以使用精度来指定小数位数或有效数字位数。精度由一个点号(.)和数字来表示。
double f = 1234.56789;
String s1 = String.format("%.2f", f);
String s2 = String.format("%.4f", f);
String s3 = String.format("%+.2f", f);
System.out.println(s1);
System.out.println(s2);
System.out.println(s3);
1234.57
1234.5679
+1234.57
在这个例子中,.2f 表示保留两位小数,.4f 表示保留四位小数,而 %+.2f 表示保留两位小数,并在正数前面加上加号。
- 日期格式化
占位符 %t 后面可以跟随字母来表示时间格式。
- %tB:日期的月份,全名,例如:June
- %tb:日期的月份,缩写,例如:Jun
- %tm:日期的月份,两位数,例如:06
- %tY:4 位数的年份,例如:2023
- %ty:2 位数的年份,例如:23
- %tk:24 小时制的小时,不带前导零,例如:20
- %tH:24 小时制的小时,两位数,例如:20
- %tl:12 小时制的小时,不带前导零,例如:8
- %tI:12 小时制的小时,两位数,例如:08
- %tM:分钟,两位数,例如:30
- %tS:秒数,两位数,例如:45
- %tL:毫秒数,三位数,例如:886
- %tN:纳秒数,9 位数,例如:886456123
- %tp:上午/下午(大小写不同),例如:上午
- %tz:时区偏移量,例如:+0800
- %tZ:时区缩写,例如:CST
- %tj:1 年中的第几天,例如:166
- %tD:U.S.日期格式(MM/DD/YY),例如:06/15/23
- %tF:ISO 日期格式,例如:2023-06-15
- %tc:完整的日期和时间,例如:Wed Jun 15 07:32:24 CST 2023
- %tr:12 小时格式的时间(上午/下午),例如:02:45:30 下午
- %tR:24 小时格式的时间(格式为 HH:mm)
- %tT:24 小时格式的时间,例如:14:45:30
- %ts:从 1970 年 1 月 1 日 00:00:00 GMT 开始的秒数,例如:168412870
- %tQ:从 1970 年 1 月 1 日 00:00:00 GMT 开始的毫秒数,例如:168412870886
Calendar c = Calendar.getInstance();
Date date = c.getTime();
String s1 = String.format("%tB", date);
String s2 = String.format("%tb", date);
String s3 = String.format("%tm", date);
String s4 = String.format("%tY", date);
String s5 = String.format("%ty", date);
String s6 = String.format("%tk", date);
String s7 = String.format("%tH", date);
String s8 = String.format("%tl", date);
String s9 = String.format("%tI", date);
String s10 = String.format("%tM", date);
String s11 = String.format("%tS", date);
String s12 = String.format("%tL", date);
String s13 = String.format("%tN", date);
String s14 = String.format("%tp", date);
String s15 = String.format("%tz", date);
String s16 = String.format("%tZ", date);
String s17 = String.format("%tj", date);
String s18 = String.format("%tD", date);
String s20 = String.format("%tF", date);
String s21 = String.format("%tc", date);
String s22 = String.format("%tr", date);
String s23 = String.format("%tR", date);
String s24 = String.format("%tT", date);
String s25 = String.format("%ts", date);
String s26 = String.format("%tQ", date);
System.out.println("当前时间:"+DateUtil.format(date, "yyyy-MM-dd HH:mm:ss"));
System.out.println("%tB:" + s1);
System.out.println("%tb:" + s2);
System.out.println("%tm:" + s3);
System.out.println("%tY:" + s4);
System.out.println("%ty:" + s5);
System.out.println("%tk:" + s6);
System.out.println("%tH:" + s7);
System.out.println("%tl:" + s8);
System.out.println("%tI:" + s9);
System.out.println("%tM:" + s10);
System.out.println("%tS:" + s11);
System.out.println("%tL:" + s12);
System.out.println("%tN:" + s13);
System.out.println("%tp:" + s14);
System.out.println("%tz:" + s15);
System.out.println("%tZ:" + s16);
System.out.println("%tj:" + s17);
System.out.println("%tD:" + s18);
System.out.println("%tF:" + s20);
System.out.println("%tc:" + s21);
System.out.println("%tr:" + s22);
System.out.println("%tR:" + s23);
System.out.println("%tT:" + s24);
System.out.println("%ts:" + s25);
System.out.println("%tQ:" + s26);
当前时间:2023-06-15 16:04:26
%tB:六月
%tb:六月
%tm:06
%tY:2023
%ty:23
%tk:16
%tH:16
%tl:4
%tI:04
%tM:04
%tS:26
%tL:536
%tN:536000000
%tp:下午
%tz:+0800
%tZ:CST
%tj:166
%tD:06/15/23
%tF:2023-06-15
%tc:星期四 六月 15 16:04:26 CST 2023
%tr:04:04:26 下午
%tR:16:04
%tT:16:04:26
%ts:1686816266
%tQ:1686816266536
- 添加百分号%
要在格式化字符串中打印百分号 % 字符,可以使用 %% 来表示。
int n = 42;
String s = String.format("%d%%", n);
System.out.println(s);
42%
- 按顺序引用参数
指定参数的引用顺序,在%和字母中间添加[数字]$指定引用第几个参数。
String s = String.format("第二个参数是:%2$s,第三个参数是:%3$s,第一个参数是:%1$s", "hello", "world", "java");
System.out.println(s);
第二个参数是:world,第三个参数是:java,第一个参数是:hello
效率问题
String.format()相对于其他字符串拼接方法在格式化输出上具有较高的灵活性,但是在效率上的确不如其他拼接方式,例如用StringBuilder或者是StringBuffer拼接字符串。
-
在使用String.format()时,Java会创建一个新的字符串对象,该对象为格式化输出的结果,并将之返回给调用方。然而,在创建该字符串结果时,Java需要进行大量的字符串拼接和处理操作,因此这个过程会比较耗时。
-
相比之下,使用StringBuilder或者是StringBuffer拼接字符串时,我们可以一次性地将所有字符串拼接完成,避免了多次创建新的字符串对象,因此这种方式通常比使用String.format()更加高效地构建字符串。
在实际编程中,我们需要根据具体的业务场景和需求来选择最合适的字符串拼接方式,既要考虑效率问题,也要兼顾代码的可读性和可维护性。
更多推荐
所有评论(0)