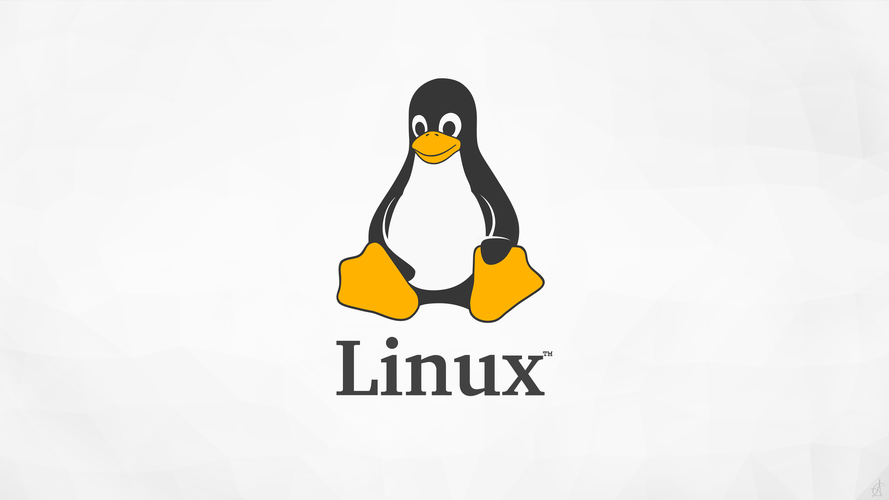
【Linux脚本篇】循环语句-for
前面讲的判断语句if和case只能做判断,可以说只能做一些判断。如果判断结果为假,将执行最后的命令(一般是exit退出脚本),如果不想退出,继续选从零开始小于10以内的次数,择正确的选项,就需要继续回到上面选择,这时就需要循环语句。

目录
🦐博客主页:大虾好吃吗的博客
🦐专栏地址:Linux从入门到精通
前面讲的判断语句if和case只能做判断,可以说只能做一些判断。如果判断结果为假,将执行最后的命令(一般是exit退出脚本),如果不想退出,继续选从零开始小于10以内的次数,择正确的选项,就需要继续回到上面选择,这时就需要循环语句。循环语句可以做类似于一级菜单、二级菜单的选项,直到选择正确的选项,最后可以退出脚本,这就是一个完整的程序。而循环语句有for和while两种,本章讲解的是for语句。
for的语法格式
for 变量名 in [取值列表]
do
循环命令语句
done
语法格式上来看,还是很简单的,我们在来几个简单的案例,让我们更加理解。
for循环案例
案例一:循环创建文件
目的是在根下filedir目录创建100个文件;在执行前先确定有没有该目录,所以就需要用到判断语句,如果没有这个目录则创建,有则不执行,
[root@daxia sh]# vim for1.sh
#!/bin/bash
text=/filedir
[ ! -d $text ] && mkdir $text
for I in {1..100}
do
touch ${text}/hehe$I
done
[root@daxia sh]# sh for1.sh
[root@daxia sh]# ls /filedir/
案例二:应用引号或转义符
for循环的取值比较复杂,我们可以使用引号或转义符。比如说下面的file1需要用单引号引起来,但是如果不使用转义符,系统会以为引起来的内容是一起的,不会打印出来单引号。而后面的hello beijing不用引号引起来,系统会以为是两个值,分开打印。
[root@daxia sh]# vim for2.sh
#!/bin/bash
for F in \'file1\' "hello beijing" file4 "hello world"
do
echo the text is $F
done
[root@daxia sh]# sh for2.sh
the text is 'file1'
the text is hello beijing
the text is file4
the text is hello world
案例三:从变量中取值
在循环里,取值列表可以是变量,先定一个变量,里面添加值,在for里面引用。
[root@daxia sh]# vim for3.sh
#!/bin/bash
List="file1 file2 file3"
for I in $List
do
echo "the text is ${I}"
done
[root@daxia sh]# sh for3.sh
the text is file1
the text is file2
the text is file3
案例四:从命令中取值
在命令中取值默认以空格为分隔符,可以使用IFS自定义分隔符。
[root@daxia sh]# vim for4.sh
#!/bin/bash
for H in $(cat /etc/hosts)
do
echo "$H"
done
[root@daxia sh]# sh for4.sh
127.0.0.1
localhost
localhost.localdomain
localhost4
localhost4.localdomain4
::1
localhost
localhost.localdomain
localhost6
localhost6.localdomain6
#以冒号做分隔符 IFS=: #以分号做分隔符 IFS=";" #以换行符做字段分隔符 IFS=$'\n'
我们可以看到上面,执行后,原本两行的文本,打印出了很多行。这就很不合理,我们后面把分隔符改成换行作为分隔符。
[root@daxia sh]# vim for4.sh
#!/bin/bash
IFS=$'\n'
for H in $(cat /etc/hosts)
do
echo "$H"
done
[root@daxia sh]# sh for4.sh
127.0.0.1 localhost localhost.localdomain localhost4 localhost4.localdomain4
::1 localhost localhost.localdomain localhost6 localhost6.localdomain6
案例五:for循环自增自减
我们来感受下自增的变化吧,根据上面几个案例,我们可以在值中写入多个用户,然后再循环创建。但是如果使用自增的话可以指定创建多少,并且不用写那么多的值。下面for语句中的i=1表示初始值为1,i<=10则是表示最多等于10或小于10,i++则是每次循环加一。这样我们就可以创建出十个不同数字的用户了,试想一下,自增还能给我们带来哪些便利呢?
[root@daxia sh]# vim for5.sh
#!/bin/bash
for (( i=1;i<=10;i++ ))
do
useradd sc$i
done
[root@daxia sh]# sh for5.sh
[root@daxia sh]# tail -10 /etc/passwd
sc1:x:1000:1000::/home/sc1:/bin/bash
sc2:x:1001:1001::/home/sc2:/bin/bash
sc3:x:1002:1002::/home/sc3:/bin/bash
sc4:x:1003:1003::/home/sc4:/bin/bash
sc5:x:1004:1004::/home/sc5:/bin/bash
sc6:x:1005:1005::/home/sc6:/bin/bash
sc7:x:1006:1006::/home/sc7:/bin/bash
sc8:x:1007:1007::/home/sc8:/bin/bash
sc9:x:1008:1008::/home/sc9:/bin/bash
sc10:x:1009:1009::/home/sc10:/bin/bash
同时输出1-9的升序和降序,注意:多个值之间是用逗号分隔,结束用分号分隔。
[root@daxia sh]# vim for5_1.sh
#!/bin/bash
for (( a=1,b=9;a<=10;a++,b-- ))
do
echo "num is $a $b"
done
[root@daxia sh]# sh for5_1.sh
num is 1 9
num is 2 8
num is 3 7
num is 4 6
num is 5 5
num is 6 4
num is 7 3
num is 8 2
num is 9 1
num is 10 0
用另外一种方式来实现升序和降序
[root@daxia sh]# vim for5_2.sh
#!/bin/bash
a=0
b=10
for i in {1..9}
do
let a++
let b--
echo "num is $a $b"
done
[root@daxia sh]# sh for5_2.sh
num is 1 9
num is 2 8
num is 3 7
num is 4 6
num is 5 5
num is 6 4
num is 7 3
num is 8 2
num is 9 1
案例六:批量创建用户
我们先看看RANDOM随机数生成命令,100表示最大生成的随机数,然后+1表示从1开始,如果不+1则随机数可能是0。
[root@daxia sh]# echo $((RANDOM % 100 + 1))
这次脚本写一个批量创建用户,提示用户输入前缀、数量,指定root用户才能执行脚本,密码随机(使用RANDOM命令),前缀不能为空。
[root@daxia sh]# vim for6.sh
#!/bin/bash
if [ ! $UID -eq 0 ] && [ $USER != "root" ];then
echo "你不是管理员,执行失败"
exit
fi
read -p "请输入你的用户前缀:" User_a
if [ -z $User_a ];then
echo "请输入有效的前缀"
exit
fi
read -p "请输入你的用户数量:" User_b
if [[ ! $User_b =~ ^[0-9]+$ ]];then
echo "请输入整数"
exit
fi
read -p "是否创建用户【y|n】:" yn
case $yn in
y)
for i in $(seq $User_b)
do
user=${User_a}$i
id $user &> /dev/null
if [ $? -eq 0 ];then
echo "useradd:user $user already exists"
else
user_pass=$(echo $((RANDOM)) | md5sum | cut -c 2-10 )
useradd $user
echo "$user_pass" | passwd --stdin $user &> /dev/null
echo "用户:$user 密码:$user_pass " >> /tmp/user.txt
echo "useradd:user $user add successfull,密码在/tmp/user.txt"
fi
done
;;
n)
exit
;;
*)
echo "error:请输入【y|n】:"
esac
[root@daxia sh]# sh for6.sh
请输入你的用户前缀:zhang
请输入你的用户数量:3
是否创建用户【y|n】:y
useradd:user zhang1 add successfull,密码在/tmp/user.txt
useradd:user zhang2 add successfull,密码在/tmp/user.txt
useradd:user zhang3 add successfull,密码在/tmp/user.txt
[root@daxia sh]# cat /tmp/user.txt
用户:zhang1 密码:29a106702
用户:zhang2 密码:6ded89b39
用户:zhang3 密码:b984e2b01
自行测试吧,切换用户测试非root用户(非0用户),执行脚本测试是否执行。然后修改脚本成批量删除用户,测试是否成功。
案例七:批量探测主机
批量检查主机(以8.0网段为例),并检查主机22端口是否开启。检查22端口需要使用nmap命令,需要提前安装。为了节省时间,这里就扫描三台主机了。
[root@daxia sh]# vim for7.sh
#!/bin/bash
for i in {100..102}
do
Ip=192.168.8.$i
ping -c 1 -w 1 $Ip &> /dev/null
if [ $? -eq 0 ];then
echo "$Ip is ok"
echo "$Ip" >> /tmp/ip.log
else
echo "$Ip is down"
fi
done
wait
echo "scan host ip is done"
echo "scan ssh port is starting"
for i in $(cat /tmp/ip.log)
do
nmap $i |grep "22" &> /dev/null
if [ $? -eq 0 ];then
echo "$i 22端口已开启"
echo "$i 22端口已开启" >> /tmp/port.log
fi
done
[root@daxia sh]# sh for7.sh
192.168.8.100 is ok
192.168.8.101 is down
192.168.8.102 is down
scan host ip is done
scan ssh port is starting
192.168.8.100 22端口已开启
192.168.8.100 22端口已开启
192.168.8.100 22端口已开启
案例八:随机点名
在一个文档中写入人名,通过过滤筛选出一个。先用wc命令过滤出student.txt中有几个人,来决定循环几次。在通过随机数计算出第几位,并使用sed命令确定人名,循环外面则是计算出的人名高亮显示。
[root@daxia sh]# cat student.txt
张三
李四
王五
马六
朱七
[root@daxia sh]# vim for8.sh
#!/bin/bash
num=$(wc -l student.txt|awk '{print $1}')
for i in {1..$num}
do
stu_num=$(( RANDOM % $num + 1 ))
sed -n "${stu_num}p" student.txt
sleep 0.1
done
name_stu=$(sed -n "${stu_num}p" student.txt)
echo -e "就是你了:\033[32m $name_stu \033[0m"
[root@daxia sh]# sh for8.sh
李四
就是你了: 李四
[root@daxia sh]# sh for8.sh
朱七
就是你了: 朱七
案例九:随机猜数字
[root@daxia sh]# vim for9.sh
#!/bin/bash
num=$(echo $(( RANDOM % 100 )))
i=0
while true
do
read -p "请输入一个随机数字【1-100】:" sz
if [[ ! $sz =~ ^[0-9]+$ ]];then
echo "请输入有效的整数"
continue
fi
if [ $sz -gt $num ];then
echo "你的数字过大"
elif [ $sz -lt $num ];then
echo "你的数字过小"
else
echo "恭喜,你猜对了!!!"
break
fi
let i++
done
echo "你总共猜了 $(( $i + 1 )) 次。"
[root@daxia sh]# sh for9.sh
请输入一个随机数字【1-100】:50
你的数字过小
请输入一个随机数字【1-100】:80
你的数字过大
请输入一个随机数字【1-100】:70
你的数字过大
请输入一个随机数字【1-100】:60
你的数字过小
请输入一个随机数字【1-100】:65
你的数字过大
请输入一个随机数字【1-100】:63
你的数字过大
请输入一个随机数字【1-100】:62
你的数字过大
请输入一个随机数字【1-100】:61
恭喜,你猜对了!!!
你总共猜了 8 次。
[root@daxia sh]#
创作不易,求关注,点赞,收藏,学习,谢谢~
更多推荐
所有评论(0)