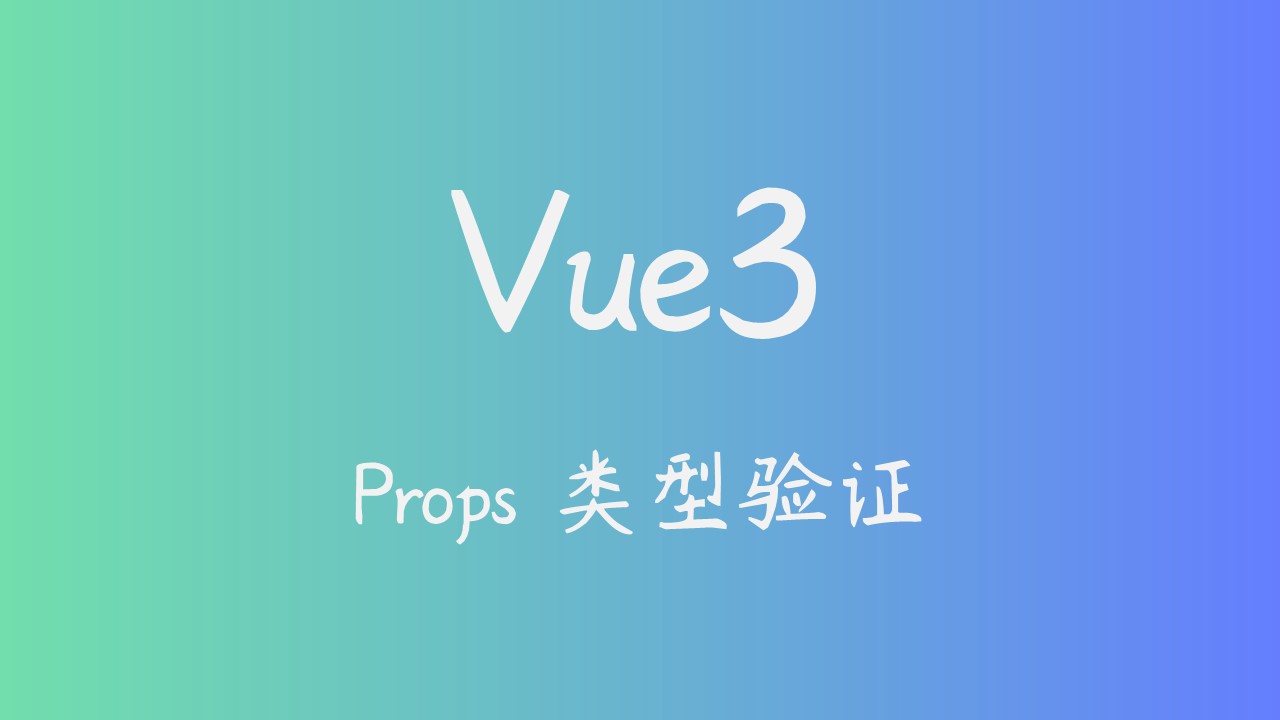
vue3 自定义 props 类型验证(复杂类型验证)
vue3,使用 PropType 自定义 Props 验证
·
分为:运行时声明 和 基于类型的声明
vue3官方文档
1,运行时声明
1.1,基础使用
<script setup lang="ts">
const props = defineProps({
foo: { type: String, required: true },
bar: Number
})
props.foo // string
props.bar // number | undefined
</script>
1.2,自定义类型验证
使用 PropType
工具类型进行自定义类型验证
PropType 工具类型,提供了一种类型安全的方式来定义和验证组件属性的类型。它使得开发者可以在编写组件时明确指定属性的类型要求,以确保组件在使用时能够得到正确的属性值,并提供更好的开发体验和错误检测。
换句话说,可以用自定义的接口或类型进行 props 验证。
<script setup lang="ts">
import type { PropType } from 'vue'
interface Book {
name: string
want: string
}
const props = defineProps({
book: Object as PropType<Book>
// 指定默认值
book: {
type: Object as PropType<Book>,
default: () => ({
name: '下雪天的夏风',
want: '求关注'
}),
},
})
</script>
我们也可以自定义一个函数
// utils.ts
import type { PropType } from 'vue'
export const definePropType = <T>(val: any): PropType<T> => val
使用:
<script setup lang="ts">
import { definePropType } from './utils'
interface Book {
name: string
want: string
}
const props = defineProps({
book: definePropType<Book>(Object)
})
</script>
1.3,默认值
const props = defineProps({
book: {
type: Object as PropType<Book>,
default: () => ({
name: '下雪天的夏风',
want: '求关注'
}),
},
})
或
const props = defineProps({
book: {
type: definePropType<Book>(Object),
default: () => ({
name: '下雪天的夏风',
want: '求关注'
}),
}
})
2,基于类型的声明
2.1,基础使用
通过泛型参数来定义 props 的类型
<script setup lang="ts">
const props = defineProps<{
foo: string // required
bar?: number // partial
}>()
</script>
注意:
标注是否必填的方式,和运行时声明不一样 foo: { type: String, required: true }
2.2,自定义类型验证
<script setup lang="ts">
import type { PropType } from 'vue'
interface Book {
name: string
want: string
}
const props = defineProps<{book: Book}>()
</script>
2.3,默认值
当使用基于类型的声明时,无法直接设置默认值。需要通过 withDefaults
编译器宏解决:
const props = withDefaults(defineProps<{book: Book}>(), {
book: () => ({
name: '下雪天的夏风',
want: '求关注'
})
})
注意:withDefaults
也会对默认值做类型检查!
interface Book {
name: string
want: string
}
const props = withDefaults(defineProps<{book: Book}>(), {
// 报错,类型 "{ name: string; want: string; }" 中缺少属性 "want",但类型 "Book" 中需要该属性。ts(2322)
book: () => ({
name: '下雪天的夏风'
})
})
以上。
更多推荐
所有评论(0)