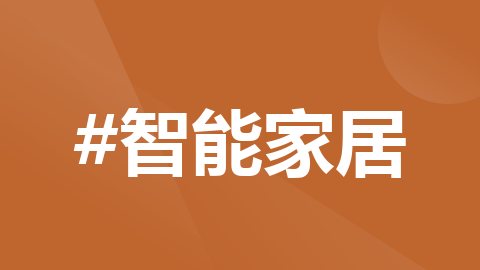
[ARM+Linux]智能家居项目
本次智能家居项目采用的是简化main函数代码,便于阅读。所有控制以及外设的设备都做成一个个对象,分别将命令控制的连成一个控制链表,外设设备做成一个外设设备的链表,这样做是为了方便以后功能模块的添加。其中为了能分别做好控制,我们采用多线程来实现。指令工厂:socket、语音控制(由于树莓派只有一个串口,所以我将Wemos D1作为服务器,树莓派作为客户端通过socket连接,实现指令控制)树莓派通过
本次智能家居项目采用的是简单工厂设计模式简化main函数代码,便于阅读。所有控制以及外设的设备都做成一个个对象,分别将命令控制的连成一个控制链表,外设设备做成一个外设设备的链表,这样做是为了方便以后功能模块的添加。其中为了能分别做好控制,我们采用多线程来实现。
指令工厂:
socket、语音控制(由于树莓派只有一个串口,所以我将Wemos D1作为服务器,树莓派作为客户端通过socket连接,实现指令控制)
设备工厂:
树莓派、语音模块、wemos D1、继电器组、摄像头、火焰传感器、电磁锁、蜂鸣器。
树莓派通过串口连接各语音模块LD2330,检测语音的识别结果,分析语音识别的结果来对家电设备进行控制。树莓派摄像头拍摄到人脸之后通过HTTPS访问翔云平台的人脸识别方案对比照片的base64编码来进行人脸识别开锁。由于语音模块占用了树莓派唯一的串口位,为了保留语音控制,所以,我将Wemos D1做成了服务器,让树莓派变为客户端使用socket和wemos进行连接。wemos通过读取树莓派作为客户端发送过来的数据来控制家电设备,完成了对于树莓派的接口拓展,以便控制更多的设备。
每个模块实现的步骤:
将所有设备和指令模块分别通过链表连接起来,以便于随意添加设备,指令工厂发出指令(通过语音模块----->串口\socket------->网络通信),会创建并进入相应的线程,线程中通过比较设备名来判断发出的指令是什么以及要控制哪个设备,再通过寻找设备名函数来定位设备,从而调用相应封装好的函数来实现相应的功能(我们所写的代码都是在用户态,需要在虚拟文件系统中实现系统调用sys_open,通过sys_call透过虚拟文件系统调动内核从而实现对硬件的控制)
mainPro.c
#include <stdlib.h>
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <pthread.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <unistd.h>
#include <sys/stat.h>
#include <fcntl.h>
#include "contrlDevices.h"
#include "inputCommand.h"
pthread_t voiceThread; //注意:定义线程不使用指针以免空指针异常
pthread_t socketThread; //注意:不建议线程传参(链表头)所以定为全局变量
pthread_t fireThread;
pthread_t cameraThread;
pthread_t clientWemosThread;
struct InputCommander *pCommandHead = NULL;
struct Devices *pdeviceHead = NULL;
struct InputCommander *socketHandler = NULL;
struct InputCommander *clientHandler = NULL;
pthread_mutex_t mutex; //定义互斥量(锁)
//pthread_cond_t cond; //条件
int c_fd; //注意:涉及到多线程不要轻易的去传参
//摄像头相关,改变返回值命名,因为C语言中没有这样的返回值
#define true 1
#define false 0
typedef unsigned int bool;
char buf[1024] = {'\0'};
struct Devices *findDeviceByName(char *name,struct Devices *phead) //查询设备
{
if(phead == NULL){
return NULL;
}
while(phead != NULL){
if(strstr(phead->deviceName,name) != NULL){
return phead;
}
phead = phead->next;
}
return NULL;
}
struct InputCommander *findCommandByName(char *name,struct InputCommander *phead) //查询控制
{
if(phead == NULL){
return NULL;
}
while(phead != NULL){
if(strcmp(phead->commandName,name) == 0){
return phead;
}
phead = phead->next;
}
return NULL;
}
void *voice_thread(void *arg) //语音线程
{
int i = 0;
int nread;
struct InputCommander *voiceHandler = NULL;
struct Devices *deviceTmp = NULL;
voiceHandler = findCommandByName("voice",pCommandHead); //在控制工厂找到语音模块
if(voiceHandler == NULL){
printf("find voiceHandler error\n");
pthread_exit(NULL);
}else{
if(voiceHandler->Init(voiceHandler,NULL,NULL) < 0){ //语音模块初始化
printf("voice init error\n");
pthread_exit(NULL); //退出线程
}else{
printf("%s init success\n",voiceHandler->commandName);
} //语音初始化完成
pthread_mutex_lock(&mutex); //加锁【有待研究】
//为什么加这个锁呢,我的想法是在语音读取一级指令的时候,为了避免其它线程对于 紧接着读取二级指令的干扰
while(1){
memset(voiceHandler->comand,'\0',sizeof(voiceHandler->comand));
nread = voiceHandler->getCommand(voiceHandler); //读取来自语音模块的串口数据
if(nread == 0){
//printf("noData from voice,please say again\n");
}else if(strstr(voiceHandler->comand,"all") != NULL){
printf("close all light\n");
deviceTmp = findDeviceByName("yu",pdeviceHead);
deviceTmp->close(deviceTmp->pinNum);
deviceTmp = findDeviceByName("ke",pdeviceHead);
deviceTmp->close(deviceTmp->pinNum);
deviceTmp = findDeviceByName("chu",pdeviceHead);
deviceTmp->close(deviceTmp->pinNum);
deviceTmp = findDeviceByName("shui",pdeviceHead);
deviceTmp->close(deviceTmp->pinNum);
}
else{
deviceTmp = findDeviceByName(voiceHandler->comand,pdeviceHead);
if(deviceTmp == NULL){
printf("findDeviceByName error\n");
}
else{
printf("findDevice = %s\n",deviceTmp->deviceName);
deviceTmp->deviceInit(deviceTmp->pinNum);
deviceTmp->open(deviceTmp->pinNum);
}
}
}
pthread_mutex_unlock(&mutex); //解锁
}
}
size_t readData1( void *ptr, size_t size, size_t nmemb, void *stream) //cameraContrlPostUrl函数里的回调函数
{
memset(buf,'\0',1024);
strncpy(buf,ptr,1024);
}
char *getBase641(char *picture) //获取照片64流
{
int fd;
int len;
char cmd[256] = {'\0'};
sprintf(cmd,"base64 %s > pictureBase64File",picture); //将照片的64流导入到一个文件中
system(cmd);
fd = open("./pictureBase64File",O_RDWR); //打开有照片64流的文件
len = lseek(fd,0,SEEK_END); //计算文件的大小(巧用lseek光标的移动)
lseek(fd,0,SEEK_SET); //光标回到首位置
char *readBuf = (char *)malloc(len); //如果不是用动态,当我们将这个readBuf指针返回去,则会段错误
read(fd,readBuf,len); //因为,readBuf是局部变量,静态内存,程序一运行完毕,里面的内容被释放(C语言基础)
close(fd);
system("rm ./pictureBase64File");
return readBuf;
}
bool cameraContrlPostUrl() //通过libcurl跨平台网络协议访问翔云人工智能平台人脸识别放案
{
CURL *curl;
CURLcode res;
char *postString;
struct Devices *deviceTmp = NULL;
//翔云人工智能平台人脸识别方案所需要的信息参数
char *img1;
char *img2;
char *key = "9ST43UmYcwtn8ZgWtHaXag";
char *secret = "f87c4f5c3bb94c25b728848b54c637a9";
int typeId = 21;
char *format = "xml";
chdir("/home/pi/mjpg-streamer/mjpg-streamer-experimental"); //改变当前文件路径
system("./start.sh"); //运行摄像头
chdir("/home/pi/yu/smartHome5_camera+face");
system("wget http://172.20.10.2:8080/?action=snapshot -O ./visitor.jpg"); //截图实时视频的照片
img1 = getBase641("./visitor.jpg"); //获取照片64流
img2 = getBase641("./tx.jpg");//与之前已经上传好的照片比对
int len = strlen(key)+strlen(secret)+strlen(img1)+strlen(img2)+124;
postString = (char *)malloc(strlen(key)+strlen(secret)+strlen(img1)+strlen(img2)+124);
memset(postString,'\0',len);
sprintf(postString,"&img1=%s&img2=%s&key=%s&secret=%s&typeId=%d&format=%s", //指定post内容,用$符号拼接,下面函数要发送到翔云去对比识别
img1,img2,key,secret,typeId,format);
system("rm visitor.jpg");
curl = curl_easy_init();
if (curl)
{
curl_easy_setopt(curl, CURLOPT_COOKIEFILE, "/tmp/cookie.txt"); // 指定cookie文件
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, postString); // ָ指定post内容,用$符号拼接
curl_easy_setopt(curl, CURLOPT_URL, "https://netocr.com/api/faceliu.do"); // ָ指定url
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, readData1); //将返回的http头输出到fp指向的文件
res = curl_easy_perform(curl);
printf("ok = %d\n",res);
curl_easy_cleanup(curl);
//printf("%s\n",buf);
if(strstr(buf,"是") != NULL){ //如果回调函数readData1中有“是”这个字,证明识别人脸成功是同一个人
deviceTmp = findDeviceByName("chu",pdeviceHead);
deviceTmp->open(deviceTmp->pinNum);
deviceTmp = findDeviceByName("yu",pdeviceHead);
deviceTmp->open(deviceTmp->pinNum);
deviceTmp = findDeviceByName("ke",pdeviceHead);
deviceTmp->open(deviceTmp->pinNum);
deviceTmp = findDeviceByName("shui",pdeviceHead);
deviceTmp->open(deviceTmp->pinNum);
}else{
printf("not a same of man\n");
}
}
return true;
}
void *read_thread(void *datas) //通过socket读取客户端发来的数据
{
int n_read;
struct Devices *deviceTmp = NULL;
while(1){
memset(socketHandler->comand,'\0',sizeof(socketHandler->comand));
n_read = read(c_fd,socketHandler->comand,sizeof(socketHandler->comand)); //读取客户端发来的数据
if(n_read == -1){
perror("read_thread");
}else if(n_read > 0){
//printf("getCommand:%s\n",socketHandler->comand);
//处理客户端读到的命令
if(strstr(socketHandler->comand,"op_shui") != NULL){
deviceTmp = findDeviceByName("shui",pdeviceHead);
deviceTmp->deviceInit(deviceTmp->pinNum);
deviceTmp->open(deviceTmp->pinNum);
}
if(strstr(socketHandler->comand,"cl_shui") != NULL){
deviceTmp = findDeviceByName("shui",pdeviceHead);
deviceTmp->deviceInit(deviceTmp->pinNum);
deviceTmp->close(deviceTmp->pinNum);
}
if(strstr(socketHandler->comand,"op_yu") != NULL){
deviceTmp = findDeviceByName("yu",pdeviceHead);
deviceTmp->deviceInit(deviceTmp->pinNum);
deviceTmp->open(deviceTmp->pinNum);
}
if(strstr(socketHandler->comand,"cl_yu") != NULL){
deviceTmp = findDeviceByName("yu",pdeviceHead);
deviceTmp->deviceInit(deviceTmp->pinNum);
deviceTmp->close(deviceTmp->pinNum);
}
if(strstr(socketHandler->comand,"op_ke") != NULL){
deviceTmp = findDeviceByName("ke",pdeviceHead);
deviceTmp->deviceInit(deviceTmp->pinNum);
deviceTmp->open(deviceTmp->pinNum);
}
if(strstr(socketHandler->comand,"cl_ke") != NULL){
deviceTmp = findDeviceByName("ke",pdeviceHead);
deviceTmp->deviceInit(deviceTmp->pinNum);
deviceTmp->close(deviceTmp->pinNum);
}
if(strstr(socketHandler->comand,"op_chu") != NULL){
deviceTmp = findDeviceByName("chu",pdeviceHead);
deviceTmp->deviceInit(deviceTmp->pinNum);
deviceTmp->open(deviceTmp->pinNum);
}
if(strstr(socketHandler->comand,"cl_chu") != NULL){
deviceTmp = findDeviceByName("chu",pdeviceHead);
deviceTmp->deviceInit(deviceTmp->pinNum);
deviceTmp->close(deviceTmp->pinNum);
}
if(strcmp(socketHandler->comand,"1") == 0){ //发送数据给wemos
memset(clientHandler,'\0',sizeof(clientHandler));
strcpy(clientHandler->comand,"1");
write(clientHandler->sfd,clientHandler->comand,strlen(clientHandler->comand));
}
if(strcmp(socketHandler->comand,"2") == 0){ //发送数据给wemos
memset(clientHandler,'\0',sizeof(clientHandler));
strcpy(clientHandler->comand,"2");
write(clientHandler->sfd,clientHandler->comand,strlen(clientHandler->comand));
}
if(strstr(socketHandler->comand,"face") != NULL){ //进行人脸识别
//deviceTmp = findDeviceByName("face",pdeviceHead);
//deviceTmp->cameraInit(deviceTmp);
cameraContrlPostUrl(); //调用人脸识别
}
if(strstr(socketHandler->comand,"cl_all") != NULL){
deviceTmp = findDeviceByName("chu",pdeviceHead);
deviceTmp->deviceInit(deviceTmp->pinNum);
deviceTmp->close(deviceTmp->pinNum);
deviceTmp = findDeviceByName("yu",pdeviceHead);
deviceTmp->deviceInit(deviceTmp->pinNum);
deviceTmp->close(deviceTmp->pinNum);
deviceTmp = findDeviceByName("ke",pdeviceHead);
deviceTmp->deviceInit(deviceTmp->pinNum);
deviceTmp->close(deviceTmp->pinNum);
deviceTmp = findDeviceByName("shui",pdeviceHead);
deviceTmp->deviceInit(deviceTmp->pinNum);
deviceTmp->close(deviceTmp->pinNum);
}
}
else{
printf("client quit\n");
exit(-1); //客户端退出,服务器程序退出
//pthread_exit(NULL); //退出线程
}
}
}
void *socket_thread(void *datas) //开启socket服务端,并将socket服务端初始化
{
int n_read = 0;
pthread_t readPthread;
struct sockaddr_in c_addr;
memset(&c_addr,0,sizeof(struct sockaddr_in));
int clen = sizeof(struct sockaddr_in);
socketHandler = findCommandByName("socketServer",pCommandHead); //在控制工厂找到socket
if(socketHandler == NULL){
printf("find socketHandler error\n");
pthread_exit(NULL);
}else{
printf("%s init success\n",socketHandler->commandName);
}
socketHandler->Init(socketHandler,NULL,NULL); //初始化socket
while(1){
c_fd = accept(socketHandler->sfd,(struct sockaddr *)&c_addr, &clen);
pthread_create(&readPthread,NULL,read_thread,NULL);
}
}
void *fire_thread(void *datas) //火灾线程
{
int status;
struct Devices *fireDeviceTmp = NULL;
struct Devices *buzzerDeviceTmp = NULL;
fireDeviceTmp = findDeviceByName("fire",pdeviceHead); //在设备工厂找到火灾模块
buzzerDeviceTmp = findDeviceByName("buzzser",pdeviceHead);
fireDeviceTmp->deviceInit(fireDeviceTmp->pinNum); //火灾模块初始化
buzzerDeviceTmp->deviceInit(buzzerDeviceTmp->pinNum);
printf("fire_thread init\n");
while(1){
delay(2000);
status = fireDeviceTmp->readStatus(fireDeviceTmp->pinNum); //读取火灾模块实时数据
//printf("fire get data = %d\n",status);
if(status == 0){
buzzerDeviceTmp->open(buzzerDeviceTmp->pinNum);
}else{
buzzerDeviceTmp->close(buzzerDeviceTmp->pinNum);
}
}
}
void *camera_thread(void *datas)
{
}
void *clientWemos_Thread(void *datas) //连接wemos线程
{
char *p;
//struct InputCommander *clientHandler = NULL; //放到全局,因为我要在socket那里接收用户客户端client的数据,然后发给wemos
//做客户端连接wemosD1服务器
clientHandler = findCommandByName("client",pCommandHead); //在控制工厂找到客户连接端
if(clientHandler == NULL){
printf("find clientHandler error\n");
exit(-1);
}else{
clientHandler->Init(clientHandler,NULL,NULL); //client初始化
}
while(1){ //获取服务端输入的命令数据,发送到wemos执行,这里只是调试作用,实际上我们需要接收的是客户端发来的数据。
memset(clientHandler,'\0',sizeof(clientHandler));
printf("input your contrl wemosD1 command::\n");
fgets(clientHandler->comand,sizeof(clientHandler->comand),stdin); //不用gets,有警告
if((p = strchr(clientHandler->comand,'\n')) != NULL)
*p = '\0'; //手动将\n位置处的值变为0
write(clientHandler->sfd,clientHandler->comand,strlen(clientHandler->comand)); //向wemosD1发送数据
}
}
int main()
{
char name[32] = {'\0'};
//树莓派库初始化
if(wiringPiSetup() == -1){
printf("硬件接口初始化失败\n");
return -1;
}
pthread_mutex_init(&mutex,NULL); //初始化互斥量(锁)
//pthread_cond_init(&cond,NULL); //条件的创建(动态初始化)
//1、指令工厂初始化
pCommandHead = addVoiceContrlToInputCommanderLink(pCommandHead);
pCommandHead = addsocketContrlToInputCommanderLink(pCommandHead);
pCommandHead = addclientContrlToInputCommanderLink(pCommandHead);
//2、设备控制工程初始化
pdeviceHead = addBathroomLightToDeviceLink(pdeviceHead);
pdeviceHead = addupStairLightToDeviceLink(pdeviceHead);
pdeviceHead = addlivingroomLightToDeviceLink(pdeviceHead);
pdeviceHead = addrestaurantLightToDeviceLink(pdeviceHead);
pdeviceHead = addFireToDeviceLink(pdeviceHead);
pdeviceHead = addBuzzerToDeviceLink(pdeviceHead);
//pdeviceHead = addcameraContrlToDeviceLink(pdeviceHead);
//int pthread_create(pthread_t *restrict tidp, const pthread_attr_t *restrict attr, void *(*start_rtn)(void *), void *restrict arg);
//3、线程池的建立
//3.1、语音线程
pthread_create(&voiceThread,NULL,voice_thread,NULL); //参数2:线程属性,一般设置为NULL,参数3:线程干活的函数,参数4:往voice_thread线程里面传送数据。
//3.2、socket服务器线程
pthread_create(&socketThread,NULL,socket_thread,NULL);
//3.3、火灾线程
pthread_create(&fireThread,NULL,fire_thread,NULL);
//3.4、摄像头线程
//pthread_create(&cameraThread,NULL,camera_thread,NULL);
//3.5、作为客户端连接wemosD1服务器
pthread_create(&clientWemosThread,NULL,clientWemos_Thread,NULL);
//等待线程
pthread_join(voiceThread,NULL);
pthread_join(socketThread,NULL);
pthread_join(fireThread,NULL);
pthread_join(cameraThread,NULL);
pthread_join(clientWemosThread,NULL);
pthread_mutex_destroy(&mutex); //销毁互斥量
//pthread_cond_destroy(&cond); //条件的销毁
/*开始创建设备工厂的调试代码
while(1){
printf("input:\n");
memset(name,'\0',sizeof(name));
gets(name);
tmp = findDeviceByName(name,pdeviceHead);
if(tmp != NULL){
tmp->deviceInit(tmp->pinNum);
tmp->open(tmp->pinNum);
}
}
*/
return 0;
}
设备工厂:
voice.c
/语音控制设备
#include <wiringPi.h>
#include <stdio.h>
#include <stdlib.h>
#include <wiringSerial.h>
#include <unistd.h>
#include <string.h>
#include "inputCommand.h"
int getCommand(struct InputCommander *voicer) //获取语音指令
{
int nread = 0;
memset(voicer->comand, '\0', sizeof(voicer->comand));
nread = read(voicer->fd, voicer->comand, sizeof(voicer->comand));
return nread;
}
//语音模块初始化
int voiceInit(struct InputCommander *voicer, char *ipAdress, char *port)
{
int fd;
if ((fd = serialOpen(voicer->deviceName, 9600)) == -1)
{
printf("语音初始化失败\n");
exit(-1);
}
voicer->fd = fd;
printf("语音初始化结束\n");
return fd;
}
//语音模块结构体
struct InputCommander voiceContrl = {
.commandName = "voice",
.deviceName = "/dev/ttyAMA0",
.comand = {'\0'},
.Init = voiceInit,
.getCommand = getCommand,
.log = {'\0'},
.next = NULL};
struct InputCommander *addVoiceContrlToInputCommanderLink(struct InputCommander *phead)
{
if (phead == NULL)
{
return &voiceContrl;
}
else
{
voiceContrl.next = phead;
phead = &voiceContrl;
}
return phead;
}
bathroomLight.c (浴室灯):
#include "contrlDevices.h"
int bathroomLightOpen(int pinNum)
{
digitalWrite (pinNum,LOW);
}
int bathroomLightClose(int pinNum)
{
digitalWrite (pinNum,HIGH);
}
int bathroomLightCloseInit(int pinNum)
{
pinMode (pinNum, OUTPUT);
digitalWrite (pinNum,HIGH);
}
int bathroomLightCloseStatus(int status)
{
}
struct Devices bathroomLight = {
//.deviceName = "bathroomLight",
.deviceName = "yu",
.pinNum = 22,
.open = bathroomLightOpen,
.close = bathroomLightClose,
.deviceInit = bathroomLightCloseInit,
.changStatus = bathroomLightCloseStatus
};
struct Devices *addBathroomLightToDeviceLink(struct Devices *phead)
{
if(phead == NULL){
return &bathroomLight;
}else{
bathroomLight.next = phead;
phead = &bathroomLight;
}
return phead;
}
livingroomLight.c (睡房灯):
#include "contrlDevices.h"
int livingroomLightOpen(int pinNum)
{
digitalWrite (pinNum,LOW);
}
int livingroomLightClose(int pinNum)
{
digitalWrite (pinNum,HIGH);
}
int livingroomLightCloseInit(int pinNum)
{
pinMode (pinNum, OUTPUT);
digitalWrite (pinNum,HIGH);
}
int livingroomLightCloseStatus(int status)
{
}
struct Devices livingroomLight = {
//.deviceName = "livingroomLight",
.deviceName = "shui",
.pinNum = 21,
.open = livingroomLightOpen,
.close = livingroomLightClose,
.deviceInit = livingroomLightCloseInit,
.changStatus = livingroomLightCloseStatus
};
struct Devices *addlivingroomLightToDeviceLink(struct Devices *phead)
{
if(phead == NULL){
return &livingroomLight;
}else{
livingroomLight.next = phead;
phead = &livingroomLight;
}
return phead;
}
restaurantLight.c (厨房灯)
#include "contrlDevices.h"
int restaurantLightOpen(int pinNum)
{
digitalWrite (pinNum,LOW);
}
int restaurantLightClose(int pinNum)
{
digitalWrite (pinNum,HIGH);
}
int restaurantLightCloseInit(int pinNum)
{
pinMode (pinNum, OUTPUT);
digitalWrite (pinNum,HIGH);
}
int restaurantLightCloseStatus(int status)
{
}
struct Devices restaurantLight = {
//.deviceName = "restaurantLight",
.deviceName = "chu",
.pinNum = 23,
.open = restaurantLightOpen,
.close = restaurantLightClose,
.deviceInit = restaurantLightCloseInit,
.changStatus = restaurantLightCloseStatus
};
struct Devices *addrestaurantLightToDeviceLink(struct Devices *phead)
{
if(phead == NULL){
return &restaurantLight;
}else{
restaurantLight.next = phead;
phead = &restaurantLight;
}
return phead;
}
upstairLight.c (客厅灯)
#include "contrlDevices.h"
int upStairLightOpen(int pinNum)
{
digitalWrite (pinNum,LOW);
}
int upStairLightClose(int pinNum)
{
digitalWrite (pinNum,HIGH);
}
int upStairLightCloseInit(int pinNum)
{
pinMode (pinNum, OUTPUT);
digitalWrite (pinNum,HIGH);
}
int upStairLightCloseStatus(int status)
{
}
struct Devices upStairLight = {
//.deviceName = "upStairLight",
.deviceName = "ke",
.pinNum = 24,
.open = upStairLightOpen,
.close = upStairLightClose,
.deviceInit = upStairLightCloseInit,
.changStatus = upStairLightCloseStatus
};
struct Devices *addupStairLightToDeviceLink(struct Devices *phead)
{
if(phead == NULL){
return &upStairLight;
}else{
upStairLight.next = phead;
phead = &upStairLight;
}
return phead;
}
buzzer.c(蜂鸣器)
#include "contrlDevices.h"
int buzzerOpen(int pinNum)
{
digitalWrite (pinNum,LOW);
}
int buzzerClose(int pinNum)
{
digitalWrite (pinNum,HIGH);
}
int buzzerInit(int pinNum)
{
pinMode (pinNum, OUTPUT);
digitalWrite (pinNum,HIGH);
}
struct Devices buzzer = {
.deviceName = "buzzser",
.pinNum = 7,
.open = buzzerOpen,
.close = buzzerClose,
.deviceInit = buzzerInit
};
struct Devices *addBuzzerToDeviceLink(struct Devices *phead)
{
if(phead == NULL){
return &buzzer;
}else{
buzzer.next = phead;
phead = &buzzer;
}
return phead;
}
fire.c (火焰传感器)
#include "contrlDevices.h"
int fireInit(int pinNum)
{
pinMode (pinNum,INPUT);
digitalWrite (pinNum,HIGH);
}
int readFireStatus(int pinNum)
{
return digitalRead(pinNum);
}
struct Devices fire = {
.deviceName = "fire",
.pinNum = 25,
.deviceInit = fireInit,
.readStatus = readFireStatus
};
struct Devices *addFireToDeviceLink(struct Devices *phead)
{
if(phead == NULL){
return &fire;
}else{
fire.next = phead;
phead = &fire;
}
return phead;
}
camera.c (摄像头)
#include "contrlDevices.h"
#include <string.h>
#include <stdlib.h>
#include <sys/types.h>
#include <unistd.h>
#include <sys/stat.h>
#include <fcntl.h>
#define true 1
#define false 0
typedef unsigned int bool;
char resultBuf[1024] = {'\0'};
size_t readData( void *ptr, size_t size, size_t nmemb, void *stream) //回调函数
{
strncpy(resultBuf,ptr,1024);
//printf("%s\n",resultBuf);
}
char *getBase64(char *picture) //获取图片的base64流
{
int fd;
int len;
char cmd[256] = {'\0'};
sprintf(cmd,"base64 %s > pictureBase64File",picture);
system(cmd);
fd = open("./pictureBase64File",O_RDWR);
len = lseek(fd,0,SEEK_END);
lseek(fd,0,SEEK_SET);
char *readBuf = (char *)malloc(len); //如果不是用动态,当我们将这个readBuf指针返回去,则会段错误
read(fd,readBuf,len); //因为,readBuf是局部变量,静态内存,程序一运行完毕,里面的内容被释放(C语言基础)
close(fd);
system("rm ./pictureBase64File");
return readBuf;
}
bool cameraContrl_postUrl(struct Devices *camera)
{
CURL *curl;
CURLcode res;
char *postString;
char *img1;
char *img2;
system("raspistill -w 700 -h 525 -o ./visitor.jpg");
img1 = getBase64("./visitor.jpg");
img2 = getBase64("./me1.jpg");
int len = strlen(camera->key)+strlen(camera->secret)+strlen(img1)+strlen(img2)+124;
postString = (char *)malloc(strlen(camera->key)+strlen(camera->secret)+strlen(img1)+strlen(img2)+124);
memset(postString,'\0',len);
sprintf(postString,"&img1=%s&img2=%s&key=%s&secret=%s&typeId=%d&format=%s",
img1,img2,camera->key,camera->secret,camera->typeId,camera->format);
system("rm visitor.jpg");
curl = curl_easy_init();
if (curl)
{
curl_easy_setopt(curl, CURLOPT_COOKIEFILE, "/tmp/cookie.txt"); // 指定cookie文件
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, postString); // ָ指定post内容,用$符号拼接
curl_easy_setopt(curl, CURLOPT_URL, "https://netocr.com/api/faceliu.do"); // ָ指定url
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, readData1); //将返回的http头输出到fp指向的文件
res = curl_easy_perform(curl);
res = curl_easy_perform(curl);
//printf("ok = %d\n",res);
printf("%s\n",resultBuf);
if(strstr(resultBuf,"是") != NULL){
printf("ok\n");
}else{
printf("no\n");
}
curl_easy_cleanup(curl);
}
return true;
}
struct Devices cameraContrl = {
.deviceName = "camera",
.key = "9ST43UmYcwtn8ZgWtHaXag",
.secret = "f87c4f5c3bb94c25b728848b54c637a9",
.typeId = 21,
.format = "xml",
.cameraInit = cameraContrl_postUrl,
.next = NULL
};
struct Devices *addcameraContrlToDeviceLink(struct Devices *phead)
{
if(phead == NULL){
return &cameraContrl;
}else{
cameraContrl.next = phead;
phead = &cameraContrl;
}
return phead;
}
client_wemos.c (树莓派作为客户端 连接wemos D1服务器)
#include <wiringPi.h>
#include <stdio.h>
#include <stdlib.h>
#include <wiringSerial.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <string.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include "inputCommand.h"
int clientInit(struct InputCommander *client,char *ipAdress,char *port)
{
struct sockaddr_in addr;
memset(&addr,0,sizeof(struct sockaddr_in));
//1.socket 创建套接字
int s_fd=socket(AF_INET, SOCK_STREAM,0);
if (s_fd==-1)
{
perror("socket");
exit(-1);
}
addr.sin_family=AF_INET;
addr.sin_port=htons(atoi(client->port));
inet_aton(client->ipAdress,&addr.sin_addr);
//2.connect 连接服务器
if(connect(s_fd,(struct sockaddr *)&addr,sizeof(struct sockaddr))==-1) //这里是连接wemosD1
{
perror("connect");
exit(-1);
}
printf("client wemosD1 connect....\n");
client->sfd = s_fd;
return s_fd;
}
struct InputCommander clientContrl = {
.commandName = "client", //加入到控制链表的名字
.comand = {'\0'}, //命令
.port = "8888", //wemosD1 端口号
.ipAdress = "192.168.43.36", //wemosD1 IP地址
.Init = clientInit, //树莓派客户端初始化
.next = NULL
};
struct InputCommander *addclientContrlToInputCommanderLink(struct InputCommander *phead)
{
if(phead == NULL){
return &clientContrl;
}else{
clientContrl.next = phead;
phead = &clientContrl;
}
return phead;
}
socketContrl.c (socket控制)
#include <wiringPi.h>
#include <stdio.h>
#include <stdlib.h>
#include <wiringSerial.h>
#include <unistd.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <arpa/inet.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <string.h>
#include <sys/types.h>
#include <sys/stat.h>
#include <fcntl.h>
#include "inputCommand.h"
int socketgetCommand(struct InputCommander *socketMes)
{
int c_fd;
int n_read;
struct sockaddr_in c_addr;
memset(&c_addr,0,sizeof(struct sockaddr_in));
int clen = sizeof(struct sockaddr_in);
//4.accept
c_fd = accept(socketMes->sfd,(struct sockaddr *)&c_addr, &clen);
n_read = read(c_fd,socketMes->comand,sizeof(socketMes->comand));
if(n_read == -1){
perror("read");
}else if(n_read > 0){
printf("\nget:%d\n",n_read);
}else{
printf("client quit\n");
}
return n_read;
}
int socketInit(struct InputCommander *socketMes,char *ipAdress,char *port)
{
/*形参虽然定多了,用不上,咱不管*/
int s_fd;
struct sockaddr_in s_addr;
memset(&s_addr,0,sizeof(struct sockaddr_in));
//1.socket
s_fd = socket(AF_INET,SOCK_STREAM,0);
if(s_fd == -1){
perror("socked");
exit(-1);
}
s_addr.sin_family = AF_INET;
s_addr.sin_port = htons(atoi(socketMes->port));
inet_aton(socketMes->ipAdress,&s_addr.sin_addr);
//2.bind
bind(s_fd, (struct sockaddr *)&s_addr,sizeof(struct sockaddr_in));
//3.listen
listen(s_fd,10);
printf("socket Server listening......\n");
socketMes->sfd = s_fd;
return s_fd;
}
struct InputCommander socketContrl = {
.commandName = "socketServer",
.comand = {'\0'},
.port = "8083",
.ipAdress = "172.20.10.2",
.Init = socketInit,
.getCommand = socketgetCommand,
.log = {'\0'},
.next = NULL
};
struct InputCommander *addsocketContrlToInputCommanderLink(struct InputCommander *phead)
{
if(phead == NULL){
return &socketContrl;
}else{
socketContrl.next = phead;
phead = &socketContrl;
}
return phead;
}
voiceContrl.c(语音控制设备)
#include <wiringPi.h>
#include <stdio.h>
#include <stdlib.h>
#include <wiringSerial.h>
#include <unistd.h>
#include <string.h>
#include "inputCommand.h"
int getCommand(struct InputCommander *voicer)
{
int nread = 0;
memset(voicer->comand,'\0',sizeof(voicer->comand));
nread = read(voicer->fd,voicer->comand,sizeof(voicer->comand));
return nread;
}
int voiceInit(struct InputCommander *voicer,char *ipAdress,char *port)
{
/*形参虽然定多了,用不上,咱不管*/
int fd;
if((fd = serialOpen(voicer->deviceName,9600)) == -1){
exit(-1);
}
voicer->fd = fd;
return fd;
}
struct InputCommander voiceContrl = {
.commandName = "voice",
.deviceName = "/dev/ttyAMA0",
//.boTelv = "9600";
.comand = {'\0'},
.Init = voiceInit,
.getCommand = getCommand,
.log = {'\0'},
.next = NULL
};
struct InputCommander *addVoiceContrlToInputCommanderLink(struct InputCommander *phead)
{
if(phead == NULL){
return &voiceContrl;
}else{
voiceContrl.next = phead;
phead = &voiceContrl;
}
return phead;
}
头文件
contrlDevices.h(外设设备的头文件)
#include <wiringPi.h>
#include <stdio.h>
#include <curl/curl.h>
typedef unsigned int bool;
struct Devices
{
char deviceName[128]; //设备名
int status; //读取到的数据
int pinNum; //引脚号
int (*open)(int pinNum); //打开设备函数指针
int (*close)(int pinNum); //关闭设备函数指针
int (*deviceInit)(int pinNum); //设备初始化函数指针
int (*readStatus)(int pinNum); //读取数据函数指针
int (*changStatus)(int status); //改变数据函数指针
//摄像头相关的
CURL *curl;
char *key;
char *secret;
int typeId;
char *format;
bool (*cameraInit)(struct Devices *camera);
int yesNum;
int noNum;
struct Devices *next;
};
//每个设备加到链表函数的声明
struct Devices *addBathroomLightToDeviceLink(struct Devices *phead);
struct Devices *addupStairLightToDeviceLink(struct Devices *phead);
struct Devices *addlivingroomLightToDeviceLink(struct Devices *phead);
struct Devices *addrestaurantLightToDeviceLink(struct Devices *phead);
struct Devices *addFireToDeviceLink(struct Devices *phead);
struct Devices *addBuzzerToDeviceLink(struct Devices *phead);
struct Devices *addcameraContrlToDeviceLink(struct Devices *phead);
inputCommand.h (控制的头文件)
#include <wiringPi.h>
#include <stdio.h>
struct InputCommander
{
char commandName[128]; //socket名
char deviceName[128]; //串口名
char comand[32]; //控制命令
int (*Init)(struct InputCommander *voicer,char *ipAdress,char *port); //socket初始化
int (*getCommand)(struct InputCommander *voicer); //读取数据
char log[1024];
int fd;
char port[12]; //端口号
char ipAdress[32]; //IP地址
int sfd;
int cfd;
struct InputCommander *next;
};
//每个控制添加到控制链表的函数声明
struct InputCommander *addVoiceContrlToInputCommanderLink(struct InputCommander *phead);
struct InputCommander *addsocketContrlToInputCommanderLink(struct InputCommander *phead);
struct InputCommander *addclientContrlToInputCommanderLink(struct InputCommander *phead);
本次项目过程还是比较艰辛,因为脑子里面始终不能有一个完整的项目流程,对简单工厂模式还不够熟悉,还有就是在使用多线程时,极易出现段错误,段错误的产生呢主要是
1.访问不存在的内存地址:
野指针没有开辟空间就往里面拷贝
2.访问只读的内存地址:
错误做法:往字符串常量空间拷贝新的数据
3.访问系统保护的内存地址
4.栈溢出
我遇到很多次段错误,大部分就是没有动态创建指针地址,没有开辟空间就去赋值造成的
我的解决方法就是不断地去printf打印调试信息从而去找到出现段错误的地方
更多推荐
所有评论(0)