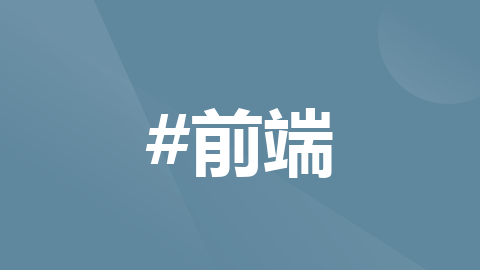
Vue3面试题:20道含答案和代码示例的练习题
答:Composition API是Vue3中新增的一种API,它可以让开发者更加灵活地组织和重用组件逻辑。答:Vue3中使用Proxy对象来实现响应式数据。计算属性可以根据响应式数据的变化自动更新。方法来监听响应式数据的变化,并在数据变化时自动执行函数。方法来监听响应式数据的变化,并在数据变化时执行回调函数。方法来创建一个响应式数据对象。方法将一个响应式数据对象的属性转换为响应式数据引用。方法来

- Vue3中响应式数据的实现原理是什么?
答:Vue3中使用Proxy对象来实现响应式数据。当数据发生变化时,Proxy会自动触发更新。
const state = {
count: 0
}
const reactiveState = new Proxy(state, {
set(target, key, value) {
target[key] = value
console.log('数据已更新')
return true
}
})
reactiveState.count = 1 // 数据已更新
- Vue3中的Composition API是什么?
答:Composition API是Vue3中新增的一种API,它可以让开发者更加灵活地组织和重用组件逻辑。
import { reactive, computed } from 'vue'
export default {
setup() {
const state = reactive({
count: 0
})
const doubleCount = computed(() => state.count * 2)
function increment() {
state.count++
}
return {
state,
doubleCount,
increment
}
}
}
- Vue3中如何使用自定义指令?
答:Vue3中使用app.directive
方法来定义自定义指令。自定义指令需要返回一个对象,其中包含指令钩子函数。
import { createApp } from 'vue'
const app = createApp({})
app.directive('focus', {
mounted(el) {
el.focus()
}
})
<template>
<input v-focus>
</template>
- Vue3中如何使用Teleport组件?
答:Vue3中使用<teleport>
组件来实现Portal功能。需要将<teleport>
组件放在需要传送的目标位置,并指定to
属性。
<template>
<button @click="showDialog = true">Show Dialog</button>
<teleport to="body">
<dialog v-if="showDialog">
<h1>Dialog Title</h1>
<p>Dialog Content</p>
<button @click="showDialog = false">Close</button>
</dialog>
</teleport>
</template>
- Vue3中如何使用Suspense组件?
答:Vue3中使用<suspense>
组件来实现异步组件加载时的占位符。需要在<suspense>
组件中使用<template v-slot>
指定占位符内容。
<template>
<suspense>
<template v-slot:fallback>
<div>Loading...</div>
</template>
<async-component />
</suspense>
</template>
- Vue3中如何使用provide和inject实现跨层级组件通信?
答:Vue3中使用provide
和inject
方法来实现跨层级组件通信。在父组件中使用provide
方法提供数据,在子组件中使用inject
方法注入数据。
import { provide, inject } from 'vue'
const Parent = {
setup() {
const state = reactive({
count: 0
})
provide('state', state)
return {
state
}
}
}
const Child = {
setup() {
const state = inject('state')
return {
state
}
}
}
- Vue3中如何使用watchEffect方法?
答:Vue3中使用watchEffect
方法来监听响应式数据的变化,并在数据变化时自动执行函数。
import { watchEffect } from 'vue'
const state = reactive({
count: 0
})
watchEffect(() => {
console.log(state.count)
})
- Vue3中如何使用watch方法?
答:Vue3中使用watch
方法来监听响应式数据的变化,并在数据变化时执行回调函数。
import { watch } from 'vue'
const state = reactive({
count: 0
})
watch(() => state.count, (newValue, oldValue) => {
console.log(newValue, oldValue)
})
- Vue3中如何使用computed方法?
答:Vue3中使用computed
方法来创建计算属性。计算属性可以根据响应式数据的变化自动更新。
import { computed } from 'vue'
const state = reactive({
count: 0
})
const doubleCount = computed(() => state.count * 2)
console.log(doubleCount.value) // 0
state.count = 1
console.log(doubleCount.value) // 2
- Vue3中如何使用ref方法?
答:Vue3中使用ref
方法来创建一个响应式数据的引用。引用的值可以通过.value
访问和修改。
import { ref } from 'vue'
const count = ref(0)
console.log(count.value) // 0
count.value++
console.log(count.value) // 1
- Vue3中如何使用reactive方法?
答:Vue3中使用reactive
方法来创建一个响应式数据对象。对象的属性会自动监听变化。
import { reactive } from 'vue'
const state = reactive({
count: 0
})
console.log(state.count) // 0
state.count++
console.log(state.count) // 1
- Vue3中如何使用toRefs方法?
答:Vue3中使用toRefs
方法将一个响应式数据对象的属性转换为响应式数据引用。
import { reactive, toRefs } from 'vue'
const state = reactive({
count: 0
})
const refs = toRefs(state)
console.log(refs.count.value) // 0
state.count++
console.log(refs.count.value) // 1
- Vue3中如何使用createApp方法创建一个Vue实例?
答:Vue3中使用createApp
方法来创建一个Vue实例,并使用mount
方法将实例挂载到DOM上。
import { createApp } from 'vue'
import App from './App.vue'
const app = createApp(App)
app.mount('#app')
- Vue3中如何使用defineComponent方法定义一个组件?
答:Vue3中使用defineComponent
方法来定义一个组件,并使用setup
方法来编写组件逻辑。
import { defineComponent } from 'vue'
export default defineComponent({
props: {
name: String
},
setup(props) {
const message = `Hello, ${props.name}!`
return {
message
}
}
})
- Vue3中如何使用provide和inject方法实现全局状态管理?
答:Vue3中使用provide
和inject
方法实现全局状态管理。可以在根组件中使用provide
方法提供全局状态,在其他组件中使用inject
方法注入全局状态。
import { provide, inject } from 'vue'
const app = createApp(...)
const globalState = reactive({
count: 0
})
app.provide('globalState', globalState)
const Child = {
setup() {
const state = inject('globalState')
return {
state
}
}
}
- Vue3中如何使用createRouter方法创建一个路由实例?
答:Vue3中使用createRouter
方法创建一个路由实例,并使用use
方法将路由实例安装到Vue实例中。
import { createRouter, createWebHashHistory } from 'vue-router'
const router = createRouter({
history: createWebHashHistory(),
routes: [
{
path: '/',
component: Home
},
{
path: '/about',
component: About
}
]
})
const app = createApp(...)
app.use(router)
- Vue3中如何使用createStore方法创建一个Vuex实例?
答:Vue3中使用createStore
方法创建一个Vuex实例,并使用provide
方法将实例注入到Vue实例中。
import { createStore } from 'vuex'
const store = createStore({
state: {
count: 0
},
mutations: {
increment(state) {
state.count++
}
}
})
const app = createApp(...)
app.provide('store', store)
const Child = {
setup() {
const store = inject('store')
return {
store
}
}
}
- Vue3中如何使用defineAsyncComponent方法创建一个异步组件?
答:Vue3中使用defineAsyncComponent
方法创建一个异步组件,并使用import
语句加载组件文件。
import { defineAsyncComponent } from 'vue'
const AsyncComponent = defineAsyncComponent(() => import('./AsyncComponent.vue'))
export default {
components: {
AsyncComponent
}
}
- Vue3中如何使用createSSRApp方法创建一个服务端渲染实例?
答:Vue3中使用createSSRApp
方法创建一个服务端渲染实例,并使用renderToString
方法将实例渲染为HTML字符串。
import { createSSRApp } from 'vue'
import App from './App.vue'
const app = createSSRApp(App)
const html = await renderToString(app)
- Vue3中如何使用withModifiers方法为事件添加修饰符?
答:Vue3中使用withModifiers
方法为事件添加修饰符。可以在事件名后面使用.
符号指定修饰符。
<template>
<button @click.prevent="">Click</button>
</template>
import { withModifiers } from 'vue'
const handleClick = withModifiers(() => {
console.log('clicked')
}, ['prevent'])
更多推荐
所有评论(0)