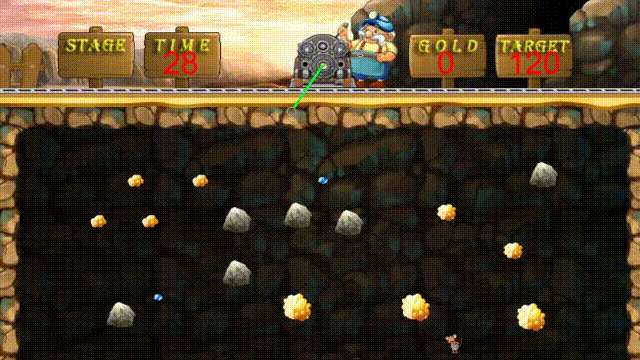
unity黄金矿工
经典黄金矿工玩法,绳子左右摇摆,鼠标点击发射钩子。
·
一、 介绍
经典黄金矿工玩法,绳子左右摇摆,鼠标点击发射钩子
二、 知识点
枚举
动画制作
设置父对象 other.transform.parent = transform;
text组件
时间的控制:使用 Time.time
三、
四、 碰撞检测
挂在钩子上,钩住东西后,禁用碰撞器
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CheckObjects : MonoBehaviour {
public void OnTriggerEnter2D(Collider2D other)
{
other.transform.parent = transform; // 把 other 对象的父物体设置为当前物体
transform.root.GetComponent<GameManage>().GetState = State.Shorten; // 获取当前物体的根物体上的 GameManage 组件,将其 GetState 属性设置为 State.Shorten
for (int i = 0; i < transform.childCount; i++) // 遍历当前物体的所有子物体
{
transform.GetChild(i).GetComponent<Collider2D>().enabled = false; // 将当前物体的第 i 个子物体的 Collider2D 组件的 enabled 属性设置为 false,从而禁用它的碰撞器
}
transform.GetComponent<Collider2D>().enabled = false; // 禁用当前物体的碰撞器
}
}
五、 游戏控制器
枚举,绳子有三种形态:摇摆、拉伸、收缩
设置目标分数面板
设置当前分数面板
设置时间面板
设置奖励:大金子、小金子、钻石、野猪
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public enum State // 定义一个名为 State 的枚举类型,表示状态
{
Rock, // 摇摆
Stretch, // 拉伸
Shorten // 缩短
}
public class GameManage : MonoBehaviour {
private State state; // 状态
private Vector3 dir; // 方向
private Transform rope; // 绳子
private Transform ropeCord; // 绳索
private float length; // 长度
private int grade; // 分数
private float times; // 时间
public Text timeText; // 显示时间的 Text 组件
public Text gradeText; // 显示分数的 Text 组件
public State GetState // 获取状态,为属性
{
set { state = value; }
get { return state ; }
}
void Start () { // 在 Start 函数中初始化变量
state = State.Rock;
dir = Vector3.back;
rope = transform.GetChild(0);
ropeCord = rope.GetChild(0);
length = 1;
times = 30;
grade = 0;
}
void Update () { // 在 Update 函数中更新游戏状态
if (times <= 0) { times = 0; UnityEditor.EditorApplication.isPlaying = false; return; } // 如果时间到了,退出游戏
if (state == State.Rock) // 如果当前状态是 Rock
{
Rock(); // 调用 Rock 函数
if (Input.GetMouseButtonDown(0)) state = State.Stretch; // 如果鼠标左键按下,将状态设置为 Stretch
}
else if (state == State.Stretch) // 如果当前状态是 Stretch
{
Stretch(); // 调用 Stretch 函数
}
else if (state == State.Shorten) // 如果当前状态是 Shorten
{
Shorten(); // 调用 Shorten 函数
}
times -= Time.deltaTime; // 时间减少
timeText.text = ((int)times).ToString(); // 更新时间的 Text 组件
gradeText.text = grade.ToString(); // 更新分数的 Text 组件
}
private void Rock() // 在 Rock 函数中控制绳子的摆动
{
if (rope.localRotation.z <= -0.5f) // 如果绳子摆到了一定角度
dir = Vector3.forward; // 改变绳子摆动的方向
else if (rope.localRotation.z >= 0.5f)
dir = Vector3.back;
rope.Rotate(dir * 60 * Time.deltaTime); // 旋转绳子
}
private void Stretch() // 在 Stretch 函数中控制绳子的拉伸
{
if (length >= 7.5f) { state = State.Shorten; return; } // 如果长度达到上限,将状态设置为 Shorten
length += 5*Time.deltaTime; // 增加长度
rope.localScale = new Vector3(rope.localScale.x, length, rope.localScale.z); // 更新绳子的大小
ropeCord.localScale = new Vector3(ropeCord.localScale.x, 1 / length, ropeCord.localScale.z); // 更新绳索的大小
}
private void Shorten() // 在 Shorten 函数中控制绳子的缩短
{
if (length <= 1) // 如果长度已经最小了
{
length = 1; // 将长度设置为 1
state = State.Rock; // 将状态设置为 Rock
if (0 != ropeCord.childCount) // 如果绳索上挂着物品
{
grade += GetGrade(ropeCord.GetChild(0).tag); // 计算分数
Destroy(ropeCord.GetChild(0).gameObject); // 删除挂着的物品
}
ropeCord.GetComponent<Collider2D>().enabled = true; // 开启绳索的碰撞检测
return;
}
length -= Time.deltaTime; // 减少长度
rope.localScale = new Vector3(rope.localScale.x, length, rope.localScale.z); // 更新绳子的大小
ropeCord.localScale = new Vector3(ropeCord.localScale.x, 1 / length, ropeCord.localScale.z); // 更新绳索的大小
}
private int GetGrade(string tag) // 根据挂着的物品的标签计算分数
{
int num = 0;
六、 动画
金子反光
老铺奔跑
钻石反光
七、 下载工程文件
https://wwez.lanzoul.com/iohjp0tjiexe
更多推荐
所有评论(0)