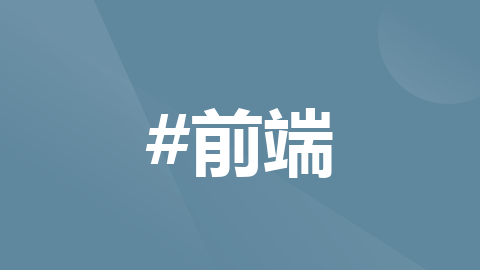
vue axios封装,同步执行
第一步安装:npm install --save。第一步,在api文件下新建一个https.js文件。第三步,在main.js 中声明原型使用他。第二步:在res下创建一个。再新建一个api.js文件。在main.js引入。第四步,在页面中使用。
·
基础封装
第一步安装:npm install --save axios
在main.js引入
如下:
import axios from 'axios'
Vue.prototype.$axios=axios
第二步:在res下创建一个api文件夹
再新建一个api.js文件
import axios from 'axios';
axios.defaults.timeout = 30000;
// 添加响应拦截器
axios.interceptors.response.use(function (response) {
//可以写if判断,提前拦截错误信息
return response;
}, function (err) {
return Promise.reject(err);
});
export function apiGet(url, params){
return new Promise((resolve, reject) =>{
axios.get(url, {
params: params,
headers:{"token":sessionStorage.getItem('token')}
}).then(res => {
resolve(res.data);
}).catch(err =>{
reject(err.data)
})
});
}
export function apiPost(url, params){
return new Promise((resolve, reject) => {
axios({
method: 'post',
url:url,
data:params
}).then(res => {
resolve(res.data);
}).catch(err =>{reject(err.data)})
});
}
第三步,在main.js 中声明原型使用他
import {apiGet,apiPost} from './api/api'
Vue.prototype.$apiGet = apiGet
Vue.prototype.$apiPost = apiPost
第四步,在页面中使用
this.$apiGet(参数1(链接),参数2(数据没有数据可以不写)).then(res=>{
//成功执行then
}).catch(err=>{
//失败执行catch
})
//$apiPost同理
二次封装
第一步,在api文件下新建一个https.js文件
//导入刚刚写的api.js中的get,post方法
import {apiGet,apiPost} from './api'
//get获取登录验证码
export function getCode(){
return new Promise((resolve,reject)=>{
apiGet(获取验证码的链接).then(res=>{
//成功执行
if (res.code == 0) {
resolve(res)
}
}).catch(err=>{
//失败执行
reject(err)
})
})
}
//post用户登录
export function getLogon(传过来的参数){
return new Promise((resolve,reject)=>{
apiPost(登录链接,参数).then(
//成功执行
(res) => {
if (res.code == 0) {
resolve('登录成功')
} else {
this.$message(res.msg)
console.log(res)
}
}).catch(err=>{
//失败执行
reject(err)
})
})
}
页面使用
<button @click="fun()">
登录
</button>
<script>
//首先导入方法
import {getCode,getLogon} from '../api/https'
export default{
created(){
//获取验证码
getCode().then(res=>{
console.log('我的验证码是'+res.msg)
}).catch(err=>{
console.log(err.msg)
})
}
//登录
methode:{
fun(){
let data = {
vercode: this.code,//表单获取的验证码
name: this.user,//表单获取的用户名
pwd: this.pwd,//表单获取的密码
}
getLogon(data).then(res=>{
console.log(res.msg)
}).catch(err=>{
console.log(err.msg)
})
}
}
}
</script>
同步执行
export default{
//在事件前加上 async
async created(){
//赋值的方法,用await先拿到第一个接口的结果,再去请求第二个接口
let code=await getCode()
console.log('我的验证码是'+code)
//此时正常调用第二个接口,第一个接口返回正常才会执行第二个接口
getLogon(data).then(res=>{
console.log(res.msg)
})
}
}
更多推荐
所有评论(0)