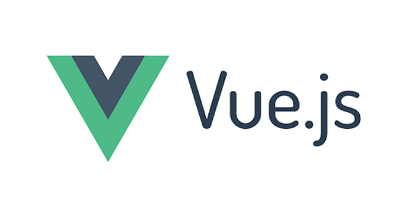
moment方法获取当前时间并对时间进行处理
获取时间,用js来获取时间的话还要进行处理。不过vue底层帮我们封装好了现成的moment()方法。用moment()来获取时间及进行时间处理
·
我们在日常开发vue项目中,有时会用到时间,用js来获取时间的话还要进行处理。
不过vue底层帮我们封装好了现成的moment()方法。
1、首先vue项目中要安装到moment
npm install moment --save
2、引入
import moment from 'moment'
3、使用
基础用法:
//获取当前时间
var now = moment().toDate();
console.log(now) // Tue Apr 04 2023 15:16:33 GMT+0800 (中国标准时间)
//格式化当前时间
var now = moment().format("YYYY-MM-DD HH:mm:ss");
console.log(now); // 2023-04-04 15:17:59
//获取这个月初时间
let startMonth = moment().startOf("month").toDate();
console.log(startMonth); // Sat Apr 01 2023 00:00:00 GMT+0800 (中国标准时间)
//获取今天开始的时间
let dayOfStart = moment().startOf('day').toDate();
console.log(dayOfStart); // Tue Apr 04 2023 00:00:00 GMT+0800 (中国标准时间)
//获取今天结束的时间
let dayOfEnd = moment().endOf('day').toDate();
console.log(dayOfEnd); // Tue Apr 04 2023 23:59:59 GMT+0800 (中国标准时间)
//获取+n小时 例:+2小时
let lateHour = moment().add(2,'hour').toDate();
console.log(lateHour); // Tue Apr 04 2023 17:24:33 GMT+0800 (中国标准时间)
//获取+n天 例:+5天
let lateDay = moment().add(+5,'day').toDate();
console.log(lateDay); // Sun Apr 09 2023 15:26:10 GMT+0800 (中国标准时间)
//获取-n天 例:-5天
let beforeDay = moment().subtract(5,'day').toDate();
console.log(beforeDay); // Thu Mar 30 2023 15:27:12 GMT+0800 (中国标准时间)
// 获取+n月 例:+2月
let lateMonth = moment().add(2,'month').toDate();
console.log(lateMonth);// Sun Jun 04 2023 15:29:57 GMT+0800 (中国标准时间)
// 获取-n月 例:-2月
let beforeMonth = moment().subtract(2,'month').toDate();
console.log(lateHour);// Sat Feb 04 2023 15:31:34 GMT+0800 (中国标准时间)
moment()方法里面有很多的API, 例:
.toData(): 原生 Date 对象的副本
//获取当前时间
var now = moment().toDate();
console.log(now) // Tue Apr 04 2023 15:16:33 GMT+0800 (中国标准时间)
.format(): 它接受一串令牌并将其替换为其相应的值,你可以对它返回的格式进行规定
//格式化当前时间
var now = moment().format("YYYY-MM-DD HH:mm:ss");
console.log(now); // 2023-04-04 15:17:59
.toArray(): 会返回一个包含年份、月份、月份的日期、小时、分钟、秒钟、毫秒的数组。
var now = moment().toArray();
console.log(now); // [2023, 3, 4, 15, 57, 48, 385]
.toObject() :这会返回一个包含年份、月份、月份的日期、小时、分钟、秒钟、毫秒的对象。
var now = moment().toObject();
console.log(now); // {years: 2023, months: 3, date: 4, hours: 16, minutes: 0, …}
获取传入月数的天数
const dataTime1 = moment("2023-02", "YYYY-MM").daysInMonth(); // 28
有了这些基础API,我们就可以进行组合来进行时间获取:
获取当前时间及一月前时间
const dataTime = [
moment().subtract(1, "months").format("YYYY-MM-DD HH:mm:ss"),
moment().format("YYYY-MM-DD HH:mm:ss"),
]; // ['2023-03-04 15:34:27', '2023-04-04 15:34:27']
获取上个月的第一天和未来一个月的最后一天
const dataTime = [
moment().subtract(1,'months').date(1).format("YYYY-MM-DD HH:mm:ss"),
moment().add(2,'months').date(0).format("YYYY-MM-DD HH:mm:ss"),
]; // ['2023-03-01 15:42:19', '2023-05-31 15:42:19']
还可以对两个时间进行对比
判断是否早于
var now = moment("2023-04-01").isBefore("2023-04-04");
console.log(now); // true;
判断是否相等
var now = moment("2023-04-04").isSame("2023-04-04");
console.log(now); // true;
判断是否晚于
var now = moment("2023-04-04").isAfter("2023-04-01");
console.log(now); // true;
判断是否在时间范围内
var now = moment("2023-04-02").isBetween("2023-04-01", "2023-04-04");
console.log(now); // true;
以上是一些常用的API和使用方法示例,更多方法可以去官网查看:
更多推荐
所有评论(0)