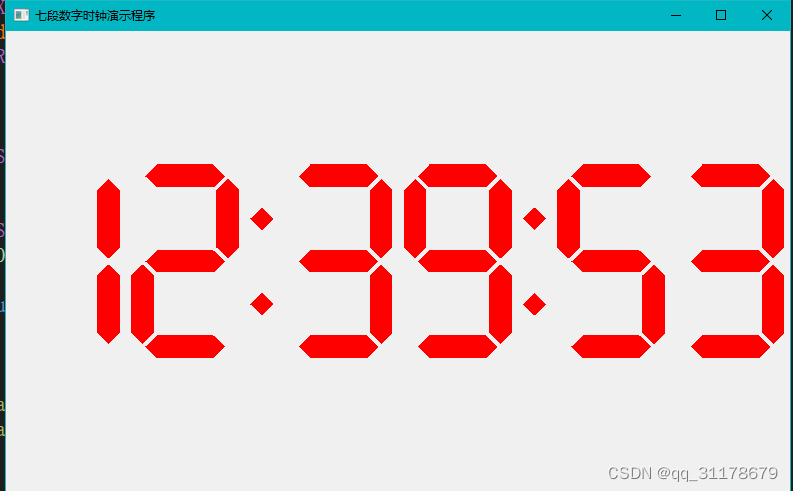
七段数字时钟演示程序
【代码】七段数字时钟演示程序。

一键AI生成摘要,助你高效阅读
问答
·
程序最终效果
源代码:
/*-------------------------------------------
WinMain.cpp --七段数字时钟演示程序
GreenLeaf1976 791869691@qq.com
----------------------------------------------*/
#include <windows.h>
#define ID_TIMER 1
HINSTANCE g_hInstance = 0;
//主窗口回调函数
LRESULT CALLBACK AfxWndProc(HWND hWnd, UINT uMsg, WPARAM wParam, LPARAM lParam);
//主函数WinMain
int WINAPI WinMain(_In_ HINSTANCE hInstance,
_In_opt_ HINSTANCE hPrevInstance,
_In_ LPSTR lpCmdLine, _In_ int nShowCmd)
{
g_hInstance = hInstance;
//定义窗口类名
TCHAR szClassName[] = TEXT("AfxWndClassName");
//设计窗口类
WNDCLASSEX wcEx = { 0 };
wcEx.cbSize = sizeof(WNDCLASSEX); //窗口类结构大小
wcEx.cbClsExtra = 0; //窗口类额外数据
wcEx.cbWndExtra = 0; //窗口额外数据
wcEx.style = CS_VREDRAW | CS_HREDRAW; //窗口类样式
wcEx.lpfnWndProc = AfxWndProc; //窗口类过程函数
wcEx.lpszClassName = szClassName; //窗口类名
wcEx.lpszMenuName = nullptr; //菜单句柄
wcEx.hbrBackground = (HBRUSH)(COLOR_3DFACE + 1);//窗口类背景色
wcEx.hInstance = hInstance; //应用程序实例
wcEx.hCursor = LoadCursor(NULL, IDC_ARROW); //设置默认光标
wcEx.hIconSm = LoadIcon(NULL, IDI_APPLICATION); //窗口类小图标
wcEx.hIcon = wcEx.hIconSm; //窗口类大图标
//注册窗口类
if (!RegisterClassEx(&wcEx))
{
MessageBox(nullptr, TEXT("窗口类注册失败!"), TEXT("窗口类注册消息"), MB_ICONERROR);
return -1;
}
//创建窗口
HWND hWnd = CreateWindowEx(0, //窗口扩展样式
szClassName, //窗口类名称
TEXT("我的窗口"), //窗口标题文本
WS_OVERLAPPEDWINDOW, //窗口样式
100, 100, 800, 500, //窗口显示的矩形位置(x,y,widht,height)
nullptr, //父窗口句柄
nullptr, //窗口菜单句柄(如果是子窗口就是窗口的ID号)
hInstance, //应用程序实例
0); //附加数据
if (!hWnd)
{
MessageBox(hWnd, TEXT("窗口创建失败!"), TEXT("窗口创建消息"), MB_ICONERROR);
return -2;
}
//显示与更新窗口
ShowWindow(hWnd, nShowCmd);
UpdateWindow(hWnd);
SetForegroundWindow(hWnd); //设置为前台显示窗口
//消息循环
MSG msg;
while (GetMessage(&msg, nullptr, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
return msg.wParam;
}
//绘制要显示的数字的形状
void DisplayDigit(HDC hdc, int iNumber)
{
//数字的显示字段顺序为:
//1,是上面一横,
//2,第二排左面一竖
//3,第二排右面一竖
//4,中间一横
//5,第四排左边一竖
//6,第四排右边一竖
//7,最后一排的一横
static BOOL fSevenSegment[10][7] = {
1,1,1,0,1,1,1, //0
0,0,1,0,0,1,0, //1
1,0,1,1,1,0,1, //2
1,0,1,1,0,1,1, //3
0,1,1,1,0,1,0, //4
1,1,0,1,0,1,1, //5
1,1,0,1,1,1,1, //6
1,0,1,0,0,1,0, //7
1,1,1,1,1,1,1, //8
1,1,1,1,0,1,1 //9
};
//七段数字的各个棱形的坐标
//可在绘图上画好图形后,用工具测量实际坐标值
static POINT ptSegment[7][6] = {
7,6,11,2,31,2,35,6,31,10,11,10, //最上面一横的坐标
6,7,10,11,10,31,6,35,2,31,2,11, //上面左边一竖的坐标
36,7,40,11,40,31,36,35,32,31,32,11, //上面右边一竖的坐标
7,36,11,32,31,32,35,36,31,40,11,40, //中间一横的坐标
6,37,10,41,10,61,6,65,2,61,2,41, //下面左边一竖的坐标
36,37,40,41,40,61,36,65,32,61,32,41,//下面右边一竖的坐标
7,66,11,62,31,62,35,66,31,70,11,70 //最下面一横的坐标
};
int iSeg;
for (iSeg = 0; iSeg < 7; iSeg++)
{
if (fSevenSegment[iNumber][iSeg])
Polygon(hdc, ptSegment[iSeg], 6);
}
}
//绘制数字
void DisplayTwoDigits(HDC hdc, int iNumber, BOOL fSuppress)
{
if (fSuppress || (iNumber / 10 != 0))
DisplayDigit(hdc, iNumber / 10);
OffsetWindowOrgEx(hdc, -42, 0, nullptr);
DisplayDigit(hdc, iNumber % 10);
OffsetWindowOrgEx(hdc, -42, 0, nullptr);
}
//绘制冒号
void DisplayColon(HDC hdc)
{
POINT ptColon[2][4] = {
2,21,6,17,10,21,6,25,
2,51,6,47,10,51,6,55
};
Polygon(hdc, ptColon[0], 4);
Polygon(hdc, ptColon[1], 4);
OffsetWindowOrgEx(hdc, -12, 0, nullptr);
}
//绘制时间
void DisplayTime(HDC hdc, BOOL f24Hour, BOOL fSuppress)
{
SYSTEMTIME st;
GetLocalTime(&st);
if (f24Hour)
DisplayTwoDigits(hdc, st.wHour, fSuppress);
else
DisplayTwoDigits(hdc, (st.wHour %= 12) ? st.wHour : 12, fSuppress);
DisplayColon(hdc);
DisplayTwoDigits(hdc, st.wMinute, TRUE);
DisplayColon(hdc);
DisplayTwoDigits(hdc, st.wSecond, TRUE);
}
LRESULT CALLBACK AfxWndProc(HWND hWnd, UINT uMsg, WPARAM wParam, LPARAM lParam)
{
static BOOL f24Hour, fSupperss;
static HBRUSH hBrushRed;
static int cxClient, cyClient;
HDC hdc;
PAINTSTRUCT ps;
TCHAR szBuffer[2];
switch (uMsg)
{
case WM_CREATE:
SetWindowText(hWnd, TEXT("七段数字时钟演示程序"));
hBrushRed = CreateSolidBrush(RGB(255, 0, 0));
SetTimer(hWnd, ID_TIMER, 1000, nullptr);
//break;
case WM_SETTINGCHANGE:
GetLocaleInfo(LOCALE_USER_DEFAULT, LOCALE_ITIME, szBuffer, 2);
f24Hour = (szBuffer[0] == '1');
GetLocaleInfo(LOCALE_USER_DEFAULT, LOCALE_ITLZERO, szBuffer, 2);
fSupperss = (szBuffer[0] == '0');
InvalidateRect(hWnd, nullptr, TRUE);
break;
case WM_SIZE:
cxClient = LOWORD(lParam);
cyClient = HIWORD(lParam);
break;
case WM_TIMER:
InvalidateRect(hWnd, nullptr, TRUE);
break;
case WM_PAINT:
hdc = BeginPaint(hWnd, &ps);
SetMapMode(hdc, MM_ISOTROPIC);
SetWindowExtEx(hdc, 276, 72, nullptr);
SetViewportExtEx(hdc, cxClient, cyClient, nullptr);
SetWindowOrgEx(hdc, 138, 36, nullptr);
SetViewportOrgEx(hdc, cxClient / 2, cyClient / 2, nullptr);
SelectObject(hdc, GetStockObject(NULL_PEN));
SelectObject(hdc, hBrushRed);
DisplayTime(hdc, f24Hour, fSupperss);
EndPaint(hWnd, &ps);
break;
case WM_DESTROY:
KillTimer(hWnd, ID_TIMER);
DeleteObject(hBrushRed);
PostQuitMessage(0);
return 0;
default:
break;
}
return DefWindowProc(hWnd, uMsg, wParam, lParam);
}
更多推荐
所有评论(0)