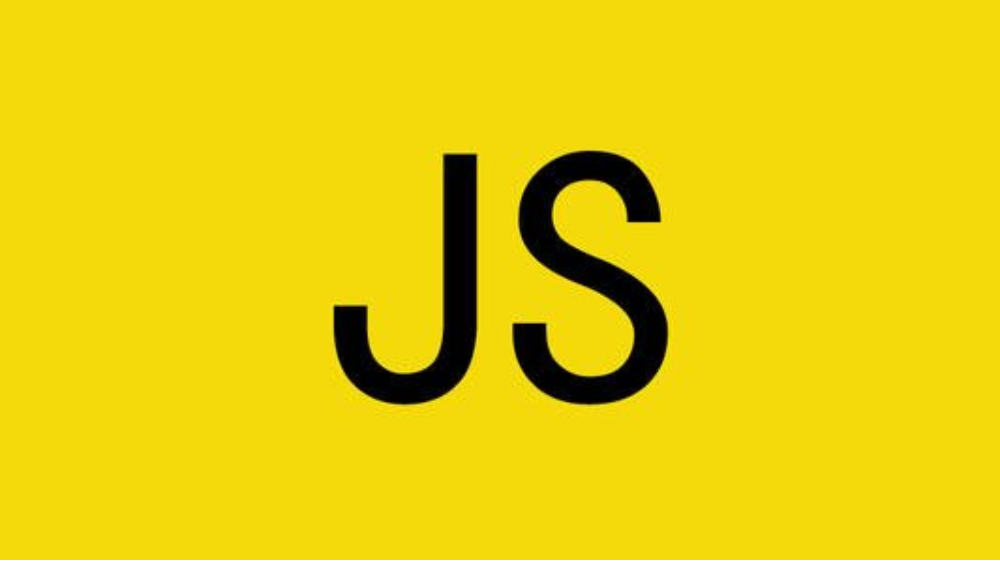
js中reduce()函数的用法
reduce()方法对每一个数组的每一个元素按序执行一个由您提供的reduce函数。每一次运行reduce会将先前元素的计算结果作为参数传入,最后将其结果汇总为单个返回值。第一次执行回调函数时,不存在“上一次的计算结果”。如果需要回调函数从数组索引为0的开始执行,则需要传递初始值,否则数组的索引为0的值作为初始值initalValue,迭代器从第二个元素开始执行(索引为1而不是0)

😁 作者简介:一名大三的学生,致力学习前端开发技术
⭐️个人主页:夜宵饽饽的主页
❔ 系列专栏:JavaScript小贴士
👐学习格言:成功不是终点,失败也并非末日,最重要的是继续前进的勇气
🔥前言:
这是我自己有关array.prototype.reduce()函数的用法的总结,如有不足和错误,欢迎大家更好的补充和纠正
1.Array.prototype.reduce()函数的用法
定义:
reduce()方法对每一个数组的每一个元素按序执行一个由您提供的reduce函数。每一次运行reduce会将先前元素的计算结果作为参数传入,最后将其结果汇总为单个返回值。
第一次执行回调函数时,不存在“上一次的计算结果”。如果需要回调函数从数组索引为0的开始执行,则需要传递初始值,否则数组的索引为0的值作为初始值initalValue,迭代器从第二个元素开始执行(索引为1而不是0)
语法:
reduce((previousValue, currentValue, currentIndex, array) => { /* … */ }, initialValue)
参数:
callbackfn
一个“reduce”函数,包含四个参数:
- previousValue:上一次调用callbackFn时的返回值,在一次调用时,若指定初始值initialValue,其值为initalvalue,否则为数组索引为0的元素array[0]
- currentValue:数组中正在处理的元素,在第一次调用时,若指定初始值initalValue,其值则为数组索引为0的元素array[0],否则为array[1]
- currentIndex:数组中正在处理的元素索引,若指定了初始值initvalue,则起始索引号,否则从索引1起的。
- array:用于遍历的数组
initalvalue(可选)
作为第一次调用callback函数时的参数previousValue的值,若指定了初始值initalValue,则currentValue则将使用数组第一个元素,否则previosValue的值是将是数组的第一个元素,而currentValue将使用数组第二个元素
返回值
使用reduce回调函数遍历整个数组后的结果
异常
TypeError
数组为空且初始值未提供
扩展知识点:
如果数组为空且未指定初始值 initialValue,则会抛出
TypeError
。
reduce
不会直接改变调用它的对象,但对象可被调用的 callbackfn 所改变。遍历的元素范围是在第一次调用 callbackfn 之前确定的。所以即使有元素在调用开始后被追加到数组中,这些元素也不会被 callbackfn 访问。如果数组现有的元素发生了变化,传递给 callbackfn 的值将会是元素被reduce
访问时的值(即发生变化后的值);在调用reduce
开始后,尚未被访问的元素若被删除,则其将不会被reduce
访问。
基本使用
1.将二维数组转化为一维
//将二维数组转化一维数组
let fla=[[0,1],[2,3],[4,5]].reduce(
function(pre,cur){
return pre.concat(cur)
},
[]
)
console.log("二维数组转化一维数组:"+fla);
//二维数组转化一维数组:0,1,2,3,4,5
2.计算数组中每一个元素出现的次数
//计算数组中每一个元素出现的次数
let names=['Alice','Bob','Tiff','Bruce','Alice']
let countedNames=names.reduce((allName,curname) =>{
if(curname in allName){
allName[curname]++
}
else{
allName[curname]=1
}
return allName
},{})
console.log(countedNames);
//{ Alice: 2, Bob: 1, Tiff: 1, Bruce: 1 }
3.按属性对object分类
let peple=[
{name:'Alice',age:21},
{name:'Mex',age:20},
{name:'Jane',age:20}
];
function groupBy(objectArray,property) {
return objectArray.reduce(function(pre,cur){
let key=cur[property]
if(!pre[key]){
pre[key]=[]
}
pre[key].push(cur)
return pre
},{})
}
let groupByPeople=groupBy(peple,'age')
console.log(groupByPeople)
/**{
'20': [ { name: 'Mex', age: 20 }, { name: 'Jane', age: 20 } ],
'21': [ { name: 'Alice', age: 21 } ]
}*/
4.使用扩展运算符和initialValue绑定包含在对象数组中的数组
let friends = [{
name: 'Anna',
books: ['Bible', 'Harry Potter'],
age: 21
}, {
name: 'Bob',
books: ['War and peace', 'Romeo and Juliet'],
age: 26
}, {
name: 'Alice',
books: ['The Lord of the Rings', 'The Shining'],
age: 18
}]
let allbook=friends.reduce((pre,cur) =>{
let key=cur['books']
return [...pre,...key]
},['alphoter'])
console.log(allbook);
/**
[
'alphoter',
'Bible',
'Harry Potter',
'War and peace',
'Romeo and Juliet',
'The Lord of the Rings',
'The Shining'
]
*/
6.数组去重
//数组去重
let myArray = ['a', 'b', 'a', 'b', 'c', 'e', 'e', 'c', 'd', 'd', 'd', 'd']
const myArrayNo =myArray.reduce(function(pre,cur){
if(pre.indexOf(cur)==-1){
pre.push(cur)
}
return pre
},[])
console.log(myArrayNo);
//[ 'a', 'b', 'c', 'e', 'd' ]
7.使用reduce 实现map
if (!Array.prototype.mapUsingReduce) {
Array.prototype.mapUsingReduce = function(callback, initialValue) {
return this.reduce(function(mappedArray, currentValue, currentIndex, array) {
mappedArray[currentIndex] = callback.call(initialValue, currentValue, currentIndex, array)
return mappedArray
}, [])
}
}
[1, 2, , 3].mapUsingReduce(
(currentValue, currentIndex, array) => currentValue + currentIndex + array.length
) // [5, 7, , 10]
最后
🌼 如果觉得笔者写的不错,可以看看笔者以下的文章喔 😃
更多推荐
所有评论(0)