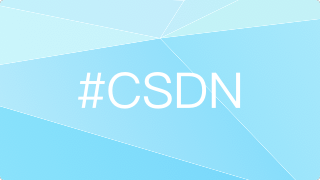
Mybatis 批量更新update详解
mybatis

本文作为知识点记录,拷贝自:https://blog.csdn.net/bbj12345678/article/details/118436343
Mybatis 批量更新update详解
1. 更新单条记录
UPDATE course SET name = 'course1' WHEREid = 'id1';
2. 更新多条记录的同一个字段为同一个值
UPDATE course SET name='course1' WHERE id in('id1','id2','id3);
3. 更新多条记录为多个字段为不同的值
3.1 普通写法
比较普通的写法,是通过循环,一次执行update语句。
Mybatis 写法如下:
<update id="updateBatch" parameterType="java.util.List">
<foreach collection="list" item="item" index="index" open="" close="" separator=";">
update course
<set>
name = #{item.name}
</set>
where id = #{item.id}
</foreach>
</update>
一条记录update一次,性能比较差,容易造成阻塞。
3.2 使用 case when
MySQL没有提供直接的方法来实现批量更新,但可以使用case when语法来实现这个功能。
UPDATE course
SET
name = CASE id
WHEN 1 THEN 'name1'
WHEN 2 THEN 'name2'
WHEN 3 THEN 'name3'
END,
title = CASE id
WHEN 1 THEN 'New Title 1'
WHEN 2 THEN 'New Title 2'
WHEN 3 THEN 'New Title 3'
END
WHERE id IN (1,2,3)
这条sql的意思是,如果id为1,则name的值为name1,title的值为New Title 1,以此类推。
在Mybatis中的配置如下:
<updateid="updateBatch"parameterType="list">
update course
<trim prefix="set" suffixOverrides=",">
<trim prefix="name=case" suffix="end,">
<foreach collection="list" item="item" index="index">
<if test="item.name!=null">
when id=#{item.id} then #{item.name}
</if>
</foreach>
</trim>
<trim prefix="title =case" suffix="end,">
<foreach collection="list" item="item" index="index">
<if test="item.title!=null">
when id=#{item.id} then #{item.title}
</if>
</foreach>
</trim>
</trim>
where
<foreach collection="list" separator="or" item="item" index="index">
id=#{item.id}
</foreach>
</update>
<trim>属性说明
1.prefix、suffix 表示在<trim>标签包裹的部分的前面、后面添加的内容;
2.如果同时有prefixOverrides、suffixOverride表示会用prefix、suffix覆盖Overrides中的内容;
3.如果只有prefixOverrides、suffixOverrrides表示删除开头的、结尾的xxxOverrrides指定的内容
4. sql批量更新
看另外一个示例:
<updateid="updateBatch"parameterType="java.util.List">
update mydata_table
set
status =
<foreach collection="list" item="item" index="index" separator=" " open="case ID" close="end">
when #{item.id} then #{item.status}
</foreach>
where id in
<foreach collection="list" index="index" item="item" separator="," open="(" close=")">
#{item.id,jdbcType=BIGINT}
</foreach>
</update>
其中when…then…是sql中的“switch”语法。这里借助Mybatis的<foreach>语法来拼凑成了批量更新的sql,上面的意思就是批量更新id在updateBatch参数所传递List中的数据的status字段。还可以使用<trim>实现同样的功能,代码如下:
<update id="updateBatch" parameterType="java.util.List">
update mydata_table
<trim prefix="set" suffixOverrides=",">
<trim prefix="status =case" suffix="end,">
<foreach collection="list" item="item" index="index">
when id=#{item.id} then #{item.status}
</foreach>
</trim>
</trim>
where id in
<foreach collection="list" index="index" item="item" separator="," open="(" close=")">
#{item.id,jdbcType=BIGINT}
</foreach>
</update>
其结构如下:
update mydata_table
set
status =
case
when id = #{item.id} then #{item.status}//此处应该是<foreach>展开值
...
end
where id in (...);
而如果当需要为某个字段设置默认值的时候可以使用else
<trim prefix="status = case" suffix="end,">
<foreach collection="list" item="item" index="index">
when id=#{item.id} then #{item.status}
</foreach>
else default_value
</trim>
这样的话只有要更新的list中status != null && status != -1的数据才能进行status更新.其他的将使用默认值更新.如果要保持原数据不变呢?即满足条件的更新,不满足条件的保持原数据不变,简单的来做就是再加一个,因为mybatis中没有if…else…语法,但可以通过多个实现同样的效果,如下:
<trim prefix="status =case" suffix="end,">
<foreach collection="list" item="item" index="index">
<if test="item.status !=null and item.status != -1">
when id=#{item.id} then #{item.status}
</if>
<if test="item.status == null or item.status == -1">
when id=#{item.id} then mydata_table.status //这里就是原数据
</if>
</foreach>
</trim>
整体批量更新的写法如下:
<updateid="updateBatch"parameterType="java.util.List">
update mydata_table
<trim prefix="set" suffixOverrides=",">
<trim prefix="status =case" suffix="end,">
<foreach collection="list" item="item" index="index">
<if test="item.status !=null and item.status != -1">
when id=#{item.id} then #{item.status}
</if>
<if test="item.status == null or item.status == -1">
when id=#{item.id} then mydata_table.status//原数据
</if>
</foreach>
</trim>
</trim>
where id in
<foreach collection="list" index="index" item="item" separator="," open="(" close=")">
#{item.id,jdbcType=BIGINT}
</foreach>
</update>
1.组装多个update语句,这种方式需要设置jdbc连接 allowMultiQueries=true
<update id="updateEquementWaterTest" parameterType="java.util.List">
<foreach collection="list" item="item" index="index">
update rent_hl_room l
SET l.water_meter_id=#{item.equipmentCode},
l.water_meter_source_type=#{item.equipmentSource}
WHERE
l.room_id=#{item.roomId};
</foreach>
</update>
2.case when
UPDATE
rent_hl_room l
SET
electricity_meter_id =
CASE
WHEN room_id = 1942 THEN 180524348
WHEN room_id = 1945 THEN 180524480
END,
electricity_meter_source_type =
CASE
WHEN room_id = 1942 THEN ym
WHEN room_id = 1945 THEN ym
END
WHERE room_id = 1942
OR room_id = 1945
<update id="updateEquementWater" parameterType="java.util.List">
update rent_hl_room l
<trim prefix="set" suffixOverrides=",">
<trim prefix="water_meter_id =case" suffix="end,">
<foreach collection="list" item="item" index="index">
<if test="item.equipmentCode!=null">
when room_id=#{i.roomId} then #{i.equipmentCode}
</if>
</foreach>
</trim>
<trim prefix=" water_meter_source_type =case" suffix="end,">
<foreach collection="list" item="item" index="index">
<if test="itemequipmentSource!=null">
when room_id=#{i.roomId} then #{i.equipmentSource}
</if>
</foreach>
</trim>
</trim>
where
<foreach collection="list" separator="or" item="item" index="index" >
room_id=#{item.roomId}
</foreach>
</update>
经测试 100条数据的时候第一种方式的效率比第二种差不多高1倍。。。。。
更多推荐
所有评论(0)