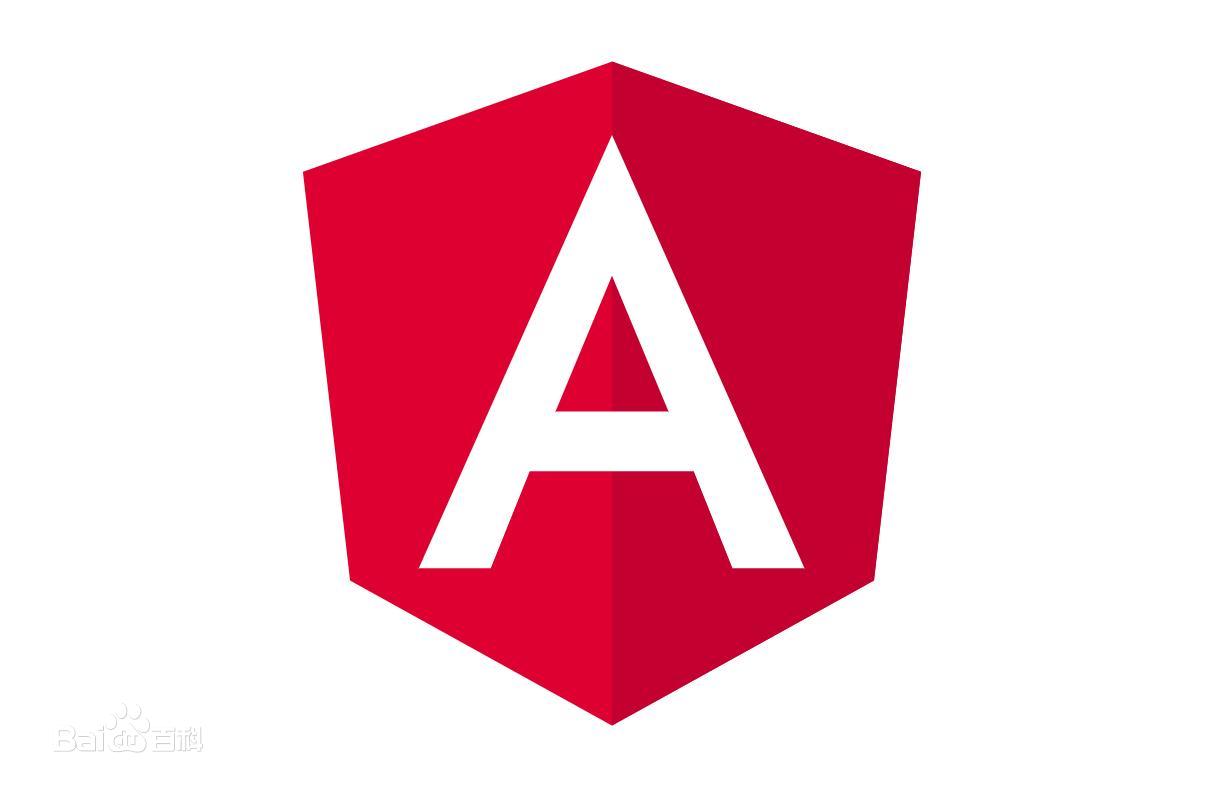
Angular动态创建组件
动态组件是指在运行时根据需要创建的组件,可以将它们添加到 DOM 中,也可以从 DOM 中移除它们。调用ViewContainerRef 的createComponent 方法,根据组件的工厂对象创建组件的实例,并将其添加到容器元素中。在创建组件实例后,我们可以设置组件的属性、订阅组件事件,并将组件插入到 DOM 中。根据需要,可以更新组件的输入属性,订阅组件的输出事件,或调用组件的方法来与组件进
在 Angular 中,可以使用动态组件来动态创建和销毁组件。动态组件是指在运行时根据需要创建的组件,可以将它们添加到 DOM 中,也可以从 DOM 中移除它们。Angular 提供了两种方式来实现动态组件:通过 ViewContainerRef 和 ComponentFactoryResolver 来创建和管理动态组件。
使用 ViewContainerRef 和 ComponentFactoryResolver 创建动态组件的过程如下:
-
获取容器元素的ViewContainerRef 对象,该对象提供了一种将动态组件添加到 DOM 中的方式。
-
获取组件的工厂对象,该对象可以根据组件的类型或名称创建组件的实例。
-
调用ViewContainerRef 的createComponent 方法,根据组件的工厂对象创建组件的实例,并将其添加到容器元素中。
-
根据需要,可以更新组件的输入属性,订阅组件的输出事件,或调用组件的方法来与组件进行交互。
-
如果不再需要该组件,可以调用ViewContainerRef 的remove 方法将其从容器元素中移除,或调用
destroy 方法销毁该组件的实例。
下面是一个示例代码:
import { Component, ComponentFactoryResolver, ViewChild, ViewContainerRef } from '@angular/core';
import { MyComponent } from './my-component';
@Component({
selector: 'app-root',
template: '<ng-container #container></ng-container>',
})
export class AppComponent {
@ViewChild('container', { read: ViewContainerRef }) container: ViewContainerRef;
constructor(private componentFactoryResolver: ComponentFactoryResolver) {}
createComponent() {
// 获取组件工厂
const componentFactory = this.componentFactoryResolver.resolveComponentFactory(MyComponent);
// 创建组件实例
const componentRef = this.container.createComponent(componentFactory);
// 设置组件属性
componentRef.instance.myInput = 'input value';
// 订阅组件事件
componentRef.instance.myOutput.subscribe((event) => {
console.log('Received event:', event);
});
}
removeComponent() {
// 移除组件
this.container.remove();
}
}
在上面的示例代码中,我们首先使用 ViewChild 注解来获取容器元素的引用。然后,我们在 createComponent() 方法中获取 MyComponent 组件的组件工厂,并使用 createComponent() 方法创建组件实例。在创建组件实例后,我们可以设置组件的属性、订阅组件事件,并将组件插入到 DOM 中。在 removeComponent() 方法中,我们可以调用 ViewContainerRef 的 remove 方法将组件从 DOM 中移除。
更多推荐
所有评论(0)