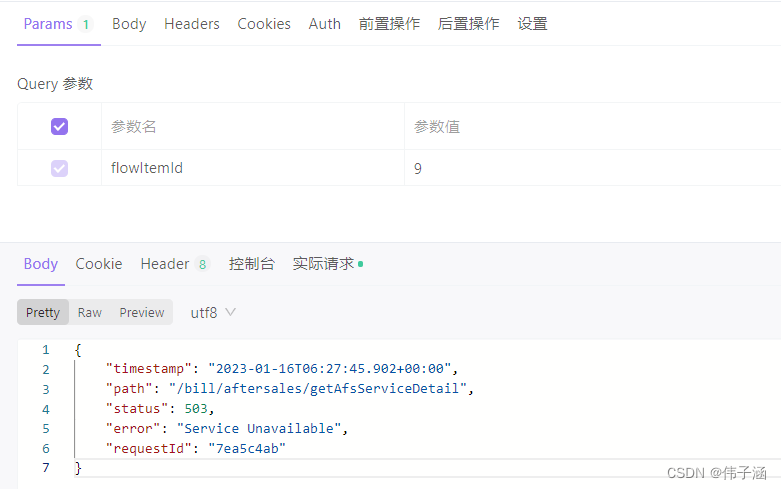
【微服务网关---Gateway 的全局异常处理器】
Gateway网关统一全局异常处理操作方便前端看到这里要精细化翻译,默认返回用户是看不懂的 所以需要配置一个 Gateway 的全局异常处理器如果没有网关全局异常的 会如下截图以上就是今天要讲的内容,本文仅仅简单 所以需要配置一个 Gateway 的全局异常处理器。
·
前言
Gateway网关统一全局异常处理操作 方便前端看到 这里要精细化翻译,默认返回用户是看不懂的 所以需要配置一个 Gateway 的全局异常处理器
如果没有网关全局异常的 会如下截图
一、使用步骤
1.编写 GlobalExceptionHandler
代码如下:
package cn.cws.framework.gatewayservice.handler;
import cn.cws.framework.core.common.service.ApiResult;
import cn.cws.framework.gatewayservice.handler.code.GatewayErrorCode;
import cn.cws.framework.gatewayservice.util.WebFrameworkUtils;
import lombok.extern.slf4j.Slf4j;
import org.springframework.boot.web.reactive.error.ErrorWebExceptionHandler;
import org.springframework.core.annotation.Order;
import org.springframework.http.server.reactive.ServerHttpRequest;
import org.springframework.http.server.reactive.ServerHttpResponse;
import org.springframework.stereotype.Component;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.server.ResponseStatusException;
import org.springframework.web.server.ServerWebExchange;
import reactor.core.publisher.Mono;
/**
* Gateway 的全局异常处理器,将 Exception 翻译成 ApiResult + 对应的异常编号
*
* 在功能上,和 核心 的 GlobalExceptionHandler 类是一致的
*
* @author zw
*/
@Component
@Order(-1) // 保证优先级高于默认的 Spring Cloud Gateway 的 ErrorWebExceptionHandler 实现
@Slf4j
public class GlobalExceptionHandler implements ErrorWebExceptionHandler {
@Override
public Mono<Void> handle(ServerWebExchange exchange, Throwable ex) {
// 已经 commit,则直接返回异常
ServerHttpResponse response = exchange.getResponse();
if (response.isCommitted()) {
return Mono.error(ex);
}
// 转换成 CommonResult
ApiResult<?> result;
if (ex instanceof ResponseStatusException) {
result = responseStatusExceptionHandler(exchange, (ResponseStatusException) ex);
} else {
result = defaultExceptionHandler(exchange, ex);
}
// 返回给前端
return WebFrameworkUtils.writeJSON(exchange, result);
}
/**
* 处理 Spring Cloud Gateway 默认抛出的 ResponseStatusException 异常
*/
private ApiResult<?> responseStatusExceptionHandler(ServerWebExchange exchange,
ResponseStatusException ex) {
// TODO zw 这里要精细化翻译,默认返回用户是看不懂的
ServerHttpRequest request = exchange.getRequest();
log.error("[responseStatusExceptionHandler][uri({}/{}) 发生异常]", request.getURI(), request.getMethod(), ex);
if (ex.getRawStatusCode()== GatewayErrorCode.SYS_GATEWAY_ERROR_NACOS.getCode()){
return ApiResult.buildFail(ex.getRawStatusCode(), " 请联系管理人员 : "+ex.getReason()+GatewayErrorCode
.SYS_GATEWAY_ERROR_NACOS.getMsg());
}
return ApiResult.buildFail(ex.getRawStatusCode(), ex.getReason());
// return ApiResult.buildFail(ex.getRawStatusCode(), ex.getReason());
}
/**
* 处理系统异常,兜底处理所有的一切
*/
@ExceptionHandler(value = Exception.class)
public ApiResult<?> defaultExceptionHandler(ServerWebExchange exchange,
Throwable ex) {
ServerHttpRequest request = exchange.getRequest();
log.error("[defaultExceptionHandler][uri({}/{}) 发生异常]", request.getURI(), request.getMethod(), ex);
// TODO zw 是否要插入异常日志呢?
// 返回 ERROR CommonResult
log.error("[responseStatusExceptionHandler][uri({}/{}) 发生异常]", request.getURI(), request.getMethod(), ex);
return ApiResult.buildFail(-1, ex.getMessage());
// return ApiResult.buildFail(-1,ex.getStackTrace().toString());
}
}
2.WebFrameworkUtils 工具类
代码如下:
package cn.cws.framework.gatewayservice.util;
import cn.cws.framework.core.common.util.JsonKit;
import lombok.extern.slf4j.Slf4j;
import org.springframework.cloud.gateway.route.Route;
import org.springframework.cloud.gateway.support.ServerWebExchangeUtils;
import org.springframework.core.io.buffer.DataBufferFactory;
import org.springframework.http.HttpHeaders;
import org.springframework.http.MediaType;
import org.springframework.http.server.reactive.ServerHttpRequest;
import org.springframework.http.server.reactive.ServerHttpResponse;
import org.springframework.web.server.ServerWebExchange;
import reactor.core.publisher.Mono;
/**
* Web 工具类
*
*
* @author zw
*/
@Slf4j
public class WebFrameworkUtils {
private static final String HEADER_TENANT_ID = "tenant-id";
private WebFrameworkUtils() {}
/**
* 将 Gateway 请求中的 header,设置到 HttpHeaders 中
*
* @param tenantId 租户编号
* @param httpHeaders WebClient 的请求
*/
public static void setTenantIdHeader(Long tenantId, HttpHeaders httpHeaders) {
if (tenantId == null) {
return;
}
httpHeaders.set(HEADER_TENANT_ID, String.valueOf(tenantId));
}
public static Long getTenantId(ServerWebExchange exchange) {
String tenantId = exchange.getRequest().getHeaders().getFirst(HEADER_TENANT_ID);
return tenantId != null ? Long.parseLong(tenantId) : null;
}
/**
* 返回 JSON 字符串
*
* @param exchange 响应
* @param object 对象,会序列化成 JSON 字符串
*/
@SuppressWarnings("deprecation") // 必须使用 APPLICATION_JSON_UTF8_VALUE,否则会乱码
public static Mono<Void> writeJSON(ServerWebExchange exchange, Object object) {
// 设置 header
ServerHttpResponse response = exchange.getResponse();
response.getHeaders().setContentType(MediaType.APPLICATION_JSON_UTF8);
// 设置 body
return response.writeWith(Mono.fromSupplier(() -> {
DataBufferFactory bufferFactory = response.bufferFactory();
try {
return bufferFactory.wrap(JsonKit.toJsonByte(object));
} catch (Exception ex) {
ServerHttpRequest request = exchange.getRequest();
log.error("[writeJSON][uri({}/{}) 发生异常]", request.getURI(), request.getMethod(), ex);
return bufferFactory.wrap(new byte[0]);
}
}));
}
/**
* 获得请求匹配的 Route 路由
*
* @param exchange 请求
* @return 路由
*/
public static Route getGatewayRoute(ServerWebExchange exchange) {
return exchange.getAttribute(ServerWebExchangeUtils.GATEWAY_ROUTE_ATTR);
}
}
3.json 工具类
代码如下:
package cn.cws.framework.core.common.util;
import com.fasterxml.jackson.annotation.JsonFilter;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.core.JsonFactory;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.*;
import com.fasterxml.jackson.databind.ser.FilterProvider;
import com.fasterxml.jackson.databind.ser.impl.SimpleBeanPropertyFilter;
import com.fasterxml.jackson.databind.ser.impl.SimpleFilterProvider;
import com.fasterxml.jackson.datatype.jsr310.JavaTimeModule;
import lombok.SneakyThrows;
import java.io.IOException;
import java.io.StringWriter;
import java.util.List;
import java.util.Map;
/**
* @author zw
*/
public class JsonKit {
public static final ObjectMapper OBJECT_MAPPER = createObjectMapper();
private static final ObjectMapper IGNORE_OBJECT_MAPPER = createIgnoreObjectMapper();
private static ObjectMapper createIgnoreObjectMapper() {
ObjectMapper objectMapper = createObjectMapper();
objectMapper.addMixIn(Object.class, DynamicMixIn.class);
return objectMapper;
}
/**
* 初始化ObjectMapper
*/
public static ObjectMapper createObjectMapper() {
ObjectMapper objectMapper = new ObjectMapper();
objectMapper.configure(JsonParser.Feature.ALLOW_UNQUOTED_FIELD_NAMES, true);
objectMapper.configure(DeserializationFeature.ACCEPT_EMPTY_STRING_AS_NULL_OBJECT, true);
objectMapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false);
objectMapper.configure(DeserializationFeature.USE_BIG_DECIMAL_FOR_FLOATS, true);
objectMapper.setSerializationInclusion(JsonInclude.Include.NON_NULL);
objectMapper.configure(SerializationFeature.WRITE_DATES_AS_TIMESTAMPS, false);
objectMapper.configure(SerializationFeature.FAIL_ON_EMPTY_BEANS, false);
objectMapper.registerModule(new JavaTimeModule());
return objectMapper;
}
public static String object2Json(Object o) {
StringWriter sw = new StringWriter();
JsonGenerator gen = null;
try {
gen = new JsonFactory().createGenerator(sw);
OBJECT_MAPPER.writeValue(gen, o);
} catch (IOException e) {
throw new RuntimeException("不能序列化对象为Json", e);
} finally {
if (null != gen) {
try {
gen.close();
} catch (IOException e) {
throw new RuntimeException("不能序列化对象为Json", e);
}
}
}
return sw.toString();
}
@SneakyThrows
public static byte[] toJsonByte(Object object) {
return OBJECT_MAPPER.writeValueAsBytes(object);
}
@SneakyThrows
public static String toJsonString(Object object) {
return OBJECT_MAPPER.writeValueAsString(object);
}
/**
* @param ignoreFiledNames 要忽略的属性名
*/
public static String object2Json(Object o, String... ignoreFiledNames) {
try {
SimpleBeanPropertyFilter theFilter = SimpleBeanPropertyFilter.serializeAllExcept(ignoreFiledNames);
FilterProvider filters = new SimpleFilterProvider().addFilter("dynamicFilter", theFilter);
return IGNORE_OBJECT_MAPPER.writer(filters).writeValueAsString(o);
} catch (IOException e) {
throw new RuntimeException("不能序列化对象为Json", e);
}
}
@JsonFilter("dynamicFilter")
private static class DynamicMixIn {
}
public static Map<String, Object> object2Map(Object o) {
return OBJECT_MAPPER.convertValue(o, Map.class);
}
/**
* 将 json 字段串转换为 对象.
*
* @param json 字符串
* @param clazz 需要转换为的类
*/
public static <T> T json2Object(String json, Class<T> clazz) {
try {
return OBJECT_MAPPER.readValue(json, clazz);
} catch (IOException e) {
throw new RuntimeException("将 Json 转换为对象时异常,数据是:" + json, e);
}
}
/**
* 将 json 字段串转换为 List.
*/
public static <T> List<T> json2List(String json, Class<T> clazz) {
JavaType type = OBJECT_MAPPER.getTypeFactory().constructCollectionType(List.class, clazz);
try {
return OBJECT_MAPPER.readValue(json, type);
} catch (Exception e) {
return null;
}
}
/**
* 将 json 字段串转换为 数据.
*/
public static <T> T[] json2Array(String json, Class<T[]> clazz) throws IOException {
return OBJECT_MAPPER.readValue(json, clazz);
}
public static <T> T node2Object(JsonNode jsonNode, Class<T> clazz) {
try {
T t = OBJECT_MAPPER.treeToValue(jsonNode, clazz);
return t;
} catch (JsonProcessingException e) {
throw new RuntimeException("将 Json 转换为对象时异常,数据是:" + jsonNode.toString(), e);
}
}
public static JsonNode object2Node(Object o) {
try {
if (o == null) {
return OBJECT_MAPPER.createObjectNode();
} else {
return OBJECT_MAPPER.convertValue(o, JsonNode.class);
}
} catch (Exception e) {
throw new RuntimeException("不能序列化对象为Json", e);
}
}
/**
* JsonNode转换为Java泛型对象,可以是各种类型。
*
* @param json String
* @param tr TypeReference,例如: new TypeReference< List<FamousUser> >(){}
* @return List对象列表
*/
public static <T> T json2GenericObject(String json, TypeReference<T> tr) {
if (json == null || "".equals(json)) {
throw new RuntimeException("将 Json 转换为对象时异常,数据是:" + json);
} else {
try {
return (T) OBJECT_MAPPER.readValue(json, tr);
} catch (Exception e) {
throw new RuntimeException("将 Json 转换为对象时异常,数据是:" + json, e);
}
}
}
}
4. 网关异常状态码
package cn.cws.framework.gatewayservice.handler.code;
import cn.cws.framework.core.common.constant.ModelEnum;
/**
* @description: 网关异常状态码
* @author:zw,微信:yingshengzw
* @date: 2023/1/16
* @Copyright: 公众号:搬砖暗夜码农 | 博客:https://itzhouwei.com - 沉淀、分享、成长,让自己和他人都能有所收获!
*/
@SuppressWarnings("all")
public enum GatewayErrorCode {
SYS_GATEWAY_ERROR_NACOS(503," 服务没有注册到nacos上");
private String msg;
private int code;
GatewayErrorCode(int code, String msg) {
this.code = code;
this.msg = msg;
}
public String getMsg() {
return msg;
}
public void setMsg(String msg) {
this.msg = msg;
}
public int getCode() {
return code;
}
public void setCode(int code) {
this.code = code;
}
}
5. 测试结果如下
总结
以上就是今天要讲的内容,本文仅仅简单 所以需要配置一个 Gateway 的全局异常处理器
更多推荐
所有评论(0)