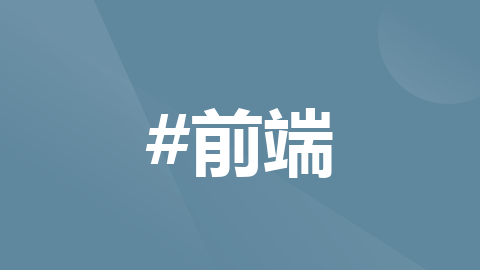
vite+vue3+ts 手把手教你创建一个vue3项目
Vue3+Vite3+TS前端工程化实践

一.pnpm管理项目
为什么选择它?
- 快: pnpm是同类工具速度的将近⒉倍
- 高效: node_modules中的所有文件均链接自单一存储位置
- 支持单体仓库:monorepo,单个源码仓库中包含多个软件包的支持
- 权限严格: pnpm 创建的node_modules默认并非扁平结构,因此代码无法对任意软件包进行访问
二.Vite介绍
- 极速的服务启动,使用原生ESM文件,无需打包!(原来整个项目的代码打包在一起,然后才能启动服务)
- 轻量快速的热重载无论应用程序大小如何,都始终极快的模块热替换(HMR)
- 丰富的功能对TypeScript、JSx、CSS等支持开箱即用。
- 优化的构建可选“多页应用”或“库”模式的预配置 Rollup 构建
- 通用的插件在开发和构建之间共享Rollup-superset插件接口。
- 完全类型化的API灵活的API和完整TypeScript
Vite3修复了400+issuse,减少了体积,Vite决定每年发布一个新的版本
三.项目初始化
pnpm init # 初始化package.json
pnpm install vite -D #安装vite
3.1 package.json
增加启动命令
"scripts": {
"dev": "vite",
"build": "vite build"
},
3.2 index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>vite</title>
</head>
<body>
<!-- 稍后vue项目挂载到这个元素上 -->
<div id="app"></div>
<!-- vite时基于esModule的 -->
<script src="./src/main.ts" type="module"></script>
</body>
</html>
3.3 src/main.ts
pnpm install vue #安装vue
import {createApp} from 'vue';
import App from './App.vue';//这里会报错,不支持.vue
createApp(App).mount('#app');
3.4 src/env.d.ts
// 声明文件,用来识别.vue文件的类型=>垫片 【ts只能处理ts文件,.vue结尾得文件要模块声明】
declare module '*.vue' {
import type {DefineComponent} from 'vue';
const component:DefineComponent<{},{},any>
export default component;
}
3.5 vite.config.ts
我们需要让vite支持.vue文件的解析
pnpm install @vitejs/plugin-vue -D
import { defineConfig } from "vite";
import vue from "@vitejs/plugin-vue";//解析.vue文件
// vite默认只会编译ts
export default defineConfig({
plugins:[vue()]
})
3.6 vue-tsc
- Vite仅执行
.ts
文件的转译工作,并不执行任何类型检查。vue-tsc
可以对Vue3进行Typescript类型校验
pnpm install typescript vue-tsc -D
创建
tsconfig.json
{
"compilerOptions": {
"target": "esnext", // 目标转化的语法
"module": "esnext", // 转化的格式
"moduleResolution": "node", // 解析规则
"strict": true, // 严格模式
"sourceMap": true, // 启动sourcemap调试
"jsx": "preserve", // 不允许ts编译jsx语法
"esModuleInterop": true, // es6和commonjs 转化
"lib": ["esnext", "dom"], // 支持esnext和dom语法
"baseUrl": ".",
"paths": {
"@/*":["src/*"] // @符号的真实含义 还需要配置vite别名 和declare module
}
},
// 编译哪些文件
"include": ["src/**/*.ts", "src/**/*.d.ts", "src/**/*.tsx", "src/**/*.vue","./auto-imports.d.ts"]
}
vue-tsc:
Vue 官方提供的命令,用于执行 TS 的类型检查。它在执行时会根据项目中的 tsconfig.json 文件配置进行类型检查
noEmit:
TS 编译器的选项,使用 --noEmit 选项后,编译器仅执行类型检查,而不会生成任何实际的编译输出
"scripts": {
"dev": "vite",
"build": "vue-tsc --noEmit && vite build"
},
四.Eslint
代码校验配置
校验代码规范
开发项目需要安装vscode
插件volar
npx eslint --init
4.1 校验语法并提示错误行数
? How would you like to use ESLint? ...
To check syntax only # 只校验语法
> To check syntax and find problems # 校验语法,找到问题所在
To check syntax, find problems, and enforce code style # 校验语法,找到问题所在,并且强制代码样式
4.2 采用js-module
? What type of modules does your project use? ...
> JavaScript modules (import/export)
CommonJS (require/exports)
None of these
4.3 项目采用vue
语法
? Which framework does your project use? ...
React
> Vue.js
None of these
4.4 代码运行环境
? Where does your code run?
√ Browser
√ Node
4.5 配置文件用什么风格的
? What format do you want your config file to be in? ...
> JavaScript
YAML
JSON
pnpm i eslint-plugin-vue@latest @typescript-eslint/eslint-plugin@latest @typescript-eslint/parser@latest eslint@latest -D
支持vue中ts
eslint
配置
pnpm i @vue/eslint-config-typescript -D
4.4 .eslintrc.js
module.exports = {
env: {
// 环境 针对哪些环境的语言 window
browser: true,
es2021: true, //new Promise
node: true,
},
/* 继承某些已有的规则 */
extends: [
// 继承了哪些规则,别人写好的规则拿来用
'eslint:recommended',
'plugin:vue/vue3-essential', // eslint-plugin-vue
'plugin:@typescript-eslint/recommended', // typescript 规则 @typescript-eslint/eslint-plugin
],
overrides: [],
// 可以解析.vue文件
parser: 'vue-eslint-parser', // esprima babel-eslint @typescript-eslint/parser
parserOptions: {
parser: '@typescript-eslint/parser', // 解析ts文件的
ecmaVersion: 'latest',
sourceType: 'module',
},
plugins: ['vue', '@typescript-eslint'],
/*
* 自定义规则 plugins + rules= extends
* "off" 或 0 ==> 关闭规则
* "warn" 或 1 ==> 打开的规则作为警告(不影响代码执行)
* "error" 或 2 ==> 规则作为一个错误(代码不能执行,界面报错)
*/
rules: {
// eslint (http://eslint.cn/docs/rules)
"no-var": "error", // 要求使用 let 或 const 而不是 var
"no-multiple-empty-lines": ["error", { max: 1 }], // 不允许多个空行
"no-use-before-define": "off", // 禁止在 函数/类/变量 定义之前使用它们
"prefer-const": "off", // 此规则旨在标记使用 let 关键字声明但在初始分配后从未重新分配的变量,要求使用 const
"no-irregular-whitespace": "off", // 禁止不规则的空白
// typeScript (https://typescript-eslint.io/rules)
"@typescript-eslint/no-unused-vars": "error", // 禁止定义未使用的变量
"@typescript-eslint/prefer-ts-expect-error": "error", // 禁止使用 @ts-ignore
"@typescript-eslint/no-inferrable-types": "off", // 可以轻松推断的显式类型可能会增加不必要的冗长
"@typescript-eslint/no-namespace": "off", // 禁止使用自定义 TypeScript 模块和命名空间。
"@typescript-eslint/no-explicit-any": "off", // 禁止使用 any 类型
"@typescript-eslint/ban-types": "off", // 禁止使用特定类型
"@typescript-eslint/explicit-function-return-type": "off", // 不允许对初始化为数字、字符串或布尔值的变量或参数进行显式类型声明
"@typescript-eslint/no-var-requires": "off", // 不允许在 import 语句中使用 require 语句
"@typescript-eslint/no-empty-function": "off", // 禁止空函数
"@typescript-eslint/no-use-before-define": "off", // 禁止在变量定义之前使用它们
"@typescript-eslint/ban-ts-comment": "off", // 禁止 @ts-<directive> 使用注释或要求在指令后进行描述
"@typescript-eslint/no-non-null-assertion": "off", // 不允许使用后缀运算符的非空断言(!)
"@typescript-eslint/explicit-module-boundary-types": "off", // 要求导出函数和类的公共类方法的显式返回和参数类型
// vue (https://eslint.vuejs.org/rules)
"vue/no-v-html": "off", // 禁止使用 v-html
"vue/script-setup-uses-vars": "error", // 防止<script setup>使用的变量<template>被标记为未使用,此规则仅在启用该no-unused-vars规则时有效。
"vue/v-slot-style": "error", // 强制执行 v-slot 指令样式
"vue/no-mutating-props": "off", // 不允许组件 prop的改变
"vue/custom-event-name-casing": "off", // 为自定义事件名称强制使用特定大小写
"vue/attributes-order": "off", // vue api使用顺序,强制执行属性顺序
"vue/one-component-per-file": "off", // 强制每个组件都应该在自己的文件中
"vue/html-closing-bracket-newline": "off", // 在标签的右括号之前要求或禁止换行
"vue/max-attributes-per-line": "off", // 强制每行的最大属性数
"vue/multiline-html-element-content-newline": "off", // 在多行元素的内容之前和之后需要换行符
"vue/singleline-html-element-content-newline": "off", // 在单行元素的内容之前和之后需要换行符
"vue/attribute-hyphenation": "off", // 对模板中的自定义组件强制执行属性命名样式
"vue/require-default-prop": "off", // 此规则要求为每个 prop 为必填时,必须提供默认值
"vue/multi-word-component-names": "off" // 要求组件名称始终为 “-” 链接的单词
}
};
4.5.eslintignore配置
node_modules
dist
*css
*jpg
*jpeg
*png
*gif
*.d.ts
最终安装
vscode
中eslint
插件:eslint
只是检测代码规范
"scripts": {
"dev": "vite",
"build": "vue-tsc --noEmit && vite build",
"lint": "eslint --fix --ext .ts,.tsx,.vue src --quiet", // src下的.ts,.tsx,.vue文件,忽略warn报错
},
五.Prettier代码风格配置
5.1eslint中进行配置
在eslint中集成prettier配置
# eslint-plugin-prettier:把eslint和prettier关联到一起
# @vue/eslint-config-prettier :关于vue的代码格式化
pnpm install prettier eslint-plugin-prettier @vue/eslint-config-prettier -D
module.exports = {
env: {
// 环境 针对哪些环境的语言 window
browser: true,
es2021: true, //new Promise
node: true
},
extends: [
// 继承了哪些规则,别人写好的规则拿来用
"eslint:recommended",
"plugin:vue/vue3-essential", // eslint-plugin-vue
"plugin:@typescript-eslint/recommended", // typescript 规则
"@vue/eslint-config-prettier" //或者 @vue/prettier
],
overrides: [],
// 可以解析.vue文件
parser: "vue-eslint-parser", // esprima babel-eslint @typescript-eslint/parser
parserOptions: {
parser: "@typescript-eslint/parser", // 解析ts文件的
ecmaVersion: "latest",
sourceType: "module"
},
plugins: ["vue", "@typescript-eslint"],
rules: {
'vue/multi-word-component-names': 'off', //组件命名校验关闭
// 自定义规则// 自带的prettier规则
"prettier/prettier": [
"error",
{
singleQuote: false, // 使用双引号
semi: false, // 末尾添加分号 var a=1
tabWidth: 2, //tab=2
trailingComma: "none", // {a:1,}有无逗号
useTabs: false,
endOfLine: "auto"
}
]
}
}
5.2.prettierrc.js
格式化代码风格 =>给vscode插件用的
要和上面的rules中的"prettier/prettier"
里的配置统一
module.exports = {
singleQuote: false, // 使用双引号
semi: false, // 末尾添加分号 var a=1
tabWidth: 2, //tab=2
trailingComma: "none", // {a:1,}
useTabs: false,
endOfLine: "auto"
}
.prettierignore
node_modules
dist
最终安装
vscode
中Prettier
插件:prettier
只是用来格式化代码这里需要配置
Format On Save
为启用,保存时自动格式化Default Foramtter
选择Prettier - Code formatter
.eslint
和.prettierrc.js
要配合使用
5.3 editorconfig-编译器配置
编译器配置
http://editorconfig.org
.editorconfig
root=true
[*] # 表示所有文件适用
charset=utf-8 # 设置文件字符集为 utf-8
indent_style=space # 缩进风格(tab | space)
indent-size=2 # 缩进大小
end_of_line=lf # 控制换行类型(lf | cr | crlf)
trim_trailing_whitespace = true # 去除行首的任意空白字符
insert_final_newline = true # 始终在文件末尾插入一个新行
[*.md] # 表示仅 md 文件适用以下规则
max_line_length = off
trim_trailing_whitespace = false
最终安装
vscode
中EditorConfig for VS Code
插件
六.husky
git init
pnpm install husky -D
"scripts": {
"dev": "vite",
"build": "vue-tsc --noEmit && vite build",
"lint": "eslint --fix --ext .ts,.tsx,.vue src --quiet",
+ "prepare":"husky install"
},
npx husky add .husky/pre-commit "pnpm lint" # 添加提交之前的钩子,会执行pnpm lint做代码校验
七.commitlint
类型 | 描述 |
---|---|
build | 主要目的是修改项目构建系统(例如glup,webpack,rollup的配置)的提交 |
chore | 不属于以上类型的其它类型 |
ci | 主要目的是修改项目继续集成流程(例如Travis,Jenkins,GitLab Cl,Circle等)的提交 |
docs | 文档更新 |
feat | 新功能、新特性 |
fix | 修改bug |
perf | 更改代码,以提高性能 |
refactor | 代码重构(重构,在不影响代码内部行为、功能下的代码修改) |
revert | 恢复上一次提交 |
style | 不影响程序逻辑的代码修改(修改空白字符,格式缩进,补全缺失的分号等,没有改变代码逻辑) |
test | 测试用例新增、修改 |
代码提交检测
pnpm install @commitlint/cli @commitlint/config-conventional -D
npx husky add .husky/commit-msg "npx --no-install commitlint --edit $1"
commitlint.config.js
配置
module.exports = {
// 继承的规则
extends: ['@commitlint/config-conventional'],
// 定义规则类型,可有可无
rules: {
// type 类型定义,表示 git 提交的 type 必须在以下类型范围内
'type-enum': [
2,
'always',
[
'feat', // 新功能 feature
'fix', // 修复 bug
'docs', // 文档注释
'style', // 代码格式(不影响代码运行的变动)
'refactor', // 重构(既不增加新功能,也不是修复bug)
'perf', // 性能优化
'test', // 增加测试
'chore', // 构建过程或辅助工具的变动
'revert', // 回退
'build' // 打包
]
],
// subject 大小写不做校验
'subject-case': [0]
}
};
git commit -m"feat: 初始化工程"
八.路由配置
pnpm install vue-router
import { createRouter, createWebHistory } from "vue-router"
const getRoutes = () => {
const files = import.meta.glob("../views/*.vue")
// ../views/About.vue () => import("/src/views/About.vue")
// ../views/Home.vue () => import("/src/views/Home.vue")
return Object.entries(files).map(([file, module]) => {
console.log(file, module)
const name = file
.match(/\.\.\/views\/([^.]+?)\.vue/i)?.[1]
?.toLocaleLowerCase()
console.log(name)
return {
path: "/" + name,
component: module
}
})
}
export default createRouter({
history: createWebHistory(),
routes: getRoutes()
// [
// {
// path: "/home",
// component: () => import("../views/home.vue")
// }
// ]
})
// env.d.ts文件
/// <reference types="vite/client"/>
// main.ts
import router from "./router/index"
createApp(App).use(router).mount("#app")
九.组件name属性设置
pnpm i vite-plugin-vue-setup-extend
1.配置vite.config.ts
import { defineConfig } from 'vite'
import VueSetupExtend from 'vite-plugin-vue-setup-extend'
export default defineConfig({
plugins:[vue(), VueSetupExtend()]
})
2.使用
<script setup name="User">
</script>
十.编写功能
1.自动引入插件
pnpm install unplugin-auto-import -D
vite.config.ts
生成
.eslintrc-auto-import.json
文件和auto-imports.d.ts
文件
import AutoImport from "unplugin-auto-import/vite"
export default defineConfig({
plugins: [
vue(),
AutoImport({
imports: ["vue", "vue-router"] //生成auto-imports.d.ts文件
// eslintrc: { enabled: true } //第一次启动生成就不用生成了 生成.eslintrc-auto-import.json文件
})
]
})
.eslintrc.js
让eslint支持
module.exports = {
extends: [
// 继承了哪些规则,别人写好的规则拿来用
"eslint:recommended",
"plugin:vue/vue3-essential", // eslint-plugin-vue
"plugin:@typescript-eslint/recommended", // typescript 规则
"@vue/eslint-config-prettier",
+ "./.eslintrc-auto-import.json"
],
}
tsconfig.json
让ts支持
{
// 编译哪些文件
"include": [
"src/**/*.ts",
"src/**/*.d.ts",
"src/**/*.tsx",
"src/**/*.vue",
"./auto-imports.d.ts"
]
}
2.路径别名
vite.config.ts
import path from "path"
resolve: {
alias: [
// 配置和rollup一样
{ find: "@", replacement: path.resolve(__dirname, "src") }
]
}
tsconfig.json
import Todo from "@/components/todo/index.vue"
点他跳转到对应文件
{
"compilerOptions": {
"baseUrl": ".",
"paths": {
"@/*": ["src/*"] // @符号的真实含义 还需要配置vite别名 和declare module
},
},
}
3.解析TSX文件
pnpm install @vitejs/plugin-vue-jsx -D
vite.config.ts
import jsx from "@vitejs/plugin-vue-jsx"
// vite默认只会编译ts
export default defineConfig({
plugins: [
jsx(),
],
})
更多推荐
所有评论(0)