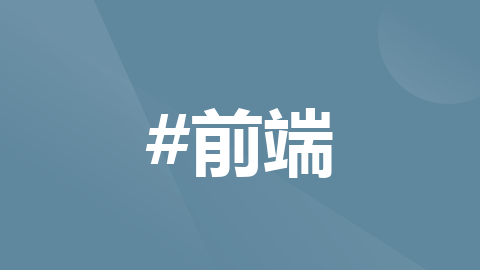
Vue3 + ESLint + Prettier
Vue3 + ESLint + Prettier,setting.json配置,文件嵌套显示
Vue3 + ESLint + Prettier 配置规则
1 初始化项目
新建 vue-cli 项目。如果已有项目,可直接跳到最后一步。
① 在 cmd 中输入如下代码,开始创建项目。
提示:如果没有安装 vue-cli ,执行
npm install -g @vue/cli
安装即可。
vue create "项目名称"
② 选择 Manually select features(手动选择功能)。
③ 根据自己需求选择,这里只选择了 Linter / Formatter。
④ 选择版本。
⑤ 选择 ESLint + Prettier。
⑥ 选择 Lint on save。
⑦ 选择 In dedicated config files。
⑧ 是否将此次配置保存为未来项目的预设。
⑨ 如果项目中未安装 ESLint 和 Prettier 则需要安装如下依赖,可对照下图进行配置。
注意:请去掉 package.json 中的
"type": "module"
属性(如果它存在的话)。
npm i @babel/core -D
npm i @babel/eslint-parser -D
npm i @vue/cli-plugin-eslint -D
npm i eslint -D
npm i eslint-config-prettier -D
npm i eslint-plugin-prettier -D
npm i eslint-plugin-vue -D
npm i prettier -D
// 用到 TS 的需要加入如下依赖
npm i @typescript-eslint/eslint-plugin -D
npm i @typescript-eslint/parser -D
npm i @vue/cli-plugin-typescript -D
npm i @vue/eslint-config-typescript -D
npm i typescript -D
至此,初始化项目完成。
2 配置 ESLint
① 安装 ESLint 扩展。
② 配置 eslint 规则文件,在项目根目录下创建 .eslintrc.js
文件,然后加入如下配置。
以下只是一些配置,具体可参考 ESLint 中文网
module.exports = {
root: true,
env: {
node: true
},
extends: [
"plugin:vue/vue3-essential",
"eslint:recommended",
// "@vue/typescript/recommended", // ts 支持
"plugin:prettier/recommended",
],
parserOptions: {
parser: '@babel/eslint-parser'
},
overrides: [
{
files: ['**/__tests__/*.{j,t}s?(x)', '**/tests/unit/**/*.spec.{j,t}s?(x)'],
env: {
jest: true
}
}
],
rules: {
/*
"off" 或 0 - 关闭规则
"warn" 或 1 - 打开规则作为警告(不影响退出代码)
"error" 或 2 - 打开规则作为错误(触发时退出代码为 1)
*/
'array-bracket-spacing': [2, 'never'], // 在数组括号内强制保持一致的间距
'block-spacing': [2, 'always'], // 在打开块之后和关闭块之前禁止或强制块内的空格
'brace-style': [2, '1tbs'], // 对块执行一致的大括号样式
'comma-dangle': [2, 'never'], // 不允许尾随逗号
'comma-spacing': [
2,
{
before: false,
after: true
}
], // 在逗号前后强制保持一致的间距
'comma-style': [2, 'last'], // 强制使用一致的逗号样式
'consistent-return': [
2,
{
treatUndefinedAsUnspecified: true
}
], // 要求 return 语句始终或从不指定值
'computed-property-spacing': [2, 'never'], // 在计算的属性括号内强制执行一致的间距
'constructor-super': 2, // 在构造函数中需要 super() 调用
curly: 2, // 强制所有控制语句使用一致的括号风格
'default-case': 2, // 在 switch 语句中需要 default 个案例
'eol-last': [2, 'always'], // 在文件末尾要求或禁止换行
'func-call-spacing': [2, 'never'], // 要求或不允许函数标识符及其调用之间有间距
'guard-for-in': 2, // 要求 for-in 循环包含 if 语句
indent: [
2,
2,
{
SwitchCase: 1
}
], // 强制一致的缩进
'jsx-quotes': [2, 'prefer-double'], // 强制在 JSX 属性中一致地使用双引号或单引号
'key-spacing': [
2,
{
beforeColon: false,
afterColon: true
}
], // 在对象字面属性中强制键和值之间的间距一致
'new-cap': 0, // 设置名字首字母为大写的函数可以不为构造函数
/* 'new-cap': [
2,
{
newIsCap: true,
capIsNewExceptionPattern: '(Type[a-zA-Z0-9]+|Deco[a-zA-Z0-9]+)+'
}
], */
'new-parens': 2, // 在调用不带参数的构造函数时强制或禁止使用括号
'no-case-declarations': 2, // 不允许在case/default子句中使用词法声明
'no-class-assign': 2, // 不允许重新分配类成员
'no-compare-neg-zero': 2, // 禁止与 -0 进行比较
'no-cond-assign': [2, 'always'], // 禁止条件语句中出现赋值操作符
'no-console': 0, // 允许出现console
'no-const-assign': 2, // 建议使用const
'no-constant-condition': 2, // 禁止在条件中使用常量表达式
'no-control-regex': 2, // 禁止在正则表达式中使用控制字符
'no-debugger': 0, // 可以使用debugger
'no-delete-var': 2, // 不允许删除变量
'no-dupe-args': 2, // 禁止 function 定义中出现重名参数
'no-dupe-class-members': 2, // 禁止重复的类成员
'no-dupe-keys': 2, // 禁止对象字面量中出现重复的 key
'no-duplicate-case': 2, // 禁止出现重复的 case 标签
'no-empty': 2, // 禁止出现空语句块
'no-empty-character-class': 2, // 禁止在正则表达式中使用空字符集
'no-empty-pattern': 2, // 禁止空的解构模式
'no-ex-assign': 2, // 禁止对 catch 子句的参数重新赋值
'no-extra-boolean-cast': 2, // 禁止不必要的布尔转换
'no-extra-parens': [2, 'functions'], // 禁止不必要的括号
'no-extra-semi': 0, // 取消禁止不必要的分号
'no-fallthrough': 2, // 不允许 case 语句的失败
'no-func-assign': 2, // 禁止对 function 声明重新赋值
'no-global-assign': [
2,
{
exceptions: []
}
], // 不允许分配给原生对象或只读全局变量
'no-inner-declarations': 0, // 禁止在嵌套的块中出现变量声明或 function 声明
'no-invalid-regexp': 2, // 禁止 RegExp 构造函数中存在无效的正则表达式字符串
'no-irregular-whitespace': 2, // 禁止不规则的空白
'no-mixed-spaces-and-tabs': 2, // 不允许使用混合空格和制表符进行缩进
'no-multi-assign': 2, // 禁止使用链式赋值表达式
'no-multiple-empty-lines': 2, // 禁止多个空行
'no-new-symbol': 2, // 禁止带有 Symbol 对象的 new 运算符
'no-obj-calls': 2, // 禁止把全局对象作为函数调用
'no-octal': 2, // 禁止八进制字面
'no-prototype-builtins': 2, // 禁止直接调用 Object.prototypes 的内置属性
'no-redeclare': 2, // 禁止变量重新声明
'no-regex-spaces': 2, // 禁止正则表达式字面量中出现多个空格
'no-self-assign': 2, // 禁止两边完全相同的赋值
'no-sparse-arrays': 2, // 禁用稀疏数组
'no-template-curly-in-string': 0, // 禁止在常规字符串中出现模板字面量占位符语法
'no-this-before-super': 2, // 在构造函数中调用 super() 之前禁止 this/super
'no-undef': 0, // 除非在 /*global */ 注释中提及,否则不允许使用未声明的变量
'no-undefined': 0, // 禁止使用 undefined 作为标识符
'no-unexpected-multiline': 2, // 禁止出现令人困惑的多行表达式
'no-unreachable': 2, // 禁止在 return、throw、continue 和 break 语句之后出现不可达代码
'no-unsafe-finally': 2, // 禁止在 finally 语句块中出现控制流语句
'no-unsafe-negation': 2, // 禁止对关系运算符的左操作数使用否定操作符
'no-unused-labels': 2, // 禁止未使用的标签
'no-use-before-define': 2, // 在定义之前禁止使用变量
'no-useless-escape': 2, // 禁止不必要的转义字符
'no-var': 2, // 禁止使用var
'prefer-const': 2, // 声明后从不重新分配的变量需要 const 声明
quotes: 0, // 双引号可无
'require-yield': 2, // 要求生成器函数包含 yield
semi: 0, // 句尾省略分号
'space-before-function-paren': 0, // 在函数括号之前不使用间距
strict: 2, // 要求或禁止严格模式指令
'use-isnan': 2, // 要求使用 isNaN() 检查 NaN
'valid-jsdoc': 0, // 强制执行有效且一致的 JSDoc 注释
'valid-typeof': 2, // 强制 typeof 表达式与有效的字符串进行比较
'vue/html-self-closing': [
2,
{
html: {
void: 'always',
normal: 'always',
component: 'always'
},
svg: 'always',
math: 'always'
}
], // 设置自闭合标签
/* <template>
<!-- ✓ GOOD -->
<div/>
<img/>
<MyComponent/>
<svg><path d=""/></svg>
<!-- ✗ BAD -->
<div></div>
<img>
<MyComponent></MyComponent>
<svg><path d=""></path></svg>
</template>
*/
'vue/max-attributes-per-line': [
1,
{
singleline: {
max: 1
},
multiline: {
max: 1
}
}
], // vue/每行最大属性 在单行情况下可接受的属性数量可配置(默认值为 1),在多行情况下,每行可接受的属性数量可配置(默认值 1)。
'vue/singleline-html-element-content-newline': 0 // 在单行元素的内容之前和之后强制使用换行符
},
}
③ 配置 eslint 忽略文件,在项目根目录下创建 .eslintignore
文件,然后加入如下配置。
配置忽略文件后,eslint 不会再检查与忽略文件的规则相匹配的文件或目录,可以按需自定义规则。
*.sh
node_modules
*.md
*.woff
*.ttf
.vscode
.idea
dist
/public
/docs
.husky
.local
/bin
Dockerfile
3 配置 Prettier
① 安装 Prettier 扩展。
② 配置 prettier 规则文件,在项目根目录下创建 .prettierrc.js
文件,然后加入如下配置。
可以根据需求自定义规则,具体可查看 prettier 规则。
module.exports = {
overrides: [
{
files: '.prettierrc',
options: {
parser: 'json'
}
}
],
printWidth: 120, // 一行最多 120 字符
tabWidth: 2, // 使用 2 个空格缩进
semi: false, // 句尾省略分号
singleQuote: true, // 使用单引号而不是双引号
singleAttributePerLine: true, // 多个 attribute 的元素应该分多行撰写,每个 attribute 一行;只有一个 attribute 的一行显示
useTabs: false, // 用制表符而不是空格缩进行
quoteProps: 'as-needed', // 仅在需要时在对象属性两边添加引号
jsxSingleQuote: false, // 在 JSX 中使用单引号而不是双引号
trailingComma: 'none', // 末尾不需要逗号
bracketSpacing: true, // 大括号内的首尾需要空格
bracketSameLine: false, // 将多行 HTML(HTML、JSX、Vue、Angular)元素反尖括号需要换行
arrowParens: 'always', // 箭头函数,只有一个参数的时候,也需要括号 avoid
rangeStart: 0, // 每个文件格式化的范围是开头-结束
rangeEnd: Infinity, // 每个文件格式化的范围是文件的全部内容
requirePragma: false, // 不需要写文件开头的 @prettier
insertPragma: false, // 不需要自动在文件开头插入 @prettier
proseWrap: 'preserve', // 使用默认的折行标准 always
htmlWhitespaceSensitivity: 'ignore', // 根据显示样式决定 html 要不要折行
vueIndentScriptAndStyle: false, //(默认值)对于 .vue 文件,不缩进 <script> 和 <style> 里的内容
endOfLine: 'lf', // 换行符使用 lf 在Linux和macOS以及git存储库内部通用\n
embeddedLanguageFormatting: 'auto', //(默认值)允许自动格式化内嵌的代码块,
}
③ 配置 prettier 忽略文件,在项目根目录下创建 .prettierignore
文件,然后加入如下配置。
配置忽略文件后通过运行
prettier --write
,可以格式化所有内容(不会破坏你不想要的文件,或阻塞生成的文件),可以根据需求自定义。
dist
.local
.output.js
node_modules
**/*.svg
**/*.sh
public
.npmrc
*-lock.yaml
4 配置 settings.json
这里配置的是本地的 settings.json 文件,若只在项目中使用,在项目根目录 .vscode 文件夹下的 settings.json 中配置。
① 打开 settings.json 文件。
② 将以下代码加入 settings.json 文件中(按需添加)。
{
"workbench.iconTheme": "vscode-icons",
"editor.tabSize": 2,
"editor.fontSize": 16,
"editor.minimap.enabled": false,
"workbench.colorTheme": "Atom One Dark",
"editor.defaultFormatter": "esbenp.prettier-vscode",
"workbench.startupEditor": "none",
"emmet.triggerExpansionOnTab": true,
"files.eol": "\n",
"search.exclude": {
"**/node_modules": true,
"**/*.log": true,
"**/*.log*": true,
"**/bower_components": true,
"**/dist": true,
"**/elehukouben": true,
"**/.git": true,
"**/.gitignore": true,
"**/.svn": true,
"**/.DS_Store": true,
"**/.idea": true,
"**/.vscode": false,
"**/yarn.lock": true,
"**/tmp": true,
"out": true,
"dist": true,
"node_modules": true,
"CHANGELOG.md": true,
"examples": true,
"res": true,
"screenshots": true,
"yarn-error.log": true,
"**/.yarn": true
},
"files.exclude": {
"**/.cache": true,
"**/.editorconfig": true,
"**/.eslintcache": true,
"**/bower_components": true,
"**/.idea": true,
"**/tmp": true,
"**/.git": true,
"**/.svn": true,
"**/.hg": true,
"**/CVS": true,
"**/.DS_Store": true
},
"files.watcherExclude": {
"**/.git/objects/**": true,
"**/.git/subtree-cache/**": true,
"**/.vscode/**": true,
"**/node_modules/**": true,
"**/tmp/**": true,
"**/bower_components/**": true,
"**/dist/**": true,
"**/yarn.lock": true
},
"files.associations": {
"*.scss": "scss"
},
// 配置保存时要进行的代码操作
"editor.formatOnSave": true,
"editor.codeActionsOnSave": {
"source.fixAll.eslint": "explicit",
"source.fixAll.stylelint": "explicit"
},
// stylelint
"stylelint.enable": true,
"stylelint.validate": ["css", "less", "postcss", "scss", "vue", "sass"],
// liveSassCompile
"liveSassCompile.settings.generateMap": false,
"liveSassCompile.settings.formats": [
{
"format": "expanded",
"extensionName": ".css",
"savePath": "/assets/css",
"savePathReplacementPairs": null
},
{
"format": "compressed",
"extensionName": ".min.css",
"savePath": "/assets/css",
"savePathReplacementPairs": null
}
],
"liveSassCompile.settings.showOutputWindowOn": "Error",
"liveSassCompile.settings.autoprefix": ["> 1%", "last 3 versions"],
// i18n-ally
"i18n-ally.localesPaths": ["src/locales/lang"],
"i18n-ally.keystyle": "nested",
"i18n-ally.sortKeys": true,
"i18n-ally.namespace": true,
"i18n-ally.pathMatcher": "{locale}/{namespaces}.{ext}",
"i18n-ally.enabledParsers": ["json"],
"i18n-ally.sourceLanguage": "en",
"i18n-ally.displayLanguage": "zh-CN",
"i18n-ally.enabledFrameworks": ["vue", "react"],
// 针对某种语言,配置替代编辑器设置
"[javascriptreact]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[typescript]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[typescriptreact]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[html]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[css]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[less]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[scss]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[markdown]": {
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
"[vue]": {
"editor.codeActionsOnSave": {
"source.fixAll.eslint": "explicit",
"source.fixAll.stylelint": "explicit"
},
"editor.defaultFormatter": "esbenp.prettier-vscode"
},
// 控制相关文件嵌套展示
"explorer.fileNesting.enabled": true,
"explorer.fileNesting.expand": false,
"explorer.fileNesting.patterns": {
"*.asax": "$(capture).*.cs, $(capture).*.vb",
"*.ascx": "$(capture).*.cs, $(capture).*.vb",
"*.ashx": "$(capture).*.cs, $(capture).*.vb",
"*.aspx": "$(capture).*.cs, $(capture).*.vb",
"*.bloc.dart": "$(capture).event.dart, $(capture).state.dart",
"*.c": "$(capture).h",
"*.cc": "$(capture).hpp, $(capture).h, $(capture).hxx",
"*.component.ts": "$(capture).component.html, $(capture).component.spec.ts, $(capture).component.css, $(capture).component.scss, $(capture).component.sass, $(capture).component.less",
"*.cpp": "$(capture).hpp, $(capture).h, $(capture).hxx",
"*.cs": "$(capture).*.cs",
"*.cshtml": "$(capture).cshtml.cs",
"*.csproj": "*.config, *proj.user, appsettings.*, bundleconfig.json",
"*.css": "$(capture).css.map, $(capture).*.css",
"*.cxx": "$(capture).hpp, $(capture).h, $(capture).hxx",
"*.dart": "$(capture).freezed.dart, $(capture).g.dart",
"*.ex": "$(capture).html.eex, $(capture).html.heex, $(capture).html.leex",
"*.go": "$(capture)_test.go",
"*.java": "$(capture).class",
"*.js": "$(capture).js.map, $(capture).*.js, $(capture)_*.js",
"*.jsx": "$(capture).js, $(capture).*.jsx, $(capture)_*.js, $(capture)_*.jsx",
"*.master": "$(capture).*.cs, $(capture).*.vb",
"*.module.ts": "$(capture).resolver.ts, $(capture).controller.ts, $(capture).service.ts",
"*.pubxml": "$(capture).pubxml.user",
"*.resx": "$(capture).*.resx, $(capture).designer.cs, $(capture).designer.vb",
"*.tex": "$(capture).acn, $(capture).acr, $(capture).alg, $(capture).aux, $(capture).bbl, $(capture).blg, $(capture).fdb_latexmk, $(capture).fls, $(capture).glg, $(capture).glo, $(capture).gls, $(capture).idx, $(capture).ind, $(capture).ist, $(capture).lof, $(capture).log, $(capture).lot, $(capture).out, $(capture).pdf, $(capture).synctex.gz, $(capture).toc, $(capture).xdv",
"*.ts": "$(capture).js, $(capture).d.ts.map, $(capture).*.ts, $(capture)_*.js, $(capture)_*.ts",
"*.tsx": "$(capture).ts, $(capture).*.tsx, $(capture)_*.ts, $(capture)_*.tsx",
"*.vbproj": "*.config, *proj.user, appsettings.*, bundleconfig.json",
"*.vue": "$(capture).*.ts, $(capture).*.js, $(capture).story.vue",
"*.xaml": "$(capture).xaml.cs",
"+layout.svelte": "+layout.ts,+layout.ts,+layout.js,+layout.server.ts,+layout.server.js",
"+page.svelte": "+page.server.ts,+page.server.js,+page.ts,+page.js ",
".clang-tidy": ".clang-format, .clangd, compile_commands.json",
".env": "*.env, .env.*, .envrc, env.d.ts",
".gitignore": ".gitattributes, .gitmodules, .gitmessage, .mailmap, .git-blame*",
".project": ".classpath",
"BUILD.bazel": "*.bzl, *.bazel, *.bazelrc, bazel.rc, .bazelignore, .bazelproject, WORKSPACE",
"CMakeLists.txt": "*.cmake, *.cmake.in, .cmake-format.yaml, CMakePresets.json",
"I*.cs": "$(capture).cs",
"artisan": "*.env, .babelrc*, .codecov, .cssnanorc*, .env.*, .envrc, .htmlnanorc*, .lighthouserc.*, .mocha*, .postcssrc*, .terserrc*, api-extractor.json, ava.config.*, babel.config.*, contentlayer.config.*, cssnano.config.*, cypress.*, env.d.ts, formkit.config.*, formulate.config.*, histoire.config.*, htmlnanorc.*, jasmine.*, jest.config.*, jsconfig.*, karma*, lighthouserc.*, playwright.config.*, postcss.config.*, puppeteer.config.*, server.php, svgo.config.*, tailwind.config.*, tsconfig.*, tsdoc.*, unocss.config.*, vitest.config.*, webpack.config.*, webpack.mix.js, windi.config.*",
"astro.config.*": "*.env, .babelrc*, .codecov, .cssnanorc*, .env.*, .envrc, .htmlnanorc*, .lighthouserc.*, .mocha*, .postcssrc*, .terserrc*, api-extractor.json, ava.config.*, babel.config.*, contentlayer.config.*, cssnano.config.*, cypress.*, env.d.ts, formkit.config.*, formulate.config.*, histoire.config.*, htmlnanorc.*, jasmine.*, jest.config.*, jsconfig.*, karma*, lighthouserc.*, playwright.config.*, postcss.config.*, puppeteer.config.*, svgo.config.*, tailwind.config.*, tsconfig.*, tsdoc.*, unocss.config.*, vitest.config.*, webpack.config.*, windi.config.*",
"cargo.toml": ".clippy.toml, .rustfmt.toml, cargo.lock, clippy.toml, cross.toml, rust-toolchain.toml, rustfmt.toml",
"composer.json": ".php*.cache, composer.lock, phpunit.xml*, psalm*.xml",
"default.nix": "shell.nix",
"deno.json*": "*.env, .env.*, .envrc, api-extractor.json, env.d.ts, import-map.json, import_map.json, jsconfig.*, tsconfig.*, tsdoc.*",
"dockerfile": ".dockerignore, docker-compose.*, dockerfile*",
"flake.nix": "flake.lock",
"gatsby-config.*": "*.env, .babelrc*, .codecov, .cssnanorc*, .env.*, .envrc, .htmlnanorc*, .lighthouserc.*, .mocha*, .postcssrc*, .terserrc*, api-extractor.json, ava.config.*, babel.config.*, contentlayer.config.*, cssnano.config.*, cypress.*, env.d.ts, formkit.config.*, formulate.config.*, gatsby-browser.*, gatsby-node.*, gatsby-ssr.*, gatsby-transformer.*, histoire.config.*, htmlnanorc.*, jasmine.*, jest.config.*, jsconfig.*, karma*, lighthouserc.*, playwright.config.*, postcss.config.*, puppeteer.config.*, svgo.config.*, tailwind.config.*, tsconfig.*, tsdoc.*, unocss.config.*, vitest.config.*, webpack.config.*, windi.config.*",
"gemfile": ".ruby-version, gemfile.lock",
"go.mod": ".air*, go.sum",
"go.work": "go.work.sum",
"mix.exs": ".credo.exs, .dialyzer_ignore.exs, .formatter.exs, .iex.exs, .tool-versions, mix.lock",
"next.config.*": "*.env, .babelrc*, .codecov, .cssnanorc*, .env.*, .envrc, .htmlnanorc*, .lighthouserc.*, .mocha*, .postcssrc*, .terserrc*, api-extractor.json, ava.config.*, babel.config.*, contentlayer.config.*, cssnano.config.*, cypress.*, env.d.ts, formkit.config.*, formulate.config.*, histoire.config.*, htmlnanorc.*, jasmine.*, jest.config.*, jsconfig.*, karma*, lighthouserc.*, next-env.d.ts, playwright.config.*, postcss.config.*, puppeteer.config.*, svgo.config.*, tailwind.config.*, tsconfig.*, tsdoc.*, unocss.config.*, vitest.config.*, webpack.config.*, windi.config.*",
"nuxt.config.*": "*.env, .babelrc*, .codecov, .cssnanorc*, .env.*, .envrc, .htmlnanorc*, .lighthouserc.*, .mocha*, .postcssrc*, .terserrc*, api-extractor.json, ava.config.*, babel.config.*, contentlayer.config.*, cssnano.config.*, cypress.*, env.d.ts, formkit.config.*, formulate.config.*, histoire.config.*, htmlnanorc.*, jasmine.*, jest.config.*, jsconfig.*, karma*, lighthouserc.*, playwright.config.*, postcss.config.*, puppeteer.config.*, svgo.config.*, tailwind.config.*, tsconfig.*, tsdoc.*, unocss.config.*, vitest.config.*, webpack.config.*, windi.config.*",
"package.json": ".browserslist*, .circleci*, .commitlint*, .cz-config.js, .czrc, .dlint.json, .dprint.json, .editorconfig, .eslint*, .firebase*, .flowconfig, .github*, .gitlab*, .gitpod*, .huskyrc*, .jslint*, .lintstagedrc*, .markdownlint*, .node-version, .nodemon*, .npm*, .nvmrc, .pm2*, .pnp.*, .pnpm*, .prettier*, .releaserc*, .sentry*, .stackblitz*, .styleci*, .stylelint*, .tazerc*, .textlint*, .tool-versions, .travis*, .versionrc*, .vscode*, .watchman*, .xo-config*, .yamllint*, .yarnrc*, Procfile, apollo.config.*, appveyor*, azure-pipelines*, bower.json, build.config.*, commitlint*, crowdin*, dangerfile*, dlint.json, dprint.json, firebase.json, grunt*, gulp*, jenkins*, lerna*, lint-staged*, nest-cli.*, netlify*, nodemon*, nx.*, package-lock.json, package.nls*.json, phpcs.xml, pm2.*, pnpm*, prettier*, pullapprove*, pyrightconfig.json, release-tasks.sh, renovate*, rollup.config.*, stylelint*, tslint*, tsup.config.*, turbo*, typedoc*, unlighthouse*, vercel*, vetur.config.*, webpack*, workspace.json, xo.config.*, yarn*",
"pubspec.yaml": ".metadata, .packages, all_lint_rules.yaml, analysis_options.yaml, build.yaml, pubspec.lock, pubspec_overrides.yaml",
"pyproject.toml": ".pdm.toml, pdm.lock, pyproject.toml",
"quasar.conf.js": "*.env, .babelrc*, .codecov, .cssnanorc*, .env.*, .envrc, .htmlnanorc*, .lighthouserc.*, .mocha*, .postcssrc*, .terserrc*, api-extractor.json, ava.config.*, babel.config.*, contentlayer.config.*, cssnano.config.*, cypress.*, env.d.ts, formkit.config.*, formulate.config.*, histoire.config.*, htmlnanorc.*, jasmine.*, jest.config.*, jsconfig.*, karma*, lighthouserc.*, playwright.config.*, postcss.config.*, puppeteer.config.*, quasar.extensions.json, svgo.config.*, tailwind.config.*, tsconfig.*, tsdoc.*, unocss.config.*, vitest.config.*, webpack.config.*, windi.config.*",
"readme*": "authors, backers*, changelog*, citation*, code_of_conduct*, codeowners, contributing*, contributors, copying, credits, governance.md, history.md, license*, maintainers, readme*, security.md, sponsors*",
"remix.config.*": "*.env, .babelrc*, .codecov, .cssnanorc*, .env.*, .envrc, .htmlnanorc*, .lighthouserc.*, .mocha*, .postcssrc*, .terserrc*, api-extractor.json, ava.config.*, babel.config.*, contentlayer.config.*, cssnano.config.*, cypress.*, env.d.ts, formkit.config.*, formulate.config.*, histoire.config.*, htmlnanorc.*, jasmine.*, jest.config.*, jsconfig.*, karma*, lighthouserc.*, playwright.config.*, postcss.config.*, puppeteer.config.*, remix.*, svgo.config.*, tailwind.config.*, tsconfig.*, tsdoc.*, unocss.config.*, vitest.config.*, webpack.config.*, windi.config.*",
"rush.json": ".browserslist*, .circleci*, .commitlint*, .cz-config.js, .czrc, .dlint.json, .dprint.json, .editorconfig, .eslint*, .firebase*, .flowconfig, .github*, .gitlab*, .gitpod*, .huskyrc*, .jslint*, .lintstagedrc*, .markdownlint*, .node-version, .nodemon*, .npm*, .nvmrc, .pm2*, .pnp.*, .pnpm*, .prettier*, .releaserc*, .sentry*, .stackblitz*, .styleci*, .stylelint*, .tazerc*, .textlint*, .tool-versions, .travis*, .versionrc*, .vscode*, .watchman*, .xo-config*, .yamllint*, .yarnrc*, Procfile, apollo.config.*, appveyor*, azure-pipelines*, bower.json, build.config.*, commitlint*, crowdin*, dangerfile*, dlint.json, dprint.json, firebase.json, grunt*, gulp*, jenkins*, lerna*, lint-staged*, nest-cli.*, netlify*, nodemon*, nx.*, package-lock.json, package.nls*.json, phpcs.xml, pm2.*, pnpm*, prettier*, pullapprove*, pyrightconfig.json, release-tasks.sh, renovate*, rollup.config.*, stylelint*, tslint*, tsup.config.*, turbo*, typedoc*, unlighthouse*, vercel*, vetur.config.*, webpack*, workspace.json, xo.config.*, yarn*",
"shims.d.ts": "*.d.ts",
"svelte.config.*": "*.env, .babelrc*, .codecov, .cssnanorc*, .env.*, .envrc, .htmlnanorc*, .lighthouserc.*, .mocha*, .postcssrc*, .terserrc*, api-extractor.json, ava.config.*, babel.config.*, contentlayer.config.*, cssnano.config.*, cypress.*, env.d.ts, formkit.config.*, formulate.config.*, histoire.config.*, htmlnanorc.*, jasmine.*, jest.config.*, jsconfig.*, karma*, lighthouserc.*, mdsvex.config.js, playwright.config.*, postcss.config.*, puppeteer.config.*, svgo.config.*, tailwind.config.*, tsconfig.*, tsdoc.*, unocss.config.*, vitest.config.*, webpack.config.*, windi.config.*",
"vite.config.*": "*.env, .babelrc*, .codecov, .cssnanorc*, .env.*, .envrc, .htmlnanorc*, .lighthouserc.*, .mocha*, .postcssrc*, .terserrc*, api-extractor.json, ava.config.*, babel.config.*, contentlayer.config.*, cssnano.config.*, cypress.*, env.d.ts, formkit.config.*, formulate.config.*, histoire.config.*, htmlnanorc.*, jasmine.*, jest.config.*, jsconfig.*, karma*, lighthouserc.*, playwright.config.*, postcss.config.*, puppeteer.config.*, svgo.config.*, tailwind.config.*, tsconfig.*, tsdoc.*, unocss.config.*, vitest.config.*, webpack.config.*, windi.config.*",
"vue.config.*": "*.env, .babelrc*, .codecov, .cssnanorc*, .env.*, .envrc, .htmlnanorc*, .lighthouserc.*, .mocha*, .postcssrc*, .terserrc*, api-extractor.json, ava.config.*, babel.config.*, contentlayer.config.*, cssnano.config.*, cypress.*, env.d.ts, formkit.config.*, formulate.config.*, histoire.config.*, htmlnanorc.*, jasmine.*, jest.config.*, jsconfig.*, karma*, lighthouserc.*, playwright.config.*, postcss.config.*, puppeteer.config.*, svgo.config.*, tailwind.config.*, tsconfig.*, tsdoc.*, unocss.config.*, vitest.config.*, webpack.config.*, windi.config.*"
},
// 控制终端在其缓冲区中保留的最大行数。
"terminal.integrated.scrollback": 1000,
// 本地 prettier 配置
"prettier.printWidth": 120,
"prettier.tabWidth": 2,
"prettier.semi": false,
"prettier.singleQuote": true,
"prettier.singleAttributePerLine": true,
"prettier.useTabs": false,
"prettier.quoteProps": "as-needed",
"prettier.jsxSingleQuote": false,
"prettier.trailingComma": "none",
"prettier.bracketSpacing": true,
"prettier.arrowParens": "always",
"prettier.requirePragma": false,
"prettier.insertPragma": false,
"prettier.proseWrap": "preserve",
"prettier.htmlWhitespaceSensitivity": "ignore",
"prettier.vueIndentScriptAndStyle": false,
"prettier.endOfLine": "auto",
"prettier.embeddedLanguageFormatting": "auto",
"vite.autoStart": false,
"path-intellisense.mappings": {
"@/": "${workspaceFolder}/src"
}
}
重启项目,完工!
更多推荐
所有评论(0)