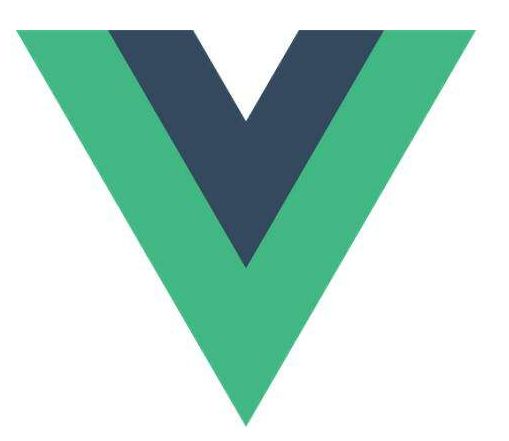
Vue 03-Axios的使用详解
Axios的基本使用
·
基本概念
1.什么是Axios?
Axios 是一个基于 promise 网络请求库,作用于 node.js 和浏览器中。它是 isomorphic 的(即同一套代码可以运行在浏览器和 node.js 中)。
2.Axios的特性(摘自官网)
- 从浏览器创建 XMLHttpRequests
- 从 node.js 创建 http 请求
- 支持 Promise API
- 拦截请求和响应
- 转换请求和响应数据
- 取消请求
- 自动转换 JSON 数据
- 客户端支持防御 XSRF
如何使用Axios
1. 安装Axios
npm install axios -g
2.在前端导入Axios
<script src="https://unpkg.com/axios/dist/axios.min.js"></script>
导入Vue
import Vue from 'vue'
import axios from 'axios'
import VueAxios from 'vue-axios'
Vue.use(VueAxios, axios)
3.执行代码
发送一个post请求
const axios = require("axios");
axios
.get("/user?ID=12345")
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
})
.then(function () {
});
axios
.get("/user", {
params: {
ID: 12345,
},
})
.then(function (response) {
console.log(response);
})
.catch(function (error) {
console.log(error);
})
.then(function () {
});
async function getUser() {
try {
const response = await axios.get("/user?ID=12345");
console.log(response);
} catch (error) {
console.error(error);
}
}
4. 练习:使用Axios制作一个天气页面
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Title</title>
<link rel="stylesheet" href="index.css">
<style>
.el-header, .el-footer {
background-color: #B3C0D1;
color: #333;
text-align: center;
line-height: 60px;
}
.el-aside {
background-color: #D3DCE6;
color: #333;
text-align: center;
line-height: 200px;
}
.el-main {
background-color: #E9EEF3;
color: #333;
text-align: center;
line-height: 160px;
}
body > .el-container {
margin-bottom: 40px;
}
.el-container:nth-child(5) .el-aside,
.el-container:nth-child(6) .el-aside {
line-height: 260px;
}
.el-container:nth-child(7) .el-aside {
line-height: 320px;
}
img{
width: 100px;
height: 100px;
}
</style>
</head>
<body>
<!--v-cloak-->
<el-container id="root">
<el-header>
<el-row type="flex" justify="center">
<el-col :span="8">
<el-form :inline="true">
<el-form-item
label="城市名称">
<el-input @keydown.enter.native="searchWeather" style="margin-top: 8px;" lable-width="80px" v-model="city" placeholder="请输入城市名称"></el-input>
</el-form-item>
<el-form-item>
<el-button style="margin-top: 10px;" @click="searchWeather" type="primary" round>查询</el-button>
</el-form-item>
</el-form>
</el-col>
</el-row>
<el-row type="flex" justify="center">
<el-col :span="24" style="color: red;font-size: 20px;font-weight: 400;">
<p>{{cityWeather.ganmao}}</p>
</el-col>
</el-row>
</el-header>
<el-main style="height: 580px" >
<el-row :gutter="10">
<el-col :span="4">
<div v-if="cityWeather.yesterday != undefined" >
<h3 align="center" v-text="'日期:'+cityWeather.yesterday.date"></h3>
<p align="center" style="height: 20px;">
<img v-if="cityWeather.yesterday.type=='晴'" src="./images/1.png"/>
<img v-else-if="cityWeather.yesterday.type=='阴'" src="./images/2.png"/>
<img v-else-if="cityWeather.yesterday.type=='多云'" src="./images/3.png"/>
<img v-else-if="cityWeather.yesterday.type=='小雨'" src="./images/4.png"/>
</p>
<p align="center" style="height: 20px;">
{{cityWeather.yesterday.type}}
</p>
<p align="center" style="height: 20px;">
高温: {{cityWeather.yesterday.high}}
</p>
<p align="center" style="height: 20px;">
低温:{{cityWeather.yesterday.low}}
</p>
</div>
</el-col>
<el-col :span="4" v-if="cityWeather.forecast != undefined"
v-for="(weather,index) in cityWeather.forecast" :key="index">
<div >
<h3 align="center" v-text="'日期:'+weather.date"></h3>
<p align="center" style="height: 20px;">
<img v-if="weather.type=='晴'" src="./images/1.png"/>
<img v-else-if="weather.type=='阴'" src="./images/2.png"/>
<img v-else-if="weather.type=='多云'" src="./images/3.png"/>
<img v-else-if="weather.type=='小雨'" src="./images/4.png"/>
</p>
<p align="center" style="height: 20px;">
{{weather.type}}
</p>
<p align="center" style="height: 20px;">
高温: {{weather.high}}
</p>
<p align="center" style="height: 20px;">
低温:{{weather.low}}
</p>
</div>
</el-col>
</el-row>
</el-main>
</el-container>
<script src="vue.js"></script>
<script src="axios.min.js"></script>
<!-- 引入组件库 -->
<script src="index.js"></script>
<script>
new Vue({
el: '#root',
data() {
return {
city: '西安',
cityWeather: {}
}
}, methods: {
searchWeather() {
axios.get('http://wthrcdn.etouch.cn/weather_mini?city=' + this.city)
.then((response) => {
this.cityWeather = response.data.data;
console.log(this.cityWeather)
});
}
}
});
</script>
</body>
</html>
Axios如何实现跨域请求
School的相关代码
<template>
<div>
<h2>学校名称:{{ name }}</h2>
<h2>学校地址:{{ address }}</h2>
<button @click="getBookId">点击发起axios请求</button>
</div>
</template>
<script>
import pubSub from 'pubsub-js';
import axios from "axios";
export default {
name: "School",
data() {
return {
name: '猿究院',
address: '太白南路',
pubId: ''
}
}, methods: {
myShowInfo(msg, data) {
console.log("这是School组件,收到了数据:" + msg + data);
},
getBookId() {
axios.get('http://localhost:8080/book/books/selectBooksId/4946')
.then(
response => {
console.log(response);
},
error => {
console.log(error);
}
)
}
},
mounted() {
// 订阅消息
this.pubId = pubSub.subscribe('myShowInfo', this.myShowInfo);
},
beforeDestroy() {
// 取消订阅的消息 this.myShowInfo
pubSub.unsubscribe(this.pubId);
}
}
</script>
<style scoped>
</style>
Student的相关代码
<template>
<div>
<h2>学生姓名:{{ name }} </h2>
<h2>学生性别:{{ sex }} </h2>
<button @click="sendStudentName">点我传递学生信息</button>
</div>
</template>
<script>
import pubSub from 'pubsub-js'
export default {
name: "Student",
data() {
return {
name: '张三',
sex: '男'
}
},methods:{
sendStudentName(){
pubSub.publish('myShowInfo',this.name);
}
}
}
</script>
<style scoped>
</style>
App的代码
<template>
<div>
<!-- 通过父组件 给子组件绑定一个自定事件 实现子组件给父组件 -->
<!-- ****** 传递数据 -->
<School></School>
<Student ></Student>
</div>
</template>
<script>
import Student from "@/components/Student";
import School from "@/components/School";
export default {
name: 'App',
data() {
return {}
}, components: {
School,
Student
}
}
</script>
更多推荐
所有评论(0)