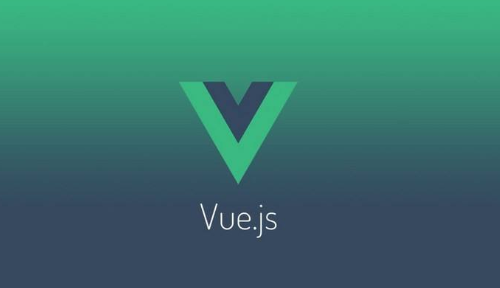
Vue路由
路由的使用方法
·
注意事项
- 路由组件通常放在pages文件夹,一般组件通常存放在components文件夹。
- 通过切换,‘隐藏’了的路由组件,,默认是被销毁掉的,需要的时候再去挂载。
- 每个组件都有自己的$route属性,里面存储着自己的路由信息。
- 整个应用只有一个router,可以通过组件的$router属性获取到。
基本使用
- Vue2项目执行:npm install vue-router@3.5.2
- 导入插件:import VueRouter from 'vue-router'
- 应用插件:Vue.use(VueRouter)
- 编写router配置项,一般都是在src目录下创建router文件夹中编写router配置文件index.js,编写配置项:
import VueRouter from 'vue-router' import About from '../components/About' import Home from '../components/Home' //创建并暴露一个路由器 export default new VueRouter({ routes:[ { path:"/about", component:About }, { path:"/home", component:Home } ] })
-
使用<router-link to="/about">About</router-link>去代替<a href='about'>About</a>
-
实现切换(active-class可配置高亮样式)
<!-- active-class当点击时选中的样式,默认选中第一个 --> <router-link class="list-group-item" active-class="active" to="/about">About</router-link>
-
指定显示的位置
<!-- 指定路由呈现位置 --> <router-view></router-view>
例子
main.js
import Vue from "vue"
import App from "./App"
import VueRouter from 'vue-router'
import router from "./router/index"
Vue.config.productionTip = false;
Vue.use(VueRouter)
new Vue({
el: "#app",
render: h => h(App),
router:router
})
router/index.js
import VueRouter from 'vue-router'
import About from '../components/About'
import Home from '../components/Home'
//创建并暴露一个路由器
export default new VueRouter({
routes:[
{
path:"/about",
component:About
},
{
path:"/home",
component:Home
}
]
})
App.vue
<template>
<div>
<div>
<div class="row">
<div class="col-xs-offset-2 col-xs-8">
<div class="page-header"><h2>Vue Router Demo</h2></div>
</div>
</div>
<div class="row">
<div class="col-xs-2 col-xs-offset-2">
<div class="list-group">
<!-- router-lick实现路由的跳转 -->
<!-- active-class当点击时选中的样式,默认选中第一个 -->
<router-link class="list-group-item" active-class="active" to="/about">About</router-link>
<router-link class="list-group-item" active-class="active" to="/home">Home</router-link>
</div>
</div>
<div class="col-xs-6">
<div class="panel">
<div class="panel-body">
<!-- 指定路由呈现位置 -->
<router-view></router-view>
</div>
</div>
</div>
</div>
</div>
</div>
</template>
<script>
import About from "./components/About"
import Home from "./components/Home"
export default {
name: "App",
components: {
About,
Home
},
};
</script>
<style>
</style>
About.vue与Home.vue
<template>
<h2>我是About的内容</h2>
</template>
<script>
export default {
name:"About"
}
</script>
<style>
</style>
--------------------------------------------------------------------------------
<template>
<h2>我是Home的内容</h2>
</template>
<script>
export default {
name:"Home"
}
</script>
<style>
</style>
多级路由
- 配置路由规则,使用children配置项:
export default new VueRouter({ routes:[ { path:"/about", component:About }, { path:"/home", component:Home, //通过children配置子路由 children:[ { //此处一定不要写:/news path:"news", component:News }, { path:"message", component:Message } ] } ] })
-
调转(要写完整路径)
<router-link to='/home/news'>News</router-link>
跳转路由并携带参数
下面的代码通过query来传递参数
query传值
通过url的方式传值
<!-- 通过url加参数的方式传递参数 -->
<router-link :to="`/home/message/detail?id=${m.id}&title=${m.title}`">{{ m.title }}</router-link>
<!-- 通过query的方式获取参数 -->
<template>
<ul>
<li>消息编号:{{$route.query.id}}</li>
<li>消息标题:{{$route.query.title}}</li>
</ul>
</template>
通过对象的方式传值
<!-- 通过对象的方式传递参数 -->
<router-link
:to="{
path: '/home/message/detail',
query: {
id: m.id,
title: m.title,
},
}">
{{ m.title }}
</router-link>
<!-- 通过query的方式获取参数 -->
<template>
<ul>
<li>消息编号:{{$route.query.id}}</li>
<li>消息标题:{{$route.query.title}}</li>
</ul>
</template>
命名路由
children:[
{
name:"xin", //给路由起名字
path:"detail",
component:Detail
}
]
<router-link
:to="{
//跳转到指定名字的路由
name: 'xin',
query: {
id: m.id,
title: m.title,
},
}">
{{ m.title }}
</router-link>
<router-link
:to="{
//跳转到指定名字的路由
name: 'xin'}">
{{ m.title }}
</router-link>
params传值
注意:路由携带params参数时,若使用to的对象写法,则不能使用path配置项,必须使用name配置。
配置路由接收params参数
routes:[
{
//使用params跳转必须要用name属性进行跳转
name:"hahaha",
//使用占位符接收params参数
path:"detail/:id/:title"
component:About
}
]
//------------------------------------------------------
//跳转并携带params参数,to的字符串写法
<router-link :to='/home/message/detail/666/标题'>跳转</router-link>
//跳转并携带params参数,to的对象写法
<router-link :to='{
name:"hahaha",
params:{
id:666,
title:"你好"
}
}'>跳转</router-link>
//接收参数
<h1>{{$route.params.id}}</h1>
路由的props配置
作用:让路由组件更方便的收到参数
{
name:"xiangqing",
path:"detail:id",
component:Detail
//第一种写法:props值为对象,该对象中所有的key-value的组合最终都会通过props传给Detail组件
//props:{a:900}
//第二种写法:props值为布尔值,布尔值为true,则把路由收到的所有params参数通过props传给Detail组件
//props:true
//第三种写法:props值为函数,该函数返回的对象中每一组key-value都会通过props传给Detail组件
//为回调函数,参数为路由的$route
props(route){
return {
id:route.query.id,
title.route.query.title
}
}
}
<router-link>的replace属性
- 作用:控制路由跳转时操作浏览器历史记录的模式。
- 浏览器的历史记录有两种写入方式,分别是push和replace,push时追加历史记录,replace是替换当前记录,路由跳转时候默认为push。
- 开启replace模式。
<router-link replace :to="{path: '/home/message/detail',query: {id: m.id,title: m.title,},}">{{ m.title }}</router-link>
编程式路由导航
编程式路由导航:不借助<router-link>的路由导航,让路由更加灵活
可以给按钮点击事件中添加下面五种代码。
//以push的方式跳转
pushShow(m){
this.$router.push({
name:"xiangqing",
query:{
id:m.id,
title:m.title
}
})
}
//以replace的方式跳转
replacShow(m){
this.$router.replace({
name:"xiangqing",
query:{
id:m.id,
title:m.title
}}
)
//后退一步
back(){
this.$router.back();
}
//前进一步
forward(){
this.$router.forward();
}
testGo(){
//如果为正数向前前进n步,如果为负数向后后退n步
this.$router.go(1);
}
路由缓存
路由切换的时候会销毁当前的路由去创建显示新的路由,如果被销毁的路由中有输入框中有信息,信息也会被销毁。
keep-alive标签可以解决这种问题,默认是缓存所有的显示路由,可以通过include属性去设置对指定的组件进行缓存。
<keep-alive>作用:让不展示的路由组件保持挂载,不被销毁。
<keep-alive>
<router-view></router-view>
</keep-alive>
<!-- 对News组件内容进行缓存,其它的组件都不缓存 -->
<keep-alive include="News">
<router-view></router-view>
</keep-alive>
<!-- 对News组件内容进行缓存,其它的组件都不缓存,配置多个 -->
<keep-alive :include="['News','Mwssage']">
<router-view></router-view>
</keep-alive>
activated与deactivated
路由组件所独有的两个生命钩子,用于捕获路由组件的激活状态,与moundted同级
当组件获取焦点时会自动调用:activated()
当组件失去焦点时会自动调用:deactivated()
路由守卫
作用:对路由进行权限控制
执行顺序:前置路由,后置路由,路由组件
前置路由守卫
const router=new VueRouter({
routes: [
{
path: "/about",
component: About
}
]
})
//全局前置路由守卫,初始化或者路由之前跳转时会触发
//to:目标路由的信息,name属性为路由名
//from:来源路由的信息,name属性为路由名
//next:是否放行的函数,执行就放行
router.beforeEach((to,from,next)=>{
next(); //执行next函数放行
})
meta属性
const router=new VueRouter({
routes: [
{
path: "/about",
component: About,
//meta用于配置自定义属性
meta:{isAuth:true}
}
]
})
//使用时meta.isAuth
后置路由守卫
//创建并暴露一个路由器
const router = new VueRouter({
routes: [
{
path: "/about",
component: About,
meta: { title: "about" },
}
]
})
//全局后置路由守卫,before执行后执行
router.afterEach((to, from) => {
//跳转之后改变网页标题
document.title = to.meta.title || '主页';
})
独享路由守卫
某一个路由独享的守卫,用法与前置路由守卫一样。
//创建并暴露一个路由器
const router = new VueRouter({
routes: [
{
path: "/about",
component: About,
meta: { title: "about" },
beforeEnter(to,from,next){
next(); //执行next放行
}
},
]
})
组件内守卫
写在组件配置项中与mounted同级。
用法与全局路由一致、
//通过路由规则进入时调用
beforeRouteEnter(to,from,next){
}
//通过路由规则离开时调用
beforeRouteleave(to,from,next){
}
路由器的两种工作模式
更多推荐
所有评论(0)