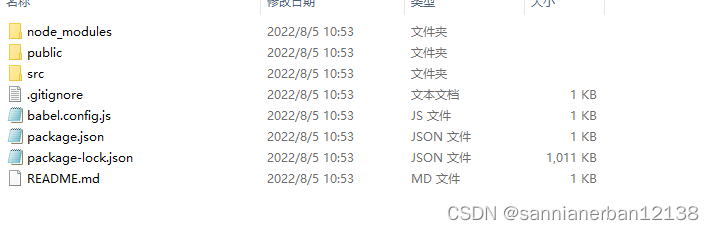
Cesium+Vue项目环境搭建(不需要改动任何配置)
最简单的Cesium+Vue项目搭建方式
·
提示:文章写完后,目录可以自动生成,如何生成可参考右边的帮助文档
一、项目环境准备
- 首先你需要有一个Vue2.x的项目,你可以通过Vue/Cli提供的图形化界面很快的创建一个Vue2.x的项目出来.
项目目录如图
- 为当前项目添加Cesium,在当前项目的命令行输入npm i cesium -S,安装完成后,从当前目录进入node_modules\cesium\Build\Cesium
将当前文件夹内所有文件拷贝到项目的public文件中
最后public文件夹如图
到这里项目环境就算是准备完成了.
二、完善项目,成功运行Cesium
1.在index.html引入Cesium
代码如下:
<!DOCTYPE html>
<html lang="">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<link rel="icon" href="<%= BASE_URL %>favicon.ico">
<title><%= htmlWebpackPlugin.options.title %></title>
<!--在这里引入Cesium和css文件-->
<script src="./Cesium.js"></script>
<link href="./Widgets/widgets.css" rel="stylesheet">
</head>
<body>
<noscript>
<strong>We're sorry but <%= htmlWebpackPlugin.options.title %> doesn't work properly without JavaScript enabled. Please enable it to continue.</strong>
</noscript>
<div id="app"></div>
<!-- built files will be auto injected -->
</body>
</html>
2.创建一个类用于存储Cesium对象
当我们在index.html中引入了Cesium,js后,默认Cesium对象就挂载到了Window对象上面,但是在后续开发中如果你在代码中直接使用Cesium,代码编辑器不容易识别出来,推荐创建一个静态类,将Cesium对象挂载到这个类上面.同时这个类还包括初始化场景的函数.
在src文件夹中创建一个js文件,文件名随意,我这里叫CesiumByZh.js
代码如下:
export default class CesiumByZh {
static Cesium;
static viewer;
static cesiumDefaultStartTime = new Date(2022, 2, 28, 12, 0, 0).getTime();
static cesiumDefaultEndTime = new Date(2022, 2, 28, 12, 0, 0).getTime() + 86400000;
constructor() {
}
static init(id, funhander) {
try {
// @ts-ignore
if (window.Cesium){
// @ts-ignore
CesiumByZh.Cesium=window.Cesium;
// @ts-ignore
CesiumByZh.viewer = new CesiumByZh.Cesium.Viewer(id, {
//天地图(这里是我自己申请的天地图Key,想用天地图的可以自己申请,很简单免费的)
imageryProvider: new CesiumByZh.Cesium.WebMapTileServiceImageryProvider({
url: 'http://t0.tianditu.gov.cn/img_w/wmts?tk=30cc127d81b6563a4f9eb761a01aa5a6',
layer: 'img',
style: 'default',
tileMatrixSetID: 'w',
format: 'tiles',
maximumLevel: 19
}),
});
//开启深度地形测试
CesiumByZh.viewer.scene.globe.depthTestAgainstTerrain = true;
CesiumByZh.Cesium.Ion.defaultAccessToken = 'eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJqdGkiOiI2MzY5NWI4YS02Y2IwLTRhMGYtOGE3MS03NGY5N2Q3NDJjODUiLCJpZCI6MzY0MjYsImlhdCI6MTYwMzQ0MDM1Nn0.oIxHAr3gEFCqTPO7BepTyoPQN77pXH3zRUqY4X7IpQM';
//是否显示大气层
CesiumByZh.viewer.scene.globe.showGroundAtmosphere = false;
//是否开启实时光照
CesiumByZh.viewer.scene.globe.enableLighting = false;
//是否显示帧数
CesiumByZh.viewer.scene.debugShowFramesPerSecond = true;
//修改home位置
CesiumByZh.Cesium.Camera.DEFAULT_VIEW_RECTANGLE = CesiumByZh.Cesium.Rectangle.fromDegrees(103, 30, 104, 29);
//屏蔽双击实体摄像头拉近
CesiumByZh.viewer.cesiumWidget.screenSpaceEventHandler.removeInputAction(CesiumByZh.Cesium.ScreenSpaceEventType.LEFT_DOUBLE_CLICK);
//屏蔽ctrl加鼠标左键旋转视图的操作器
CesiumByZh.viewer.scene.screenSpaceCameraController.tiltEventTypes = [CesiumByZh.Cesium.CameraEventType.MIDDLE_DRAG, CesiumByZh.Cesium.CameraEventType.PINCH];
if (funhander != null) {
// @ts-ignore
funhander();
}
//去除因为光照导致模型颜色变暗
CesiumByZh.viewer.scene.light = new CesiumByZh.Cesium.DirectionalLight({
direction: new CesiumByZh.Cesium.Cartesian3(0.354925, -0.890918, -0.283358)
});
}
}catch (e) {
}
}
}
3.创建Cesium的视图容器,并初始化视图
- 创建一个组件,用于当做Cesium视图的容器,并且将这个组件添加到视图中,我这里创建的叫cesiumViewer.vue,
代码如下:
<template>
<div id="cesiumContainer">
</div>
</template>
<script>
import CesiumByZh from "../CesiumByZh";
export default {
name: 'cesiumViewer',
props: {
msg: String
},
mounted() {
//三维场景初始化
CesiumByZh.init('cesiumContainer', function () {
//这里可以写初始化完成后想要执行的操作
});
}
}
</script>
<!-- Add "scoped" attribute to limit CSS to this component only -->
<style >
html, body, #cesiumContainer {
width: 100%;
height: 100%;
margin: 0;
padding: 0;
overflow: hidden;
position: absolute;
}
</style>
2.我把当前页面放在与HelloWord.vue同一目录下,参照引入HelloWorld.vue的方式,在Home.vue页面引入cesiumViewer.vue
<template>
<div class="home">
<!--<HelloWorld msg="Welcome to Your Vue.js App"/>-->
<cesiumViewer/>
</div>
</template>
<script>
// @ is an alias to /src
import HelloWorld from '@/components/HelloWorld.vue'
import cesiumViewer from '@/components/cesiumViewer.vue'
export default {
name: 'Home',
components: {
HelloWorld,
cesiumViewer
}
}
</script>
3.将App.vue里面的原本的一些内容删除,运行项目,查看结果,大功告成
最终效果图
总结
这种引入模式避免了Cesium参与webpack的打包过程,可以避免很多因为webpack配置没对导致的问题
在后续开发中,有需要使用Cesium对象的,都可以直接从CesiumByZh这个类上面去获取哦
更多推荐
所有评论(0)