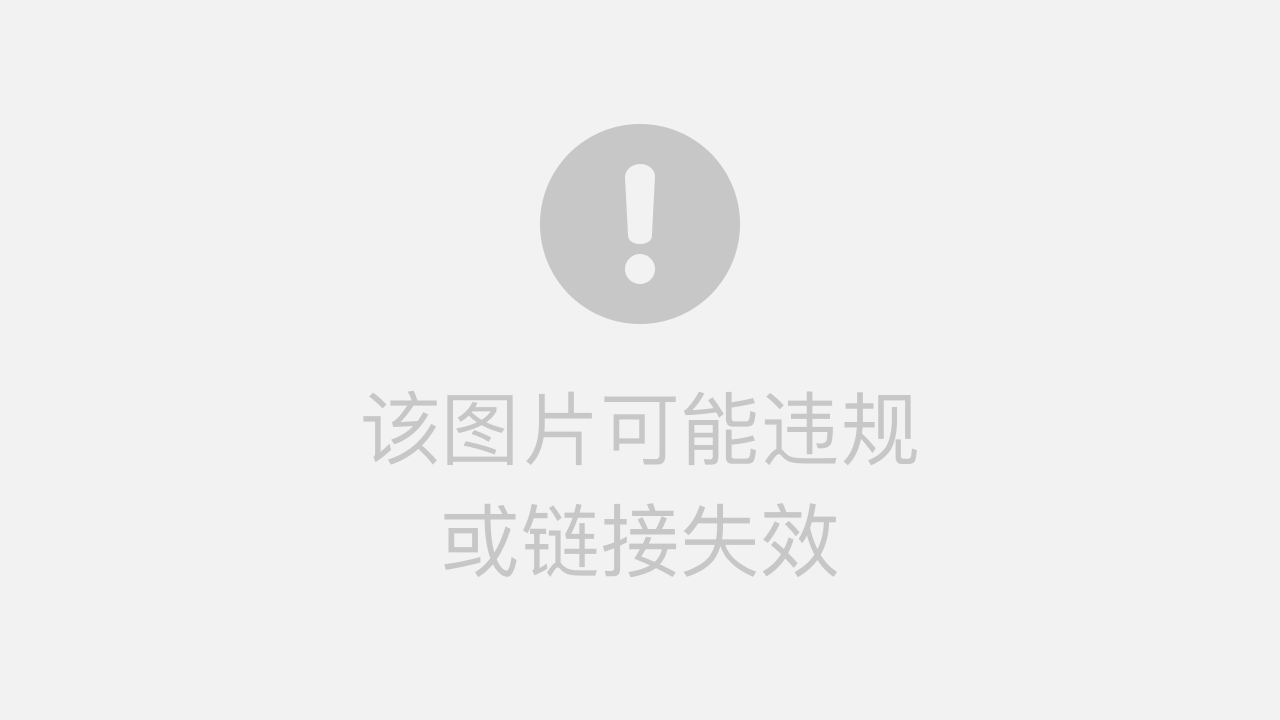
【vue】vue + ECharts 实现中国地图
vue + ECharts 实现中国地图
·
1.效果图:
2.实现步骤
- 引入ECharts
ECharts 官网地址:https://echarts.apache.org/zh/index.html
npm install echarts --save
- 下载中国地图及各省份地图的json数据
下载地址:https://datav.aliyun.com/portal/school/atlas/area_selector
- 重命名地图json文件名字并复制到public ----> static---->map文件夹下
- 创建省份名字转拼音的工具类map-utils.js
// 省份对应的拼音
const nameToPinyin = {
安徽省: 'ah',
澳门特别行政区: 'am',
北京市: 'bj',
福建省: 'fj',
甘肃省: 'gs',
广东省: 'gd',
广西壮族自治区: 'gx',
贵州省: 'gz',
海南省: 'hn',
河北省: 'hb',
河南省: 'hn1',
黑龙江省: 'hlj',
湖北省: 'hb1',
湖南省: 'hn2',
吉林省: 'jl',
江苏省: 'js',
江西省: 'jx',
辽宁省: 'ln',
内蒙古自治区: 'nmg',
宁夏回族自治区: 'nx',
青海省: 'qh',
山东省: 'sd',
山西省: 'sx',
陕西省: 'sx1',
上海市: 'sh',
四川省: 'sc',
台湾省: 'tw',
天津市: 'tj',
西藏自治区: 'xz',
香港特别行政区: 'xg',
新疆维吾尔自治区: 'xj',
云南省: 'yn',
浙江省: 'zj',
重庆市: 'cq',
}
// 获取省份名称及地图矢量数据的地址
export function getProvinceMapInfo(arg){
// static/map/province 为各省份json数据
const path = `/static/map/province/${nameToPinyin[arg]}.json`
return{
key: nameToPinyin[arg],
path: path
}
}
- 下载echarts暗色主题
下载地址:https://echarts.apache.org/zh/theme-builder.html
- 创建vue文件,并使用ECharts
<template>
<!-- 具备一个宽高的dom -->
<div ref="main" style="height: 879px ;width: 100%;margin: 0;padding: 0;overflow: hidden" @dblclick="revertMap"></div>
</template>
<script>
// 导入echarts
import * as echarts from 'echarts';
// 中国地图
import chianJson from '@/map/china.json'
// 导入echarts主题
require('@/theme/chalk')
// axios
import axios from 'axios'
// 各省份对应的矢量数据地址
import {getProvinceMapInfo} from '@/utils/map-utils'
export default {
name: "china-map",
data() {
return {
chart: ''
}
},
mounted() {
this.$nextTick(() => {
this.initChart()
window.addEventListener('resize', this.screenAdapter)
this.screenAdapter()
})
},
methods: {
initChart() {
// init第一个参数 ref定义的的,第二个参数主题
this.chart = echarts.init(this.$refs.main, 'chalk')
echarts.registerMap("chain", chianJson)
this.chart.setOption({
title: {
text: '中国地图',
left: 20,
top: 20,
},
geo: {
type: 'map', // 类型
map: 'chain',
top: '5%', // 距离顶部
bottom: '5%', // 距离底部
itemStyle: { // 地图区域的样式。
areaColor: '#2E72BF', // 地图整体区域的颜色
borderColor: '#333', //地图边界线的颜色
},
},
// 自定义提示框的内容
tooltip: {
trigger: 'item',
// 自定义提示框的内容
formatter(params) {
return (
"<div>" +
params.data.name +
" <br> " +
params.seriesName +
"</div>"
);
}
},
// 散点图
series: [
{
type: 'effectScatter', //特效散点图
rippleEffect: { // 涟漪效果
scale: 5,
brushType: 'stroke'
},
coordinateSystem: 'geo', // 使用坐标
name: "一线城市",
data: [
{name: "北京", value: [116.46, 39.92]},
{name: "上海", value: [121.48, 31.22]},
{name: "广州", value: [113.23, 23.16]},
{name: "深圳", value: [114.07, 22.62]},
]
},
{
type: 'effectScatter', //特效散点图
rippleEffect: { // 涟漪效果
scale: 5,
brushType: 'stroke'
},
coordinateSystem: 'geo', // 使用坐标
name: "二线城市",
data: [
{name: "南京", value: [118.78, 32.04]},
{name: "杭州", value: [120.19, 30.26]},
{name: "成都", value: [104.06, 30.67]},
{name: "武汉", value: [114.31, 30.52]},
]
},
{
type: 'effectScatter', //特效散点图
rippleEffect: { // 涟漪效果
scale: 5,
brushType: 'stroke'
},
coordinateSystem: 'geo', // 使用坐标
name: "三线城市",
data: [
{name: "拉萨", value: [91.11, 29.97]},
{name: "乌鲁木齐", value: [87.68, 43.77]},
{name: "西宁", value: [101.74, 36.56]},
{name: "兰州", value: [103.73, 36.03]},
]
},
],
legend: { // 图例组件
left: '5%',
bottom: '5%',
orient: 'vertical', // 竖着排列
data: ['一线城市', '二线城市', '三线城市']
},
})
this.chart.on('click', async arg =>{
const provinceInfo = getProvinceMapInfo(arg.name)
console.log("provinceInfo",provinceInfo)
// 如果存在key执行下面内容
if(provinceInfo.key !== undefined){
// 获取static下面的json数据
const res = await axios.get("http://localhost:9528" + provinceInfo.path)
echarts.registerMap(provinceInfo.key, res.data)
const changeOption={
geo:{
map: provinceInfo.key
}
}
this.chart.setOption(changeOption)
}
})
},
// 当浏览器的大小发生变化的时候,会调用此方法来完成适配
screenAdapter() {
// 获取自适应大小
const titleFontSize = this.$refs.main.offsetWidth / 100 * 3.6
console.log("titleFontSize", titleFontSize)
// 自适应配置
const adapterOption = {
title: {
textStyle: {
fontSize: titleFontSize // 自适应字体大小
}
},
legend: { // 图例组件
itemWidth: titleFontSize / 2,
itemHeight: titleFontSize / 2,
itemGap: titleFontSize / 2,
textStyle: {
fontSize: titleFontSize / 2 // 自适应字体大小
}
},
}
this.chart.setOption(adapterOption)
// 自适应
this.chart.resize()
},
// 回退
revertMap(){
const revertOption={
geo:{
map: 'chain'
}
}
this.chart.setOption(revertOption)
},
},
}
</script>
<style lang="scss" scoped>
.dashboard-container{
height: 100%;
width: 100%;
}
</style>
更多推荐
所有评论(0)