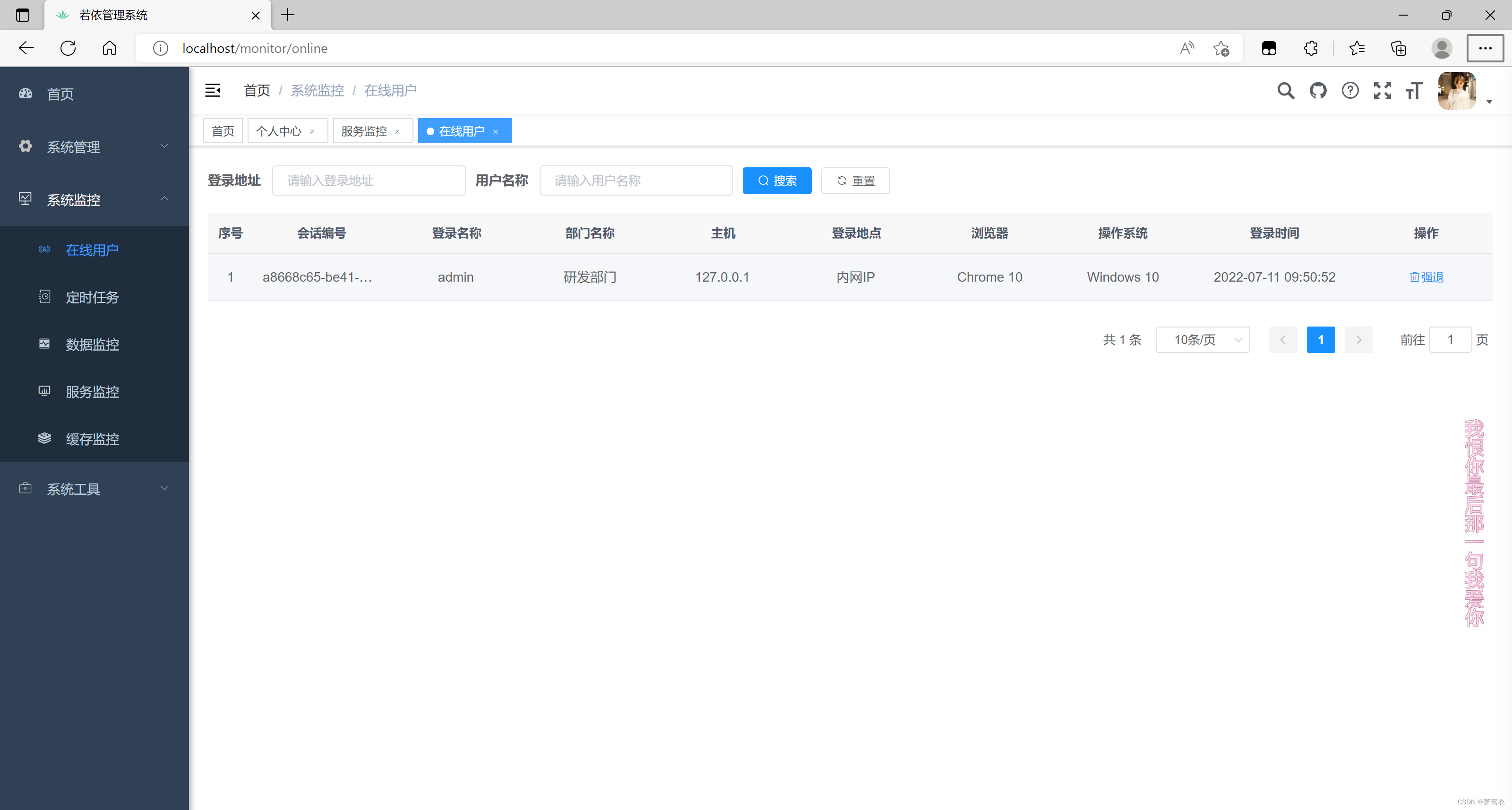
【若依】框架:第01讲前后端分离项目
本身是一个优秀的简单的后台管理系统框架,前端(Vue,ElementUI),后端(Spring Boot、Spring Security、Redis & Jwt),本身可以直接拉下来直接使用.它的强大之处在于代码自动生成器的使用,可以根据数据库的表对应生成全套前后端代码,代码植入后可以直接使用,复杂业务只需在基础代码上进行修改增强即可,减少了重复代码的编写,提高了开发效率。...
·
一、准备工作
1.IDEA准备
将下载好的若依项目导入IDEA,导入后配置MAVEN环境,等待下载完成。重点关注ruoyi-admin和ruoyi-system两个文件夹,前者放controller,后者放实体类、mapper、service
2.打开VS Code前端编写
①打开文件夹选择若依前端项目(ruoyi-ui)文件夹
②信任此作者进入
③重点关心src文件夹下的api和views两个文件夹
3.右键以管理员身份启动redis-server服务
上图表示成功启动
4.IDEA启动
5.VS Code启动
①新建终端
②下方黑窗口输入口令,只要没有error就算启动成功,报错后可尝试重启电脑,理论上环境变量自动生成
npm install --registry=https://registry.npmmirror.com
③启动服务
npm run dev
自动弹出若依管理系统表示项目已经启动成功。
二、后台代码编写
1.在ruoyi-system中创建自己的包basic
①编写实体类、mapper以及service层代码
实体类
public class Channel extends BaseEntity {
private String id; //32位主键ID
private String channelName; //栏目名
private Integer isShow; //是否显示
//private Date updateTime; //更新时间,父类中有子类删掉即可
//手动生成getter和setter
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getChannelName() {
return channelName;
}
public void setChannelName(String channelName) {
this.channelName = channelName;
}
public Integer getIsShow() {
return isShow;
}
public void setIsShow(Integer isShow) {
this.isShow = isShow;
}
}
mapper
public interface ChannelMapper {
/**
* 添加栏目
* @param channel
* @return
*/
public int insertChannel(Channel channel);
/**
* 根据ID删除栏目
* @param id
* @return
*/
public int deleteChannelById(String id);
/**
* 修改栏目
* @param channel
* @return
*/
public int updateChannel(Channel channel);
/**
* 根据ID查询栏目
* @param id
* @return
*/
public Channel selectChannelById(String id);
/**
* 根据条件,查询栏目列表
* @param channel
* @return
*/
public List<Channel> selectChannelList(Channel channel);
}
mapper.xml(basic和system不能同级可以选择打开文件根目录,在mapper文件夹中新建basic文件夹,回到项目刷新即可)
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.ruoyi.basic.mapper.ChannelMapper">
<resultMap id="ChannelResult" type="Channel">
<result property="id" column="id"></result>
<result property="channelName" column="channel_name"></result>
<result property="isShow" column="is_show"></result>
<result property="updateTime" column="update_time"></result>
</resultMap>
<sql id="selectChannelVO">
select id,channel_name,is_show,update_time from cms_channel
</sql>
<select id="selectChannelById" parameterType="String" resultMap="ChannelResult">
<include refid="selectChannelVO"></include>
where id=#{id}
</select>
<select id="selectChannelList" parameterType="Channel" resultMap="ChannelResult">
<include refid="selectChannelVO"></include>
<where>
<if test="channelName != null and channelName != ''">and channel_name like concat(#{channelName},'%')</if>
<if test="isShow != null">and is_show=#{isShow}</if>
</where>
</select>
<insert id="insertChannel" parameterType="Channel">
insert into
cms_channel (id,channel_name,is_show,update_time)
values
(#{id},#{channelName},#{isShow},#{updateTime})
</insert>
<delete id="deleteChannelById" parameterType="String">
delete from cms_channel where id=#{id}
</delete>
<update id="updateChannel" parameterType="Channel">
update cms_channel
set channel_name=#{channelName},is_show=#{isShow},update_time=#{updateTime}
where id=#{id}
</update>
</mapper>
service层
public interface IChannelService {
/**
* 添加栏目
* @param channel
* @return
*/
public int insertChannel(Channel channel);
/**
* 根据ID删除栏目
* @param id
* @return
*/
public int deleteChannelById(String id);
/**
* 修改栏目
* @param channel
* @return
*/
public int updateChannel(Channel channel);
/**
* 根据ID查询栏目
* @param id
* @return
*/
public Channel selectChannelById(String id);
/**
* 根据条件,查询栏目列表
* @param channel
* @return
*/
public List<Channel> selectChannelList(Channel channel);
}
service层实现类
@Service
public class ChannelServiceImpl implements IChannelService {
@Autowired(required = false)
private ChannelMapper channelMapper;
@Override
public int insertChannel(Channel channel) {
//id不是自增,需要生成32位主键ID
String id = UUID.randomUUID().toString().replace("-","");
channel.setId(id);
return channelMapper.insertChannel(channel);
}
@Override
public int deleteChannelById(String id) {
return channelMapper.deleteChannelById(id);
}
@Override
public int updateChannel(Channel channel) {
return channelMapper.updateChannel(channel);
}
@Override
public Channel selectChannelById(String id) {
return channelMapper.selectChannelById(id);
}
@Override
public List<Channel> selectChannelList(Channel channel) {
return channelMapper.selectChannelList(channel);
}
}
2.在ruoyi-admin中创建自己的包basic
controller
@RestController
@RequestMapping("/basic/channel")
public class ChannelController extends BaseController {
@Autowired
private IChannelService channelService;
@PostMapping("/add")
public AjaxResult add(Channel channel){
int rows = channelService.insertChannel(channel);
return toAjax(rows);//如果rows > 0就是成功,否则失败
}
@DeleteMapping("/remove")
public AjaxResult remove(String id){
int rows = channelService.deleteChannelById(id);
return toAjax(rows);//如果rows > 0就是成功,否则失败
}
@PutMapping("/edit")
public AjaxResult edit(Channel channel){
int rows = channelService.updateChannel(channel);
return toAjax(rows);//如果rows > 0就是成功,否则失败
}
@GetMapping("/get_info")
public AjaxResult grtInfo(String id){
return AjaxResult.success(channelService.selectChannelById(id));
// Channel channel = channelService.selectChannelById(id);
// return AjaxResult.success(channel);
}
@GetMapping("/list")
public TableDataInfo list(Channel channel){
startPage();//分页函数,注意:该函数必须和select语句写在一起
List<Channel> channels = channelService.selectChannelList(channel);
return getDataTable(channels);
}
}
更多推荐
所有评论(0)