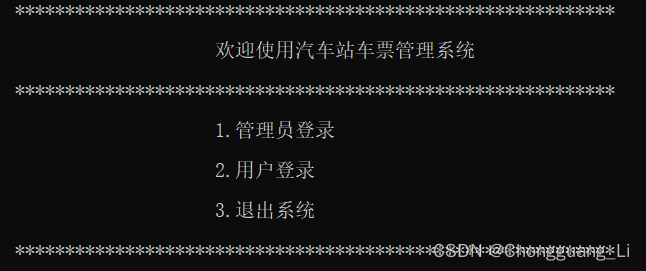
C++课设:汽车站车票管理系统
汽车站管理系统,分为管理员和乘客两部分
·
整个程序分为两个部分,管理员系统和乘客的系统,其中管理员系统中设置了登录界面,同时也使用了*号将密码给隐藏起来
建立了日志模块,当天增删的车次第二天才能奏效。
调用了系统时间,乘客购票时如果在发车前十分钟或者发车后将无法购票。
注意:程序使用时应当先建立bus.txt文档
文档格式如下
程序如下:
头文件
head.h
#pragma once
// * 这个调试的时候用的之后可以删除掉
#include<fstream>
#include<iostream>
#include<fstream>
#include<iomanip>
#include<stdlib.h>
#include<string.h>
#include<conio.h>
#include <windows.h>
#include <ctime>
#define LEN sizeof(Timetable)
#pragma warning (disable:4996)// * 这个不要抄,是我编译器的问题
using namespace std;
function.h
#pragma once
// * 定义结构体
typedef struct
{
char no[10] = {'\0'};// * 班次
int hour;// * 发车时间*小时
int minute;// * 发车时间 * 分钟
char Starting_station[10] = {'\0'};// * 始发站
char Last_station[10] = { '\0' };// * 终点站
float time;// * 行车时长
int fare;// * 票价
int max_number;// * 最大载客量
int sold_number;// * 以售票数
}Timetable;
//==========================================================================================
// * 主函数之间的函数声明
void AdminMode(Timetable* timetables, char* filename);
void PassagerMode(Timetable* timetables, char* filename);
//===============================================================================================
// * 窗口函数的声明
int MainWindowSelect();
int AdminWindowSelect();
int PassagerWindowSelect();
//=================================================================================================================
// * 预处理和结束后处理的函数声明
void Signin();// * 登录
int ReadFromFile(Timetable* timetables, int n, char* filename);// * 从文件中读入
int WritetoFile(Timetable* timetables, int n, char* filename);// * 将数据保存到文件中
void GenerateLogFileName(char* LogFileName);// * 根据当天日期生成日志名
int InitializationPassagerMode(Timetable* timetables,
char* LogFileName,
char* filename);// * 初始化乘客模式,如果有日志,就读日志,如果没有,就读取车次文件
void SortbyTime(Timetable* timetables, int n);// * 将timetable里面的元素按照发车的时间进行排序
int Quit();// * 退出程序
// * 传入相应的车次号,判断是否停止售票和退票
int StopSevice(Timetable* timetables, int n, char* no);
//=====================================================================================================================
// * 管理员的函数
int AddBus(Timetable* timetables, int n);// * 添加车次
void ShowTimeTable(Timetable* timetables, int n);// * 列出所有车次
void Query(Timetable* timetables, int n);// * 查询车次
int DelBus(Timetable* timetables, int n);// * 删除车次
//=======================================================================================
// * 乘客的函数
void TicketOrder(Timetable* timetables, int n);// * 订票
void TicketDelete(Timetable* timetables, int n);// * 退票
// =========================================================================================
// * 辅助的界面设计的函数
void Enter();// * 输入回车控制
void Space();//输入20个空格
void Star();//输入星形
// =============================================================================================
// * 主要功能函数中间的辅助函数
// * 选择查询的方式
int QueryChioce();
// * 输出下标为i的timetables结构体里面的所有值
void OutPutBus(Timetable* timetables, int i);
// * 输入车次号,返回下标
int find(Timetable* timetables, int n, char* no);
// * 按终点站进行查询,返回终点站的数目,数组b[]返回终点站的下标
int find(Timetable* timetables, int n, char* Last_station, int* b);
// * 生成日志文件
void BuildLogFile(char* LogFileName);
// * 在每列前面显示每列信息名字
void BeforeOutPutBus();
// * 输出下标为i的timetables结构体里面的所有值(管理员版本)
void OutPutBus_2(Timetable* timetables, int i);
// * 在每列前面显示每列信息名字(管理员版本)
void BeforeOutPutBus_2();
主函数 main.cpp
#include"head.h"
#include"function.h"
int main()
{
char filename[20];
strcpy(filename, "bus.txt");
int UserChoice;// * 用户的选择
Timetable timetables[100];
while (1)
{
switch (UserChoice = MainWindowSelect())
{
case 1: AdminMode(timetables, filename); break;
case 2: PassagerMode(timetables, filename); break;
case 3: if (Quit() != 1)
continue;
}
if (UserChoice == 3)
break;
}
return 0;
}
void AdminMode(Timetable* timetables, char* filename)// * 这里可能要改
{
Signin();// * 登录,到时候要加回来
int n = ReadFromFile(timetables, 0, filename);
while (1)
{
system("cls");
int AdminChoice = AdminWindowSelect();
switch (AdminChoice)
{
case 1: n = AddBus(timetables, n); // * 添加车次信息
WritetoFile(timetables, n, filename);
system("pause");
break;
case 2: ShowTimeTable(timetables, n); // * 浏览时刻表
system("pause");
break;
case 3: Query(timetables, n); // * 车辆信息查询
system("pause");
break;
case 4: n = DelBus(timetables, n); // * 删除车辆信息
system("pause");
WritetoFile(timetables, n, filename);
break;
case 5: WritetoFile(timetables, n, filename); // * 返回上级菜单
return;
}
}
}
void PassagerMode(Timetable* timetables, char* filename)
{
char LogFileName[200];
GenerateLogFileName(LogFileName);// * 根据当天的日期生成日志名称
int n = InitializationPassagerMode(timetables, LogFileName, filename);
int PassagerChoice;
while (1)
{
system("cls");
switch (PassagerChoice = PassagerWindowSelect())
{
case 1: Query(timetables, n); // * 查找车辆信息
system("pause");
break;
case 2: TicketOrder(timetables, n); // * 购买车票
WritetoFile(timetables, n, LogFileName);
system("pause");
break;
case 3: TicketDelete(timetables, n); // * 退回车票
WritetoFile(timetables, n, LogFileName);
system("pause");
break;
case 4: WritetoFile(timetables, n, LogFileName);
return;
}
}
}
预备功能函数 pre.cpp
#include"head.h"
#include"function.h"
// * 管理员登录
void Signin()
{
int i;// * 用来循环输入密码便于隐藏
int j = 0;
char account[40];
while (1)
{
char password[50] = { '\0' };// * 初始化密码数组
Star();
Space();
Space();
cout << "欢迎使用汽车站车票管理系统\n\n";
Star();
Space();
Space();
cout << "账号:";
cin >> account;
cin.ignore();// * 消化掉换行符
Space();
Space();
cout << "\n密码:";
system("cls");
i = 0;// * 隐藏密码
while (1)
{
Star();
Space();
Space();
cout << "欢迎使用汽车站车票管理系统\n\n";
Star();
Space();
Space();
cout << "账号:";
cout << account << endl;
cout << endl;
Space();
Space();
cout << "密码:";
for (j = 0; j < i; j++)
cout << "*";
password[i] = getch();
// cout << endl << password;// * 这个到时候要删掉的
if (password[i] == '\r')
break;
// * 输入backspace的时侯删除字符串中的字符
if (password[i] == '\b')
{
password[i] = '\0';
i--;
// * 防止减多了
if (i != -1)
i--;
}
system("cls");
i++;
if (i > 20)
{
break;
}
}
// * 比较是否一致
if (!strcmp(account, "admin") && !strcmp(password, "123456\r") )
{
system("cls");
return;
}
cout << "\n\n\n";
Space();
Space();
cout << "输入的账号或密码有误\n";
Space();
Space();
system("pause");
system("cls");
}
}
// * 从文件中读取数据,返回数据的数量
int ReadFromFile(Timetable* timetables, int n, char* filename)
{
fstream file;
file.open(filename, ios::in);
// * 如果找不到文件就建立一个新的文件
if (file.fail())
{
file.close();
file.open(filename, ios::out);
file.close();
file.open(filename, ios::in);
}
while (!file.eof())
{
file >> timetables[n].no;
// * 防止第一行是空的被读取进去
if (file.eof())
{
file.close();
break;
}
file >> timetables[n].hour;
file >> timetables[n].minute;
file >> timetables[n].Starting_station;
file >> timetables[n].Last_station;
file >> timetables[n].time;
file >> timetables[n].fare;
file >> timetables[n].max_number;
file >> timetables[n].sold_number;
n++;
}
return n;
}
// * 将数据保存到文件中
int WritetoFile(Timetable* timetables, int n, char* filename)
{
SortbyTime(timetables, n);
fstream file;
file.open(filename, ios::out);
int i; // * 循环变量
// * 如果n为0的话就不写入文件了
if (n == 0)
{
file.close();
return 0;
}
//====================================================
file << setw(10) << timetables[0].no;
file << setw(10) << timetables[0].hour;
file << setw(10) << timetables[0].minute;
file << setw(10) << timetables[0].Starting_station;
file << setw(10) << timetables[0].Last_station;
file << setw(10) << timetables[0].time;
file << setw(10) << timetables[0].fare;
file << setw(10) << timetables[0].max_number;
file << setw(10) << timetables[0].sold_number;
for (i = 1; i < n; i++)
{
cout.setf(ios::left);
file << endl;
file << setw(10) << timetables[i].no;
file << setw(10) << timetables[i].hour;
file << setw(10) << timetables[i].minute;
file << setw(10) << timetables[i].Starting_station;
file << setw(10) << timetables[i].Last_station;
file << setw(10) << timetables[i].time;
file << setw(10) << timetables[i].fare;
file << setw(10) << timetables[i].max_number;
file << setw(10) << timetables[i].sold_number;
}
// * 分开写入文件是因为如果不分开最后一个endl会往下走一行导致一行空的被读取
// =============================================================
return 0;
}
// * 根据当天日期生成日志名
void GenerateLogFileName(char* LogFileName)
{
time_t t = time(0);
struct tm* time;
time = localtime(&t);
char year[5], month[2], day[3];// * 定义接受年月日字符串的字符串数组
// * 时间是int型,将它转换为char型
itoa(time->tm_mday, day, 10);
itoa(time->tm_year + 1900, year, 10);
itoa(time->tm_mon + 1, month, 10);
strcpy(LogFileName, year);
strcat(LogFileName, "-");
strcat(LogFileName, month);
strcat(LogFileName, "-");
strcat(LogFileName, day);
strcat(LogFileName, ".log");
}
// * 将timetable里面的元素按照发车的时间进行排序
void SortbyTime(Timetable* timetables, int n)
{
// * 采用冒泡排序法
int i, j;
Timetable t;// * 用来交换的量
// * 冒泡排序
for (i = 0; i < n - 1; i++)
{
for (j = 0; j < n - 1 - i; j++)
{
if (timetables[j].hour * 60 + timetables[j].minute > timetables[j + 1].hour * 60 + timetables[j + 1].minute)
{
t = timetables[j + 1];
timetables[j + 1] = timetables[j];
timetables[j] = t;
}
}
}
}
// * 传入相应的车次号,判断是否停止售票和退票
int StopSevice(Timetable* timetables, int n, char* no)
{
struct tm* local;// * 时间结构体
time_t t = time(0);// * 把当前的时间给t
local = localtime(&t);// * 获取当前系统时间
int i = find(timetables, n, no);
if ((local -> tm_hour * 60 + local -> tm_min) + 10 < timetables[i].hour * 60 + timetables[i].minute)
{
return 0;
}
return 1;
}
// ** 辅助函数
//
//
// * 查询车辆时选择是要按车次查询还是终点站查询
int QueryChioce()
{
int choice;
Star();
Space();
Space();
cout << "请选择查询方式\n\n";
Star();
Space();
Space();
cout << "1.按照车次查询\n\n";
Space();
Space();
cout << "2.按照终点站查询\n\n";
Star();
Space();
Space();
cout << "请输入查询方式:";
cin >> choice;
// * 这里没有如果输入了1,2以外的数的处理情况
// * 之后可以选择补加
system("cls");
return choice;
}
// * 输出下标为i的timetables结构体里面的所有值
void BeforeOutPutBus()
{
cout.setf(ios::left);
cout << setw(10) << "车次号";
cout << setw(10) << "发车时间";
cout << " ";
cout << setw(10) << "始发站";
cout << setw(10) << "终点站";
cout << setw(10) << "预计用时";
cout << setw(10) << "票价";
cout << setw(15) << "最大载客量";
cout << setw(10) << "已售票数" << endl;
}
void OutPutBus(Timetable* timetables, int i)
{
cout.setf(ios::left);
cout << setw(10) << timetables[i].no;
cout << timetables[i].hour << ":";
cout << setw(10) << timetables[i].minute;
cout << setw(10) << timetables[i].Starting_station;
cout << setw(10) << timetables[i].Last_station;
cout << setw(10) << timetables[i].time;
cout << setw(10) << timetables[i].fare;
cout << setw(15) << timetables[i].max_number;
cout << setw(10) << timetables[i].sold_number << endl;
}
// * 输入车次号,返回下标
int find(Timetable* timetables, int n, char* no)
{
int i; // * 循环变量
for ( i = 0; i < n; i++)
{
if (!strcmp(no, timetables[i].no))
{
return i;
}
}
return -1;
}
// * 按终点站进行查询,返回终点站的数目,数组b[]返回终点站的下标
int find(Timetable* timetables, int n, char* Last_station, int* b)
{
int i; // * 循环变量
int num = 0; // * 记录车次的数目
for (i = 0; i < n; i++)
{
if (!strcmp(Last_station, timetables[i].Last_station))
{
b[num] = i;
num++;
}
}
if (num != 0)
{
return num;
}
else
return -1;
}
// * 上面那个是乘客的输出,这个是管理员的输出(doge)
void BeforeOutPutBus_2()
{
cout.setf(ios::left);
cout << setw(10) << "车次号";
cout << setw(10) << "发车时间";
cout << " ";
cout << setw(10) << "始发站";
cout << setw(10) << "终点站";
cout << setw(10) << "预计用时";
cout << setw(10) << "票价";
cout << setw(15) << "最大载客量" << endl;
}
void OutPutBus_2(Timetable* timetables, int i)
{
cout.setf(ios::left);
cout << setw(10) << timetables[i].no;
cout << timetables[i].hour << ":";
cout << setw(10) << timetables[i].minute;
cout << setw(10) << timetables[i].Starting_station;
cout << setw(10) << timetables[i].Last_station;
cout << setw(10) << timetables[i].time;
cout << setw(10) << timetables[i].fare;
cout << setw(15) << timetables[i].max_number << endl;
}
美化窗口函数 windows.cpp
#include"head.h"
#include"function.h"
#define NUM1 20
#define NUM2 60
int MainWindowSelect() // * 主菜单
{
int choice;
Star();
Space();
Space();
cout << "欢迎使用汽车站车票管理系统\n\n";
Star();
Space();
Space();
cout << "1.管理员登录\n\n";
Space();
Space();
cout << "2.用户登录\n\n";
Space();
Space();
cout << "3.退出系统\n\n";
Star();
// * 进行选择
while (1)
{
cout << "请输入你要选择进行的操作:";
cin >> choice;
system("cls");
return choice;
}
}
int AdminWindowSelect() // * 管理员菜单
{
int choice;
Star();
Space();
Space();
cout << "欢迎进入管理员系统\n\n";
Star();
Space();
Space();
cout << "1.添加车次信息\n\n";
Space();
Space();
cout << "2.浏览时刻表\n\n";
Space();
Space();
cout << "3.车辆信息查询\n\n";
Space();
Space();
cout << "4.删除车辆信息\n\n";
Space();
Space();
cout << "5.返回上级菜单\n\n";
Star();
// * 进行选择
while (1)
{
cout << "请输入你要选择进行的操作:";
cin >> choice;
system("cls");
return choice;
}
return 0;
}
int PassagerWindowSelect() // * 乘客菜单
{
int choice;
Star();
Space();
Space();
cout << "欢迎进入乘客系统\n\n";
Star();
Space();
Space();
cout << "1.车辆信息查询\n\n";
Space();
Space();
cout << "2.购票\n\n";
Space();
Space();
cout << "3.退票\n\n";
Space();
Space();
cout << "4.返回上级菜单\n\n";
Star();
// * 进行选择
while (1)
{
cout << "请输入你要选择进行的操作:";
cin >> choice;
system("cls");
return choice;
}
return 0;
}
// * 退出程序
int Quit()
{
char judge;
while (1)
{
cout << "\n\n\n";
Space();
Space();
cout << "是否退出系统(y/n):";
cin >> judge;
if (judge == 'y' || judge == 'Y')
{
system("cls");
return 1;
}
if (judge == 'n' || judge == 'N')
{
system("cls");
return 0;
}
system("cls");
}
}
// * 输入回车控制
void Enter()
{
int i;
for (i = 0; i < 3; i++)
{
cout << endl;
}
}
//输入20个空格
void Space()
{
for (size_t i = 0; i < NUM1; i++)
{
cout << " ";
}
}
//输入星形
void Star()
{
Space();
for (size_t i = 0; i < NUM2; i++)
{
cout << "*";
}
cout << "\n\n";
}
管理员功能函数 admin.cpp
#include"head.h"
#include"function.h"
// * 添加车次
int AddBus(Timetable* timetables, int n)
{
int i;// * 循环变量
// * 调整输出格式
cout << "\n\n\n";
Space();
Space();
cout << "请输入车次号:";
cin >> timetables[n].no;
cout << endl;
for ( i = 0; i < n; i++)
{
if (!strcmp(timetables[i].no, timetables[n].no))
{
cout << "已存在相同车次";
return n;
}
}
Space();
Space();
cout << "请输入出发时间(小时):";
cin >> timetables[n].hour;
cout << endl;
Space();
Space();
cout << "请输入出发时间(分钟):";
cin >> timetables[n].minute;
cout << endl;
Space();
Space();
cout << "请输入始发站:";
cin >> timetables[n].Starting_station;
cout << endl;
Space();
Space();
cout << "请输入终点站:";
cin >> timetables[n].Last_station;
cout << endl;
Space();
Space();
cout << "请输入大约时长:";
cin >> timetables[n].time;
cout << endl;
Space();
Space();
cout << "请输入票价:";
cin >> timetables[n].fare;
cout << endl;
Space();
Space();
cout << "请输入最大载客量:";
cin >> timetables[n].max_number;
timetables[n].sold_number = 0;
return n + 1;
}
// * 列出所有车次
void ShowTimeTable(Timetable* timetables, int n)
{
int i;
BeforeOutPutBus_2();
for ( i = 0; i < n; i++)
{
OutPutBus_2(timetables, i);
// * 驼峰命名法
}
}
// * 车辆信息查询
void Query(Timetable* timetables, int n)
{
char find_both[20];// * 储存查询用的变量
int low[100];
int choice = QueryChioce(); // * 用来选择
int i; // * 循环变量
if (choice == 1)// * 按车次查询
{
Star();
Space();
Space();
cout << "请输入要查询的车次:";
cin >> find_both;
// * 判断find的返回值是否是-1,如果是,输出车次不存在并返回
if (find(timetables, n, find_both) == -1)
{
cout << "所查询的车次不存在!" << endl;
return;
}
BeforeOutPutBus();
OutPutBus(timetables, find(timetables, n, find_both));
}
else
{
Star();
Space();
Space();
cout << "请输入要查询的终点站:";
cin >> find_both;
// * 判断find的返回值是否是-1,如果是,输出车次不存在并返回
if (find(timetables, n, find_both, low) == -1)
{
cout << "所查询的终点站不存在!" << endl;
return;
}
BeforeOutPutBus();
for ( i = 0; i < find(timetables, n, find_both, low); i++)
{
OutPutBus(timetables, low[i]);
}
}
}
// * 删除车辆信息
int DelBus(Timetable* timetables, int n)
{
char find_no[20];
int i;
Star();
Space();
Space();
cout << "请输入要删除的车次:";
cin >> find_no;
// * 判断是否存在
if (find(timetables, n, find_no) == -1)
{
Space();
Space();
cout << "所删除的车次不存在!\n";
return n;
}
// * 删除车次
for (i = find(timetables, n, find_no); i < n; i++)
{
timetables[i] = timetables[i + 1];
}
return n - 1;
}
乘客功能函数 passage.cpp
#include"head.h"
#include"function.h"
// * 初始化乘客模式,如果有日志,就读日志,如果没有,就读取车次文件
int InitializationPassagerMode(Timetable* timetables, char* LogFileName, char* filename)
{
int n;// * 统计车次数量
int i; // * 将车次的售票量初始化为0的循环变量
fstream file_log;// * 定义日志文件操作
fstream file;// * 定义bus.txt的操作
file_log.open(LogFileName, ios::in);
if (file_log.fail())
{
// BuildLogFile(LogFileName);// * 这个函数有点没用了
n = ReadFromFile(timetables, 0, filename);
for ( i = 0; i < n; i++)
{
timetables[i].sold_number = 0;
}
WritetoFile(timetables, n, LogFileName);
return n;
}
file_log.close();
n = ReadFromFile(timetables, 0, LogFileName);
return n;
}
// * 订票
void TicketOrder(Timetable* timetables, int n)
{
char find_no[20];// * 要购买的车次
int num;
cout << "\n\n\n";
Space();
Space();
cout << "请输入要购买的车次:";
cin >> find_no;// * 输入要购买的车次
// * 判断车次是否存在
if (find(timetables, n, find_no) == -1)
{
Space();
Space();
cout << "车次不存在" << endl;
return;
}
// * 判断无法购票的条件
if (StopSevice(timetables, n, find_no))
{
Space();
Space();
cout << "无法购票!(即将发车或已经发车)" << endl;
return;
}
Space();
Space();
cout << "请输入要购买数目:";
cin >> num;// * 输入要购买数目
if ((timetables[find(timetables, n, find_no)].max_number
- timetables[find(timetables, n, find_no)].sold_number) < num)
{
Space();
Space();
cout << "余票不足!" << endl;
return;
}
timetables[find(timetables, n, find_no)].sold_number += num;
}
// * 退票
void TicketDelete(Timetable* timetables, int n)
{
char find_no[20];// * 要退票的车次
int num;// * 退票数量
cout << "\n\n\n";
Space();
Space();
cout << "请输入要退票的车次:";
cin >> find_no;// * 输入要退票的车次
// * 判断车次是否存在
if (find(timetables, n, find_no) == -1)
{
Space();
Space();
cout << "车次不存在" << endl;
return;
}
// * 判断无法退票的条件
if (StopSevice(timetables, n, find_no))
{
Space();
Space();
cout << "无法退票!(即将发车或已经发车)" << endl;
return;
}
Space();
Space();
cout << "请输入要退票数目:";
cin >> num;// * 输入要退票数目
if (timetables[find(timetables, n, find_no)].sold_number < num)
{
Space();
Space();
cout << "输入退票数目有误!" << endl;
return;
}
timetables[find(timetables, n, find_no)].sold_number -= num;
}
程序如有bug欢迎指正,编译器为2019,#pragma warning (disable:4996)是为了让一些不安全的函数能够正常使用
(使用请标明出处)
更多推荐
所有评论(0)