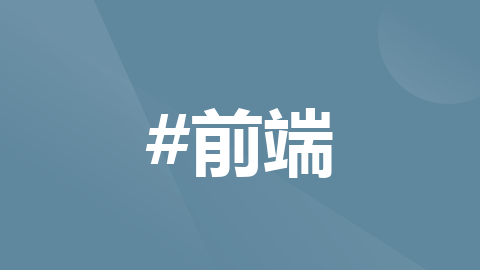
vue时间过滤器(moment)
JavaScript 日期处理类库,用于日期格式转换的。官网地址npm下载:npm install moment方法一 :局部使用在需要用的组件中引入: import moment from “moment”;使用方法 this.num = moment(this.num).format(“YYYY年”);方法二:全局使用在全局中配置(main.js文件)// main.jsimport mome
·
JavaScript 日期处理类库,用于日期格式转换的。官网地址
以下打印时间 均已 “2022-05-06 16:02:43”时间左右为准推算
npm下载: npm install moment
方法一 :局部使用
在需要用的组件中引入: import moment from “moment”;
使用方法 this.num = moment(this.num).format(“YYYY年”);
方法二:全局使用
在全局中配置(main.js文件)
// main.js
import moment from 'moment'
Vue.filter('dateFormat', (str, partten = "YYYY-MM-DD HH:mm:ss") => {
return moment(str).format(partten);
})
// 在组件中使用方法一:
<p class="mui-ellipsis">
<span>发表时间: {{ item.add_time | dateFormat }} </span>
</p>
// 2022-5-6 15:53:47
// 在组件中使用方法二:
<p class="mui-ellipsis">
<span>发表时间: {{ item.add_time | dateFormat("YYYY-MM-DD") }} </span>
</p>
// 2022-5-6
方法三:封装常用的日期处理函数
在src文件下新建utils文件夹(此文件夹一般存放自己封装的公共的工具类函数),新建tool.js文件。
//封装项目中常用的日期转换格式的函数
// 在tool.js文件中引入
import moment from "moment";
// 年-月-日
export const shortTime = function (value, formater = "YYYY-MM-DD") {
return moment(value).format(formater);
};
// 年-月-日 时:分:秒
export const time = function (value, formater = "YYYY-MM-DD HH:mm:ss") {
return moment(value).format(formater);
};
// 年/月/日 时:分:秒
export const time1 = function (value, formater = "YYYY/MM/DD HH:mm:ss") {
return moment(value).format(formater);
};
// 年/月/日 时:分
export const leaveTime = function (value) {
return moment(value).format("YYYY-MM-DD HH:mm");
};
// 年-月
export const monthTime = function (value) {
return moment(value).format("YYYY-MM");
};
// 年/月
export const monthTime1 = function (value) {
return moment(value).format("YYYY/MM");
};
// 年-月-日
export const monthTime2 = function (value) {
return moment(value).format("YYYY-MM-DD");
};
// 每月第一天
export const monthOne = function (value) {
return moment(value).format("YYYY-MM-01");
};
// 每月第一天精确
export const monthOnes = function (value) {
return moment(value).format("YYYY-MM-01 00:00:00");
};
// 补全00:00:00
export const addZero = function (value) {
return moment(value).format("YYYY-MM-DD 00:00:00");
};
// 月数
export const MonTime = function (value) {
return moment(value).format("MM");
};
// 天数
export const dayTime = function (value) {
return moment(value).format("DD");
};
// 时:分:秒
export const secondsTime = function (value) {
return moment(value).format("HH:mm:ss");
};
// 时:分
export const secondShortTime = function (value) {
return moment(value).format("HH:mm");
};
使用
// 在 ***.vue 页面中使用
// 引入自定义的函数名
// shortTime 是封装YYYY-MM-DD时间格式的函数名,
// time 是封装YYYY-MM-DD HH:mm:ss时间格式的函数名
import { shortTime, time } from "@/utils/tool";
let date = new Date()
console.log(date) // Fri May 06 2022 16:02:43 GMT+0800 (中国标准时间)
console.log(shortTime(date)) // 2022-05-06
console.log(time(date)) // 2022-05-06 16:02:43
日期格式化
moment().format('MMMM Do YYYY, h:mm:ss a'); // 举例:五月 6日 2022, 4:41:23 下午
moment().format('dddd'); // 举例:星期五
moment().format("MMM Do YY"); // 举例:5月 6日 22
moment().format('YYYY [escaped] YYYY'); // 举例:2022 escaped 2022
moment().format(); // 举例:2022-05-06T16:41:23+08:00
相对时间
moment("20111031", "YYYYMMDD").fromNow(); // ? 年前
moment("20120620", "YYYYMMDD").fromNow(); // ? 年前
moment().startOf('day').fromNow(); // ? 小时前
moment().endOf('day').fromNow(); // ? 小时内
moment().startOf('hour').fromNow(); // ? 分钟前
日历时间
//subtract 时间之前
moment().subtract(10, 'days').calendar(); // 举例:2022/04/26
moment().subtract(6, 'days').calendar(); // 举例:上星期六16:41
moment().subtract(3, 'days').calendar(); // 举例:上星期二16:41
moment().subtract(1, 'days').calendar(); // 举例:昨天16:41
moment().calendar(); // 举例:今天16:41
//add 时间之前
moment().add(1, 'days').calendar(); // 举例:明天16:41
moment().add(3, 'days').calendar(); // 举例:下星期一16:41
moment().add(10, 'days').calendar(); // 举例:2022/05/16
多语言支持
moment.locale(); // zh-cn 语言(文化)代码与国家地区
moment().format('LT'); // 16:41
moment().format('LTS'); // 16:41:23
moment().format('L'); // 2022/05/06
moment().format('l'); // 2022/5/6
moment().format('LL'); // 2022年5月6日
moment().format('ll'); // 2022年5月6日
moment().format('LLL'); // 2022年5月6日下午4点41分
moment().format('lll'); // 2022年5月6日 16:41
moment().format('LLLL'); // 2022年5月6日星期五下午4点41分
moment().format('llll'); // 2022年5月6日星期五 16:41
日期格式
格式 | 含义 | 举例 | 备注 |
---|---|---|---|
yyyy | 年 | 2022 | 同YYYY |
M | 月 | 5 | 不补0 |
MM | 月 | 01 | |
d | 日 | 6 | 不补0 |
dd | 日 | 06 | |
dddd | 星期 | 星期五 | |
H | 小时 | 8 | 24小时制;不补0 |
HH | 小时 | 16 | 24小时制 |
h | 小时 | 4 | 12小时制,须和 A 或 a 使用;不补0 |
hh | 小时 | 04 | 12小时制,须和 A 或 a 使用 |
m | 分钟 | 4 | 不补0 |
mm | 分钟 | 04 | |
s | 秒 | 5 | 不补0 |
ss | 秒 | 05 | |
A | AM/PM | AM | 仅 format 可用,大写 |
a | am/pm | am | 仅 format 可用,小写 |
更多推荐
所有评论(0)