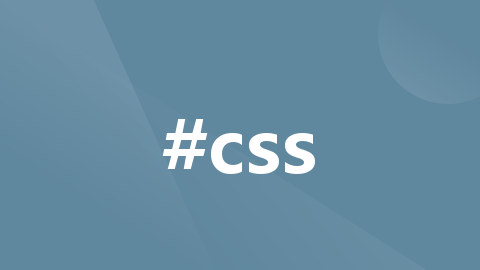
uniapp scss 的用法
scss的一些基本用法
·
变量的定义
//定义一个变量
{
$color:red;
//在css代码内部使用;
.content{
border:1px solid $color;
}
}
嵌套
//可以在css里面进行多层嵌套
{
.father{
.children{
.sun{
}
}
}
}
&符号
代替父元素 一般配合嵌套使用
scss
.container{
&-inner{
width:100px;
height:100px;
}
}
css
.container .container-inner{
width:100px;
height:100px;
}
插值 #{}
可以理解为 es6的模板字符串
/*scss*/
$name: "info";
$attr: "border";
.content-#{$name} {
#{$attr}-color: #ccc;
}
/*css*/
.content-info {
border-color: #ccc;
}
也可以在函数调用函数
定义一个函数
// 横屏转换
@function tovmin($rpx){//$rpx为需要转换的字号
@return #{$rpx * 100 / 750}vmin;
}
.container{
height:calc( 100vh = #{tovmin(200)} );
}
@mixin的使用
mixin与include配套使用
//不带参数
@mixin text-ellipsis () {
white-space: nowrap;
overflow: hidden;
text-overflow: ellipsis;
}
//使用
.text{
@include text-ellipsis;
}
//带参数
// flex布局(设置默认参数 跟函数的默认参数一样使用)
@mixin flex($content:center){
display: flex;
justify-content:$content;
align-items: center;
flex-wrap:wrap;
}
//使用
.container{
@include(space-between)
}
继承 @extend
.font{
color:red;
font-size:20px
}
.text{
@extend .font;
}
编译结果
.text{
color:red;
font-size:20px;
}
@content的使用
有点类似于vue组件的插槽
@mixin hover {
&:not([disabled]):hover {
@content;
}
}
.button {
border: 1px solid black;
@include hover {
border-width: 2px;
}
}
// 编译后
.button {
border: 1px solid black;
}
.button:not([disabled]):hover {
border-width: 2px;
}
@for循环
@for $var form <开始值> through <结束值>
@for $var form <开始值> to <结束值>
through 不包括 结束值
to 包括结束值
/*scss*/
$columns: 3;
@for $i from 1 through $columns {
.col-#{$i} {
width: 100% / $columns * $i
}
}
@for $i from 1 to $columns {
.row-#{$i} {
width: 100% / $columns * $i
}
}
/*css*/
.col-1 {
width: 25%
}
.col-2 {
width: 50%
}
.col-3 {
width: 75%
}
.row-1 {
width: 25%
}
.row-2 {
width: 50%
}
@if 条件语句
与js 中的if else相同 找到条件便会停止
@mixin txt($weight) {
color: white;
@if $weight == bold {
font-weight: bold;
}
@else if $weight == light {
font-weight: 100;
}
@else {
font-weight: normal;
}
}
.txt1 {
@include txt(bold);
}
编译结果:
.txt1{
color:white;
font-weight:bold
}
@at-root
会将嵌套的class属性提到最外层,一般的配合嵌套使用
.father{
@at-root .child{
border:1px solid black;
}
}
编译为
.father{
}
.child{
border:1px solid black;
}
@use
引入scss文件
@use "path/to/file" as prefix;
使用
.box{
width:prefix.$width;
}
Math
支持js中的一些math运算
p {
z-index: abs($number: -15); // 15
z-index: ceil(5.8); //6
z-index: max(5, 1, 6, 8, 3); //8
opacity: random(); // 随机 0-1
}
更多推荐
所有评论(0)