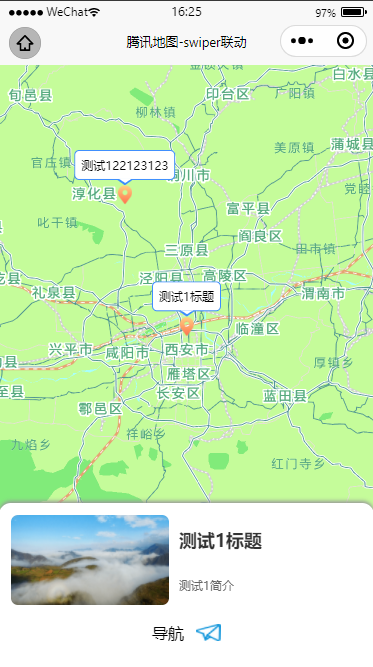
uni-app(微信小程序)中“腾讯地图”和swiper联动效果,点击移动到标记点并显示详情及导航
uni-app(微信小程序)中“腾讯地图”和swiper联动效果,点击移动到标记点并显示详情及导航
·
1、先看效果
uni-app (微信小程序中)
2、实现方式
- 加载地图(我用的腾讯地图)
使用uni.createMapContext
创建地图
onLoad() {
this.map.myMapObj = uni.createMapContext("myMap", this); // 得到map对象
this.getData();//加载数据
},
- swiper盒子放入页面,样式可根据要求自行修改
直接使用uni-app中封装的swiper,注意swiper盒子应该包含在地图map标签内部
<swiper class="swiper-box" :current="currentswiper" @change="swiperChange($event)" display-multiple-items=1>
<block>
<swiper-item class="swiperitem" v-for="(item,index) in markersData" :key='index'>
<view class="swiper-box1">
<view class="txt-img">
<image src="https://pic.imgdb.cn/item/61ef49652ab3f51d9100dd7e.png" mode=""></image>
<view class="txt-info">
<text class="title">{{item.title}}</text>
<text class="discrip">{{item.discripe}}</text>
</view>
</view>
<view class="navigation" @click="handleMapLocation(item.latitude,item.longitude,item.title)">
<text class="txt">导航</text>
<image src="../static/lnwd-img/daohang.png" mode=""></image>
</view>
</view>
</swiper-item>
</block>
</swiper>
- 具体实现
使用map组件的@markertap
点击方法,点击时调用moveToLocation
方法将地图中心移动到相应标记点,然后再动态修改swiper组件的current值
。swiper滑动时,使用swiper组件的@change
(current 改变时会触发)方法,滑动时调用map组件的moveToLocation
方法,将地图中心移动至swiper滑块对应的经纬度
markertap(e) { //标记点点击事件
let marker = this.map.markers.find(item => {return item.id == e.detail.markerId});
if(!marker) {
uni.showToast({
title: '数据加载失败',
icon:'none',
duration: 2000
});
} else {
//移动到当前定位地点
this.map.myMapObj.moveToLocation({
latitude: marker.latitude,
longitude: marker.longitude
});
};
this.currentswiper = e.detail.markerId
},
swiperChange(event) {
//移动到当前定位地点
console.log(event.detail.current)
this.map.myMapObj.moveToLocation({
latitude: this.markersData[event.detail.current]._source.latitude,
longitude: this.markersData[event.detail.current]._source.longitude
});
},
3、整体代码
<template>
<view class="map-page">
<map id="myMap" style="width: 100%; height: 90vh;" :show-location="true" :latitude="map.latitude" :longitude="map.longitude" :scale="map.scale" :markers="map.markers" :circles="map.circles" @markertap="markertap" >
<swiper class="swiper-box" :current="currentswiper" @change="swiperChange($event)" display-multiple-items=1>
<block>
<swiper-item class="swiperitem" v-for="(item,index) in testData" :key='index'>
<view class="swiper-box1">
<view class="txt-img">
<image src="https://pic.imgdb.cn/item/61ef49652ab3f51d9100dd7e.png" mode=""></image>
<view class="txt-info">
<text class="title">{{item.title}}</text>
<text class="discrip">{{item.discripe}}</text>
</view>
</view>
<view class="navigation" @click="handleMapLocation(item.latitude,item.longitude,item.title)">
<text class="txt">导航</text>
<image src="../static/lnwd-img/daohang.png" mode=""></image>
</view>
</view>
</swiper-item>
</block>
</swiper>
</map>
</view>
</template>
<style lang="less" scoped>
@import 'index.less';
</style>
<script>
export default {
data() {
return {
currentswiper: 0,//swiper的current,默认显示第一个
testData: [],//swiper数据
map: {
myMapObj: {},
latitude: '', //默认地址是后台返回数据的第一条
longitude: '',
scale: 8.5, // 默认16
markers: [],//标记点
marker: {}
},
};
},
onLoad() {
this.map.myMapObj = uni.createMapContext("myMap", this); // 得到map对象
this.getData();//加载数据
},
methods: {
swiperChange(event) {
//移动到当前定位地点
console.log(event.detail.current)
this.map.myMapObj.moveToLocation({
latitude: this.markersData[event.detail.current]._source.latitude,
longitude: this.markersData[event.detail.current]._source.longitude
});
},
markertap(e) { //标记点点击事件
let marker = this.map.markers.find(item => {return item.id == e.detail.markerId});
if(!marker) {
uni.showToast({
title: '数据加载失败',
icon:'none',
duration: 3000
});
} else {
this.map.myMapObj.moveToLocation({
latitude: marker.latitude,
longitude: marker.longitude
}); //移动到当前定位地点
};
this.currentswiper = e.detail.markerId
},
getData() { //获取地图显示的数据
uni.request({
url: '/jiekou',//后台接口地址,根据实际需要修改
method: 'POST',
header:{
'Content-Type' : 'application/json'
},
data: {
data:'canshu'//需要传的参数,根据需要修改
},
success: res => {
//请求成功
console.log(res);
this.map.latitude = res.data.data[0].latitude
this.map.longitude = res.data.data[0].longitude
this.map.markers = [];
res.data.data.forEach((item, index) => {
let marker = {
"id": index,
"latitude": item.latitude,
"longitude": item.longitude,
"width": '30rpx',
"height": '40rpx',
"iconPath": '../static/lnwd-img/location.png',
"callout": {//标记点顶部气泡
"content": item.title,
"display": "ALWAYS",
"padding": 6,
"borderRadius": 5,
"borderWidth": 1,
"borderColor": "#3D8DFE"
},
"customData": {
"title": item.title,
"describe": item.discripe,
}
};
this.map.markers.push(marker);
this.$set(this, "markersData", res.data.data);
});
console.log(this.markersData, 'this.markersData')
console.log(this.map, 'this.map')
},
fail: () => {}
});
},
handleMapLocation(lat,long,titl) {
console.log('进入导航')
// 获取定位信息
uni.getLocation({
type: 'wgs84', //返回可以用于uni.openLocation的经纬度
// 用户允许获取定位
success: (res) => {
console.log(res, '经纬度===>')
if (res.errMsg == "getLocation:ok") {
uni.openLocation({
// 传入你要去的纬度
latitude: lat,
// 传入你要去的经度
longitude: long,
// 传入你要去的地址信息 不填则为空
address: titl,
// 缩放大小
scale: 18,
success: function() {
console.log('成功的回调success');
}
});
}
},
// 用户拒绝获取定位后 再次点击触发
fail: function(res) {
console.log(res)
if (res.errMsg == "getLocation:fail auth deny") {
uni.showModal({
content: '检测到您没打开获取信息功能权限,是否去设置打开?',
confirmText: "确认",
cancelText: '取消',
success: (res) => {
if (res.confirm) {
uni.openSetting({
success: (res) => {
console.log('确定');
}
})
} else {
console.log('取消');
return false;
}
}
})
}
}
});
},
}
}
</script>
更多推荐
所有评论(0)