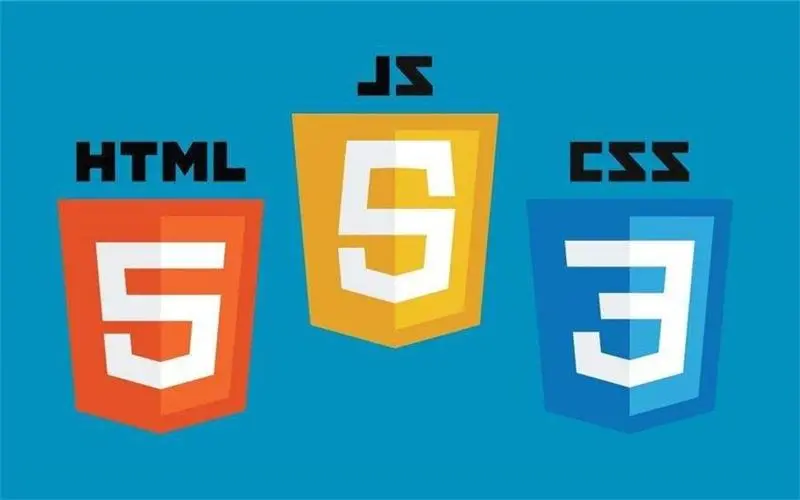
vue使用百度OCR拍照识别图片信息
原理1、获得access_token(可以前端向百度发送请求获取,也可以后端获取,传token给前端,建议后端获取),本文默认前端已获得access_token。2、调用摄像头或本地文件夹获得证件照片3、将证件照片转化为base64格式4、发送请求,反写证件信息到输入框方法1:新建handlmg.js,做好准备工作/**** @param {*base64字符串} dataurl* @return
·
原理
1、获得access_token(可以前端向百度发送请求获取,也可以后端获取,传token给前端,建议后端获取),本文默认前端已获得access_token。
2、调用摄像头或本地文件夹获得证件照片
3、将证件照片转化为base64格式
4、发送请求,反写证件信息到输入框
网址
https://ai.baidu.com/ai-doc/OCR/Ek3h7xypm
方法
1:新建handlmg.js,做好准备工作
/**
*
* @param {*base64字符串} dataurl
* @return {Blob文件类型}
*/
export function dataURLtoBlob(dataurl) {
var arr = dataurl.split(','), mime = arr[0].match(/:(.*?);/)[1],
bstr = atob(arr[1]), n = bstr.length, u8arr = new Uint8Array(n);
while(n--){
u8arr[n] = bstr.charCodeAt(n);
}
return new Blob([u8arr], {type:mime});
}
/**
* 采用递归算法,在移动端也测试过,10M也基本没问题,现在的手机随便一张照片
* 就上3M了,这可以减少不少服务器的负担
*
* @param {*img标签的加载事件参数} img
* @return {*处理最终结果返回图片base64编码} base64data
*
*/
export function scaleimg(img){
let height=img.currentTarget.height;
let width=img.currentTarget.width;
let base64data='';
let can=document.createElement("canvas");
can.height=height;
can.width=width;
let casimg=can.getContext('2d');
casimg.clearRect(0, 0,width,height);
casimg.drawImage(img.currentTarget,0,0,width,height);
base64data=can.toDataURL('image/jpeg'); //获取在canvas元素中的图片截图base64编码
let size=Math.round( dataURLtoBlob(base64data).size/1024); //获取压缩前的图片大小
let maxsize=800; //设置压缩后的最大值
if(size>maxsize){
if(size<1300){
img.currentTarget.height=Math.round(height*(3/4));
img.currentTarget.width=Math.round(width*(3/4));
return scaleimg(img)
}
else if(size<1800){
img.currentTarget.height=Math.round(height*(2/3));
img.currentTarget.width=Math.round(width*(2/3));
return scaleimg(img)
}
else{
img.currentTarget.height=Math.round(height/2);
img.currentTarget.width=Math.round(width/2);
return scaleimg(img)
}
}
else{
console.log('压缩后大小'+size);
return base64data;
}
}
2:在vue中创建input框
<input type="file" @change="uploadImg" accept="image/*" />
3:新建request.js,封装axios
import axios from 'axios'
import { MessageBox, Message } from 'element-ui'
import store from '@/store'
import { getToken } from '@/utils/auth'
// create an axios instance
const service = axios.create({
baseURL: process.env.VUE_APP_BASE_API, // url = base url + request url
// withCredentials: true, // send cookies when cross-domain requests
timeout: 5000 // request timeout
})
// request interceptor
service.interceptors.request.use(
config => {
// do something before request is sent
if (store.getters.token) {
// let each request carry token
// ['X-Token'] is a custom headers key
// please modify it according to the actual situation
config.headers['X-Token'] = getToken()
}
return config
},
error => {
// do something with request error
console.log(error) // for debug
return Promise.reject(error)
}
)
// response interceptor
service.interceptors.response.use(
/**
* If you want to get http information such as headers or status
* Please return response => response
*/
/**
* Determine the request status by custom code
* Here is just an example
* You can also judge the status by HTTP Status Code
*/
response => {
const res = response.data
// if the custom code is not 20000, it is judged as an error.
if (res.code !== 20000) {
Message({
message: res.message || 'Error',
type: 'error',
duration: 5 * 1000
})
// 50008: Illegal token; 50012: Other clients logged in; 50014: Token expired;
if (res.code === 50008 || res.code === 50012 || res.code === 50014) {
// to re-login
MessageBox.confirm('You have been logged out, you can cancel to stay on this page, or log in again', 'Confirm logout', {
confirmButtonText: 'Re-Login',
cancelButtonText: 'Cancel',
type: 'warning'
}).then(() => {
store.dispatch('user/resetToken').then(() => {
location.reload()
})
})
}
return Promise.reject(new Error(res.message || 'Error'))
} else {
return res
}
},
error => {
console.log('err' + error) // for debug
Message({
message: error.message,
type: 'error',
duration: 5 * 1000
})
return Promise.reject(error)
}
)
export default service
4:新建upload.js,接口文件
import request from '@/api/request'
//获取列表
export const uploadImg = data =>
request({
url: '/uploadimage',
method: 'POST',
data: data
})
5:在js中调用
import { dataURLtoBlob, scaleimg } from "@/utils/handlmg.js"
import { uploadImg } from '@/api/upload.js'
uploadImg(e) {
let name = e.target.name // 可用于文件校验
let filesize = file.size / 1024 // 可用于文件校验
let file = e.target.files[0]
let data = new FileReader()
let img = new Image()
let formatdata = ""
data.onload = e1 => {
img.onload = e => {
// console.log("压缩前大小" + dataURLtoBlob(e1.target.result).size / 1024); //压缩前大小
if (/^image\/([a-zA-Z]|\*)+$/.test(file.type)) {
formatdata = scaleimg(e)
//#region 提交Blob格式图片文件
// let config = {
// headers: { "Content-Type": "multipart/form-data" }
};
let param = new FormData()
param.append("file", dataURLtoBlob(formatdata));
uploadImg(param).then(res => {
document.getElementById('img').src=res.data
}).catch(res=>{
//#endregion
}
};
img.src = e1.target.result //触发加载img load事件
};
data.readAsDataURL(file) //触发加载filereader load事件
}
最后将数据传到后端,由后端解析(通过ocr),至此完结
效果如下
更多推荐
所有评论(0)