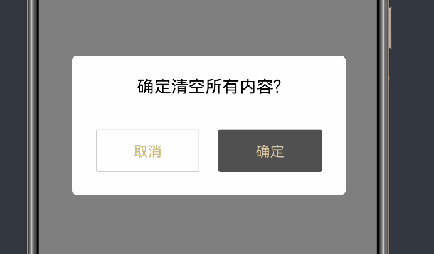
鸿蒙HarmonyOS学习笔记之基于CommonDialog实现自定义ConfirmDialog
文章目录效果预览一、基本概念二、接口说明三、实现1.ConfirmDialog类2.Dialog整体布局dialog_confirm.xml3.按钮点击效果button_confirm_dialog_ok.xmlbutton_state_natural_confirm_dialog_ok.xmlbutton_state_pressed_confirm_dialog_ok.xmlbutton_con
·
效果预览
在虚拟机上运行效果,只能通过点击取消和确定按钮关闭弹窗
一、基本概念
CommonDialog是一种在弹出框消失之前,用户无法操作其他界面内容的对话框。通常用来展示用户当前需要的或用户必须关注的信息或操作。对话框的内容通常是不同组件进行组合布局,如:文本、列表、输入框、网格、图标或图片,常用于选择或确认信息。
二、接口说明
参考官方文档
三、实现
1.ConfirmDialog类
/********
*文件名: ConfirmDialog
*创建者: 醉意丶千层梦
*创建时间:2022/2/7 17:24
*描述: ConfirmDialog
********/
public class ConfirmDialog extends CommonDialog implements Component.ClickedListener {
private Context context;
private Text detailText;
private Button okBtn,cancelBtn;
private OnDialogClickListener dialogClickListener;
public ConfirmDialog(Context context) {
super(context);
this.context=context;
//居中
setAlignment(LayoutAlignment.CENTER);
//设置高度为自适应,宽度为屏幕的0.8
setSize(context.getResourceManager().getDeviceCapability().width
* context.getResourceManager().getDeviceCapability().screenDensity
/ 160*4/5, MATCH_CONTENT);
//设置是否启用触摸对话框外区域关闭对话框的功能
setAutoClosable(false);
initComponents();
LogUtils.info(getClass().getSimpleName()+" --- create dialog");
}
@Override
public void onClick(Component component) {
if (component==okBtn){
LogUtils.info(getClass().getSimpleName()+" --- ok click");
//关闭dialog
destroy();
if(dialogClickListener != null){
dialogClickListener.onOKClick();
}
}
else if(component==cancelBtn){
LogUtils.info(getClass().getSimpleName()+" --- cancel click");
//关闭dialog
destroy();
if(dialogClickListener != null){
dialogClickListener.onCancelClick();
}
}
}
/**
*按钮接口
*/
public interface OnDialogClickListener{
void onOKClick();
void onCancelClick();
}
/**
* 初始化组件以及设置对应按钮监听事件
*/
private void initComponents(){
//设置布局xml
Component component= LayoutScatter.getInstance(context)
.parse(ResourceTable.Layout_dialog_confirm,null,true);
detailText=component.findComponentById(ResourceTable.Id_text_detail);
okBtn=component.findComponentById(ResourceTable.Id_btn_ok);
cancelBtn=component.findComponentById(ResourceTable.Id_btn_cancel);
//设置监听
okBtn.setClickedListener(this::onClick);
cancelBtn.setClickedListener(this::onClick);
super.setContentCustomComponent(component);
}
public void setOnDialogClickListener(OnDialogClickListener clickListener){
dialogClickListener = clickListener;
}
/**
* 设置提示内容
*
* @param text
*/
public void setDetailText(String text){
if (detailText!=null){
detailText.setText(text);
}
}
}
2.Dialog整体布局dialog_confirm.xml
参考原神的弹窗进行设计
<?xml version="1.0" encoding="utf-8"?>
<DirectionalLayout
xmlns:ohos="http://schemas.huawei.com/res/ohos"
ohos:height="match_content"
ohos:width="match_parent"
ohos:background_element="$graphic:background_dialog_confirm"
ohos:left_margin="5vp"
ohos:orientation="vertical"
ohos:right_margin="5vp">
<DirectionalLayout
ohos:height="match_content"
ohos:width="match_parent"
ohos:background_element="#fffffff"
>
<Text
ohos:id="$+id:text_detail"
ohos:height="match_content"
ohos:width="match_parent"
ohos:top_margin="20vp"
ohos:bottom_margin="10vp"
ohos:left_margin="20vp"
ohos:right_margin="20vp"
ohos:text="确定清空所有内容?"
ohos:text_color="#000000"
ohos:text_size="18fp"
ohos:text_alignment="center"
>
</Text>
<DirectionalLayout
ohos:height="match_content"
ohos:width="match_parent"
ohos:background_element="#ffffff"
ohos:top_padding="5vp"
ohos:bottom_padding="5vp"
ohos:bottom_margin="20vp"
ohos:orientation="horizontal"
ohos:top_margin="20vp"
ohos:layout_alignment="center"
>
<Button
ohos:id="$+id:btn_cancel"
ohos:height="45vp"
ohos:width="0vp"
ohos:weight="1"
ohos:left_margin="20vp"
ohos:right_margin="10vp"
ohos:text="取消"
ohos:text_color="#D0BE76"
ohos:text_size="15fp"
ohos:background_element="$graphic:button_dialog_cancel"
>
</Button>
<Button
ohos:id="$+id:btn_ok"
ohos:height="45vp"
ohos:width="0vp"
ohos:weight="1"
ohos:left_margin="10vp"
ohos:right_margin="20vp"
ohos:background_element="$graphic:button_dialog_ok"
ohos:text="确定"
ohos:text_color="#DCC8A1"
ohos:text_size="15fp"
>
</Button>
</DirectionalLayout>
</DirectionalLayout>
</DirectionalLayout>
3.按钮点击效果
component_state_empty要在最后一个,不然会没效果
button_confirm_dialog_ok.xml
<?xml version="1.0" encoding="utf-8"?>
<state-container
xmlns:ohos="http://schemas.huawei.com/res/ohos">
<item ohos:state="component_state_pressed"
ohos:element="$graphic:button_state_pressed_confirm_dialog_ok">
</item>
<item ohos:state="component_state_empty"
ohos:element="$graphic:button_state_natural_confirm_dialog_ok">
</item>
</state-container>
button_state_natural_confirm_dialog_ok.xml
<?xml version="1.0" encoding="utf-8"?>
<shape
xmlns:ohos="http://schemas.huawei.com/res/ohos"
ohos:shape="rectangle">
<corners ohos:radius="2vp">
</corners>
<solid ohos:color="$color:gray_515151">
</solid>
<stroke
ohos:width="1vp"
ohos:color="$color:gray_515151"/>
<bounds ohos:top="1vp"></bounds>
</shape>
button_state_pressed_confirm_dialog_ok.xml
<?xml version="1.0" encoding="utf-8"?>
<shape
xmlns:ohos="http://schemas.huawei.com/res/ohos"
ohos:shape="rectangle">
<corners ohos:radius="2vp">
</corners>
<solid ohos:color="$color:gray_2C2C2C">
</solid>
<stroke
ohos:width="1vp"
ohos:color="$color:gray_2C2C2C"/>
<bounds ohos:top="1vp"></bounds>
</shape>
button_confirm_dialog_cancel.xml
<?xml version="1.0" encoding="utf-8"?>
<state-container
xmlns:ohos="http://schemas.huawei.com/res/ohos">
<item ohos:state="component_state_pressed"
ohos:element="$graphic:button_state_pressed_confirm_dialog_cancel">
</item>
<item ohos:state="component_state_empty"
ohos:element="$graphic:button_state_natural_confirm_dialog_cancel">
</item>
</state-container>
button_state_natural_confirm_dialog_cancel.xml
<?xml version="1.0" encoding="utf-8"?>
<shape
xmlns:ohos="http://schemas.huawei.com/res/ohos"
ohos:shape="rectangle">
<corners ohos:radius="2vp">
</corners>
<solid ohos:color="$color:white_FFFFFF">
</solid>
<stroke
ohos:width="1vp"
ohos:color="$color:gray_CDCDCD"/>
<bounds ohos:top="1vp"></bounds>
</shape>
button_state_pressed_confirm_dialog_cancel.xml
<?xml version="1.0" encoding="utf-8"?>
<shape
xmlns:ohos="http://schemas.huawei.com/res/ohos"
ohos:shape="rectangle">
<corners ohos:radius="2vp">
</corners>
<solid ohos:color="$color:white_DFDFDF">
</solid>
<stroke
ohos:width="1vp"
ohos:color="$color:white_DFDFDF"/>
<bounds ohos:top="1vp"></bounds>
</shape>
4.预览
四、使用
ConfirmDialog confirmDialog = new ConfirmDialog(getContext());
confirmDialog.setOnDialogClickListener(new ConfirmDialog.OnDialogClickListener() {
@Override
public void onOKClick() {
}
@Override
public void onCancelClick() {
}
});
confirmDialog.show();
更多推荐
所有评论(0)