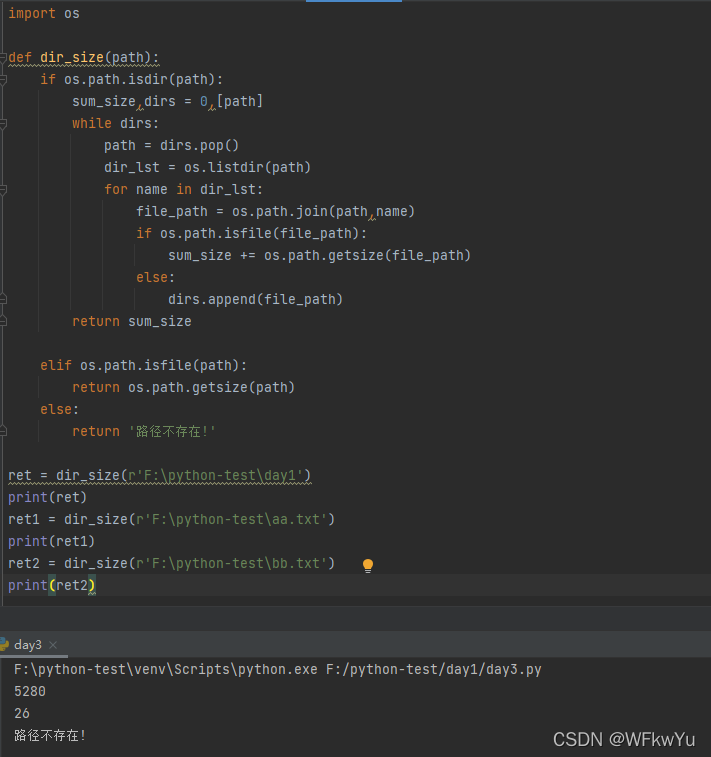
Python小技巧
1、编写一个程序,能在当前目录以及当前目录的所有子目录下查找文件名包含指定字符串的文件,并打印出相对路径。#!/usr/bin/python# -*-* coding: utf-8 -*-import osdef findfile(key_name,dir_path):filename_list = [x for x in os.listdir(dir_path) if os.path.isfil
·
1、编写一个程序,能在当前目录以及当前目录的所有子目录下查找文件名包含指定字符串的文件,并打印出相对路径。
#!/usr/bin/python
# -*-* coding: utf-8 -*-
import os
def findfile(key_name,dir_path):
filename_list = [x for x in os.listdir(dir_path) if os.path.isfile(x)]
for filename in filename_list:
if filename.find(key_name) != -1:
print(os.path.join(dir_path,filename))
else:
pass
dirname_list = [ x for x in os.listdir(dir_path) if os.path.isdir(x)]
for dirname in dirname_list:
nextdir_path=os.path.join(dir_path,dirname)
findfile(key_name,nextdir_path)
def main():
dir_path = input('请输入绝对路径:')
key_name = input('请输入要查找的字符串:')
result = findfile(key_name, dir_path)
if __name__ == '__main__':
main()
2、将字典写入文件(序列化 json.dump())
#!/usr/bin/python
# -*- coding: utf8 -*-
import json
## 定义一个字典
d = dict(name='Bob',age=20,score=88)
## 写入文件
with open('dump1.txt','w') as f:
json.dump(d,f)
三、计算文件夹大小
1、采用非递归方法
import os
def dir_size(path):
if os.path.isdir(path):
sum_size,dirs = 0,[path]
while dirs:
path = dirs.pop()
dir_lst = os.listdir(path)
for name in dir_lst:
file_path = os.path.join(path,name)
if os.path.isfile(file_path):
sum_size += os.path.getsize(file_path)
else:
dirs.append(file_path)
return sum_size
elif os.path.isfile(path):
return os.path.getsize(path)
else:
return '路径不存在!'
ret = dir_size(r'F:\python-test\day1')
print(ret)
ret1 = dir_size(r'F:\python-test\aa.txt')
print(ret1)
ret2 = dir_size(r'F:\python-test\bb.txt')
print(ret2)
2、采用递归方法
import os
def dir_size(path):
sum_size = 0
if os.path.isdir(path):
dir_lst = os.listdir(path)
for name in dir_lst:
full_path = os.path.join(path,name)
if os.path.isfile(full_path):
sum_size += os.path.getsize(full_path)
else:
ret = dir_size(full_path)
sum_size += ret
return sum_size
elif os.path.isfile(path):
return os.path.getsize(path)
else:
return '路径不存在!'
ret = dir_size(r'F:\python-test\day1')
print(ret)
ret1 = dir_size(r'F:\python-test\aa.txt')
print(ret1)
ret2 = dir_size(r'F:\python-test\bb.txt')
print(ret2)
迭代器:l=[i for i in range(10)]
生成器:g=(i for i in range(10))
print(l)
print(next(g))
classmethod和staticmethod区别?
都可以通过Class.method()的方式使用
classmethod第一个参数是cls,可以引用类变量
staticmethod使用起来和普通函数一样,只不过放在类里去组织
classmethod是为了使用类变量,staticmethod是代码组织的需要,完全可以放到类之外
class Person:
Country = 'china'
def __init__(self, name, age):
self.name = name
self.age = age
def print_name(self):
print(self.name)
@classmethod # 传入的是类cls,不是self,用来引用类变量cls.Country
def print_country(cls):
print(cls.Country)
@staticmethod # 既没有传cls也没有传self,那么就要用staticmethod
def join_name(first_name, last_name):
return print(last_name + first_name)
a = Person("Bruce", "Lee")
a.print_country()
a.print_name()
a.join_name("Bruce", "Lee")
Person.print_country()
Person.print_name(a)
Person.join_name("Bruce", "Lee")
更多推荐
所有评论(0)