基于Springboot+vue的员工管理系统--前端笔记
1.前言之前我们员工管理系统的后端笔记已经书写完毕,现在我们整理一下前端的笔记。2.安装Vue环境,并新建Vue的项目过程比较简单,此处省略....3.登录界面的开发一般的登录界面就是简单的表单:用户名、登录密码、验证码。然后我们涉及到的后台交互有2个:获取登录验证码提交表单完成登录登录交互的过程:浏览器打开登录页面动态加载登录验证码,后端生成的验证码存在前端的sessionStorage中前端提
1.前言
之前我们员工管理系统的后端笔记已经书写完毕,现在我们整理一下前端的笔记。
2.安装Vue环境,并新建Vue的项目
过程比较简单,此处省略....
3.登录界面的开发
一般的登录界面就是简单的表单:用户名、登录密码、验证码。然后我们涉及到的后台交互有2个:
- 获取登录验证码
- 提交表单完成登录
登录交互的过程:
浏览器打开登录页面
动态加载登录验证码,后端生成的验证码存在前端的sessionStorage中
前端提交用户名、密码和验证码,后台验证验证码是否匹配以及密码是否正确
登录功能实现的js代码:
<template>
<div>
<el-form :rules="rules" ref="loginForm" :model="loginForm" class="login">
<h3 class="title" style="font-family: 华文行楷; font-size: 30px;color: #545c64;margin-top: 10px">后台登录</h3>
<el-form-item prop="username">
<el-input type="text" auto-complete="false" v-model="loginForm.username" placeholder="请输入用户名"></el-input>
</el-form-item>
<el-form-item prop="password">
<el-input type="password" auto-complete="false" v-model="loginForm.password" placeholder="请输入密码"></el-input>
</el-form-item>
<el-form-item ref="code">
<el-input type="text" auto-complete="false" v-model="loginForm.code" placeholder="点击图片更换验证码"
style="width: 250px;margin-right: 5px"></el-input>
<img :src="captchaUrl" @click="update">
</el-form-item>
<el-checkbox v-model="checked" class="remember">记住我</el-checkbox>
<el-button type="primary" style="width: 100%" @click="submits">登录</el-button>
</el-form>
</div>
</template>
<script>
export default {
name:'Login',
data(){
return{
captchaUrl:'http://localhost:8001/captcha?time='+new Date(),
loginForm:{
username:"admin",
password:"123",
code:""
},
checked:true,
rules:{
username:[{
required:true,
message:"请输入用户名",
trigger:"blur"
}],
password:[{
required:true,
message:"请输入密码",
trigger:"blur"
}],
code:[{
required:true,
message:"请输入验证码",
trigger:"blur"
}]
}
}
},
methods:{
update(){
this.captchaUrl='http://localhost:8001/captcha?time='+new Date();
},
submits(){
this.$refs.loginForm.validate((valid) => {
if (valid) {
const _this=this
this.axios.post('http://localhost:8001/login',this.loginForm).then(function (response){
if (response){
console.log(response)
console.log(response.obj)
//存储用户的token
const tokenStr=response.obj.tokenHead+response.obj.token;
window.sessionStorage.setItem('tokenStr',tokenStr);
//跳转页面
const path=_this.$route.query.redirect;
_this.$router.replace((path== '/' ||path== undefined) ? '/employ': path);
}
})
}else {
this.$message.error('请输入所有的字段');
}
});
}
}
}
</script>
<style>
.login{
border-radius: 15px;
background-clip: padding-box;
margin: 180px auto;
width: 350px;
height: 400px;
padding: 15px 35px 0px 35px;
background:white;
border: 1px solid #cac6c6;
box-shadow: 0 0 25px #cac6c6;
}
.title{
margin: 10px auto 35px auto;
text-align: center;
}
.remember{
text-align: left;
margin: 0px 0px 15px;
}
.el-form-item__content{
display: flex;
align-items: center;
}
</style>
token的状态同步
submit方法中,提交表单之后,从Header中获取用户的Authroization,也就是含有用户信息的jwt,然后保存到sessionStorage中
定义全局axios拦截器
import Vue from 'vue'
import Vuex from 'vuex'
import axios from "axios";
import {Message} from "element-ui";
import router from "../router";
Vue.use(Vuex)
//请求拦截器
axios.interceptors.request.use(config=>{
//如果存在token,请求携带这个token
if(window.sessionStorage.getItem("tokenStr")){
config.headers['Authorization']=window.sessionStorage.getItem('tokenStr');
}
return config;
},error => {
console.log(error);
})
//响应拦截器
axios.interceptors.response.use(success =>{
//业务逻辑错误
if (success.status && success.status==200){
if (success.data.code==500||success.data.code==401||success.data.code==403){
Message.error({message:success.data.message});
return;
}
if(success.data.message){
Message.success({message: success.data.message});
}
}
return success.data;
},error =>{
if(error.response.code==504||error.response.code==404){
Message.error({message:"服务器被吃掉了"});
}else if(error.response.code==403){
Message.error({message:"权限不足,请联系管理员!"});
}else if(error.response.code==401){
Message.error({message:"尚未登录,请登录!"});
router.push('/');
}else{
if(error.response.data.message){
Message.error({message:error.response.data.message});
}else {
Message.error({message:"未知错误!!"});
}
}
return;
});
请求拦截器,其实可以统一为所有需要权限的请求装配上header的token信息,响应拦截器,判断states.code和error.response.status,如果是401未登录没有权限的就跳到登录界面,其他的就直接弹框显示错误。在没有登录之前不允许访问任何接口,如果执意输入接口的地址将重定向到登录界面。
import axios from "axios";
import './plugins/element.js'
import {downloadRequest} from "./util/download";
import 'font-awesome/css/font-awesome.css'
Vue.config.productionTip = false
Vue.prototype.axios=axios
Vue.prototype.downloadRequest=downloadRequest
router.beforeEach((to, from, next) => {
console.log(to),
console.log(from)
if (window.sessionStorage.getItem('tokenStr')){
initMenu(router,store);
next();
}else {
if (to.path== '/') {
next();
} else {
next('/?redirect=' + to.path);
}
}
})
new Vue({
router,
store,
render: h => h(App)
}).$mount('#app')
这样axios每次请求都会被请求拦截器和响应拦截器拦截了
登录异常弹窗效果:
4.后台管理界面的开发:
登录界面我们已经开发完毕,接下来我们就开发首页的页面
一般来说管理系统的界面都是这样的,头部是一个简单的信息展示系统名称和登录用户的信息,然后中间的左边是菜单导航栏,右边是内容。而Header和Aside是不变的,只有Main部分会跟着链接变化而变化,所以我们提取出公共的部分放在Home.Vue中,然后Main部分放在Index.vue中。我们需要重新定义路由,以及路由是Home.vue,Index.vue作为Home.vue页面的子路由,然后Home.vue中我们通过<router-view>来展示Inedx.vue的内容即可。
在router中我们可以这样修改:
Vue.use(VueRouter)
const routes = [
{
path: '/',
name:'登录',
component: Login,
hidden:true
},
{
path: '/home',
name:'系统管理',
component: Home,
children:[
{
path: '/employ',
name:'用户管理',
component: EmployMangger
},
{
path: '/personal',
name:'个人中心',
component: Personal
}
]
}
]
然后我们再书写Home的内容:
<template>
<div>
<el-container style="height: 800px; border: 1px solid #eee">
<el-header style="text-align: center; font-size: 30px;font-family: 华文行楷; background-color: #545c64;">
<span style="margin-left: 450px;margin-top: 20px; color: #eeeeee">员工后台管理系统</span>
<el-dropdown class="userinfo" @command="commandHandler">
<span style="margin-left: 450px;margin-top: 5px;- color: #42b983">
<el-avatar style="margin-top: 10px" src="https://cube.elemecdn.com/0/88/03b0d39583f48206768a7534e55bcpng.png"></el-avatar>
</span>
<el-dropdown-menu slot="dropdown">
<el-dropdown-item command="userinfo">个人中心</el-dropdown-item>
<el-dropdown-item command="setting">设置</el-dropdown-item>
<el-dropdown-item command="logout">注销登录</el-dropdown-item>
</el-dropdown-menu>
</el-dropdown>
</el-header>
<el-container>
<el-aside width="200px" style="background-color: whitesmoke">
<el-menu router unique-opened>
<el-submenu :index="index+''"
v-for="(item,index) in routes" :key="index"
v-if="!item.hidden">
<template slot="title">
<i :class="item.iconCls" style="color: lightslategray;margin-right: 5px"></i>
<span>{{item.name}}</span>
</template>
<el-menu-item :index="children.path"
v-for="(children,indexj) in item.children" :key="indexj">
<i :class="children.iconCls" style="color: lightslategray;margin-right: 5px"></i>
<span>{{children.name}}</span>
</el-menu-item>
</el-submenu>
</el-menu>
</el-aside>
<el-main>
<router-view/>
</el-main>
</el-container>
</el-container>
</div>
</template>
<style>
.el-menu-vertical-demo:not(.el-menu--collapse) {
width: 200px;
min-height: 400px;
}
.el-dropdown-link {
cursor: pointer;
color: #409EFF;
}
.el-icon-arrow-down {
font-size: 12px;
}
.userinfo{
cursor: pointer;
}
</style>
<script>
import axios from "axios";
export default {
name:'Home',
computed:{
routes(){
return this.$store.state.routes;
}
},
data() {
return {
dialogVisible:false,
isCollapse: true,
};
},
methods: {
commandHandler(command){
if (command=='logout'){
this.$confirm('此操作将注销用户, 是否继续?', '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning'
}).then(() => {
//注销登录
axios.post('http://localhost:8001/logout');
window.sessionStorage.removeItem('tokenStr');
//清空菜单信息
this.$store.commit('initRoutes',[]);
this.$router.push('/')
}).catch(() => {
this.$message({
type: 'info',
message: '已取消注销'
});
});
}
if (command=='userinfo'){
//
this.$router.push('/personal')
}
},
handleOpen(key, keyPath) {
console.log(key, keyPath);
},
handleClose(key, keyPath) {
console.log(key, keyPath);
}
}
}
</script>
首页中间的内容
<template>
<div class="title">
欢迎使用员工后台管理系统
</div>
</template>
<script>
export default {
name: "Index"
}
</script>
<style>
.title{
font-size: 50px;
font-family: 宋体;
text-align: center;
margin-top: 200px;
color: blue;
}
</style>
总体效果如下:
菜单栏是动态显示的
用户登录信息的展示:
因为我们现在已经登录成功,所以我们可以通过接口去请求获取到当前登录的信息了,这样我们就可以动态的显示用户的信息了,这个接口比骄简单,然后注销登陆的链接也一起完成,就请求接口然后把浏览器中的缓存删除就退出了
<template>
<div>
<el-container style="height: 800px; border: 1px solid #eee">
<el-dropdown-menu slot="dropdown">
<el-dropdown-item command="userinfo">个人中心</el-dropdown-item>
<el-dropdown-item command="setting">设置</el-dropdown-item>
<el-dropdown-item command="logout">注销登录</el-dropdown-item>
</el-dropdown-menu>
</el-dropdown>
<el-main>
<router-view/>
</el-main>
</el-container>
</el-container>
</div>
</template>
<style>
.el-menu-vertical-demo:not(.el-menu--collapse) {
width: 200px;
min-height: 400px;
}
.el-dropdown-link {
cursor: pointer;
color: #409EFF;
}
.el-icon-arrow-down {
font-size: 12px;
}
.userinfo{
cursor: pointer;
}
</style>
<script>
import axios from "axios";
export default {
name:'Home',
computed:{
routes(){
return this.$store.state.routes;
}
},
data() {
return {
dialogVisible:false,
isCollapse: true,
};
},
methods: {
commandHandler(command){
if (command=='logout'){
this.$confirm('此操作将注销用户, 是否继续?', '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning'
}).then(() => {
//注销登录
axios.post('http://localhost:8001/logout');
window.sessionStorage.removeItem('tokenStr');
//清空菜单信息
this.$store.commit('initRoutes',[]);
this.$router.push('/')
}).catch(() => {
this.$message({
type: 'info',
message: '已取消注销'
});
});
}
if (command=='userinfo'){
//
this.$router.push('/personal')
}
},
handleOpen(key, keyPath) {
console.log(key, keyPath);
},
handleClose(key, keyPath) {
console.log(key, keyPath);
}
}
}
</script>
动态菜单栏的显示:
首先我们封装菜单的工具类
export const initMenu=(router,store)=>{
if (store.state.routes.length>0){
return;
}
axios.get("http://localhost:8001/employ/menu").then(response=>{
if(response){
console.log(response)
//格式化Router
let fmtRoutes=formatRoutes(response);
router.addRoutes(fmtRoutes);
store.commit('initRoutes',fmtRoutes);
}
})
}
export const formatRoutes=(routes)=>{
let fmtRoutes=[];
routes.forEach(router=>{
let{
path,
component,
name,
iconCls,
children,
}=router;
if(children && children instanceof Array){
//递归
children=formatRoutes(children);
}
let fmtRouter={
path:path,
name:name,
iconCls:iconCls,
children:children,
component(reslove){
require(['../views/'+component+'.vue'],reslove);
}
}
fmtRoutes.push(fmtRouter)
});
return fmtRoutes;
}
export default new Vuex.Store({
state: {
routes:[]
},
mutations: {
initRoutes(state,data){
state.routes=data;
}
},
actions: {
},
modules: {
}
})
首先定义一个初始化菜单的方法,判断菜单是否存在,存在的话就直接返回,否则通过get请求获取菜单
我们将写死的数据简化成一个json数组数据,然后for循环展示出来,代码如下:
<el-container>
<el-aside width="200px" style="background-color: whitesmoke">
<el-menu router unique-opened>
<el-submenu :index="index+''"
v-for="(item,index) in routes" :key="index"
v-if="!item.hidden">
<template slot="title">
<i :class="item.iconCls" style="color: lightslategray;margin-right: 5px"></i>
<span>{{item.name}}</span>
</template>
<el-menu-item :index="children.path"
v-for="(children,indexj) in item.children" :key="indexj">
<i :class="children.iconCls" style="color: lightslategray;margin-right: 5px"></i>
<span>{{children.name}}</span>
</el-menu-item>
</el-submenu>
</el-menu>
</el-aside>
<el-main>
<router-view/>
</el-main>
</el-container>
同时我们还通过判断是否登录页面,是否含有token等判断提前判断是否加载菜单
代码如下:
router.beforeEach((to, from, next) => {
console.log(to),
console.log(from)
if (window.sessionStorage.getItem('tokenStr')){
initMenu(router,store);
next();
}else {
if (to.path== '/') {
next();
} else {
next('/?redirect=' + to.path);
}
}
})
new Vue({
router,
store,
render: h => h(App)
}).$mount('#app')
这里我们用到了导航守卫,前置守卫,首先判断是否存在token,如果存在,则初始化菜单,如果不存在,输入的如果是‘/’则跳转到登录的界面,否则重定向到登录的界面
个人中心:
个人中心是用来展示用户的基本信息,修改用户的基本信息和修改密码,相对比较简单
<template>
<div>
<el-card class="box-card">
<div slot="header" class="clearfix">
<span>{{admin.username}}</span>
</div>
<div class="text item">
<div>
<div>姓名:<el-tag>{{admin.username}}</el-tag></div>
<div>性别:<el-tag>{{admin.sex}}</el-tag></div>
<div>邮箱:<el-tag>{{admin.email}}</el-tag></div>
<div>地址:<el-tag>{{admin.address}}</el-tag></div>
<div style="margin-top: 15px">
<el-button type="primary" size="mini" @click="showadmin">修改用户信息</el-button>
<el-button type="danger" size="mini" @click="password">修改密码</el-button>
</div>
</div>
</div>
</el-card>
<el-dialog
title="编辑用户信息"
:visible.sync="dialogVisible"
width="30%">
<div>
<tr>
<td>用户昵称:</td>
<td><el-input v-model="admin.username"></el-input></td>
</tr>
<tr>
<td>用户性别:</td>
<td><el-input v-model="admin.sex"></el-input></td>
</tr>
<tr>
<td>用户邮箱:</td>
<td><el-input v-model="admin.email"></el-input></td>
</tr>
<tr>
<td>用户地址:</td>
<td><el-input v-model="admin.address"></el-input></td>
</tr>
</div>
<span slot="footer" class="dialog-footer">
<el-button @click="dialogVisible = false">取 消</el-button>
<el-button type="primary" @click="dialogVisible = false">确 定</el-button>
</span>
</el-dialog>
<el-dialog
title="修改用户密码"
:visible.sync="passworddialogVisible"
width="30%">
<el-form :model="passForm" status-icon :rules="rules" ref="passForm" label-width="100px">
<el-form-item label="旧密码" prop="currentPass">
<el-input type="password" v-model="passForm.currentPass" autocomplete="off" ></el-input>
</el-form-item>
<el-form-item label="新密码" prop="password">
<el-input type="password" v-model="passForm.password" autocomplete="off"></el-input>
</el-form-item>
<el-form-item label="确认密码" prop="checkPass">
<el-input type="password" v-model="passForm.checkPass" autocomplete="off"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="submitForm('passForm')">提交</el-button>
<el-button @click="resetForm('passForm')">重置</el-button>
</el-form-item>
</el-form>
<span slot="footer" class="dialog-footer">
</span>
</el-dialog>
</div>
</template>
<script>
import axios from "axios";
export default {
name: "Personal",
data(){
var validatePass = (rule, value, callback) => {
if (value === '') {
callback(new Error('请再次输入密码'));
} else if (value !== this.passForm.password) {
callback(new Error('两次输入密码不一致!'));
} else {
callback();
}
};
return{
dialogVisible:false,
passworddialogVisible:false,
admin:'',
passForm: {
password: '',
checkPass: '',
currentPass: ''
},
rules: {
password: [
{ required: true, message: '请输入新密码', trigger: 'blur' },
{ min: 6, max: 12, message: '长度在 6 到 12 个字符', trigger: 'blur' }
],
checkPass: [
{ required: true, validator: validatePass, trigger: 'blur' }
],
currentPass: [
{ required: true, message: '请输入当前密码', trigger: 'blur' },
]
}
};
},
mounted() {
this.initAdmin();
},
methods:{
showadmin(){
this.dialogVisible=true
},
password(){
this.passworddialogVisible=true
},
initAdmin(){
axios.get("http://localhost:8001/admin/info").then(response=>{
if (response){
this.admin=response
}
})
},
submitForm(formName) {
this.$refs[formName].validate((valid) => {
if (valid) {
const _this = this
this.axios.post('http://localhost:8001/people/updatePass', this.passForm).then(res => {
if (res){
_this.passworddialogVisible=false
}
})
} else {
console.log('error submit!!');
return false;
}
});
},
resetForm(formName) {
this.$refs[formName].resetFields();
}
}
}
</script>
<style scoped>
.box-card{
width: 350px;
}
</style>
员工信息界面
主要实现对员工的增删改查,数据的导入导出和分页显示.
<template>
<div>
<el-container style=" height: max-content;border: 1px solid #eee">
<el-container>
<el-main>
<div style="display: flex;justify-content: space-between">
<div >
<el-input style="width: 200px;margin-right: 20px" prefix-icon="el-icon-search" v-model.trim="employ.name" @keydown.enter.native="initEmps" placeholder="请输入员工的姓名或编号进行查询.."></el-input>
<el-input style="width: 200px;margin-right: 20px" prefix-icon="el-icon-search" v-model.trim="employ.provices" @keydown.enter.native="initEmps" placeholder="请输入员工的籍贯进行查询.."></el-input>
<el-button type="primary" icon="el-icon-search" @click="initEmps">搜索</el-button>
<el-button type="danger" icon="el-icon-delete" :disabled="this.multipleSelection.length==0" @click="deletemany">批量删除</el-button>
</div>
<div>
<el-upload
style="display: inline-flex;margin-right: 10px"
:headers="headers"
:show-file-list="false"
:before-upload="beforeUpload"
:on-success="onSuccess"
:on-error="onError"
:disabled="DataDisabled"
action="http://localhost:8001/employ/import/">
<el-button type="success" :icon="importIcon" :disabled="DataDisabled">
{{importData}}
</el-button>
</el-upload>
<el-button @click="exportData" type="success"icon="el-icon-download">
<i class="fa fa-level-up" aria-hidden="true"></i>
导出数据
</el-button>
</div>
</div>
<el-table
:data="list"
border
style="width: 100%;margin-top: 15px"
@selection-change="handleSelectionChange">
<el-table-column
type="selection"
width="55">
</el-table-column>
<el-table-column
fixed
prop="id"
label="编号"
width="50">
</el-table-column>
<el-table-column
prop="name"
label="姓名"
width="120">
</el-table-column>
<el-table-column
prop="sex"
label="性别"
width="120">
</el-table-column>
<el-table-column
prop="provices"
label="籍贯"
width="120">
</el-table-column>
<el-table-column
prop="age"
label="年龄"
width="120">
</el-table-column>
<el-table-column
prop="salary"
label="薪水"
width="120">
</el-table-column>
<el-table-column
prop="address"
label="地址"
width="140">
</el-table-column>
<el-table-column
prop="school"
label="学校"
width="120">
</el-table-column>
<el-table-column
prof="right"
label="操作"
width="200">
<div style="display: flex;justify-content: space-between" slot-scope="scope">
<el-button @click="userDelete(scope.row)" type="danger" size="mini" icon="el-icon-delete-solid">删除</el-button>
<el-button @click="findUser(scope.$index,scope.row)" type="primary" size="mini" icon=" el-icon-edit">编辑</el-button>
</div>
</el-table-column>
</el-table>
<div style="display: flex;justify-content: flex-end">
<el-pagination
background
@current-change="currentchange"
layout='prev, pager, next, jumper, ->, total'
:page-size="size"
:total="total"
/>
</div>
<div style="text-align: center;">
<el-dialog
title="修改员工信息"
:visible.sync="dialogVisible"
width="30%"
:before-close="handleClose">
<div>
<div>
<el-tag>编号:</el-tag>
<el-input v-model="updatepos.id" prefix-icon="el-icon-edit" placeholder="请输入员工编号" style="width: 150px;margin-left: 10px;height: 20px"></el-input>
</div>
<div>
<el-tag>姓名:</el-tag>
<el-input v-model="updatepos.name" prefix-icon="el-icon-edit" placeholder="请输入姓名" style="width: 150px;margin-left: 10px;height: 20px;margin-top: 10px"></el-input>
</div>
<div>
<el-tag>籍贯:</el-tag>
<el-select v-model="updatepos.provices" placeholder="请选择...."style="width: 150px;margin-left: 10px;height: 20px;margin-top: 10px">
<el-option label="北京" value="北京"></el-option>
<el-option label="天津" value="天津"></el-option>
<el-option label="上海" value="上海"></el-option>
<el-option label="甘肃" value="甘肃"></el-option>
<el-option label="深圳" value="深圳"></el-option>
<el-option label="广州" value="广州"></el-option>
<el-option label="河南" value="河南"></el-option>
<el-option label="河北" value="河北"></el-option>
<el-option label="山东" value="山东"></el-option>
<el-option label="海南" value="海南"></el-option>
</el-select>
</div>
<div>
<el-tag>年龄:</el-tag>
<el-input v-model="updatepos.age"prefix-icon="el-icon-edit" placeholder="请输入年龄" style="width: 150px;margin-left: 10px;height: 20px;margin-top: 10px"></el-input>
</div>
<div>
<el-tag>薪水:</el-tag>
<el-input v-model="updatepos.salary"prefix-icon="el-icon-edit" placeholder="请输入薪水" style="width: 150px;margin-left: 10px;height: 20px;margin-top: 10px"></el-input>
</div>
<div>
<el-tag>地址:</el-tag>
<el-input v-model="updatepos.address"prefix-icon="el-icon-edit" placeholder="请输入地址" style="width: 150px;margin-left: 10px;height: 20px;margin-top: 10px"></el-input>
</div>
<div>
<el-tag>学校:</el-tag>
<el-input v-model="updatepos.school"prefix-icon="el-icon-edit" placeholder="请输入学校" style="width: 150px;margin-left: 10px;height: 20px;margin-top: 10px"></el-input>
</div>
</div>
<span slot="footer" class="dialog-footer">
<el-button @click="dialogVisible = false">取消修改</el-button>
<el-button type="primary" @click="submitForm">保存修改</el-button>
</span>
</el-dialog>
</div>
</el-main>
</el-container>
</el-container>
</div>
</template>
<script>
import axios from "axios";
export default {
name:"EmployMangger",
data() {
return {
headers:{
Authorization:window.sessionStorage.getItem("tokenStr")
},
DataDisabled:false,
importData:'导入数据',
importIcon:'el-icon-upload2',
list:[],
total:0,
current:1,
size:10,
employ:{},
multipleSelection:[],//复选框
dialogVisible:false,//弹出框
updatepos:{//回显数据
id: '',
name: '',
provices:'',
age: '',
salary: '',
address: '',
school: ''
}
}
},
created() {
this.initEmps();
},
methods: {
//导入数据
beforeUpload(){
this.importIcon='el-icon-loading';
this.importData='正在导入...';
this.DataDisabled=true;
},
//导入成功
onSuccess(){
this.importIcon='el-icon-upload2';
this.importData='导入数据';
this.DataDisabled=false;
this.$notify({
title: '成功',
message: '数据导入成功',
type: 'success'
});
this.initEmps();
},
onError(){
this.importIcon='el-icon-upload2';
this.importData='导入数据';
this.DataDisabled=false;
},
//导出数据
exportData(){
this.downloadRequest('/export')
},
//更新数据
submitForm() {
const _this=this
this.axios.post('http://localhost:8001/employ/update',this.updatepos).then(function (response) {
if (response){
_this.dialogVisible=false;
_this.initEmps();//刷新页面
_this.$notify({
title: '成功',
message: '成功修改一条记录',
type: 'success'
});
}
})
},
handleClose(done) {
this.$confirm('确认关闭?')
.then(_ => {
done();
})
.catch(_ => {});
},
//批量删除
deletemany(){
let _this = this
this.$confirm('此操作将永久删除['+this.multipleSelection.length+']个用户, 是否继续?', '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning',
center: true
}).then(() => {
let ids='?';
this.multipleSelection.forEach(item=>{
ids+='ids='+item.id+'&';
})
axios.delete('http://localhost:8001/employ/ids/'+ids).then(function (response) {
console.log(response)
if (response) {
_this.$alert('删除成功!', {
confirmButtonText: '确定',
callback: action => {
//重新载入文档信息
location.reload()
}
});
}
})
}).catch(() => {
this.$message({
type: 'info',
message: action === 'cancel'
? '放弃保存并离开页面'
: '停留在当前页面'
})
})
},
//多选框选中的个数
handleSelectionChange(val){//val表示勾选的对象
this.multipleSelection=val;
console.log(val)
}
,
//分页显示
currentchange(current) {
this.current=current;
this.initEmps();
},
//修改数据回显数据
findUser(index,data) {
//this.updatepos=data;会出现即时修改的情况
Object.assign(this.updatepos,data);
this.dialogVisible=true;
},
//搜索功能
search(row) {
this.$router.push({//跳转页面
path: '/findby',
query: {
id: row.id
}
})
},
//删除用户
userDelete(row) {
let _this = this
this.$confirm('此操作将永久删除该用户, 是否继续?', '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning',
center: true
}).then(() => {
axios.delete('http://localhost:8001/employ/' + row.id).then(function (response) {
if (response) {
_this.$alert('删除成功!', {
confirmButtonText: '确定',
callback: action => {
location.reload()
}
});
}
})
}).catch(() => {
this.$message({
type: 'info',
message: action === 'cancel'
? '放弃保存并离开页面'
: '停留在当前页面'
})
})
},
//分页查询
initEmps() {
const _this=this;
this.axios.post('http://localhost:8001/employ/pageCodition/'+this.current+'/'+this.size,this.employ).then(function (response) {
console.log(response)
_this.list = response.data.rows;
_this.total = response.data.total;
console.log(response.data)
})
}
}
}
</script>
<style>
.el-header {
background-color: #B3C0D1;
color: #333;
line-height: 60px;
}
.el-aside {
color: #42b983;
}
</style>
添加员工的界面:
<template>
<div style="width: 300px;margin-left: 400px">
<el-form :model="ruleForm" :rules="rules" ref="ruleForm" label-width="100px" class="demo-ruleForm">
<el-form-item label="编号" prop="id">
<el-input v-model="ruleForm.id" prefix-icon="el-icon-edit" placeholder="请输入员工编号"></el-input>
</el-form-item>
<el-form-item label="姓名" prop="name">
<el-input v-model="ruleForm.name" prefix-icon="el-icon-edit" placeholder="请输入员工姓名"></el-input>
</el-form-item>
<el-form-item label="性别" prop="sex">
<el-radio-group v-model="ruleForm.sex">
<el-radio label="男"></el-radio>
<el-radio label="女"></el-radio>
</el-radio-group>
</el-form-item>
<el-form-item label="籍贯" prop="provices">
<el-select v-model="ruleForm.provices" placeholder="请选择....">
<el-option label="北京" value="北京"></el-option>
<el-option label="天津" value="天津"></el-option>
<el-option label="上海" value="上海"></el-option>
<el-option label="甘肃" value="甘肃"></el-option>
<el-option label="深圳" value="深圳"></el-option>
<el-option label="广州" value="广州"></el-option>
</el-select>
</el-form-item>
<el-form-item label="年龄" prop="age">
<el-input v-model="ruleForm.age" prefix-icon="el-icon-edit" placeholder="请输入员工年龄"></el-input>
</el-form-item>
<el-form-item label="薪资" prop="salary">
<el-input v-model="ruleForm.salary" prefix-icon="el-icon-edit" placeholder="请输入员工薪资"></el-input>
</el-form-item>
<el-form-item label="地址" prop="address">
<el-input v-model="ruleForm.address" prefix-icon="el-icon-edit" placeholder="请输入员工地址"></el-input>
</el-form-item>
<el-form-item label="学校" prop="school">
<el-input v-model="ruleForm.school" prefix-icon="el-icon-edit" placeholder="请输入学校"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="submitForm('ruleForm')">立即添加</el-button>
<el-button @click="resetForm('ruleForm')">重置</el-button>
</el-form-item>
</el-form>
</div>
</template>
<script>
import axios from "axios";
export default {
name:'AddEmploy',
data() {
return {
ruleForm: {
id: '',
name: '',
sex: '',
provices: '',
age: '',
salary: '',
address: '',
school: ''
},
rules: {
name: [
{ required: true, message: '请输入员工姓名', trigger: 'blur' },
],
provices: [
{ required: true, message: '请选择籍贯', trigger: 'blur' }
],
age: [
{ required: true, message: '请输入年龄', trigger: 'blur' }
],
address: [
{ required: true, message: '请输入地址', trigger: 'blur' }
]
}
};
},
methods: {
submitForm(formName) {
const _this=this
this.$refs[formName].validate((valid) => {
if (valid) {
axios.post('http://localhost:8001/employ/add',this.ruleForm).then(function (response) {
if (response==true){
_this.$notify({
title: '成功',
message: '成功添加一条记录',
type: 'success'
});
_this.$router.push('/employ')
}
})
} else {
return false;
}
});
},
resetForm(formName) {
this.$refs[formName].resetFields();
}
}
}
</script>
角色界面:
角色需要和菜单权限做关联,菜单权限是树形结构:
<template>
<div>
<div>
<el-input style="width: 200px;margin-right: 20px" prefix-icon="el-icon-search" v-model.trim="role.zname" placeholder="请输入角色名称进行查询.."></el-input>
<el-button type="primary" icon="el-icon-search" @click="find">搜索</el-button>
<el-button type="primary" icon="el-icon-circle-plus" @click="dialogVisible = true">新增</el-button>
</div>
<el-table
:data="tableData"
border
style="width: 900px;margin-top: 15px">
<el-table-column
prop="id"
label="编号"
width="130">
</el-table-column>
<el-table-column
prop="zname"
label="名称"
width="130">
</el-table-column>
<el-table-column
prop="name"
label="唯一编码"
width="160">
</el-table-column>
<el-table-column
fixed="right"
label="操作"
width="350">
<template slot-scope="scope">
<div>
<el-button type="success" size="mini" @click="updateRole(scope.row)" icon="el-icon-edit-outline">分配权限</el-button>
<el-button type="primary" size="mini" @click="EditRole(scope.$index,scope.row)" icon=" el-icon-edit">编辑</el-button>
<el-button type="danger" size="mini" @click="deleteCustomer(scope.row)" icon="el-icon-delete-solid">删除</el-button>
</div>
</template>
</el-table-column>
</el-table>
<div style="display: flex;justify-content: flex-end;margin-top: 15px">
<!--添加角色信息的弹框-->
<el-dialog
title="添加角色"
:visible.sync="dialogVisible"
width="30%"
:before-close="handleClose">
<el-form :model="ruleForm" :rules="rules" ref="ruleForm" label-width="100px" class="demo-ruleForm">
<el-form-item label="角色编号" prop="id">
<el-input v-model="ruleForm.id"></el-input>
</el-form-item>
<el-form-item label="角色名称" prop="zname">
<el-input v-model="ruleForm.zname"></el-input>
</el-form-item>
<el-form-item label="唯一编码" prop="name">
<el-input v-model="ruleForm.name"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="submitForm('ruleForm')">立即添加</el-button>
<el-button @click="resetForm('ruleForm')">取消</el-button>
</el-form-item>
</el-form>
</el-dialog>
<!--修改角色信息的弹框-->
<el-dialog
title="修改角色信息"
:visible.sync="dialogVisible1"
width="30%"
:before-close="handleClose1" append-to-body>
<div>
<div>
<el-tag>角色id:</el-tag>
<el-input v-model="updatepos.id"prefix-icon="el-icon-edit" placeholder="请输入角色编号" style="width: 150px;margin-left: 10px;height: 20px;margin-top: 10px"></el-input>
</div>
<div>
<el-tag>角色名称:</el-tag>
<el-input v-model="updatepos.name"prefix-icon="el-icon-edit" placeholder="请输入角色名称" style="width: 150px;margin-left: 10px;height: 20px;margin-top: 10px"></el-input>
</div>
<div>
<el-tag>唯一编码:</el-tag>
<el-input v-model="updatepos.zname"prefix-icon="el-icon-edit" placeholder="请输入唯一编码" style="width: 150px;margin-left: 10px;height: 20px;margin-top: 10px"></el-input>
</div>
</div>
<span slot="footer" class="dialog-footer">
<el-button @click="dialogVisible1 = false">取消修改</el-button>
<el-button type="primary" @click="submitForm1">保存修改</el-button>
</span>
</el-dialog>
<!--分配权限的弹框-->
<el-dialog
title="分配权限"
:visible.sync="dialogVisible2"
width="30%"
:before-close="handleClose1" append-to-body>
<div>
<el-form :model="roleForm">
<el-tree
:data="roleData"
show-checkbox
:default-expand-all=true
:default-checked-keys="selectMenus"
node-key="id"
ref="roleTree"
:props="defaultProps">
</el-tree>
</el-form>
</div>
<div slot="footer" class="dialog-footer">
<el-button @click="dialogVisible2 = false">取消</el-button>
<el-button type="primary" @click="submitForm2('roleForm')">保存</el-button>
</div>
</el-dialog>
<el-pagination
background
layout='prev, pager, next, jumper, ->, total'
:page-size=6
:total="50">
</el-pagination>
</div>
</div>
</template>
<script>
export default {
name:'Customer',
data() {
return {
selectMenus:[],//选中菜单的数组
dialogVisible: false,
dialogVisible1:false,
dialogVisible2:false,
tableData:[
{}
],
roleForm:[],
role:{},
roleData:[],
updatepos:{//回显数据
id: '',
name: '',
zname:''
},
defaultProps: {
children: 'children',
label: 'name'
},
ruleForm: {
},
rules: {
id: [
{ required: true, message: '请输入角色编号', trigger: 'blur' },
],
name: [
{ required: true, message: '请输入唯一编码', trigger: 'blur' },
],
zname: [
{ required: true, message: '请输入角色名称', trigger: 'blur' },
],
}
};
},
mounted() {
this.initCustomer()
},
methods:{
//按名称查询用户
find(){
const _this=this;
this.axios.post('http://localhost:8001/customer/findByname/',this.role).then(function (response) {
console.log(response.obj.records)
_this.roleData = response.obj.records;
})
},
//显示所有角色
initCustomer(){
const _this=this
this.axios.get("http://localhost:8001/customer/list").then(function (response){
if (response){
_this.tableData=response
}
})
},
//删除角色
deleteCustomer(row) {
let _this = this
this.$confirm('此操作将永久删除该用户, 是否继续?', '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning',
center: true
}).then(() => {
this.axios.delete('http://localhost:8001/customer/delete/'+row.id).then(function (response) {
if (response) {
_this.initCustomer();
}
})
}).catch(() => {
this.$message({
type: 'info',
message: action === 'cancel'
? '放弃保存并离开页面'
: '停留在当前页面'
})
})
},
//修改回显数据
EditRole(index,data) {
Object.assign(this.updatepos,data);
this.dialogVisible1=true
},
//修改角色信息
submitForm1() {
const _this=this
this.axios.post('http://localhost:8001/customer/update',this.updatepos).then(function (response) {
if (response){
_this.dialogVisible1=false;
_this.initCustomer();
}
})
},
//分配权限
updateRole(row){
if(row.id){
this.initMenus();
this.initSelectMenus(row.id);
}
},
initSelectMenus(row){
this.dialogVisible2=true
const _this=this
this.axios.get('http://localhost:8001/customer/mid/'+row).then(function (response) {
if (response){
console.log(response)
_this.selectMenus=response
_this.roleForm=row
}
})
},
initMenus(){
const _this=this
this.axios.get("http://localhost:8001/customer/menus").then(function (response) {
if (response){
console.log(response)
_this.roleData=response
}
})
},
//更新权限
submitForm2(formName){
var menuIds=this.$refs.roleTree.getCheckedKeys()
console.log(menuIds)
console.log(this.roleForm)
this.axios.put('http://localhost:8001/customer/?'+"rid="+this.roleForm+"&mids="+menuIds).then(response=>{
if(response){
this.dialogVisible2=false
this.initCustomer();
}
})
},
//添加角色
submitForm(formName) {
const _this=this
this.$refs[formName].validate((valid) => {
if (valid) {
this.axios.post('http://localhost:8001/customer/add',this.ruleForm).then(function (response) {
if (response){
_this.dialogVisible=false
_this.initCustomer();
}
})
} else {
return false;
}
});
},
resetForm(formName) {
this.$refs[formName].resetFields();
this.dialogVisible=false
},
handleClose1(done) {
this.$confirm('确认关闭?')
.then(_ => {
done();
})
.catch(_ => {});
},
handleClose(done) {
this.$confirm('确认关闭?')
.then(_ => {
done();
})
.catch(_ => {});
}
}
};
</script>
用户界面: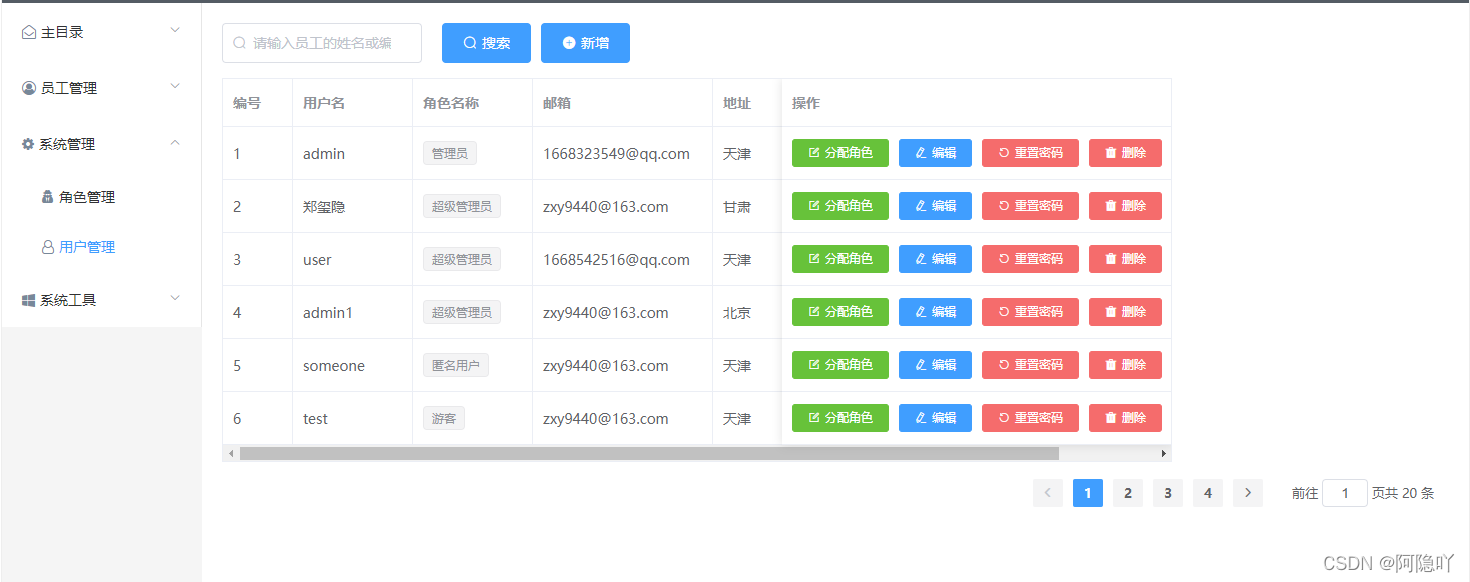
<template>
<div>
<div>
<el-input style="width: 200px;margin-right: 20px" prefix-icon="el-icon-search" v-model="searchForm.username" clearable placeholder="请输入员工的姓名或编号进行查询.."></el-input>
<el-button type="primary" icon="el-icon-search" @click="initAdmin">搜索</el-button>
<el-button type="primary" icon="el-icon-circle-plus" @click="dialogVisible = true">新增</el-button>
</div>
<el-table
:data="tableData"
border
style="width: 950px;margin-top: 15px">
<el-table-column
prop="id"
label="编号"
width="70">
</el-table-column>
<el-table-column
prop="username"
label="用户名"
width="120">
</el-table-column>
<el-table-column
prop="zname"
label="角色名称"
width="120">
<template slot-scope="scope">
<el-tag style="margin-right: 5px;" size="small" type="info" v-for="item in scope.row.roles">{{item.zname}}</el-tag>
</template>
</el-table-column>
<el-table-column
prop="email"
label="邮箱"
width="180">
</el-table-column>
<el-table-column
prop="address"
label="地址"
width="180">
</el-table-column>
<el-table-column
fixed="right"
label="操作"
width="390">
<template slot-scope="scope">
<div style="display: flex;justify-content: space-between" >
<el-button type="success" size="mini" @click="updateRole(scope.row)" icon="el-icon-edit-outline">分配角色</el-button>
<el-button type="primary" size="mini" @click="EditRole(scope.$index,scope.row)" icon=" el-icon-edit">编辑</el-button>
<el-button type="danger" size="mini" @click="repass(scope.row)" icon="el-icon-refresh-left">重置密码</el-button>
<el-button type="danger" size="mini" @click="deleteUser(scope.row)" icon="el-icon-delete-solid">删除</el-button>
</div>
</template>
</el-table-column>
</el-table>
<div style="display: flex;justify-content: flex-end;margin-top: 15px">
<!--添加用户信息的弹框-->
<el-dialog
title="添加用户"
:visible.sync="dialogVisible"
width="30%"
:before-close="handleClose">
<el-form :model="ruleForm" :rules="rules" ref="ruleForm" label-width="100px" class="demo-ruleForm">
<el-form-item label="编号" prop="id">
<el-input v-model="ruleForm.id"></el-input>
</el-form-item>
<el-form-item label="用户名" prop="username">
<el-input v-model="ruleForm.username"></el-input>
</el-form-item>
<el-form-item label="邮箱" prop="email">
<el-input v-model="ruleForm.email"></el-input>
</el-form-item>
<el-form-item label="地址" prop="address">
<el-input v-model="ruleForm.address"></el-input>
</el-form-item>
<el-form-item>
<el-button type="primary" @click="submitForm('ruleForm')">立即添加</el-button>
<el-button @click="resetForm('ruleForm')">取消</el-button>
</el-form-item>
</el-form>
</el-dialog>
<!--修改用户信息的弹框-->
<el-dialog
title="修改用户信息"
:visible.sync="dialogVisible1"
width="30%"
:before-close="handleClose1">
<div>
<div>
<el-tag>用户id:</el-tag>
<el-input v-model="updatepos.id"prefix-icon="el-icon-edit" placeholder="请输入用户编号" style="width: 150px;margin-left: 10px;height: 20px;margin-top: 10px"></el-input>
</div>
<div>
<el-tag>用户名称:</el-tag>
<el-input v-model="updatepos.username"prefix-icon="el-icon-edit" placeholder="请输入用户名称" style="width: 150px;margin-left: 10px;height: 20px;margin-top: 10px"></el-input>
</div>
<div>
<el-tag>邮箱:</el-tag>
<el-input v-model="updatepos.email"prefix-icon="el-icon-edit" placeholder="请输入邮箱" style="width: 150px;margin-left: 10px;height: 20px;margin-top: 10px"></el-input>
</div>
<div>
<el-tag>地址:</el-tag>
<el-input v-model="updatepos.address"prefix-icon="el-icon-edit" placeholder="请输入地址" style="width: 150px;margin-left: 10px;height: 20px;margin-top: 10px"></el-input>
</div>
</div>
<span slot="footer" class="dialog-footer">
<el-button @click="dialogVisible1 = false">取消修改</el-button>
<el-button type="primary" @click="submitForm1">保存修改</el-button>
</span>
</el-dialog>
<!--分配角色的弹框-->
<el-dialog
title="分配角色"
:visible.sync="dialogVisible2"
width="30%"
:before-close="handleClose1" append-to-body>
<div>
<el-form :model="roleForm">
<el-tree
:data="roleData"
show-checkbox
:default-checked-keys="selectRoles"
node-key="id"
ref="roleTree"
:props="defaultProps">
</el-tree>
</el-form>
</div>
<div slot="footer" class="dialog-footer">
<el-button @click="removerole('roleForm')">取消</el-button>
<el-button type="primary" @click="submitForm2('roleForm')">保存</el-button>
</div>
</el-dialog>
<el-pagination
background
layout='prev, pager, next, jumper, ->, total'
:page-size="5"
:total="20">
</el-pagination>
</div>
</div>
</template>
<script>
export default {
name:'People',
created() {
this.initAdmin();
const _this=this
this.axios.get("http://localhost:8001/customer/list").then(function (response) {
if (response){
console.log(response)
_this.roleData=response
}
})
},
data() {
return {
tableData:[
{}
],
searchForm:{},
updatepos:{//回显数据
id: '',
username: '',
email:'',
address:''
},
dialogVisible:false,
dialogVisible1:false,
dialogVisible2:false,
roleForm:{},
roleData:[],
selectRoles:[],//选中角色的数组
defaultProps: {
children: 'children',
label: 'zname'
},
ruleForm: {
},
rules: {
username: [
{ required: true, message: '请输入用户名', trigger: 'blur' },
],
email: [
{ required: true, message: '请输入邮箱', trigger: 'blur' },
],
address: [
{ required: true, message: '请输入地址', trigger: 'blur' },
],
},
}
},
methods: {
handleClick(row) {
console.log(row);
},
//显示所有的用户
initAdmin() {
const _this=this
this.axios.get('http://localhost:8001/people/list').then(function (response) {
if (response){
console.log(response)
_this.tableData=response.obj.records
}
})
},
handleClose(done) {
this.$confirm('确认关闭?')
.then(_ => {
done();
})
.catch(_ => {});
},
//添加用户
submitForm(formName) {
const _this=this
this.$refs[formName].validate((valid) => {
if (valid) {
this.axios.post('http://localhost:8001/people/add',this.ruleForm).then(function (response) {
if (response){
_this.dialogVisible=false
_this.initAdmin();
}
})
} else {
return false;
}
});
},
resetForm(formName) {
this.$refs[formName].resetFields();
this.dialogVisible=false
},
//删除用户
deleteUser(row) {
let _this = this
this.$confirm('此操作将永久删除该用户, 是否继续?', '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning',
center: true
}).then(() => {
this.axios.delete('http://localhost:8001/people/delete/'+row.id).then(function (response) {
if (response) {
_this.initAdmin();
}
})
}).catch(() => {
this.$message({
type: 'info',
message: action === 'cancel'
? '放弃保存并离开页面'
: '停留在当前页面'
})
})
},
//修改回显数据
EditRole(index,data) {
Object.assign(this.updatepos,data);
this.dialogVisible1=true
},
//修改角色信息
submitForm1() {
const _this=this
this.axios.post('http://localhost:8001/people/update',this.updatepos).then(function (response) {
if (response){
_this.dialogVisible1=false;
_this.initAdmin();
}
})
},
handleClose1(done) {
this.$confirm('确认关闭?')
.then(_ => {
done();
})
.catch(_ => {});
},
//分配角色
updateRole(row){
if(row.id){
this.dialogVisible2=true
const _this=this
this.axios.get('http://localhost:8001/people/rid/'+row.id).then(function (response) {
if (response){
console.log(response)
_this.selectRoles=response
_this.roleForm=row
}
})
}
},
//更新角色
submitForm2(formName){
const _this=this
var roleIds=this.$refs.roleTree.getCheckedKeys()
console.log(roleIds)
this.axios.put('http://localhost:8001/people/?'+"adminid="+this.roleForm.id+"&rolesIds="+roleIds).then(response=>{
if(response){
_this.dialogVisible2=false
_this.initAdmin();
}
})
},
removerole(formName){
this.selectRoles=null;
this.dialogVisible2=false;
},
//重置密码
repass(row){
this.$confirm('将重置用户【' + row.username + '】的密码, 是否继续?', '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: 'warning'
}).then(() => {
this.axios.post("http://localhost:8001/people/repass/"+row.id).then(res => {
console.log(row)
this.$message({
showClose:true,
message: '恭喜你,操作成功',
type: 'success',
onClose: () => {
}
});
})
})
},
},
}
</script>
<style scoped>
.box-card{
width: 350px;
}
</style>
文件管理接口:
<template>
<div>
<el-container style=" height: max-content;border: 1px solid #eee">
<el-container>
<el-main>
<div style="display: flex;justify-content: space-between;margin-top:5px">
<div>
<el-upload
class="upload-demo"
:multiple="true"
:on-success="success"
:on-error="error"
:show-file-list="false"
action="http://localhost:8001/employ/upload">
<el-button size="medium" type="primary" icon="el-icon-upload">上传文件</el-button>
<div slot="tip" class="el-upload__tip">只能上传jpg/png文件,且不超过500kb</div>
</el-upload>
</div>
</div>
<el-container style=" height: max-content;border: 1px solid #eee">
<el-container>
<el-main>
<el-table
:data="list"
style="width: 100%">
@selection-change="handleSelectionChange">
<el-table-column
type="selection"
width="55">
</el-table-column>
<el-table-column
label="文件id"
width="180">
<template slot-scope="scope">
<i class="el-icon-text"></i>
<span style="margin-left: 10px">{{ scope.row.id }}</span>
</template>
</el-table-column>
<el-table-column
label="文件名称"
width="180">
<template slot-scope="scope">
<i class="el-icon-text"></i>
<span style="margin-left: 10px">{{ scope.row.filename }}</span>
</template>
</el-table-column>
<el-table-column
label="文件地址"
width="180">
<template slot-scope="scope">
<i class="el-icon-text"></i>
<span style="margin-left: 10px">{{ scope.row.filepath }}</span>
</template>
</el-table-column>
<el-table-column label="操作">
<template slot-scope="scope">
<el-button
size="mini"
type="primary"
icon="el-icon-view"
style="margin-right: 10px"
@click="dialogVisible=true">预览</el-button>
<el-dialog
width="60%"
height="100%"
destroy-on-close
style="margin-top: -100px"
:close-on-click-modal="false"
:visible.sync="dialogVisible">
<div class="pdf">
<iframe :src="url" frameborder="0" style="width: 100%;height: 600px"></iframe>
</div>
<span slot="footer" class="dialog-footer"></span>
</el-dialog>
<el-button
size="mini"
type="primary"
icon="el-icon-download"
@click="download()">下载</el-button>
<el-button
size="mini"
type="danger"
icon="el-icon-delete"
@click="handleDelete(scope.$index, scope.row)">删除</el-button>
</template>
</el-table-column>
</el-table>
</el-main>
</el-container>
</el-container>
<div style="display: flex;justify-content: space-between;margin-top: 15px">
<div>
<el-upload
class="upload-demo"
drag
accept=".pdf"
:on-success="success"
:on-error="error"
:show-file-list="false"
action="http://localhost:8001/employ/upload/"
multiple>
<i class="el-icon-upload"></i>
<div class="el-upload__text">将文件拖到此处,或<em>点击上传</em></div>
<div class="el-upload__tip" slot="tip">只能上传pdf文件,且不超过100MB</div>
</el-upload>
</div>
</div>
</el-main>
</el-container>
</el-container>
</div>
</template>
<script>
import pdf from 'vue-pdf'
import download from 'downloadjs'
export default {
name:'Directory',
components:{
pdf
},
data() {
return {
src:'https://view.officeapps.live.com/op/embed.aspx?src=',
url:'http://localhost:8001/众安保险.pdf',
list:[],
dialogVisible:false,
}
},
mounted() {
this.src+=this.url
},
methods: {
success(response){
console.log(response.data)
if (response.successs="true"){
this.list.push(response.data)
this.$notify({
title: '成功',
message: '成功上传一份文件',
type: 'success'
});
}
},
error(){
this.$notify({
title: '失败',
message: '上传文件失败',
type: 'error'
});
},
download(){
download(this.url)
},
handleDelete(index){
this.list.splice(index,1);
},
handleSelectionChange(val){//val表示勾选的对象
this.multipleSelection=val;
console.log(val)
}
}
}
</script>
更多推荐
所有评论(0)