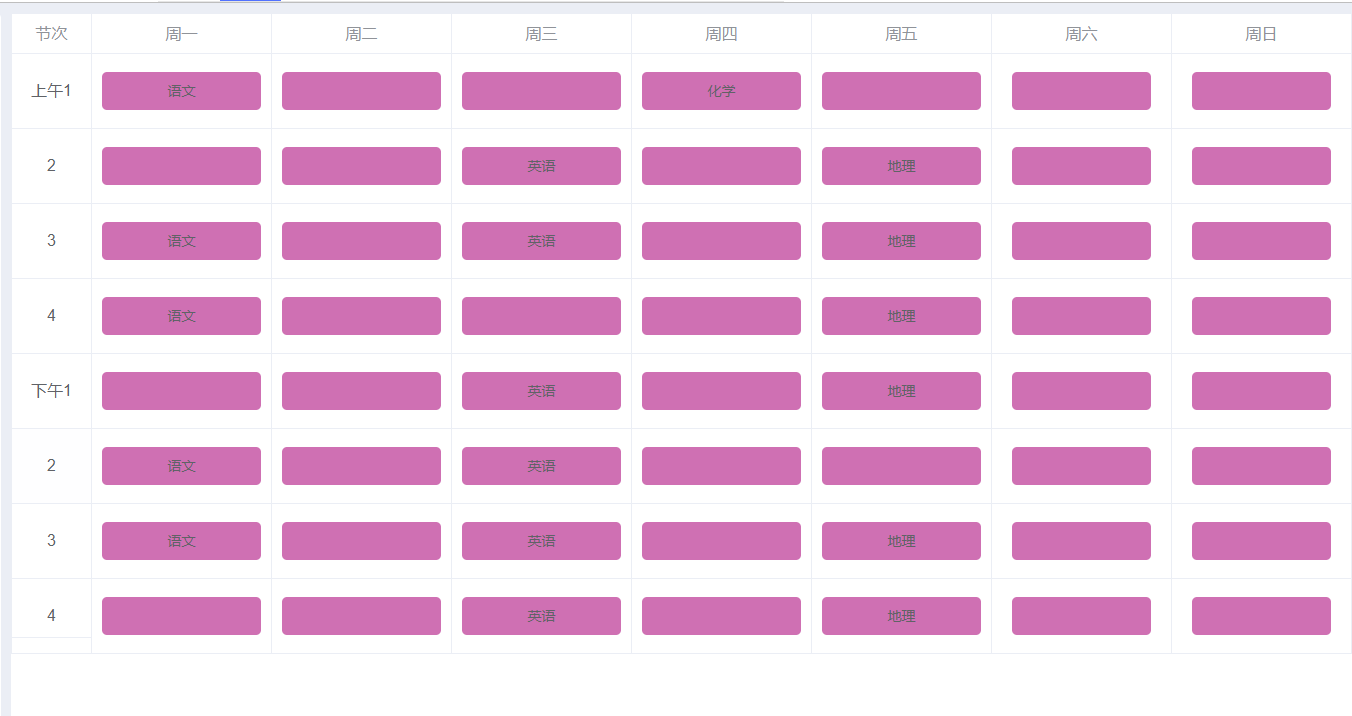
vue + el-table+draggable实现课表单元格拖拽
vue + el-table+draggable实现课表单元格拖拽<template><div class="schedule"><el-table :data="tableData" border style="width: 100%; font-size: 16px"><el-table-columnprop="index"label="节次"widt
·
vue + el-table+draggable实现课表单元格拖拽
<template>
<div class="schedule">
<el-table :data="tableData" border style="width: 100%; font-size: 16px">
<el-table-column
prop="index"
label="节次"
width="80"
align="center"
fixed
>
<template slot-scope="scope">
<p>{{ section(scope.row.index) }}</p>
</template>
</el-table-column>
<el-table-column prop="monday" label="周一" width="180" align="center">
<template slot-scope="scope">
<div
@drop="
drop($event, 'monday', scope.row.index, scope.row.monday.name)
"
@dragover="allowDrop"
>
<p
:draggable="scope.row.monday.name ? 'true' : 'false'"
class="contitem"
:style="{
background: '#cf70b3',
cursor: scope.row.monday.name ? 'move' : 'default',
}"
@dragstart="
drag($event, 'monday', scope.row.index, scope.row.monday.name)
"
>
{{ scope.row.monday.name }}
</p>
</div>
</template>
</el-table-column>
<el-table-column prop="tuesday" label="周二" width="180" align="center">
<template slot-scope="scope">
<div
@drop="
drop($event, 'tuesday', scope.row.index, scope.row.tuesday.name)
"
@dragover="allowDrop"
>
<p
:draggable="scope.row.tuesday.name ? 'true' : 'false'"
class="contitem"
:style="{
background: '#cf70b3',
}"
@dragstart="
drag($event, 'tuesday', scope.row.index, scope.row.tuesday.name)
"
>
{{ scope.row.tuesday.name }}
</p>
</div>
</template>
</el-table-column>
<el-table-column prop="wednesday" label="周三" width="180" align="center">
<template slot-scope="scope">
<div
@drop="
drop(
$event,
'wednesday',
scope.row.index,
scope.row.wednesday.name
)
"
@dragover="allowDrop"
>
<p
:draggable="scope.row.wednesday.name ? 'true' : 'false'"
class="contitem"
:style="{
background: '#cf70b3',
}"
@dragstart="
drag(
$event,
'wednesday',
scope.row.index,
scope.row.wednesday.name
)
"
>
{{ scope.row.wednesday.name }}
</p>
</div>
</template>
</el-table-column>
<el-table-column prop="thursday" label="周四" width="180" align="center">
<template slot-scope="scope">
<div
@drop="
drop($event, 'thursday', scope.row.index, scope.row.thursday.name)
"
@dragover="allowDrop"
>
<p
:draggable="scope.row.thursday.name ? 'true' : 'false'"
class="contitem"
:style="{
background: '#cf70b3',
}"
@dragstart="
drag(
$event,
'thursday',
scope.row.index,
scope.row.thursday.name
)
"
>
{{ scope.row.thursday.name }}
</p>
</div>
</template>
</el-table-column>
<el-table-column prop="friday" label="周五" width="180" align="center">
<template slot-scope="scope">
<div
@drop="
drop($event, 'friday', scope.row.index, scope.row.friday.name)
"
@dragover="allowDrop"
>
<p
:draggable="scope.row.friday.name ? 'true' : 'false'"
class="contitem"
:style="{
background: '#cf70b3',
}"
@dragstart="
drag($event, 'friday', scope.row.index, scope.row.friday.name)
"
>
{{ scope.row.friday.name }}
</p>
</div>
</template>
</el-table-column>
<el-table-column prop="saturday" label="周六" width="180" align="center">
<template slot-scope="scope">
<div
@drop="
drop($event, 'saturday', scope.row.index, scope.row.saturday.name)
"
@dragover="allowDrop"
style="padding: 10px"
>
<p
:draggable="scope.row.saturday.name ? 'true' : 'false'"
class="contitem"
:style="{
background: '#cf70b3',
cursor: scope.row.saturday.name ? 'move' : 'default',
}"
@dragstart="
drag(
$event,
'saturday',
scope.row.index,
scope.row.saturday.name
)
"
>
{{ scope.row.saturday.name }}
</p>
</div>
</template>
</el-table-column>
<el-table-column prop="sunday" label="周日" width="180" align="center">
<template slot-scope="scope">
<div
@drop="
drop($event, 'sunday', scope.row.index, scope.row.sunday.name)
"
@dragover="allowDrop"
style="padding: 10px"
>
<p
:draggable="scope.row.sunday.name ? 'true' : 'false'"
class="contitem"
:style="{
background: '#cf70b3',
}"
@dragstart="
drag($event, 'sunday', scope.row.index, scope.row.sunday.name)
"
>
{{ scope.row.sunday.name }}
</p>
</div>
</template>
</el-table-column>
</el-table>
</div>
</template>
<script>
export default {
name: "classSchedule",
components: {},
data() {
return {
type: 1,
tableData: [
{
index: "1",
monday: {
name: "语文",
},
tuesday: {
name: null,
},
wednesday: {
name: null,
},
thursday: {
name: "化学",
},
friday: {
name: null,
},
saturday: {
name: null,
},
sunday: {
name: null,
},
},
{
index: "2",
monday: {
name: null,
},
tuesday: {
name: null,
},
wednesday: {
name: "英语",
},
thursday: {
name: null,
},
friday: {
name: "地理",
},
saturday: {
name: null,
},
sunday: {
name: null,
},
},
{
index: "3",
monday: {
name: "语文",
},
tuesday: {
name: null,
},
wednesday: {
name: "英语",
},
thursday: {
name: null,
},
friday: {
name: "地理",
},
saturday: {
name: null,
},
sunday: {
name: null,
},
},
{
index: "4",
monday: {
name: "语文",
},
tuesday: {
name: null,
},
wednesday: {
name: null,
},
thursday: {
name: null,
},
friday: {
name: "地理",
},
saturday: {
name: null,
},
sunday: {
name: null,
},
},
{
index: "5",
monday: {
name: null,
},
tuesday: {
name: null,
},
wednesday: {
name: "英语",
},
thursday: {
name: null,
},
friday: {
name: "地理",
},
saturday: {
name: null,
},
sunday: {
name: null,
},
},
{
index: "6",
monday: {
name: "语文",
},
tuesday: {
name: null,
},
wednesday: {
name: "英语",
},
thursday: {
name: null,
},
friday: {
name: null,
},
saturday: {
name: null,
},
sunday: {
name: null,
},
},
{
index: "7",
monday: {
name: "语文",
},
tuesday: {
name: null,
},
wednesday: {
name: "英语",
},
thursday: {
name: null,
},
friday: {
name: "地理",
},
saturday: {
name: null,
},
sunday: {
name: null,
},
},
{
index: "8",
monday: {
name: null,
},
tuesday: {
name: null,
},
wednesday: {
name: "英语",
},
thursday: {
name: null,
},
friday: {
name: "地理",
},
saturday: {
name: null,
},
sunday: {
name: null,
},
},
],
activeItem: {
day: null,
index: null,
name: null,
},
};
},
computed: {},
watch: {},
created() {},
mounted() {},
methods: {
section(ind) {
switch (ind) {
case "1":
return "上午1";
break;
case "2":
return "2";
break;
case "3":
return "3";
break;
case "4":
return "4";
break;
case "5":
return "下午1";
break;
case "6":
return "2";
break;
case "7":
return "3";
break;
default:
return "4";
break;
}
},
drag(e, day, index, name) {
//开始
this.activeItem = { day: day, index: index, name: name };
},
allowDrop(e) {
e.preventDefault();
},
drop(e, day, index, name) {
e.preventDefault();
let newObj = {
day,
index,
name,
};
this.tableData.forEach((e, i) => {
if (i + 1 == index) {
e[day].name = this.activeItem.name;
}
if (this.activeItem.index == i + 1 && day) {
e[this.activeItem.day].name = newObj.name ? newObj.name : null;
}
});
},
},
beforeCreate() {},
beforeMount() {},
beforeUpdate() {},
updated() {},
beforeDestroy() {},
destroyed() {},
activated() {},
};
</script>
<style lang='scss' scoped>
//@import url(); 引入公共css类
.schedule {
height: calc(100% - 50px);
overflow: auto;
}
.option {
display: flex;
padding: 10px;
align-items: center;
justify-content: space-between;
width: 350px;
p {
}
p:nth-child(2) {
text-align: center;
width: 70px;
cursor: pointer;
color: #fff;
border-radius: 5px;
padding: 8px 10px;
background: #cf70b39d;
}
p:nth-child(3) {
text-align: center;
width: 70px;
cursor: pointer;
color: #fff;
border-radius: 5px;
padding: 8px 10px;
background: #93d59a;
}
p:nth-child(4) {
text-align: center;
width: 70px;
cursor: pointer;
color: #fff;
border-radius: 5px;
padding: 8px 10px;
background: #65acff;
}
.activeNum {
}
}
.contitem {
text-align: center;
height: 38px;
line-height: 38px;
border-radius: 5px;
font-size: 14px;
cursor: pointer;
}
>>> .el-table__body tr:hover > td,
>>> .el-table__body tr:hover > tr,
>>> .hover-row {
background-color: transparent !important;
}
>>> .el-table__row {
height: 55px;
}
.header {
display: flex;
justify-content: space-between;
align-items: center;
p {
width: 100px;
}
>>> .el-checkbox-group {
width: 110px;
}
}
</style>
更多推荐
所有评论(0)