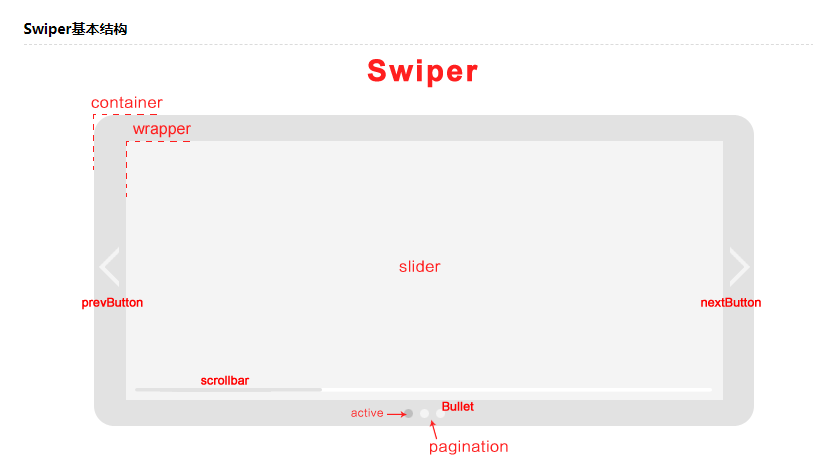
Vue2中使用Swiper3、4简单实用教程
一、安装Swiper使用Swiper的时候,一定要将Swiper版本和Vue版本对应上,否则将会报错本教程使用Vue2、VueCli脚手架和Swiper3作为demo首先使用npm install安装Swiper3npm install swiper vue-awesome-swiper@v2.6.7 --save附上Swiper中文API官网:https://www.swiper.com.cn/
·
一、安装Swiper
使用Swiper的时候,一定要将Swiper版本和Vue版本对应上,否则将会报错
本教程使用Vue2、VueCli脚手架和Swiper3作为demo
- 首先使用npm install安装Swiper3
npm install swiper vue-awesome-swiper@v2.6.7 --save
附上Swiper中文API官网:https://www.swiper.com.cn/
点击在Vue中使用低版本Swiper即可调转到Github页面,在README.md中有更多教程
二、注册Swiper
在main.js中进行全局注册
import Vue from 'vue'
import App from './App.vue'
import router from './router'
// 导入Swiper的依赖
import VueAwesomeSwiper from 'vue-awesome-swiper'
import 'swiper/swiper-bundle.css'
Vue.use(VueAwesomeSwiper)
//如果有多个组件需要use需要拆开写多个Vue.use(xxx)
三、定义Swiper组件
- 定义模板
<template>
<swiper ref="mySwiper" :options="swiperOptions">
<swiper-slide>Slide 1</swiper-slide>
<swiper-slide>Slide 2</swiper-slide>
<swiper-slide>Slide 3</swiper-slide>
<swiper-slide>Slide 4</swiper-slide>
<swiper-slide>Slide 5</swiper-slide>
<div class="swiper-pagination" slot="pagination"></div>
</swiper>
</template>
- 定义swiperOptions属性
<script>
export default {
name: 'carrousel',
data() {
return {
swiperOptions: {
pagination: {
el: '.swiper-pagination'
},
}
}
}
}
</script>
你将会得到一个这样的滑动框
四、综合DEMO
下面将以VueCli为基础搭建
- 在components中建立Swiper.vue(名字随便取)
<template>
<div class="wrapper">
<div class='part'>
<swiper class='swiper-containew' ref="mySwiper" :options="swiperOptions">
<swiper-slide v-for="item in swiperList" :key="item.id">
<img class="swiper-img" :src="baseUrl+item.img_url" alt="">
</swiper-slide>
<div class="swiper-pagination" slot="pagination"></div>
</swiper>
</div>
</div>
</template>
<script>
import axios from 'axios'
export default {
name: 'HomeSwiper',
data (){
return{
swiperOptions:{
// 需要调整Swiper组件的所有属性都在swiperOptions中定义即可
pagination:'.swiper-pagination',
// 循环不间断图片连在一起
loop:true,
// 循环时间(毫秒)
autoplay:2000,
// 展示多少张图片
slidesPerView:4,
// 图片间的间隔
spaceBetween :20
},
baseUrl: "http://127.0.0.1:9000/",
swiperList:[]
}
},
created(){
this.getswiperList()
},
methods:{
getswiperList(){
axios.get('http://127.0.0.1:9000/api/school_info/')
.then(res => {this.swiperList = res.data})
.catch(err => {console.log(err)})
}
}
}
</script>
<style>
.wrapper{
width: 100%;
height: auto;
display: block;
overflow: hidden;
}
.swiper-img{
width:100%;
}
.part{
width: 1000px;
height: auto;
display: block;
margin: 0 auto;
position: relative;
}
.swiper-containew{
margin-left: auto;
margin-right: auto;
position: relative;
overflow: hidden;
list-style: none;
padding: 0;
z-index: 1;
}
</style>
!!自己写一个接口给前端测试即可返回几张图片,或者你也可以用静态图片作为DEMO
- 在About.vue中定义如下代码
<template>
<div class="about">
<h1>This is an about page</h1>
<HomeSwiper></HomeSwiper>
</div>
</template>
<script>
import HomeSwiper from '@/components/Swiper'
export default {
name: 'About',
components: {
HomeSwiper
}
}
</script>
<style>
</style>
然后你就可以看到效果了
下面附上Swiper3API文档
https://3.swiper.com.cn/api/Slides_grid/2015/0722/283.html
箭头的属性放入swiperOptions中即可
建议新建VueCli初始项目再引入Swiper作为测试
更多推荐
所有评论(0)