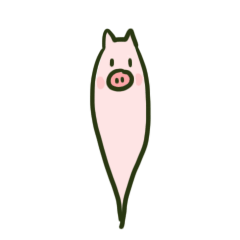
回归测试Python程序>_(Python编程 | 系统编程 | 完整的系统程序 | 一个回归测试脚本)
《Python编程》笔记专栏现以开源到Gitee,其中包含我编写的示例程序文件和官方示例程序文件,以及md形式的笔记,欢迎各位给个Star。文章目录一个回归测试脚本运行测试驱动一个回归测试脚本示例:my_PP4E/system/filetools/tester/tester.py#!/usr/bin/env python"""####################################
·
《Python编程》笔记专栏现以开源到Gitee,其中包含我编写的示例程序文件和官方示例程序文件,以及md形式的笔记,欢迎各位给个Star。
一个回归测试脚本
示例:my_PP4E/system/filetools/tester/tester.py
#!/usr/bin/env python
"""
##############################################################################
测试某一目录下的Python脚本,传入命令行参数,将stdin与管道相连,通过捕捉stdout、stderr和退
出状态来探测失败,以及获得前一次运行输出的回归记录。利用subprocess模块派生和控制流(类似Pyt
hon 2.X中的os.popen3),并可跨平台运行。流把它在subprocess中均为二进制字节。测试用到输入
、参数、输出、和错误均映射到子目录中的文件。
测试目录必须包含一个存放测试脚本的目录“Scripts”;如果有某个脚本需要获取命令行参数,则需在“A
rgs”目录下创建一个文件名为“除去.py后缀的原文件名 + .args”的文本文件;
如果需要输入,则需在“Inputs”目录下创建一个文件名为“除去.py后缀的原文件名 + .in”的文本文件;
“Errors”、“Outputs”等目录程序会自动创建。
这是一个命令行脚本,命令行参数均为可选,并不强制:第1个为测试的目录;若使用者输入参数“-f”或“
--forcegen”,则强制生成运行输出文件;若输入参数“-v”或“--verbose”,则显示详细运行情况。
##############################################################################
"""
import os
import sys
import time
import glob
from subprocess import Popen, PIPE
def verbose(*args):
print('-' * 79)
for arg in args:
print(arg)
def quiet(*args):
pass
# def getForcegen():
# if len(sys.argv) > 2:
# if sys.argv[2] == '1' or sys.argv[2] == '3':
# return True
# else:
# return False
# else:
# return False
# def getTrace():
# if len(sys.argv) > 2:
# if sys.argv[2] == '2' or sys.argv[2] == '3':
# return verbose
# else:
# return quiet
# else:
# return quiet
def pytester(
testdir=sys.argv[1] if len(sys.argv) > 1 else os.curdir,
forcegen='--forcegen' in sys.argv or '-f' in sys.argv,
trace=verbose if '--verbose' in sys.argv or '-v' in sys.argv else quiet
):
begintime = time.time()
print('开始测试:', time.asctime(), sep='')
print('在目录', os.path.abspath(testdir), '\n' * 3, sep='')
# 创建文件夹
errorsdirpath = os.path.join(testdir, 'Errors')
outputsdirpath = os.path.join(testdir, 'Outputs')
if not os.path.exists(errorsdirpath):
os.mkdir(errorsdirpath)
if not os.path.exists(outputsdirpath):
os.mkdir(outputsdirpath)
# 判断是否有“Args”、“Inputs”目录
argsdirpath = os.path.join(testdir, 'Args')
inputsdirpath = os.path.join(testdir, 'Inputs')
haveargsdir = os.path.exists(argsdirpath)
haveinputsdir = os.path.exists(inputsdirpath)
# 得到需要测试的脚本
testpatterns = os.path.join(testdir, 'Scripts', '*.py')
# print(testpatterns)
testfiles = glob.glob(testpatterns)
testfiles.sort()
# print(testfiles)
trace(os.getcwd, *testfiles)
numfail = 0
for testpath in testfiles:
testname = os.path.basename(testpath)
filename = os.path.splitext(testname)[0] # 去后缀
# 若有,则导入命令行参数
if haveargsdir:
# print(argsdirpath)
# print(filename + '.args')
argspath = os.path.join(argsdirpath, filename + '.args')
args = ''
if os.path.exists(argspath):
args = open(argspath, 'r').read().strip()
# 若有,则打开输入文件,以供后续连接管道
if haveinputsdir:
inputspath = os.path.join(inputsdirpath, filename + '.in')
inputs = b''
if os.path.exists(inputspath):
inputs = open(inputspath, 'rb').read()
# 设置输出文件路径,并删除之前错误的输出文件
outputspath = os.path.join(outputsdirpath, filename + '.out')
badoutputspath = outputspath + '.bad'
if os.path.exists(badoutputspath):
os.remove(badoutputspath)
# 设置保存错误的文件的路径,并删除之前保存错误的文件,打开文件
errorspath = os.path.join(errorsdirpath, filename + '.err')
if os.path.exists(errorspath):
os.remove(errorspath)
# 运行测试脚本,并重定向流
pypath = sys.executable
command = '{} {} {}'.format(pypath, testpath, args)
trace(command, inputs)
process = Popen(
command, shell=True, stdin=PIPE, stdout=PIPE, stderr=PIPE
)
process.stdin.write(inputs)
process.stdin.close()
outdata = process.stdout.read()
errdata = process.stderr.read()
exitcode = process.wait()
trace(outdata, errdata, exitcode)
# 分析测试结果
if exitcode:
print('程序返回值错误:', testname, exitcode, sep='')
if errdata:
open(errorspath, 'wb').write(errdata)
print('程序运行错误:', testname, errorspath, sep='')
if exitcode or errdata:
numfail += 1
open(badoutputspath, 'wb').write(outdata)
elif not os.path.exists(outputspath) or forcegen:
open(outputspath, 'wb').write(outdata)
print('运行结果生成:', testname, outputspath, sep='')
else:
prioroutdata = open(outputspath, 'rb').read()
if prioroutdata == outdata:
print('通过:', testname, outputspath)
else:
numfail += 1
open(badoutputspath, 'wb').write(outdata)
print('运行结果错误:', testname, badoutputspath)
finishtime = time.time()
print('-' * 79)
print('\n' * 3, '完成:', time.asctime(), sep='')
print('用时:', finishtime - begintime)
print('运行了{}个测试Python脚本,{}个测试失败。'.format(len(testfiles), numfail))
if __name__ == '__main__':
pytester()
运行测试驱动
测试文件在Gitee我已开源的笔记仓库中(传送门),位置是my_PP4E/system/filetools/tester/
。
运行:tester.py
$ ls
Args Inputs Scripts tester.py
$ ./tester.py
开始测试:Thu Aug 19 13:13:51 2021
在目录/media/beacherhou/Git仓库/python-programming---markdown-notes/my_PP4E/system/filetools/tester
运行结果生成:test-basic-args.py./Outputs/test-basic-args.out
运行结果生成:test-basic-stdout.py./Outputs/test-basic-stdout.out
运行结果生成:test-basic-streams.py./Outputs/test-basic-streams.out
运行结果生成:test-basic-this.py./Outputs/test-basic-this.out
程序返回值错误:test-errors-runtime.py1
程序运行错误:test-errors-runtime.py./Errors/test-errors-runtime.err
程序返回值错误:test-errors-syntax.py1
程序运行错误:test-errors-syntax.py./Errors/test-errors-syntax.err
程序返回值错误:test-status-bad.py42
运行结果生成:test-status-good.py./Outputs/test-status-good.out
-------------------------------------------------------------------------------
完成:Thu Aug 19 13:13:52 2021
用时: 0.21489739418029785
运行了8个测试Python脚本,3个测试失败。
$ ./tester.py . -v
开始测试:Thu Aug 19 13:14:07 2021
在目录/media/beacherhou/Git仓库/python-programming---markdown-notes/my_PP4E/system/filetools/tester
-------------------------------------------------------------------------------
<built-in function getcwd>
./Scripts/test-basic-args.py
./Scripts/test-basic-stdout.py
./Scripts/test-basic-streams.py
./Scripts/test-basic-this.py
./Scripts/test-errors-runtime.py
./Scripts/test-errors-syntax.py
./Scripts/test-status-bad.py
./Scripts/test-status-good.py
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-basic-args.py -command -line --stuff
b'Eggs\r\n10\r\n'
-------------------------------------------------------------------------------
b'/media/beacherhou/Git\xe4\xbb\x93\xe5\xba\x93/python-programming---markdown-notes/my_PP4E/system/filetools/tester\n/media/beacherhou/Git\xe4\xbb\x93\xe5\xba\x93/python-programming---markdown-notes/my_PP4E/system/filetools/tester/Scripts\n[argv]\n./Scripts/test-basic-args.py\n-command\n-line\n--stuff\n[interaction]\nEnter text:Eggs\rEggs\rEggs\rEggs\rEggs\rEggs\rEggs\rEggs\rEggs\rEggs\r'
b''
0
通过: test-basic-args.py ./Outputs/test-basic-args.out
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-basic-stdout.py
b''
-------------------------------------------------------------------------------
b'begin\nSpam!\nSpam!Spam!\nSpam!Spam!Spam!\nSpam!Spam!Spam!Spam!\nend\n'
b''
0
通过: test-basic-stdout.py ./Outputs/test-basic-stdout.out
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-basic-streams.py
b'aaa bbb ccc ddd\r\nSPAM!'
-------------------------------------------------------------------------------
b"['aaa', 'bbb', 'ccc', 'ddd']\nSPAM!SPAM!SPAM!SPAM!SPAM!SPAM!SPAM!SPAM!"
b''
0
通过: test-basic-streams.py ./Outputs/test-basic-streams.out
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-basic-this.py
b''
-------------------------------------------------------------------------------
b"The Zen of Python, by Tim Peters\n\nBeautiful is better than ugly.\nExplicit is better than implicit.\nSimple is better than complex.\nComplex is better than complicated.\nFlat is better than nested.\nSparse is better than dense.\nReadability counts.\nSpecial cases aren't special enough to break the rules.\nAlthough practicality beats purity.\nErrors should never pass silently.\nUnless explicitly silenced.\nIn the face of ambiguity, refuse the temptation to guess.\nThere should be one-- and preferably only one --obvious way to do it.\nAlthough that way may not be obvious at first unless you're Dutch.\nNow is better than never.\nAlthough never is often better than *right* now.\nIf the implementation is hard to explain, it's a bad idea.\nIf the implementation is easy to explain, it may be a good idea.\nNamespaces are one honking great idea -- let's do more of those!\n"
b''
0
通过: test-basic-this.py ./Outputs/test-basic-this.out
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-errors-runtime.py
b''
-------------------------------------------------------------------------------
b'starting\n'
b'Traceback (most recent call last):\n File "./Scripts/test-errors-runtime.py", line 3, in <module>\n print(1 / 0)\nZeroDivisionError: division by zero\n'
1
程序返回值错误:test-errors-runtime.py1
程序运行错误:test-errors-runtime.py./Errors/test-errors-runtime.err
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-errors-syntax.py
b''
-------------------------------------------------------------------------------
b''
b' File "./Scripts/test-errors-syntax.py", line 2\n print \'starting\'\n ^\nSyntaxError: Missing parentheses in call to \'print\'. Did you mean print(\'starting\')?\n'
1
程序返回值错误:test-errors-syntax.py1
程序运行错误:test-errors-syntax.py./Errors/test-errors-syntax.err
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-status-bad.py
b''
-------------------------------------------------------------------------------
b'starting\n'
b''
42
程序返回值错误:test-status-bad.py42
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-status-good.py 0
b''
-------------------------------------------------------------------------------
b'starting ./Scripts/test-status-good.py\n'
b''
0
通过: test-status-good.py ./Outputs/test-status-good.out
-------------------------------------------------------------------------------
完成:Thu Aug 19 13:14:07 2021
用时: 0.24712705612182617
运行了8个测试Python脚本,3个测试失败。
$ ./tester.py . --verbose
开始测试:Thu Aug 19 13:14:30 2021
在目录/media/beacherhou/Git仓库/python-programming---markdown-notes/my_PP4E/system/filetools/tester
-------------------------------------------------------------------------------
<built-in function getcwd>
./Scripts/test-basic-args.py
./Scripts/test-basic-stdout.py
./Scripts/test-basic-streams.py
./Scripts/test-basic-this.py
./Scripts/test-errors-runtime.py
./Scripts/test-errors-syntax.py
./Scripts/test-status-bad.py
./Scripts/test-status-good.py
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-basic-args.py -command -line --stuff
b'Eggs\r\n10\r\n'
-------------------------------------------------------------------------------
b'/media/beacherhou/Git\xe4\xbb\x93\xe5\xba\x93/python-programming---markdown-notes/my_PP4E/system/filetools/tester\n/media/beacherhou/Git\xe4\xbb\x93\xe5\xba\x93/python-programming---markdown-notes/my_PP4E/system/filetools/tester/Scripts\n[argv]\n./Scripts/test-basic-args.py\n-command\n-line\n--stuff\n[interaction]\nEnter text:Eggs\rEggs\rEggs\rEggs\rEggs\rEggs\rEggs\rEggs\rEggs\rEggs\r'
b''
0
通过: test-basic-args.py ./Outputs/test-basic-args.out
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-basic-stdout.py
b''
-------------------------------------------------------------------------------
b'begin\nSpam!\nSpam!Spam!\nSpam!Spam!Spam!\nSpam!Spam!Spam!Spam!\nend\n'
b''
0
通过: test-basic-stdout.py ./Outputs/test-basic-stdout.out
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-basic-streams.py
b'aaa bbb ccc ddd\r\nSPAM!'
-------------------------------------------------------------------------------
b"['aaa', 'bbb', 'ccc', 'ddd']\nSPAM!SPAM!SPAM!SPAM!SPAM!SPAM!SPAM!SPAM!"
b''
0
通过: test-basic-streams.py ./Outputs/test-basic-streams.out
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-basic-this.py
b''
-------------------------------------------------------------------------------
b"The Zen of Python, by Tim Peters\n\nBeautiful is better than ugly.\nExplicit is better than implicit.\nSimple is better than complex.\nComplex is better than complicated.\nFlat is better than nested.\nSparse is better than dense.\nReadability counts.\nSpecial cases aren't special enough to break the rules.\nAlthough practicality beats purity.\nErrors should never pass silently.\nUnless explicitly silenced.\nIn the face of ambiguity, refuse the temptation to guess.\nThere should be one-- and preferably only one --obvious way to do it.\nAlthough that way may not be obvious at first unless you're Dutch.\nNow is better than never.\nAlthough never is often better than *right* now.\nIf the implementation is hard to explain, it's a bad idea.\nIf the implementation is easy to explain, it may be a good idea.\nNamespaces are one honking great idea -- let's do more of those!\n"
b''
0
通过: test-basic-this.py ./Outputs/test-basic-this.out
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-errors-runtime.py
b''
-------------------------------------------------------------------------------
b'starting\n'
b'Traceback (most recent call last):\n File "./Scripts/test-errors-runtime.py", line 3, in <module>\n print(1 / 0)\nZeroDivisionError: division by zero\n'
1
程序返回值错误:test-errors-runtime.py1
程序运行错误:test-errors-runtime.py./Errors/test-errors-runtime.err
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-errors-syntax.py
b''
-------------------------------------------------------------------------------
b''
b' File "./Scripts/test-errors-syntax.py", line 2\n print \'starting\'\n ^\nSyntaxError: Missing parentheses in call to \'print\'. Did you mean print(\'starting\')?\n'
1
程序返回值错误:test-errors-syntax.py1
程序运行错误:test-errors-syntax.py./Errors/test-errors-syntax.err
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-status-bad.py
b''
-------------------------------------------------------------------------------
b'starting\n'
b''
42
程序返回值错误:test-status-bad.py42
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-status-good.py 0
b''
-------------------------------------------------------------------------------
b'starting ./Scripts/test-status-good.py\n'
b''
0
通过: test-status-good.py ./Outputs/test-status-good.out
-------------------------------------------------------------------------------
完成:Thu Aug 19 13:14:30 2021
用时: 0.23630595207214355
运行了8个测试Python脚本,3个测试失败。
$ ./tester.py . --verbose -f
开始测试:Thu Aug 19 13:14:39 2021
在目录/media/beacherhou/Git仓库/python-programming---markdown-notes/my_PP4E/system/filetools/tester
-------------------------------------------------------------------------------
<built-in function getcwd>
./Scripts/test-basic-args.py
./Scripts/test-basic-stdout.py
./Scripts/test-basic-streams.py
./Scripts/test-basic-this.py
./Scripts/test-errors-runtime.py
./Scripts/test-errors-syntax.py
./Scripts/test-status-bad.py
./Scripts/test-status-good.py
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-basic-args.py -command -line --stuff
b'Eggs\r\n10\r\n'
-------------------------------------------------------------------------------
b'/media/beacherhou/Git\xe4\xbb\x93\xe5\xba\x93/python-programming---markdown-notes/my_PP4E/system/filetools/tester\n/media/beacherhou/Git\xe4\xbb\x93\xe5\xba\x93/python-programming---markdown-notes/my_PP4E/system/filetools/tester/Scripts\n[argv]\n./Scripts/test-basic-args.py\n-command\n-line\n--stuff\n[interaction]\nEnter text:Eggs\rEggs\rEggs\rEggs\rEggs\rEggs\rEggs\rEggs\rEggs\rEggs\r'
b''
0
运行结果生成:test-basic-args.py./Outputs/test-basic-args.out
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-basic-stdout.py
b''
-------------------------------------------------------------------------------
b'begin\nSpam!\nSpam!Spam!\nSpam!Spam!Spam!\nSpam!Spam!Spam!Spam!\nend\n'
b''
0
运行结果生成:test-basic-stdout.py./Outputs/test-basic-stdout.out
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-basic-streams.py
b'aaa bbb ccc ddd\r\nSPAM!'
-------------------------------------------------------------------------------
b"['aaa', 'bbb', 'ccc', 'ddd']\nSPAM!SPAM!SPAM!SPAM!SPAM!SPAM!SPAM!SPAM!"
b''
0
运行结果生成:test-basic-streams.py./Outputs/test-basic-streams.out
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-basic-this.py
b''
-------------------------------------------------------------------------------
b"The Zen of Python, by Tim Peters\n\nBeautiful is better than ugly.\nExplicit is better than implicit.\nSimple is better than complex.\nComplex is better than complicated.\nFlat is better than nested.\nSparse is better than dense.\nReadability counts.\nSpecial cases aren't special enough to break the rules.\nAlthough practicality beats purity.\nErrors should never pass silently.\nUnless explicitly silenced.\nIn the face of ambiguity, refuse the temptation to guess.\nThere should be one-- and preferably only one --obvious way to do it.\nAlthough that way may not be obvious at first unless you're Dutch.\nNow is better than never.\nAlthough never is often better than *right* now.\nIf the implementation is hard to explain, it's a bad idea.\nIf the implementation is easy to explain, it may be a good idea.\nNamespaces are one honking great idea -- let's do more of those!\n"
b''
0
运行结果生成:test-basic-this.py./Outputs/test-basic-this.out
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-errors-runtime.py
b''
-------------------------------------------------------------------------------
b'starting\n'
b'Traceback (most recent call last):\n File "./Scripts/test-errors-runtime.py", line 3, in <module>\n print(1 / 0)\nZeroDivisionError: division by zero\n'
1
程序返回值错误:test-errors-runtime.py1
程序运行错误:test-errors-runtime.py./Errors/test-errors-runtime.err
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-errors-syntax.py
b''
-------------------------------------------------------------------------------
b''
b' File "./Scripts/test-errors-syntax.py", line 2\n print \'starting\'\n ^\nSyntaxError: Missing parentheses in call to \'print\'. Did you mean print(\'starting\')?\n'
1
程序返回值错误:test-errors-syntax.py1
程序运行错误:test-errors-syntax.py./Errors/test-errors-syntax.err
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-status-bad.py
b''
-------------------------------------------------------------------------------
b'starting\n'
b''
42
程序返回值错误:test-status-bad.py42
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-status-good.py 0
b''
-------------------------------------------------------------------------------
b'starting ./Scripts/test-status-good.py\n'
b''
0
运行结果生成:test-status-good.py./Outputs/test-status-good.out
-------------------------------------------------------------------------------
完成:Thu Aug 19 13:14:39 2021
用时: 0.22385382652282715
运行了8个测试Python脚本,3个测试失败。
$ ./tester.py . --verbose --forcegen
开始测试:Thu Aug 19 13:14:46 2021
在目录/media/beacherhou/Git仓库/python-programming---markdown-notes/my_PP4E/system/filetools/tester
-------------------------------------------------------------------------------
<built-in function getcwd>
./Scripts/test-basic-args.py
./Scripts/test-basic-stdout.py
./Scripts/test-basic-streams.py
./Scripts/test-basic-this.py
./Scripts/test-errors-runtime.py
./Scripts/test-errors-syntax.py
./Scripts/test-status-bad.py
./Scripts/test-status-good.py
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-basic-args.py -command -line --stuff
b'Eggs\r\n10\r\n'
-------------------------------------------------------------------------------
b'/media/beacherhou/Git\xe4\xbb\x93\xe5\xba\x93/python-programming---markdown-notes/my_PP4E/system/filetools/tester\n/media/beacherhou/Git\xe4\xbb\x93\xe5\xba\x93/python-programming---markdown-notes/my_PP4E/system/filetools/tester/Scripts\n[argv]\n./Scripts/test-basic-args.py\n-command\n-line\n--stuff\n[interaction]\nEnter text:Eggs\rEggs\rEggs\rEggs\rEggs\rEggs\rEggs\rEggs\rEggs\rEggs\r'
b''
0
运行结果生成:test-basic-args.py./Outputs/test-basic-args.out
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-basic-stdout.py
b''
-------------------------------------------------------------------------------
b'begin\nSpam!\nSpam!Spam!\nSpam!Spam!Spam!\nSpam!Spam!Spam!Spam!\nend\n'
b''
0
运行结果生成:test-basic-stdout.py./Outputs/test-basic-stdout.out
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-basic-streams.py
b'aaa bbb ccc ddd\r\nSPAM!'
-------------------------------------------------------------------------------
b"['aaa', 'bbb', 'ccc', 'ddd']\nSPAM!SPAM!SPAM!SPAM!SPAM!SPAM!SPAM!SPAM!"
b''
0
运行结果生成:test-basic-streams.py./Outputs/test-basic-streams.out
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-basic-this.py
b''
-------------------------------------------------------------------------------
b"The Zen of Python, by Tim Peters\n\nBeautiful is better than ugly.\nExplicit is better than implicit.\nSimple is better than complex.\nComplex is better than complicated.\nFlat is better than nested.\nSparse is better than dense.\nReadability counts.\nSpecial cases aren't special enough to break the rules.\nAlthough practicality beats purity.\nErrors should never pass silently.\nUnless explicitly silenced.\nIn the face of ambiguity, refuse the temptation to guess.\nThere should be one-- and preferably only one --obvious way to do it.\nAlthough that way may not be obvious at first unless you're Dutch.\nNow is better than never.\nAlthough never is often better than *right* now.\nIf the implementation is hard to explain, it's a bad idea.\nIf the implementation is easy to explain, it may be a good idea.\nNamespaces are one honking great idea -- let's do more of those!\n"
b''
0
运行结果生成:test-basic-this.py./Outputs/test-basic-this.out
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-errors-runtime.py
b''
-------------------------------------------------------------------------------
b'starting\n'
b'Traceback (most recent call last):\n File "./Scripts/test-errors-runtime.py", line 3, in <module>\n print(1 / 0)\nZeroDivisionError: division by zero\n'
1
程序返回值错误:test-errors-runtime.py1
程序运行错误:test-errors-runtime.py./Errors/test-errors-runtime.err
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-errors-syntax.py
b''
-------------------------------------------------------------------------------
b''
b' File "./Scripts/test-errors-syntax.py", line 2\n print \'starting\'\n ^\nSyntaxError: Missing parentheses in call to \'print\'. Did you mean print(\'starting\')?\n'
1
程序返回值错误:test-errors-syntax.py1
程序运行错误:test-errors-syntax.py./Errors/test-errors-syntax.err
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-status-bad.py
b''
-------------------------------------------------------------------------------
b'starting\n'
b''
42
程序返回值错误:test-status-bad.py42
-------------------------------------------------------------------------------
/home/alone/anaconda3/bin/python ./Scripts/test-status-good.py 0
b''
-------------------------------------------------------------------------------
b'starting ./Scripts/test-status-good.py\n'
b''
0
运行结果生成:test-status-good.py./Outputs/test-status-good.out
-------------------------------------------------------------------------------
完成:Thu Aug 19 13:14:46 2021
用时: 0.24061012268066406
运行了8个测试Python脚本,3个测试失败。
$ ls
Args Errors Inputs Outputs Scripts tester.py
$ tree
.
├── Args
│ ├── test-basic-args.args
│ └── test-status-good.args
├── Errors
│ ├── test-errors-runtime.err
│ └── test-errors-syntax.err
├── Inputs
│ ├── test-basic-args.in
│ └── test-basic-streams.in
├── Outputs
│ ├── test-basic-args.out
│ ├── test-basic-stdout.out
│ ├── test-basic-streams.out
│ ├── test-basic-this.out
│ ├── test-errors-runtime.out.bad
│ ├── test-errors-syntax.out.bad
│ ├── test-status-bad.out.bad
│ └── test-status-good.out
├── Scripts
│ ├── test-basic-args.py
│ ├── test-basic-stdout.py
│ ├── test-basic-streams.py
│ ├── test-basic-this.py
│ ├── test-errors-runtime.py
│ ├── test-errors-syntax.py
│ ├── test-status-bad.py
│ └── test-status-good.py
└── tester.py
5 directories, 23 files
———————————————————————————————————————————
😃 学完博客后,是不是有所启发呢?如果对此还有疑问,欢迎在评论区留言哦。
如果还想了解更多的信息,欢迎大佬们关注我哦,也可以查看我的个人博客网站BeacherHou。
更多推荐
所有评论(0)