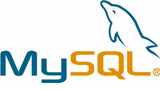
Linux系统——Mysql数据库操作
查看数据库/表和表结构、创建数据库/表和删除数据库/表、数据表的增删改查等操作、数据库表的清空表/临时表和克隆表等操作、数据库的用户授权相关操作(数据库用户管理、数据库授权)
目录
1.3显示数据表的结构(字段)——Describe(Desc)
一、数据库基本操作
1.查看数据库结构
1.1查看数据库信息——Show databases
1.2查看数据库中的表信息——Show tables
Show tables in 数据库名
use 数据库名 show tables
1.3显示数据表的结构(字段)——Describe(Desc)
详解
字段 | 含义 |
---|---|
Field | 字段名称 |
Type | 数据类型 |
Null | 是否为空 |
Key | 主键 |
Default | 默认值 |
Extra | 扩展属性 |
key主键是唯一的,但是主键可以由多个字段构成
1.4常用的数据类型
数据类型 | 含义 |
---|---|
int | 整形,用于定义整数类型的数据 |
float | 单精度浮点4字节32位,准确表示到小数点后六位 |
double | 双精度浮点8字节64位 |
char | 固定长度的字符类型 |
varchar | 可变长度的字符类型 |
text | 文本 |
image | 图片 |
decimal | 指定长度数组,decimal(5,2)表示5个有效长度数字,小数点后有两位数字 |
char如果存入数据的实际长度要比指定长度小,会补空格至指定长度,如果存入的数据的实际长度大于指定长度,低版本会被截取,高版本会报错;
1.5扩展
Mysql数据库的数据文件存放在/usr/local/mysql/data目录下,每个数据库对应一个子目录,用于存储数据表文件。如果数据表对应为三个文件,扩展名分别为".frm"、".MYD"、".MYI"
MYD”文件是MyISAM存储引擎专用,存放MyISAM表的数据。每一个MyISAM表都会有一个“.MYD”文件与之对应,同样存放于所属数据库的文件夹下,和“.frm”文件在一起。
“.MYI”文件也是专属于 MyISAM 存储引擎的,主要存放 MyISAM 表的索引相关信息。对于 MyISAM 存储来说,可以被 cache 的内容主要就是来源于“.MYI”文件中。每一个MyISAM 表对应一个“.MYI”文件,存放于位置和“.frm”以及“.MYD”一样。
MyISAM 存储引擎的表在数据库中,每一个表都被存放为三个以表名命名的物理文件
(frm,myd,myi)。 每个表都有且仅有这样三个文件做为 MyISAM 存储类型的表的存储,也就是说不管这个表有多少个索引,都是存放在同一个.MYI 文件中。
另外还有“.ibd”和 ibdata 文件,这两种文件都是用来存放 Innodb 数据的,之所以有两种文件来存放 Innodb 的数据(包括索引),是因为Innodb的数据存储方式能够通过配置来决定是使用共享表空间存放存储数据,还是独享表空间存放存储数据。独享表空间存储 方式使用“.ibd”文件来存放数据,且每个表一个“.ibd”文件,文件存放在和 MyISAM 数据相同的位置。如果选用共享存储表空间来存放数据,则会使用 ibdata 文件来存放,所有表共同使用一个(或者多个,可自行配置)ibdata 文件。
二、SQL语句
1.SQL语句概述
SQL(Structured Query Language)结构化查询语言,是关系型数据库的标准语言,用于维护管理数据库,其中包括数据查询、数据更新、访问控制、对象管理等功能
2.SQL分类
- DDL:数据定义语言
- DML:数据操纵语言
- DQL:数据查询语言
- DCL:数据控制语言
3.DDL语句——创建数据库和表(数据定义语言)
DDL语句可用于创建数据库对象,如库、表、索引等
3.1创建数据库——Create databases
mysql> show databases;
+--------------------+
| Database |
+--------------------+
| information_schema |
| mysql |
| performance_schema |
| sys |
+--------------------+
4 rows in set (0.00 sec)
mysql> create database class;
Query OK, 1 row affected (0.00 sec)
mysql> show databases;
+--------------------+
| Database |
+--------------------+
| information_schema |
| class |
| mysql |
| performance_schema |
| sys |
+--------------------+
5 rows in set (0.00 sec)
3.2创建数据表——Create table
CREATE TABLE 表名 (字段1 数据类型,字段2 数据类型[,...][,PRIMARY KEY (主键名)]);
#主键一般选择能代表唯一性的字段不允许取空值(NULL),一个表只能有一个主键。
mysql> create table class (id int not null,name char(15) not null,grate decimall(4,2),passwd char(45) default'',primary key (id));
#创建一个名为class的数据表 id取整型不为空;name字段字节数为15字节不为空;grate字段整数位最大为4位,小数位为2位;密码字段字节数为45字节;default字段默认值为空;primary key主键定义id为唯一的,也就是说id不可以重复
Query OK, 0 rows affected (0.07 sec)
mysql> show tables;
+-----------------+
| Tables_in_class |
+-----------------+
| class |
+-----------------+
1 row in set (0.00 sec)
mysql> create table class2 (id int not null,name char(15) not null,grate decimaal(4,2),passwd char(45) default'',primary key (id));
Query OK, 0 rows affected (0.01 sec)
mysql> show tables;
+-----------------+
| Tables_in_class |
+-----------------+
| class |
| class2 |
+-----------------+
2 rows in set (0.00 sec)
mysql> describe class;
#查询所建数据表定义的字段类型
+--------+--------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+--------+--------------+------+-----+---------+-------+
| id | int(11) | NO | PRI | NULL | |
| name | char(15) | NO | | NULL | |
| grate | decimal(4,2) | YES | | NULL | |
| passwd | char(45) | YES | | | |
+--------+--------------+------+-----+---------+-------+
4 rows in set (0.00 sec)
mysql> desc class2;
#可以简写为desc
+--------+--------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+--------+--------------+------+-----+---------+-------+
| id | int(11) | NO | PRI | NULL | |
| name | char(15) | NO | | NULL | |
| grate | decimal(4,2) | YES | | NULL | |
| passwd | char(45) | YES | | | |
+--------+--------------+------+-----+---------+-------+
4 rows in set (0.00 sec)
3.3删除数据库——Drop database
mysql> show databases;
+--------------------+
| Database |
+--------------------+
| information_schema |
| class |
| mysql |
| performance_schema |
| sys |
+--------------------+
5 rows in set (0.00 sec)
mysql> drop database class;
#删除指定数据库
Query OK, 1 row affected (0.00 sec)
mysql> show databases;
+--------------------+
| Database |
+--------------------+
| information_schema |
| mysql |
| performance_schema |
| sys |
+--------------------+
4 rows in set (0.00 sec)
3.4删除数据表——Drop table
DROP TABLE [数据库名.] 表名
mysql> show tables;
+-----------------+
| Tables_in_class |
+-----------------+
| class |
| class2 |
+-----------------+
2 rows in set (0.00 sec)
mysql> drop table class;
#删除指定数据表
Query OK, 0 rows affected (0.00 sec)
mysql> show tables;
+-----------------+
| Tables_in_class |
+-----------------+
| class2 |
+-----------------+
1 row in set (0.00 sec)
4.DML——管理表中的数据(数据操纵语言)
DML语句用于对表中的数据进行管理,用来插入、删除和修改数据库中的数据
- Insert:插入新数据
- Update:更新原有数据
- Delete:删除不需要的数据
4.1插入数据——Insert
INSERT INTO 表名(字段1,字段2[,...]) VALUES (字段1的值,字段2的值,...)
mysql> show tables;
+-----------------+
| Tables_in_class |
+-----------------+
| class |
+-----------------+
1 row in set (0.00 sec)
mysql> desc class;
+--------+--------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+--------+--------------+------+-----+---------+-------+
| id | int(11) | NO | PRI | NULL | |
| name | char(15) | NO | | NULL | |
| grate | decimal(4,2) | YES | | NULL | |
| passwd | char(45) | YES | | | |
+--------+--------------+------+-----+---------+-------+
4 rows in set (0.00 sec)
mysql> insert into class (id,name,grate,passwd) values(1,'cxk',88,PASSWORD('1233123'));
Query OK, 1 row affected, 1 warning (0.00 sec)
#插入数据到class数据表中,对应id为1,name为cxk,grate为88,passwd使用PASSWORD密文密码设置为123123 若不使用PASSWORD(),查询时以明文显示
mysql> select * from class;
+----+------+-------+-------------------------------------------+
| id | name | grate | passwd |
+----+------+-------+-------------------------------------------+
| 1 | cxk | 88.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
+----+------+-------+-------------------------------------------+
1 row in set (0.00 sec)
#此时看到的cxk客户的相关信息 passwor定义后的密码是加密后的
字段就是属性,值就是信息
mysql> insert into class values(2,'wyb',96,123321);
Query OK, 1 row affected (0.00 sec)
mysql> select * from class;
+----+------+-------+-------------------------------------------+
| id | name | grate | passwd |
+----+------+-------+-------------------------------------------+
| 1 | cxk | 88.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
| 2 | wyb | 96.00 | 123321 |
+----+------+-------+-------------------------------------------+
2 rows in set (0.00 sec)
#此时看到的新建用户wyb的相关信息 密码显示为明文的
4.2修改、更新数据表中的数据记录——Update
mysql> select * from class;
+----+------+-------+-------------------------------------------+
| id | name | grate | passwd |
+----+------+-------+-------------------------------------------+
| 1 | cxk | 88.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
| 2 | wyb | 96.00 | 123321 |
+----+------+-------+-------------------------------------------+
2 rows in set (0.00 sec)
mysql> update class set grate ='92';
#如若不指定条件 那么修改更新的数据信息就是数据表中所有信息
#将数据表class中所有grate字段都修改为92
Query OK, 2 rows affected (0.00 sec)
Rows matched: 2 Changed: 2 Warnings: 0
mysql> select * from class;
+----+------+-------+-------------------------------------------+
| id | name | grate | passwd |
+----+------+-------+-------------------------------------------+
| 1 | cxk | 92.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
| 2 | wyb | 92.00 | 123321 |
+----+------+-------+-------------------------------------------+
2 rows in set (0.00 sec)
mysql> update class set grate ='96' where id=2;
#where代表条件判断 更新class数据表中的grate字段 id为2的用户信息的grate字段修改为96
Query OK, 1 row affected (0.00 sec)
Rows matched: 1 Changed: 1 Warnings: 0
mysql> select * from class;
+----+------+-------+-------------------------------------------+
| id | name | grate | passwd |
+----+------+-------+-------------------------------------------+
| 1 | cxk | 92.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
| 2 | wyb | 96.00 | 123321 |
+----+------+-------+-------------------------------------------+
2 rows in set (0.00 sec)
4.3删除数据——Delete
mysql> select * from class;
+----+------+-------+-------------------------------------------+
| id | name | grate | passwd |
+----+------+-------+-------------------------------------------+
| 1 | cxk | 92.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
| 2 | wyb | 96.00 | 123321 |
+----+------+-------+-------------------------------------------+
2 rows in set (0.00 sec)
mysql> delete from class where name='cxk';
#删除特定条件的数据信息 删除class数据表中 条件为name=cxk的用户信息数据
Query OK, 1 row affected (0.00 sec)
mysql> select * from class;
+----+------+-------+--------+
| id | name | grate | passwd |
+----+------+-------+--------+
| 2 | wyb | 96.00 | 123321 |
+----+------+-------+--------+
1 row in set (0.00 sec)
mysql> insert into class values(1,'cxk',88,PASSWORD('123123'));
Query OK, 1 row affected, 1 warning (0.00 sec)
mysql> select * from class;
+----+------+-------+-------------------------------------------+
| id | name | grate | passwd |
+----+------+-------+-------------------------------------------+
| 1 | cxk | 88.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
| 2 | wyb | 96.00 | 123321 |
+----+------+-------+-------------------------------------------+
2 rows in set (0.00 sec)
mysql> delete from class;
#删除class数据表
Query OK, 2 rows affected (0.00 sec)
mysql> select * from class;
Empty set (0.00 sec)
mysql> insert into class values(1,'cxk',88,PASSWORD('123123'));
#使用delete命令行 删除该数据表还是可以重新写入新的数据
Query OK, 1 row affected, 1 warning (0.00 sec)
mysql> select * from class;
+----+------+-------+-------------------------------------------+
| id | name | grate | passwd |
+----+------+-------+-------------------------------------------+
| 1 | cxk | 88.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
+----+------+-------+-------------------------------------------+
1 row in set (0.00 sec)
5.DQL——管理表中的数据(数据查询语言)
DQL是数据查询语句,只有Select命令行,用于从数据表中查找符合条件的数据记录(查询时可以不指定条件)
5.1Select * ——查询所有
Select 字段1,字段2,......from 表名
mysql> select * from class;
#查询class数据表中所有数据
+----+------+-------+-------------------------------------------+
| id | name | grate | passwd |
+----+------+-------+-------------------------------------------+
| 1 | cxk | 88.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
+----+------+-------+-------------------------------------------+
1 row in set (0.00 sec)
mysql> select id,name from class;
#查询class数据表中id和name字段数据
+----+------+
| id | name |
+----+------+
| 1 | cxk |
+----+------+
1 row in set (0.00 sec)
mysql> select id,name,grate from class;
#查询class数据表中id、name和grate字段数据
+----+------+-------+
| id | name | grate |
+----+------+-------+
| 1 | cxk | 88.00 |
+----+------+-------+
1 row in set (0.00 sec)
5.2Where——特定条件查找
Select 字段1,字段2......from 表名 Where 条件表达式
mysql> insert into class values(2,'wyb',96,PASSWORD('123321'))
-> ;
Query OK, 1 row affected, 1 warning (0.00 sec)
mysql> select * from class;
+----+------+-------+-------------------------------------------+
| id | name | grate | passwd |
+----+------+-------+-------------------------------------------+
| 1 | cxk | 88.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
| 2 | wyb | 96.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
+----+------+-------+-------------------------------------------+
2 rows in set (0.00 sec)
mysql> select name,id,grate from class where id=2;
+------+----+-------+
| name | id | grate |
+------+----+-------+
| wyb | 2 | 96.00 |
+------+----+-------+
1 row in set (0.00 sec)
mysql> select name,id,grate from class where name='wyb';
+------+----+-------+
| name | id | grate |
+------+----+-------+
| wyb | 2 | 96.00 |
+------+----+-------+
1 row in set (0.00 sec)
5.3limit——按行数查询
按行数查询
mysql> insert into class values(3,'xzq',95,PASSWORD('123321'));
Query OK, 1 row affected, 1 warning (0.00 sec)
mysql> insert into class values(4,'lyf',95,PASSWORD('123321'));
Query OK, 1 row affected, 1 warning (0.00 sec)
mysql> select * from class;
+----+------+-------+-------------------------------------------+
| id | name | grate | passwd |
+----+------+-------+-------------------------------------------+
| 1 | cxk | 88.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
| 2 | wyb | 96.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
| 3 | xzq | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
| 4 | lyf | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
+----+------+-------+-------------------------------------------+
4 rows in set (0.00 sec)
mysql> select id,name from class limit 2;
+----+------+
| id | name |
+----+------+
| 1 | cxk |
| 2 | wyb |
+----+------+
2 rows in set (0.00 sec)
mysql> select * from class;
+----+------+-------+-------------------------------------------+
| id | name | grate | passwd |
+----+------+-------+-------------------------------------------+
| 1 | cxk | 88.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
| 2 | wyb | 96.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
| 3 | xzq | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
| 4 | lyf | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
+----+------+-------+-------------------------------------------+
4 rows in set (0.00 sec)
mysql> select id,name from class limit 2,3;
#显示第2行的后3行,也就是从第三行开始 显示三行
+----+------+
| id | name |
+----+------+
| 3 | xzq |
| 4 | lyf |
+----+------+
2 rows in set (0.00 sec)
5.4 \G——以竖向方向显示
mysql> select * from class;
+----+------+-------+-------------------------------------------+
| id | name | grate | passwd |
+----+------+-------+-------------------------------------------+
| 1 | cxk | 88.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
| 2 | wyb | 96.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
| 3 | xzq | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
| 4 | lyf | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
+----+------+-------+-------------------------------------------+
4 rows in set (0.00 sec)
mysql> select * from class\G;
*************************** 1. row ***************************
id: 1
name: cxk
grate: 88.00
passwd: *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1
*************************** 2. row ***************************
id: 2
name: wyb
grate: 96.00
passwd: *437F1809645E0A92DAB553503D2FE21DB91270FD
*************************** 3. row ***************************
id: 3
name: xzq
grate: 95.00
passwd: *437F1809645E0A92DAB553503D2FE21DB91270FD
*************************** 4. row ***************************
id: 4
name: lyf
grate: 95.00
passwd: *437F1809645E0A92DAB553503D2FE21DB91270FD
4 rows in set (0.00 sec)
ERROR:
No query specified
6.DCL——数据库用户授权(数据控制语言)
DCL语句设置用户权限(用户不存在时,则新建用户)
Grant 权限列表 ON 数据库名.表名 to 用户名@来源地址 [ Identified by '密码' ]
Alter 修改字段、数据表
6.1rename——更改数据表名称
mysql> show tables;
+-----------------+
| Tables_in_class |
+-----------------+
| class |
| class2 |
+-----------------+
2 rows in set (0.00 sec)
mysql> alter table class2 rename class3;
#修改class数据表的名字变更为class3
Query OK, 0 rows affected (0.03 sec)
mysql> show tables;
+-----------------+
| Tables_in_class |
+-----------------+
| class |
| class3 |
+-----------------+
2 rows in set (0.00 sec)
6.2add——扩展表结构字段
mysql> alter table class3 add Phone varchar(11) default '13100113399';
#修改数据表内容 新增Phone的数据列 字段字节长度为11位 默认值为13100113399
Query OK, 0 rows affected (0.08 sec)
Records: 0 Duplicates: 0 Warnings: 0
mysql> select * from class3;
+----+------+-------+-------------------------------------------+-------------+
| id | name | grate | passwd | Phone |
+----+------+-------+-------------------------------------------+-------------+
| 1 | cxk | 88.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 | 13100113399 |
| 2 | wyb | 96.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD | 13100113399 |
| 3 | xzq | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD | 13100113399 |
| 4 | lyf | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD | 13100113399 |
+----+------+-------+-------------------------------------------+-------------+
4 rows in set (0.00 sec)
6.3Change——更改数据字段内容
mysql> alter table class3 change id num varchar(15) unique key;
Query OK, 4 rows affected (0.01 sec)
Records: 4 Duplicates: 0 Warnings: 0
mysql> select * from class3;
+-----+------+-------+-------------------------------------------+-------------+
| num | name | grate | passwd | Phone |
+-----+------+-------+-------------------------------------------+-------------+
| 1 | cxk | 88.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 | 13100113399 |
| 2 | wyb | 96.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD | 13100113399 |
| 3 | xzq | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD | 13100113399 |
| 4 | lyf | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD | 13100113399 |
+-----+------+-------+-------------------------------------------+-------------+
4 rows in set (0.00 sec)
6.4Drop——删除指定数据字段内容
mysql> alter table class3 drop Phone;
Query OK, 0 rows affected (0.06 sec)
Records: 0 Duplicates: 0 Warnings: 0
mysql> select * from class3;
+-----+------+-------+-------------------------------------------+
| num | name | grate | passwd |
+-----+------+-------+-------------------------------------------+
| 1 | cxk | 88.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
| 2 | wyb | 96.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
| 3 | xzq | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
| 4 | lyf | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
+-----+------+-------+-------------------------------------------+
4 rows in set (0.00 sec)
三、高级操作
1.清空表
1.1Delete from tablename
DELETE清空表后,返回的结果内有删除的记录条目
DELETE 工作时是一行一行的删除记录数据的;如果表中有自增长字段,使用DELETE FROM 删除
所有记录后,再次新添加的记录会从原来最大的记录ID后面继续自增写入记录。
mysql> select * from class3;
+-----+------+-------+-------------------------------------------+
| num | name | grate | passwd |
+-----+------+-------+-------------------------------------------+
| 1 | cxk | 88.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
| 2 | wyb | 96.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
| 3 | xzq | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
| 4 | lyf | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
+-----+------+-------+-------------------------------------------+
4 rows in set (0.00 sec)
mysql> delete from class3;
Query OK, 4 rows affected (0.00 sec)
mysql> select * from class3;
Empty set (0.00 sec)
mysql> insert into class3 values(1,'cxk',79,123456)
-> ;
Query OK, 1 row affected (0.00 sec)
mysql> select * from class3;
+-----+------+-------+--------+
| num | name | grate | passwd |
+-----+------+-------+--------+
| 1 | cxk | 79.00 | 123456 |
+-----+------+-------+--------+
1 row in set (0.00 sec)
mysql> desc class3;
+--------+--------------+------+-----+---------+-------+
| Field | Type | Null | Key | Default | Extra |
+--------+--------------+------+-----+---------+-------+
| num | varchar(15) | NO | PRI | NULL | |
| name | char(15) | NO | | NULL | |
| grate | decimal(4,2) | YES | | NULL | |
| passwd | char(45) | YES | | | |
+--------+--------------+------+-----+---------+-------+
4 rows in set (0.00 sec)
1.2Truncate table tablename
TRUNCATE清空表后,没有返回被删除的条目: TRUNCATE 工作时是将表结构按原样重新建立,
因此在速度上TRUNCATE会比DELETE清空表快;使用TRUNCATE TABLE 清空表内数据后,
ID会从1开始重新记录
mysql> truncate table class3;
Query OK, 0 rows affected (0.00 sec)
mysql> show tables;
+-----------------+
| Tables_in_class |
+-----------------+
| class |
| class2 |
| class3 |
+-----------------+
3 rows in set (0.00 sec)
mysql> insert into class3 values(1,'cxk',56,123123) ;
Query OK, 1 row affected (0.00 sec)
mysql> select * from class3;
+-----+------+-------+--------+
| num | name | grate | passwd |
+-----+------+-------+--------+
| 1 | cxk | 56.00 | 123123 |
+-----+------+-------+--------+
1 row in set (0.00 sec)
1.3Drop form tablename
mysql> show tables;
+-----------------+
| Tables_in_class |
+-----------------+
| class |
| class2 |
| class3 |
+-----------------+
3 rows in set (0.00 sec)
mysql> drop table class3;
Query OK, 0 rows affected (0.00 sec)
mysql> show tables;
+-----------------+
| Tables_in_class |
+-----------------+
| class |
| class2 |
+-----------------+
2 rows in set (0.00 sec)
1.4 对比
区别 | Drop table tablename | Truncate table tablename | Delete table tablename |
---|---|---|---|
所属SQL语句 | 属于DDL | 属于DDL | 属于DML |
是否可回滚 | 不可回滚(无法恢复) | 不可回滚(无法恢复) | 可回滚(可恢复) |
是否带Where | 不可带Where | 不可带Where | 可带Where |
是否删除表内容和表结构 | 表内容和结构删除 | 表内容删除 | 表结构在,表内容要看where执行情况 |
删除速度 | 删除速度快 | 删除速度快 | 删除速度慢,需要逐行删除 |
速度比较 | 最快 | 次之 | 最慢 |
最好使用Delete |
当不再需要一张表的时候使用Drop;想保留部分数据的时候使用Delete,并且带上Where语句;保留表而删除所有数据的时候用Truncate
2.临时表
临时建立的表,用于保存一些临时数据,不会长期存在(下次重新进入数据库即不存在)
如果在退出连接之前,也可以可执行增删改查等操作,比如使用DROP TABLE语句手动直接删除临时表。
mysql> show tables;
+-----------------+
| Tables_in_class |
+-----------------+
| class |
| class2 |
+-----------------+
2 rows in set (0.00 sec)
mysql> create temporary table class3(id int(6) zerofill primary key auto_increment,name varchar(15) not null,cardid int(18) not null unique key,hobby varchar(60));
#创建临时表 数据表名为class3 id 整型为6字节 zerofill数值不满6位时,前面位数用0补充(00001)
#auto_increment:表示此字段为自增长字段,即每条记录自动递增1,默认从1开始递增;自增长字段数据不可以重复;自增长字段必须是主键;如添加的记录数据没有指定此字段的值且添加失败也会自动递增一次
#unique key:表示此字段唯一键约束,此字段数据不可以重复:一张表中只能有一个主键,但是一张表中可以有多个唯一键
Query OK, 0 rows affected (0.00 sec)
mysql> show tables;
#建立的临时表是看不到的
+-----------------+
| Tables_in_class |
+-----------------+
| class |
| class2 |
+-----------------+
2 rows in set (0.00 sec)
mysql> select * from class3;
Empty set (0.00 sec)
mysql> insert into class3 values(1,'cxk',12345678,ctrl);
ERROR 1054 (42S22): Unknown column 'ctrl' in 'field list'
mysql> insert into class3 values(1,'cxk',12345678,'ctrl');
Query OK, 1 row affected (0.00 sec)
mysql> select * from class3;
+--------+------+----------+-------+
| id | name | cardid | hobby |
+--------+------+----------+-------+
| 000001 | cxk | 12345678 | ctrl |
+--------+------+----------+-------+
1 row in set (0.00 sec)
mysql> insert into class3(name,cardid,hobby) values('wyb',87654321,'skateboardiing');
#若不指定id 可以自动增加
Query OK, 1 row affected (0.00 sec)
mysql> select * from class3;
+--------+------+----------+---------------+
| id | name | cardid | hobby |
+--------+------+----------+---------------+
| 000001 | cxk | 12345678 | ctrl |
| 000002 | wyb | 87654321 | skateboarding |
+--------+------+----------+---------------+
2 rows in set (0.00 sec)
此时退出数据库再重新进入
mysql> quit
Bye
[root@localhost ~]#mysql -uroot -p123456
mysql: [Warning] Using a password on the command line interface can be insecure.
Welcome to the MySQL monitor. Commands end with ; or \g.
Your MySQL connection id is 12
Server version: 5.7.17 Source distribution
Copyright (c) 2000, 2016, Oracle and/or its affiliates. All rights reserved.
Oracle is a registered trademark of Oracle Corporation and/or its
affiliates. Other names may be trademarks of their respective
owners.
Type 'help;' or '\h' for help. Type '\c' to clear the current input statement.
mysql> show databases;
+--------------------+
| Database |
+--------------------+
| information_schema |
| class |
| mysql |
| performance_schema |
| sys |
+--------------------+
5 rows in set (0.00 sec)
mysql> use class;
Reading table information for completion of table and column names
You can turn off this feature to get a quicker startup with -A
Database changed
mysql> show tables;
+-----------------+
| Tables_in_class |
+-----------------+
| class |
| class2 |
+-----------------+
2 rows in set (0.00 sec)
mysql> select * from class3;
ERROR 1146 (42S02): Table 'class.class3' doesn't exist
#临时表找不到了
3.克隆表
3.1Like方法
mysql> show tables;
+-----------------+
| Tables_in_class |
+-----------------+
| class |
| class2 |
+-----------------+
2 rows in set (0.00 sec)
mysql> create table class3 like class2;
#克隆数据表的结构
Query OK, 0 rows affected (0.01 sec)
mysql> show tables;
+-----------------+
| Tables_in_class |
+-----------------+
| class |
| class2 |
| class3 |
+-----------------+
3 rows in set (0.00 sec)
mysql> select * from class3;
Empty set (0.00 sec)
mysql> insert into class3 select * from class2;
#克隆数据表class2的数据内容
Query OK, 4 rows affected (0.00 sec)
Records: 4 Duplicates: 0 Warnings: 0
mysql> select * from class3;
+----+------+-------+-------------------------------------------+
| id | name | grate | passwd |
+----+------+-------+-------------------------------------------+
| 1 | cxk | 88.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
| 2 | wyb | 96.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
| 3 | xzq | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
| 4 | lyf | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
+----+------+-------+-------------------------------------------+
4 rows in set (0.00 sec)
3.2Show create table方法
mysql> show tables;
+-----------------+
| Tables_in_class |
+-----------------+
| class |
| class2 |
| class3 |
+-----------------+
3 rows in set (0.00 sec)
mysql> show create table class2\G
*************************** 1. row ***************************
Table: class2
Create Table: CREATE TABLE "class2" (
"id" int(11) NOT NULL,
"name" char(15) NOT NULL,
"grate" decimal(4,2) DEFAULT NULL,
"passwd" char(45) DEFAULT '',
PRIMARY KEY ("id")
) ENGINE=InnoDB DEFAULT CHARSET=utf8
1 row in set (0.00 sec)
mysql> create table class4(select * from class3);
Query OK, 4 rows affected (0.01 sec)
Records: 4 Duplicates: 0 Warnings: 0
mysql> show tables;
+-----------------+
| Tables_in_class |
+-----------------+
| class |
| class2 |
| class3 |
| class4 |
+-----------------+
4 rows in set (0.00 sec)
mysql> select * from class4;
+----+------+-------+-------------------------------------------+
| id | name | grate | passwd |
+----+------+-------+-------------------------------------------+
| 1 | cxk | 88.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
| 2 | wyb | 96.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
| 3 | xzq | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
| 4 | lyf | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
+----+------+-------+-------------------------------------------+
4 rows in set (0.00 sec)
如果想将数据迁移到其他地方使用Like备份方法;
如果想只备份数据的内容可以使用第二种方法。
四、数据库用户管理
1.新建用户
CREATE USER '用户名'@'来源地址' [IDENTIFIED BY [PASSWORD] '密码'];
- 来源地址:可以指定为localhost本机,也可以指定%(代表任意地址),或者指定为192.168.241.%(代表192.168.241网段的主机都可以登录)
- IDENTIFIED BY:不能省略,如果省略,用户密码将为空(不建议使用)
- 密码:若使用明文密码,直接输入密码即可,键入到数据库中数据库会自动加密;若使用加密密码,需要先使用Select PASSWORD('密码')命令,来获取加密密码,再将获取到的加密密码添加到PASSWORD中
mysql> use mysql;
Reading table information for completion of table and column names
You can turn off this feature to get a quicker startup with -A
Database changed
mysql> select * from user\G;
#查看用户信息 所有创建后的user用户信息均在user表里
*************************** 1. row ***************************
Host: localhost
User: root
Select_priv: Y
Insert_priv: Y
Update_priv: Y
Delete_priv: Y
Create_priv: Y
Drop_priv: Y
Reload_priv: Y
Shutdown_priv: Y
Process_priv: Y
File_priv: Y
Grant_priv: Y
References_priv: Y
Index_priv: Y
Alter_priv: Y
Show_db_priv: Y
Super_priv: Y
Create_tmp_table_priv: Y
Lock_tables_priv: Y
Execute_priv: Y
Repl_slave_priv: Y
Repl_client_priv: Y
Create_view_priv: Y
Show_view_priv: Y
Create_routine_priv: Y
Alter_routine_priv: Y
Create_user_priv: Y
Event_priv: Y
Trigger_priv: Y
Create_tablespace_priv: Y
ssl_type:
ssl_cipher:
x509_issuer:
x509_subject:
max_questions: 0
max_updates: 0
max_connections: 0
max_user_connections: 0
plugin: mysql_native_password
authentication_string: *6BB4837EB74329105EE4568DDA7DC67ED2CA2AD9
password_expired: N
password_last_changed: 2024-03-19 13:37:01
password_lifetime: NULL
account_locked: N
*************************** 2. row ***************************
Host: localhost
User: mysql.sys
Select_priv: N
Insert_priv: N
Update_priv: N
Delete_priv: N
Create_priv: N
Drop_priv: N
Reload_priv: N
Shutdown_priv: N
Process_priv: N
File_priv: N
Grant_priv: N
References_priv: N
Index_priv: N
Alter_priv: N
Show_db_priv: N
Super_priv: N
Create_tmp_table_priv: N
Lock_tables_priv: N
Execute_priv: N
Repl_slave_priv: N
Repl_client_priv: N
Create_view_priv: N
Show_view_priv: N
Create_routine_priv: N
Alter_routine_priv: N
Create_user_priv: N
Event_priv: N
Trigger_priv: N
Create_tablespace_priv: N
ssl_type:
ssl_cipher:
x509_issuer:
x509_subject:
max_questions: 0
max_updates: 0
max_connections: 0
max_user_connections: 0
plugin: mysql_native_password
authentication_string: *THISISNOTAVALIDPASSWORDTHATCANBEUSEDHERE
password_expired: N
password_last_changed: 2024-03-19 13:17:45
password_lifetime: NULL
account_locked: Y
*************************** 3. row ***************************
Host: localhost
User: cxk
Select_priv: N
Insert_priv: N
Update_priv: N
Delete_priv: N
Create_priv: N
Drop_priv: N
Reload_priv: N
Shutdown_priv: N
Process_priv: N
File_priv: N
Grant_priv: N
References_priv: N
Index_priv: N
Alter_priv: N
Show_db_priv: N
Super_priv: N
Create_tmp_table_priv: N
Lock_tables_priv: N
Execute_priv: N
Repl_slave_priv: N
Repl_client_priv: N
Create_view_priv: N
Show_view_priv: N
Create_routine_priv: N
Alter_routine_priv: N
Create_user_priv: N
Event_priv: N
Trigger_priv: N
Create_tablespace_priv: N
ssl_type:
ssl_cipher:
x509_issuer:
x509_subject:
max_questions: 0
max_updates: 0
max_connections: 0
max_user_connections: 0
plugin: mysql_native_password
authentication_string: *6691484EA6B50DDDE1926A220DA01FA9E575C18A
password_expired: N
password_last_changed: 2024-03-20 16:46:58
password_lifetime: NULL
account_locked: N
3 rows in set (0.00 sec)
ERROR:
No query specified
mysql> create user 'cxk'@'localhost' identified by 'abc123';
#新建一个user用户名为cxk 指定在本机可以登录 密码使用加密密码
Query OK, 0 rows affected (0.00 sec)
mysql> select authentication_string from user where user='cxk';
#查看user数据表 下cxk的加密密码 authentication_string代表加密密码的字段
+-------------------------------------------+
| authentication_string |
+-------------------------------------------+
| *6691484EA6B50DDDE1926A220DA01FA9E575C18A |
+-------------------------------------------+
1 row in set (0.00 sec)
2.重命名
mysql> rename user 'cxk'@'localhost' to 'wyb'@'localhost';
Query OK, 0 rows affected (0.00 sec)
mysql> select authentication_string from user where user='cxk';
Empty set (0.00 sec)
mysql> select authentication_string from user where user='wyb';
+-------------------------------------------+
| authentication_string |
+-------------------------------------------+
| *6691484EA6B50DDDE1926A220DA01FA9E575C18A |
+-------------------------------------------+
1 row in set (0.00 sec)
3.删除用户
mysql> drop user 'wyb'@'localhost';
Query OK, 0 rows affected (0.00 sec)
mysql> select authentication_string from user where user='wyb';
Empty set (0.00 sec)
4.修改当前密码
mysql> set password = password('123123');
Query OK, 0 rows affected, 1 warning (0.00 sec)
mysql> quit
Bye
[root@localhost ~]#mysql -uroot -p123123
mysql: [Warning] Using a password on the command line interface can be insecure.
Welcome to the MySQL monitor. Commands end with ; or \g.
Your MySQL connection id is 17
Server version: 5.7.17 Source distribution
Copyright (c) 2000, 2016, Oracle and/or its affiliates. All rights reserved.
Oracle is a registered trademark of Oracle Corporation and/or its
affiliates. Other names may be trademarks of their respective
owners.
Type 'help;' or '\h' for help. Type '\c' to clear the current input statement.
mysql>
5.修改其他用户密码
mysql> set password for 'cxk'@'localhost' = password('123123');
Query OK, 0 rows affected, 1 warning (0.00 sec)
mysql> quit
Bye
[root@localhost ~]#mysql -ucxk -p123123
mysql: [Warning] Using a password on the command line interface can be insecure.
Welcome to the MySQL monitor. Commands end with ; or \g.
Your MySQL connection id is 18
Server version: 5.7.17 Source distribution
Copyright (c) 2000, 2016, Oracle and/or its affiliates. All rights reserved.
Oracle is a registered trademark of Oracle Corporation and/or its
affiliates. Other names may be trademarks of their respective
owners.
Type 'help;' or '\h' for help. Type '\c' to clear the current input statement.
mysql>
6.忘记密码
[root@localhost ~]#vim /etc/my.cnf
[root@localhost ~]#sed -n '24p' /etc/my.cnf
skip-grant-tables
[root@localhost ~]#systemctl restart mysqld
[root@localhost ~]#mysql -uroot -p
Enter password:
Welcome to the MySQL monitor. Commands end with ; or \g.
Your MySQL connection id is 3
Server version: 5.7.17 Source distribution
Copyright (c) 2000, 2016, Oracle and/or its affiliates. All rights reserved.
Oracle is a registered trademark of Oracle Corporation and/or its
affiliates. Other names may be trademarks of their respective
owners.
Type 'help;' or '\h' for help. Type '\c' to clear the current input statement.
mysql>
7.修改密码
7.1Update修改密码
mysql> update mysql.user set authentication_string = password('abc123') where user='root';
Query OK, 1 row affected, 1 warning (0.01 sec)
Rows matched: 1 Changed: 1 Warnings: 1
mysql> flush privileges;
Query OK, 0 rows affected (0.00 sec)
mysql> quit
Bye
[root@localhost ~]#vim /etc/my.cnf
[root@localhost ~]#sed -n '24p' /etc/my.cnf
#skip-grant-tables
[root@localhost ~]#mysql -uroot -pabc123
mysql: [Warning] Using a password on the command line interface can be insecure.
Welcome to the MySQL monitor. Commands end with ; or \g.
Your MySQL connection id is 7
Server version: 5.7.17 Source distribution
Copyright (c) 2000, 2016, Oracle and/or its affiliates. All rights reserved.
Oracle is a registered trademark of Oracle Corporation and/or its
affiliates. Other names may be trademarks of their respective
owners.
Type 'help;' or '\h' for help. Type '\c' to clear the current input statement.
mysql> quit
Bye
7.2直接修改
[root@localhost ~]#vim /etc/my.cnf
[root@localhost ~]#sed -n '24p' /etc/my.cnf
skip-grant-tables
[root@localhost ~]#systemctl restart mysqld
[root@localhost ~]#mysql -uroot -p
Enter password:
Welcome to the MySQL monitor. Commands end with ; or \g.
Your MySQL connection id is 3
Server version: 5.7.17 Source distribution
Copyright (c) 2000, 2016, Oracle and/or its affiliates. All rights reserved.
Oracle is a registered trademark of Oracle Corporation and/or its
affiliates. Other names may be trademarks of their respective
owners.
Type 'help;' or '\h' for help. Type '\c' to clear the current input statement.
mysql> flush privileges;
Query OK, 0 rows affected (0.00 sec)
mysql> set password for root@localhost=password('123123');
Query OK, 0 rows affected, 1 warning (0.00 sec)
mysql> quit
Bye
[root@localhost ~]#vim /etc/my.cnf
[root@localhost ~]#sed -n '24p' /etc/my.cnf
#skip-grant-tables
[root@localhost ~]#systemctl restart mysqld
[root@localhost ~]#mysql -uroot -p123123
mysql: [Warning] Using a password on the command line interface can be insecure.
Welcome to the MySQL monitor. Commands end with ; or \g.
Your MySQL connection id is 3
Server version: 5.7.17 Source distribution
Copyright (c) 2000, 2016, Oracle and/or its affiliates. All rights reserved.
Oracle is a registered trademark of Oracle Corporation and/or its
affiliates. Other names may be trademarks of their respective
owners.
Type 'help;' or '\h' for help. Type '\c' to clear the current input statement.
mysql>
五、数据库用户授权
Grant提权
GRANT 权限列表 ON 数据库名.表名 TO '用户名'@'来源地址' [IDENTIFIED BY '密码'];
- 权限列表:用于列出授权使用的各种数据库操作,以逗号进行分隔,如“select, insert, update”。使用"all"表示所有权限,可授权执行任何操作。
- 数据库名.表名:用于指定授权操作的数据库和表的名称,其中可以使用通配符"*"。例如,使用“class.*"表示授权操作的对象为class数据库中的所有表。
权限 | 含义 |
---|---|
insert | 插入数据 |
select | 查询表数据 |
update | 更新表数据 |
create | 创建库/表 |
drop | 删除库/表 |
refernces | 关联数据表 |
index | 建立索引 |
alter | 更改表属性 |
creat temp orary tableslock tables | 锁表 |
execute | 运行可返回多个结果的给定的 SQL 语句 |
create view | 创建视图 |
show view | 显示视图 |
create routine | 创建存储过程 |
alter routine | 修改存储过程 |
event | 事件 |
trigger on | 创建触发器 |
1.给指定用户select权限
[root@localhost ~]#mysql -uroot -p123123
mysql: [Warning] Using a password on the command line interface can be insecure.
Welcome to the MySQL monitor. Commands end with ; or \g.
Your MySQL connection id is 4
Server version: 5.7.17 Source distribution
Copyright (c) 2000, 2016, Oracle and/or its affiliates. All rights reserved.
Oracle is a registered trademark of Oracle Corporation and/or its
affiliates. Other names may be trademarks of their respective
owners.
Type 'help;' or '\h' for help. Type '\c' to clear the current input statement.
mysql> show databases;
+--------------------+
| Database |
+--------------------+
| information_schema |
| class |
| mysql |
| performance_schema |
| sys |
+--------------------+
5 rows in set (0.00 sec)
mysql> grant select ON class.* to 'cxk'@'localhost' identified by '123123';
#授权select权限class数据库 给 cxk@localhost用户
Query OK, 0 rows affected, 2 warnings (0.00 sec)
mysql> flush privileges;
Query OK, 0 rows affected (0.00 sec)
mysql> quit
Bye
[root@localhost ~]#mysql -ucxk -p123123
mysql: [Warning] Using a password on the command line interface can be insecure.
Welcome to the MySQL monitor. Commands end with ; or \g.
Your MySQL connection id is 5
Server version: 5.7.17 Source distribution
Copyright (c) 2000, 2016, Oracle and/or its affiliates. All rights reserved.
Oracle is a registered trademark of Oracle Corporation and/or its
affiliates. Other names may be trademarks of their respective
owners.
Type 'help;' or '\h' for help. Type '\c' to clear the current input statement.
mysql> select * from class;
ERROR 1046 (3D000): No database selected
mysql> show databases;
+--------------------+
| Database |
+--------------------+
| information_schema |
| class |
+--------------------+
2 rows in set (0.01 sec)
mysql> use class;
Reading table information for completion of table and column names
You can turn off this feature to get a quicker startup with -A
Database changed
mysql> show tables;
+-----------------+
| Tables_in_class |
+-----------------+
| class |
| class2 |
| class3 |
| class4 |
+-----------------+
4 rows in set (0.00 sec)
mysql> select * from class;
+----+------+-------+-------------------------------------------+
| id | name | grate | passwd |
+----+------+-------+-------------------------------------------+
| 1 | cxk | 88.00 | *E56A114692FE0DE073F9A1DD68A00EEB9703F3F1 |
| 2 | wyb | 96.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
| 3 | xzq | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
| 4 | lyf | 95.00 | *437F1809645E0A92DAB553503D2FE21DB91270FD |
+----+------+-------+-------------------------------------------+
4 rows in set (0.00 sec)
mysql> drop table class;
ERROR 1142 (42000): DROP command denied to user 'cxk'@'localhost' for table 'class'
mysql> drop table from class;
ERROR 1064 (42000): You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near 'from class' at line 1
mysql> delete from class;
ERROR 1142 (42000): DELETE command denied to user 'cxk'@'localhost' for table 'class'
mysql> quit
Bye
2.使用Navicat图形化工具远程连接
[root@localhost ~]#mysql -uroot -p123123
mysql: [Warning] Using a password on the command line interface can be insecure.
Welcome to the MySQL monitor. Commands end with ; or \g.
Your MySQL connection id is 6
Server version: 5.7.17 Source distribution
Copyright (c) 2000, 2016, Oracle and/or its affiliates. All rights reserved.
Oracle is a registered trademark of Oracle Corporation and/or its
affiliates. Other names may be trademarks of their respective
owners.
Type 'help;' or '\h' for help. Type '\c' to clear the current input statement.
mysql> grant all privileges on class.* to 'cxk'@'192.168.241.%' identified by '123123';
#授权class数据库的所有权限 给 cxk@192.168.241网段的主机 使用密码123123可以对class数据库做相关操作
Query OK, 0 rows affected, 1 warning (0.00 sec)
mysql> flush privileges;
Query OK, 0 rows affected (0.00 sec)
mysql> quit
Bye
3.查看用户权限
[root@localhost ~]#mysql -uroot -p123123
mysql: [Warning] Using a password on the command line interface can be insecure.
Welcome to the MySQL monitor. Commands end with ; or \g.
Your MySQL connection id is 9
Server version: 5.7.17 Source distribution
Copyright (c) 2000, 2016, Oracle and/or its affiliates. All rights reserved.
Oracle is a registered trademark of Oracle Corporation and/or its
affiliates. Other names may be trademarks of their respective
owners.
Type 'help;' or '\h' for help. Type '\c' to clear the current input statement.
mysql> show grants for 'cxk'@'192.168.241.%';
+------------------------------------------------------------+
| Grants for cxk@192.168.241.% |
+------------------------------------------------------------+
| GRANT USAGE ON *.* TO 'cxk'@'192.168.241.%' |
| GRANT ALL PRIVILEGES ON "class".* TO 'cxk'@'192.168.241.%' |
+------------------------------------------------------------+
2 rows in set (0.00 sec)
mysql> show grants for 'cxk'@'localhost';
+------------------------------------------------+
| Grants for cxk@localhost |
+------------------------------------------------+
| GRANT USAGE ON *.* TO 'cxk'@'localhost' |
| GRANT SELECT ON "class".* TO 'cxk'@'localhost' |
+------------------------------------------------+
2 rows in set (0.00 sec)
4.撤销用户权限
mysql> show grants for 'cxk'@'192.168.241.%';
+------------------------------------------------------------+
| Grants for cxk@192.168.241.% |
+------------------------------------------------------------+
| GRANT USAGE ON *.* TO 'cxk'@'192.168.241.%' |
| GRANT ALL PRIVILEGES ON "class".* TO 'cxk'@'192.168.241.%' |
+------------------------------------------------------------+
2 rows in set (0.00 sec)
mysql> revoke all on class.* from 'cxk'@'192.168.241.%';
#撤销cxk用户192.168.241网段的主机所有class数据库的权限
Query OK, 0 rows affected (0.00 sec)
mysql> show grants for 'cxk'@'192.168.241.%';
+---------------------------------------------+
| Grants for cxk@192.168.241.% |
+---------------------------------------------+
| GRANT USAGE ON *.* TO 'cxk'@'192.168.241.%' |
+---------------------------------------------+
1 row in set (0.00 sec)
mysql> flush privileges;
Query OK, 0 rows affected (0.00 sec)
mysql> show grants for 'cxk'@'192.168.241.%';
+---------------------------------------------+
| Grants for cxk@192.168.241.% |
+---------------------------------------------+
| GRANT USAGE ON *.* TO 'cxk'@'192.168.241.%' |
+---------------------------------------------+
1 row in set (0.00 sec)
六、总结
1.查看数据库、数据表和表结构的操作
- 查看数据库show databases
- 查看数据表show tables
- 查看表结构describe tablename或desc tablename
2.创建库和表的操作及删除库和表的操作
- 创建库create database databasename
- 创建表create table tablename
- 删除库drop database databasename
- 删除表drop table tablename
3.数据表的增、删、改、查等操作
- 数据表增:Insert into tablename
- 数据表改:update tablename set 指定内容
- 数据表删:delete from tablename
- 数据表查:select(*、where、limit、\G)
- 数据表结构名称改:alter table tablename rename 新名称
- 数据表扩展结构增:alter table tablename add 扩展名
- 数据表字段内容改:alter table tablename change 原结构字段名称 新结构字段名称
- 数据表字段内容删:alter table tablename drop 指定结构字段
4.数据库表的清空表、临时表和克隆表操作
4.1清空表
- delete from tablename
- truncate table tablename
- drop from tablename
4.2临时表
create temporary tabletablename
4.3克隆表
- Like方法:create table 新表 like旧表------->insert into 新表 select *from旧表
- Show create table方法:create table 新表(select * from 旧表)
5.数据库的用户授权相关操作
5.1数据库用户管理
新建用户:create user '用户名'@'localhost(或者指定IP)' identified by '密码'
重命名:rename user '旧用户名'@'localhost(或者指定IP)' to '新用户名'@'localhost(或者指定IP)'
删除用户:drop user '用户名'@'localhost(或者指定IP)'
修改当前密码:set password = password('密码')
修改其他用户密码:set password for '用户名'@'localhost' = password('新密码');
修改密码:update mysql.user set authentication_string = password('abc123') where user='root'
修改密码:flush privileges------>set password for root@localhost=password('123123')
5.2数据库授权
授权:grant 权限列表 ON 数据库名.表名 to '用户名'@'来源地址' identified by '密码'
查看权限:show grants '用户名'@'来源地址'
撤销权限:revoke 权限列表 on 数据库名.表名 from '用户名'@'来源地址'
更多推荐
所有评论(0)