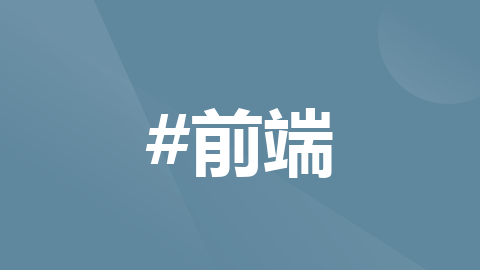
Vue-ECharts基本使用及Demo
Vue-ECharts基于EChartsv4.1.0+ 开发,依赖Vue.jsv2.2.6+。安装$ npm install echarts vue-echarts使用方法import Vue from 'vue'import ECharts from 'vue-echarts' // 在 webpack 环境下指向 components/ECharts.vue/...
Vue-ECharts基于 ECharts v4.1.0
+ 开发,依赖 Vue.js v2.2.6
+。
安装
$ npm install echarts vue-echarts
使用方法
import Vue from 'vue'
import ECharts from 'vue-echarts' // 在 webpack 环境下指向 components/ECharts.vue
// 手动引入 ECharts 各模块来减小打包体积
import 'echarts/lib/chart/bar'
import 'echarts/lib/component/tooltip'
// 如果需要配合 ECharts 扩展使用,只需要直接引入扩展包即可
// 以 ECharts-GL 为例:
// 需要安装依赖:npm install --save echarts-gl,并添加如下引用
import 'echarts-gl'
// 注册组件后即可使用
Vue.component('v-chart', ECharts)
注意事项:
Vue-ECharts 默认在 webpack 环境下会引入未编译的源码版本,如果你正在使用官方的 Vue CLI 来创建项目,可能会遇到默认配置把 node_modules
中的文件排除在 Babel 转译范围以外的问题。请按如下方法修改配置:
当使用 Vue CLI 3+ 时,需要在 vue.config.js
中的 transpileDependencies
增加 vue-echarts
及 resize-detector
,如下:
// vue.config.js
module.exports = {
transpileDependencies: [
'vue-echarts',
'resize-detector'
]
}
当使用 Vue CLI 2 的 webpack
模板时,需要按下述的方式修改 build/webpack.base.conf.js
:
{
test: /\.js$/,
loader: 'babel-loader',
- include: [resolve('src'), resolve('test')]
+ include: [
+ resolve('src'),
+ resolve('test'),
+ resolve('node_modules/vue-echarts'),
+ resolve('node_modules/resize-detector')
+ ]
}
如果你正直接配置使用 webpack,那么也请做类似的修改使其能够正常工作。
全局变量
在没有使用任何模块系统的情况下,组件将通过 window.VueECharts
变量暴露接口:
// 注册组件后即可使用
Vue.component('v-chart', VueECharts)
调用组件
<template>
<v-chart :options="polar"/>
</template>
<style>
/**
* 默认尺寸为 600px×400px,如果想让图表响应尺寸变化,可以像下面这样
* 把尺寸设为百分比值(同时请记得为容器设置尺寸)。
*/
.echarts {
width: 100%;
height: 100%;
}
</style>
<script>
import ECharts from 'vue-echarts'
import 'echarts/lib/chart/line'
import 'echarts/lib/component/polar'
export default {
components: {
'v-chart': ECharts
},
data () {
let data = []
for (let i = 0; i <= 360; i++) {
let t = i / 180 * Math.PI
let r = Math.sin(2 * t) * Math.cos(2 * t)
data.push([r, i])
}
return {
polar: {
title: {
text: '极坐标双数值轴'
},
legend: {
data: ['line']
},
polar: {
center: ['50%', '54%']
},
tooltip: {
trigger: 'axis',
axisPointer: {
type: 'cross'
}
},
angleAxis: {
type: 'value',
startAngle: 0
},
radiusAxis: {
min: 0
},
series: [
{
coordinateSystem: 'polar',
name: 'line',
type: 'line',
showSymbol: false,
data: data
}
],
animationDuration: 2000
}
}
}
}
</script>
Props (均为响应式)
initOptions
用来初始化 ECharts 实例。
theme
当前 ECharts 实例使用的主题。
options
ECharts 实例的数据。修改这个 prop 会触发 ECharts 实例的 setOption
方法。
如果直接修改 options
绑定的数据而对象引用保持不变,setOption
方法调用时将带有参数 notMerge: false
。否则,如果为 options
绑定一个新的对象,setOption
方法调用时则将带有参数 notMerge: true
。
例如,如果有如下模板:
<v-chart :options="data"/>
那么:
this.data = newObject // setOption(this.options, true)
this.data.title.text = 'Trends' // setOption(this.options, false)
group
实例的分组,会自动绑定到 ECharts 组件的同名属性上。
autoresize
(默认值:false
)
这个 prop 用来指定 ECharts 实例在组件根元素尺寸变化时是否需要自动进行重绘。
manual-update
(默认值:false
)
在性能敏感(数据量很大)的场景下,我们最好对于 options
prop 绕过 Vue 的响应式系统。当将 manual-update
prop 指定为 true
且不传入 options
prop 时,数据将不会被监听。然后,你需要用 ref
获取组件实例以后手动调用 mergeOptions
方法来更新图表。
计算属性
width
[只读]
用来获取 ECharts 实例的当前宽度。
height
[只读]
用来获取 ECharts 实例的当前高度。
computedOptions
[只读]
用来读取 ECharts 更新内部 options
后的实际数据。
方法
mergeOptions
(底层调用了 ECharts 实例的setOption
方法)
提供了一个更贴切的名称来描述 setOption
方法的实际行为。
appendData
resize
dispatchAction
showLoading
hideLoading
convertToPixel
convertFromPixel
containPixel
getDataURL
getConnectedDataURL
clear
dispose
静态方法
connect
disconnect
registerMap
registerTheme
graphic.clipPointsByRect
graphic.clipRectByRect
事件
Vue-ECharts 支持如下事件:
legendselectchanged
legendselected
legendunselected
legendscroll
datazoom
datarangeselected
timelinechanged
timelineplaychanged
restore
dataviewchanged
magictypechanged
geoselectchanged
geoselected
geounselected
pieselectchanged
pieselected
pieunselected
mapselectchanged
mapselected
mapunselected
axisareaselected
focusnodeadjacency
unfocusnodeadjacency
brush
brushselected
rendered
finished
- 鼠标事件
click
dblclick
mouseover
mouseout
mousemove
mousedown
mouseup
globalout
contextmenu
DEMO
<template>
<div>
<v-chart :options="barOptions" :autoresize="true"/>
</div>
</template>
<script>
import ECharts from 'vue-echarts'
import 'echarts/lib/chart/pie'
import 'echarts/lib/chart/bar'
import 'echarts/lib/component/tooltip'
import 'echarts/lib/component/legend'
import 'echarts/lib/component/dataZoom'
export default {
data() {
return {
barOptions: {
{
color:['#3AA1FF','#4ECB73','#ACDF82','#FBD437','#EAA674','#F2637B','#DC81D2'],
tooltip: {
trigger:'axis',
confine:true,
axisPointer:{
type:'shadow'
},
textStyle:{
fontSize:12
}
},
legend: {
bottom: 15,
itemWidth:12,
itemHeight:12,
data: [{ name: '估值资产规模', icon: 'rect', }]
},
xAxis: {
data: newData.xAxis,
axisLabel: {
interval: 0
},
axisTick: {
alignWithLabel: true
}
},
yAxis: {
axisTick:false,
axisLine: {
lineStyle: {
width: 0,
color:'#545454'
}
},
splitLine: {
lineStyle: {
color: ['#E9E9E9'],
type:'dashed'
},
},
axisLabel:{
formatter: function (value) {
return value < 10000? value : value/1000000 + '百万'
}
}
},
grid:{
left:'18%',
top: 30,
bottom:70,
right: '5%'
},
dataZoom: [{
type: 'inside',
xAxisIndex: [0],
start: 1,
end: zoomEnd,
zoomLock: true
}],
series: [{
name: '估值资产规模',
type: 'bar',
data: newData.netAssets,
barMaxWidth: 20,
}]
}
}
}
},
components: {
vChart: ECharts
}
}
</script>
更多推荐
所有评论(0)