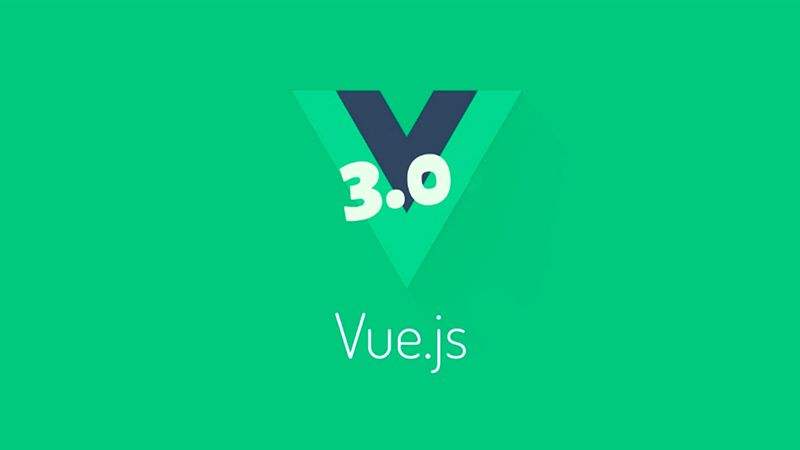
vue.config.js基本配置
vue3、vue2基本配置
·
方法一 (vue3)
vue.config.js
const { defineConfig } = require('@vue/cli-service')
module.exports = defineConfig({
transpileDependencies: true,
lintOnSave: false,
devServer: {
port: 8080,
host:'127.0.0.1',
open: true,
allowedHosts: '*'
},
publicPath: './',
chainWebpack: config => {
if (process.env.NODE_ENV === 'production') {
// #region 忽略生成环境打包的文件
var externals = {
'vue': 'Vue',
'axios': 'axios',
'element-plus': 'ElementPlus',
'vue-router': 'VueRouter',
'vuex': 'Vuex',
'nprogress': 'NProgress',
'echarts': 'echarts'
}
config.externals(externals)
// 在html文件中引入相关CDN
const cdn = {
css: [
// element-ui css
'https://unpkg.com/element-plus@2.1.7/dist/index.css',
// nprogress
'https://cdn.bootcdn.net/ajax/libs/nprogress/0.2.0/nprogress.min.css'
],
js: [
// vue
'https://cdn.bootcdn.net/ajax/libs/vue/3.2.31/vue.runtime.global.prod.min.js',
// vue-router
'https://cdn.bootcdn.net/ajax/libs/vue-router/4.0.14/vue-router.global.prod.min.js',
// vuex
'https://cdn.bootcdn.net/ajax/libs/vuex/4.0.2/vuex.global.prod.min.js',
// axios
'https://cdn.bootcdn.net/ajax/libs/axios/0.26.1/axios.min.js',
// element-plus
'https://unpkg.com/element-plus@2.1.7/dist/index.full.min.js',
// echarts
'https://cdn.bootcdn.net/ajax/libs/echarts/5.3.1/echarts.common.min.js',
// nprogress
'https://cdn.bootcdn.net/ajax/libs/nprogress/0.2.0/nprogress.min.js',
// core-js
'https://cdn.bootcdn.net/ajax/libs/core-js/3.21.1/minified.min.js',
// less
'https://cdn.bootcdn.net/ajax/libs/less.js/4.1.2/less.min.js',
// typescript
'https://cdn.bootcdn.net/ajax/libs/typescript/4.3.5/typescript.min.js'
]
}
config.plugin('html').tap(args => {
args[0].cdn = cdn
return args
})
}
config.when(process.env.NODE_ENV === 'development', config => {
config.entry('app').clear().add('./src/main.ts')
config.plugin('html').tap(args => {
// args[0].isProd = false
args[0].cdn = { css: [], js: [] }
return args
})
})
}
})
index.html
<!DOCTYPE html>
<html lang="">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<link rel="icon" href="<%= BASE_URL %>favicon.ico">
<% for(var css of htmlWebpackPlugin.options.cdn.css) { %>
<link href="<%=css%>" rel="preload" as="style">
<link rel="stylesheet" href="<%=css%>" as="style">
<% } %>
<% for(var js of htmlWebpackPlugin.options.cdn.js) { %>
<link href="<%=js%>" rel="preload" as="script">
<script src="<%=js%>"></script>
<% } %>
<title>document</title>
</head>
<body>
<noscript>
<strong>We're sorry but <%= htmlWebpackPlugin.options.title %> doesn't work properly without JavaScript enabled. Please enable it to continue.</strong>
</noscript>
<div id="app"></div>
<!-- built files will be auto injected -->
</body>
</html>
方法二 (vue2)
vue.config.js
module.exports = {
devServer: {
host:'127.0.0.1',
port: 8000,
open: true,
disableHostCheck: true
},
publicPath: process.env.NODE_ENV === 'production'
? './'
: '/',
chainWebpack: config => {
config.when(process.env.NODE_ENV === 'production', config => {
config.entry('app').clear().add('./src/main-pro.js')
config.set('externals', {
vue: 'Vue',
'vue-router': 'VueRouter',
axios: 'axios',
echarts: 'echarts',
nprogress: 'NProgress',
'vue-quill-editor': 'VueQuillEditor'
})
config.plugin('html').tap(args => {
args[0].isProd = true
return args
})
})
config.when(process.env.NODE_ENV === 'development', config => {
config.entry('app').clear().add('./src/main-dev.js')
config.plugin('html').tap(args => {
args[0].isProd = false
return args
})
})
}
}
index.html
<!DOCTYPE html>
<html lang="">
<head>
<meta charset="utf-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width,initial-scale=1.0">
<link rel="icon" href="<%= BASE_URL %>favicon.ico">
<title><%= htmlWebpackPlugin.options.isProd ? '' : 'dev - ' %>后台管理系统</title>
<% if(htmlWebpackPlugin.options.isProd){ %>
<!-- nprogress 的样式表文件 -->
<link rel="stylesheet" href="https://cdn.staticfile.org/nprogress/0.2.0/nprogress.min.css" />
<!-- 富文本编辑器 的样式表文件 -->
<link rel="stylesheet" href="https://cdn.staticfile.org/quill/1.3.4/quill.core.min.css" />
<link rel="stylesheet" href="https://cdn.staticfile.org/quill/1.3.4/quill.snow.min.css" />
<link rel="stylesheet" href="https://cdn.staticfile.org/quill/1.3.4/quill.bubble.min.css" />
<!-- element-ui 的样式表文件 -->
<link rel="stylesheet" href="https://cdn.staticfile.org/element-ui/2.4.5/theme-chalk/index.css" />
<script src="https://cdn.staticfile.org/vue/2.5.22/vue.min.js"></script>
<script src="https://cdn.staticfile.org/vue-router/3.0.1/vue-router.min.js"></script>
<script src="https://cdn.staticfile.org/axios/0.18.0/axios.min.js"></script>
<script src="https://cdn.staticfile.org/lodash.js/4.17.11/lodash.min.js"></script>
<script src="https://cdn.staticfile.org/echarts/4.1.0/echarts.min.js"></script>
<script src="https://cdn.staticfile.org/nprogress/0.2.0/nprogress.min.js"></script>
<!-- 富文本编辑器的 js 文件 -->
<script src="https://cdn.staticfile.org/quill/1.3.4/quill.min.js"></script>
<script src="https://cdn.jsdelivr.net/npm/vue-quill-editor@3.0.4/dist/vue-quill-editor.js"></script>
<!-- element-ui 的 js 文件 -->
<script src="https://cdn.staticfile.org/element-ui/2.4.5/index.js"></script>
<% } %>
</head>
<body>
<noscript>
<strong>We're sorry but <%= htmlWebpackPlugin.options.title %> doesn't work properly without JavaScript enabled. Please enable it to continue.</strong>
</noscript>
<div id="app"></div>
<!-- built files will be auto injected -->
</body>
</html>
更多推荐
所有评论(0)