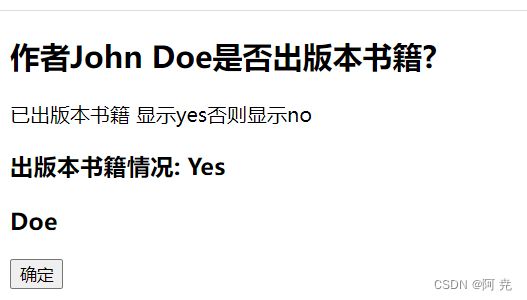
Vue的computed属性,该属性只读,如何现实修改?
Vue的computed属性,该属性只读,如何现实修改?
computed:计算属性
- 本质上是一个属性, 定义时使用方法形式
- 一定有返回值,返回值是计算属性值
- 计算属性方法体中,用到响应式属性,只要数据变化,计算属性自动重新执行
- 计算属性特点: 缓存数据
- 获取计算属性值先从缓存中获取,只有依赖的响应式属性变化时才重新计算,默认只读
- 要改为可写,需要写成对象形式,设置getter setter方法
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta http-equiv="X-UA-Compatible" content="IE=edge" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>计算属性computed</title>
</head>
<body>
<div id="app">
<h2>作者{{author.name}}是否出版本书籍?</h2>
<p>已出版本书籍 显示yes否则显示no</p>
<h3>出版本书籍情况: <span>{{ isBooks }}</span></h3>
<h3>{{fullName}}</h3>
<button @click="updateIsBooks">确定</button>
</div>
<script src="../day2/lib/vue.global.js"></script>
<script>
const { createApp } = Vue
const app =createApp({
data() {
return {
author: {
name: 'John Doe',
books: ['javascript高级编程', 'css入门', 'vue3高级编程'],
},
firstName:'Doe'
}
},
methods: {
updateIsBooks(){
this.isBooks = 'no'
this.fullName = '张三'
console.log(this.isBooks);
}
},
computed:{
isBooks(){
console.log('计算属性值');
return this.author.books.length>0?'Yes':'No'
},
// 定义成geter seter形式
fullName:{
get(){
console.log('get >>>');
return this.firstName
},
set(value){
console.log('value ',value)
this.firstName = value
}
}
}
}).mount('#app')
</script>
</body>
</html>
这里的set(vallue)接收的是 this.fullName = '张三',将张三的值传过来,设置给fullName,
computed属性只读不可写,所以我们需要创建一个对象,getter setter来完成我们属性修改。
效果如下,点击确实,更改名字。
计算属性特点:缓存
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>计算属性缓存</title>
<script src="../day1/lib/vue.global.js"></script>
</head>
<body>
<div id="app"></div>
<script>
const { createApp } = Vue
const App = {
data() {
return {
message:'hello'
}
},
methods: {
},
computed: {
reverseMessage(){
console.log('执行计算属性 reverseMessage >>> ');
return this.message.split('').reverse().join('')
}
},
/*html*/
template: `<div>
<p>文本: {{message}}</p>
<p>反转文本: {{ reverseMessage }}</p>
</div>`
}
const app = createApp(App).mount('#app')
</script>
</body>
</html>
输入message的值的时候,他本身就等于hello,所以会显示一个hello,是缓存的结果。
但是输入java,message的原来的值发生变化的时候,会执行计算属性重新计算。从而得到一个avaj。
计算属性例子:用于总价计算
其中计算方式可以使用for循环,也可以用Reduce方法
computed: {
totalPrice() {
// 计算总价
// let sum = 0
// for(let i = 0; i < this.list.length; i++){
// let product = this.list[i]
// sum = sum + product.price
// }
let sum = this.list.reduce((previous, current) => previous + current.price, 0)return sum.toFixed(2)
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>计算总价</title>
<script src="../lib/vue.global.js"></script>
</head>
<body>
<div id="app"></div>
<script>
const { createApp } = Vue
const App = {
data() {
return {
list: [
{ id: 1001, name: 'javascript高级编程', price: 88.89 },
{ id: 1002, name: 'vue高级编程', price: 118.88 },
],
}
},
methods: {
//添加商品
bindAddBook(){
let product = {id:1003,name:'react高级编程',price:200}
this.list.push(product)
}
},
computed: {
totalPrice() {
// 计算总价
// let sum = 0
// for(let i = 0; i < this.list.length; i++){
// let product = this.list[i]
// sum = sum + product.price
// }
let sum = this.list.reduce((previous, current) => previous + current.price, 0)
return sum.toFixed(2)
}
},
/*html*/
template: `<div>
<table>
<tr>
<th>序号</th>
<th>名称</th>
<th>价格</th>
</tr>
<tr v-for="item,index in list">
<td>{{item.id}}</td>
<td>{{item.name}}</td>
<td>{{item.price}}</td>
</tr>
</table>
<h2>总价: <span>{{totalPrice}}</span></h2>
<button @click="bindAddBook">添加商品</button>
</div>`
}
const app = createApp(App)
app.mount('#app')
</script>
</body>
</html>
深度监听/侦听属性watch,
- 监听一个对象的时候要加deep:true;
- 需要立即执行的时候加: immediate:true
更多推荐
所有评论(0)