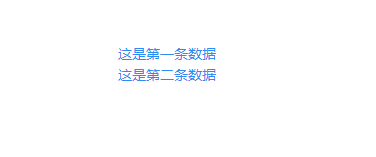
vue v-for循环列表,点击当前项(每一项),通过后台数据id进行url传参,进行页面(路由)跳转到同一个页面
官方解释:当使用路由参数时,例如从 /user/foo 导航到 /user/bar,原来的组件实例会被复用。在项目中经常遇到,用v-for循环出一个列表,点击当前项跳转到另一个页面,跳转后的页面使用的是同一个页面的基础结构,这个时候就需要使用url传参传值给后台,来返回不同数据用于渲染页面。当然,如果你觉得文章有什么让你觉得不合理、或者有更简单的实现方法又或者有理解不来的地方,希望你在看到之后能够
·
前言
在项目中经常遇到,用v-for循环出一个列表,点击当前项跳转到另一个页面,跳转后的页面使用的是同一个页面的基础结构,这个时候就需要使用url传参传值给后台,来返回不同数据用于渲染页面
1.跳转前页面(a.vue)
<template>
<ul>
<li
v-for="(item, index) in list"
:key="index"
@click="jump(item.id)"
>
{{ item.title }}
</li>
</ul>
</template>
<script>
export default {
data() {
return {
list: [
{ id: 1, title: '这是第一条数据' },
{ id: 2, title: '这是第二条数据' }
]
};
},
methods: {
// 编程式导航路由跳转
jump(id) {
this.$router.push({
name: 'b',
query: { id }
});
}
}
};
</script>
2.配置路由
打开router文件夹下index.js
import Vue from 'vue';
import VueRouter from 'vue-router';
Vue.use(VueRouter);
const routes = [
{
path: '/a',
name: 'a',
component: () => import('../views/a.vue')
},
{
path: '/b',
name: 'b',
component: () => import('../views/b.vue')
}
];
const router = new VueRouter({
mode: 'history',
base: process.env.BASE_URL,
routes
});
export default router;
3.跳转前后页面(b.vue)
01)this.$route 可以获取到从上个页面跳转到当前页面的所有路由参数
02)使用axios,将接收到id传给后台,返回不同数据进行渲染页面
<template>
<button @click="jump">回到a页面</button>
</template>
<script>
export default {
created () {
this.getData();
},
methods: {
getData () {
// 接收到a.vue页面传递的id
let id = this.$route.query.id;
this.axios.get('/api', {
params: { id }
})
.then(res => {
console.log(res);
})
.catch(err => {
console.log(err);
});
},
jump() {
this.$router.push({ name: 'a' });
}
}
}
</script>
4.点击a.vue的列表,跳转到第二页面视图,可以看到实现了id的传参
5.当点击第二个列表的时候,出现问题,id没有被传递
6.监听路由
官方解释:当使用路由参数时,例如从 /user/foo 导航到 /user/bar,原来的组件实例会被复用。因为两个路由都渲染同个组件,比起销毁再创建,复用则显得更加高效。不过,这也意味着组件的生命周期钩子不会再被调用
<template>
<button @click="jump">回到a页面</button>
</template>
<script>
export default {
watch: {
'$route': {
handler(to, from) {
if (!from) {
// 对路由变化作出响应...
this.getData();
}
},
immediate: true
}
},
methods: {
getData () {
// 接收到a.vue页面传递的id
let id = this.$route.query.id;
this.axios.get('/api', {
params: { id }
})
.then(res => {
console.log(res);
})
.catch(err => {
console.log(err);
});
},
jump() {
this.$router.push({ name: 'a' });
}
}
}
</script>
如果本篇文章对你有帮助的话,很高兴能够帮助上你。
当然,如果你觉得文章有什么让你觉得不合理、或者有更简单的实现方法又或者有理解不来的地方,希望你在看到之后能够在评论里指出来,我会在看到之后尽快的回复你。
更多推荐
所有评论(0)