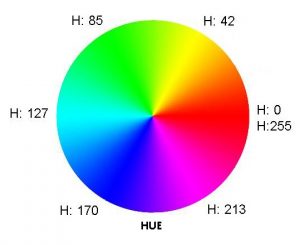
04. fastLED像素参考(颜色设置详解)
库中有两种主要的像素类型 - CRGB 类和 CHSV 类。CHSV 对象必须先转换为 CRGB 对象,然后才能写出它们。您还可以将 CHSV 对象写入 CRGB 数组,并根据需要进行转换。CRGB 颜色对象在内部使用单独的红色、绿色和蓝色通道来表示每种复合颜色,因为这与多色 LED 完全相同:它们在每个“像素”中有一个红色 LED、一个绿色 LED 和一个蓝色 LED。通过混合不同数量的红色、绿
fastLED像素参考
原文
参考:FastLED库 (taichi-maker.com)
关于颜色运算符:请参考这里
Overview 概述
There’s two main pixel types in the library - the CRGB class and the CHSV class. CHSV objects have to be converted to CRGB objects before they can be written out. You can also write CHSV objects into the CRGB array and have the translation occur as necessary.
库中有两种主要的像素类型 - CRGB 类和 CHSV 类。 CHSV 对象必须先转换为 CRGB 对象,然后才能写出它们。您还可以将 CHSV 对象写入 CRGB 数组,并根据需要进行转换。
CRGB Reference CRGB 参考
A “CRGB” is an object representing a color in RGB color space. It contains simply:
“CRGB”是表示RGB色彩空间中颜色的对象。它包含以下内容:
- a one byte value (0-255) representing the amount of red,
一个字节值 (0-255) 表示红色的数量, - a one byte value (0-255) representing the amount of green,
一个字节值 (0-255) 表示绿色的数量, - a one byte value (0-255) representing the amount of blue in a given color.
一个字节值 (0-255),表示给定颜色中的蓝色量。
Typically, when using this library, each LED strip is represented as an array of CRGB colors, one color for each LED pixel.
通常,使用此库时,每个 LED 灯条都表示为 CRGB 颜色数组,每个 LED 像素对应一种颜色。
#define NUM_LEDS 160
CRGB leds[ NUM_LEDS ];
For more general information on what the RGB color space is, see http://en.wikipedia.org/wiki/RGB_color_model
有关 RGB 色彩空间的更多一般信息,请参阅 http://en.wikipedia.org/wiki/RGB_color_model
Data Members 数据成员
CRGB has three one-byte data members, each representing one of the three red, green, and blue color channels of the color. There is more than one way to access the RGB data; each of these following examples does exactly the same thing:
CRGB 有三个单字节数据成员,每个成员代表颜色的三个红色、绿色和蓝色通道之一。访问RGB数据的方法不止一种;以下每个示例都执行完全相同的操作:
// The three color channel values can be referred to as "red", "green", and "blue"...
leds[i].red = 50;
leds[i].green = 100;
leds[i].blue = 150;
// ...or, using the shorter synonyms "r", "g", and "b"...
leds[i].r = 50;
leds[i].g = 100;
leds[i].b = 150;
// ...or as members of a three-element array:
leds[i][0] = 50; // red
leds[i][1] = 100; // green
leds[i][2] = 150; // blue
Direct Access 直接访问
You are welcome, and invited, to directly access the underlying memory of this object if that suits your needs. That is to say, there is no “CRGB::setRed( myRedValue )” method; instead you just directly store ‘myRedValue’ into the “.red” data member on the object. All of the methods on the CRGB class expect this, and will continue to operate normally. This is a bit unusual for a C++ class, but in a microcontroller environment this can be critical to maintain performance.
如果适合您的需求,欢迎您直接访问此对象的底层内存。也就是说,没有“CRGB::setRed( myRedValue )
”方法;相反,您只需将“myRedValue”直接存储到对象上的“.red”数据成员中即可。CRGB 类上的所有方法都期望这一点,并将继续正常运行。这对于 C++ 类来说有点不寻常,但在微控制器环境中,这对于保持性能至关重要。
The CRGB object “is trivially copyable”, meaning that it can be copied from one place in memory to another and still function normally.
CRGB 对象“是微不足道的可复制的”,这意味着它可以从内存中的一个位置复制到另一个位置,并且仍然可以正常运行。
Methods 方法
In addition to simply providing data storage for the RGB colors of each LED pixel, the CRGB class also provides several useful methods color-manipulation, some of which are implemented in assembly language for speed and compactness. Often using the class methods described here is faster and smaller than hand-written C/C++ code to achieve the same thing.
除了简单地为每个 LED 像素的 RGB 颜色提供数据存储外,CRGB 类还提供了几种有用的颜色处理方法,其中一些是用汇编语言实现的,以提高速度和紧凑性。通常使用这里描述的类方法比手写的 C/C++ 代码更快、更小来实现同样的事情。
Setting RGB Colors 设置 RGB 颜色
CRGB colors can be set by assigning values to the individual red, green, and blue channels. In addition, CRGB colors can be set a number of other ways which are often more convenient and compact. The two pieces of code below perform the exact same function.
可以通过为各个红色、绿色和蓝色通道分配值来设置 CRGB 颜色。此外,CRGB颜色还可以通过许多其他方式进行设置,这些方式通常更方便,更紧凑。下面的两段代码执行完全相同的功能。
//Example 1: set color from red, green, and blue components individually
leds[i].red = 50;
leds[i].green = 100;
leds[i].blue = 150;
//Example 2: set color from red, green, and blue components all at once
leds[i] = CRGB( 50, 100, 150);
Some performance-minded programmers may be concerned that using the ‘high level’, ‘object-oriented’ code in the second example comes with a penalty in speed or code size. However, this is simply not the case; the examples above generate literally identical machine code, taking up exactly the same amount of program memory, and executing in exactly the same amount of time. Given that, the choice of which way to write the code, then, is entirely a matter of personal taste and style. All other things being equal, the simpler, higher-level, more object-oriented code is generally recommended.
一些注重性能的程序员可能会担心,在第二个示例中使用“高级”、“面向对象”的代码会降低速度或代码大小。然而,事实并非如此。上面的例子生成了完全相同的机器代码,占用了完全相同的程序内存量,并在完全相同的时间内执行。鉴于此,选择哪种方式编写代码完全是个人品味和风格的问题。在所有其他条件相同的情况下,通常建议使用更简单、更高级别、更面向对象的代码。
Here are the other high-level ways to set a CRGB color in one step:
以下是一步设置 CRGB 颜色的其他高级方法:
// Example 3: set color via 'hex color code' (0xRRGGBB)
leds[i] = 0xFF007F;
// Example 4: set color via any named HTML web color
leds[i] = CRGB::HotPink;
// Example 5: set color via setRGB
leds[i].setRGB( 50, 100, 150);
Again, for the performance-minded programmer, it’s worth noting that all of the examples above compile down into exactly the same number of machine instructions. Choose the method that makes your code the simplest, most clear, and easiest to read and modify.
同样,对于注重性能的程序员来说,值得注意的是,上面的所有示例都编译成完全相同数量的机器指令。选择使代码最简单、最清晰、最易于阅读和修改的方法。
Colors can also be copied from one CRGB to another:
颜色也可以从一个 CRGB 复制到另一个 CRGB:
// Copy the CRGB color from one pixel to another
leds[i] = leds[j];
If you are copying a large number of colors from one (part of an) array to another, the standard library function memmove can be used to perform a bulk transfer; the CRGB object “is trivially copyable”.
如果要将大量颜色从一个(部分)数组复制到另一个数组,则可以使用标准库函数 memmove 来执行批量传输;CRGB 对象“是微不足道的可复制的”。
// Copy ten led colors from leds[src .. src+9] to leds[dest .. dest+9]
memmove( &leds[dest], &leds[src], 10 * sizeof( CRGB) );
Performance-minded programmers using AVR/ATmega MCUs to move large number of colors in this way may wish to use the alternative “memmove8” library function, as it is measurably faster than the standard libc “memmove”.
使用AVR/ATmega MCU以这种方式移动大量颜色,具有性能意识的程序员可能希望使用替代的“memmove8
”库函数,因为它比标准的libc
“memmove
”快得多。
Setting HSV Colors 设置 HSV 颜色
Introduction to HSV HSV简介
CRGB color objects use separate red, green, and blue channels internally to represent each composite color, as this is exactly the same way that multicolor LEDs do it: they have one red LED, one green LED, and one blue LED in each ‘pixel’. By mixing different amounts of red, green, and blue, thousands or millions of resultant colors can be displayed.
CRGB 颜色对象在内部使用单独的红色、绿色和蓝色通道来表示每种复合颜色,因为这与多色 LED 完全相同:它们在每个“像素”中有一个红色 LED、一个绿色 LED 和一个蓝色 LED。通过混合不同数量的红色、绿色和蓝色,可以显示数千或数百万种生成的颜色。
However, working with raw RGB values in your code can be awkward in some cases. For example, it is difficult to work express different tints and shades of a single color using just RGB values, and it can be particular daunting to describe a ‘color wash’ in RGB that cycles around a rainbow of hues while keeping a constant brightness.
不过,在某些情况下,在代码中使用原始 RGB 值可能会很麻烦。例如,仅使用 RGB 值很难表达单一颜色的不同色调和深浅,而用 RGB 值描述 “洗色”,即在彩虹色调中循环,同时保持恒定的亮度,则尤其令人生畏。
To simplify working with color in these ways, the library provides access to an alternate color model based on three different axes: Hue, Saturation, and Value (or ‘Brightness’). For a complete discussion of HSV color, see http://en.wikipedia.org/wiki/HSL_and_HSV , but briefly:
为了简化以这些方式处理颜色的过程,该库提供了对基于三个不同轴的备用颜色模型的访问:色相、饱和度和亮度。有关HSV颜色的完整讨论,请参阅 http://en.wikipedia.org/wiki/HSL_and_HSV,但简要介绍:
- Hue is the ‘angle’ around a color wheel
色相是色轮周围的“角度” - Saturation is how ‘rich’ (versus pale) the color is
饱和度是颜色的“丰富”程度(相对于苍白) - Value is how ‘bright’ (versus dim) the color is
值是颜色的“亮度”(相对于暗淡度)
HSV颜色简介
HSV(Hue, Saturation, Value)是根据颜色的直观特性由A. R. Smith在1978年创建的一种颜色表达方法。该方法中的三个参数分别是:色调(H),饱和度(S),明亮度(V)。
HSV参数
色调H
色调参数取值范围为0~255。如上图所示,从红色开始按逆时针方向计算。红色为0,绿色为85,蓝色为170。
饱和度S
饱和度S表示颜色接近光谱色的程度。取值范围为0~255,值越大,颜色越饱和(越接近本色)。值越小,颜色越接近白色。当数值为零时,颜色为白色。
亮度V
明亮度V表示颜色明亮的程度,取值范围为0~255。对于FastLED库来说,该数值越大,则控制光带的亮度越亮。反之数值越小,则控制光带的亮度越低。当数值为零时,光带完全熄灭。
In the library, the “hue” angle is represented as a one-byte value ranging from 0-255. It runs from red to orange, to yellow, to green, to aqua, to blue, to purple, to pink, and back to red. Here are the eight cardinal points of the hue cycle in the library, and their corresponding hue angle.
在库中,“色调”角度表示为一个字节值,范围为 0-255。它从红色到橙色,到黄色,到绿色,到浅绿色,到蓝色,到紫色,到粉红色,再回到红色。以下是库中色相循环的八个基点,以及它们对应的色相角度。
Click here for full-size chart.
点击这里查看全尺寸图表。
-
Red (0…) "HUE_RED
-
Orange (32…) “HUE_ORANGE”
-
Yellow (64…) “HUE_YELLOW”
-
Green (96…) “HUE_GREEN”
-
Aqua (128…) “HUE_AQUA”
-
Blue (160…) “HUE_BLUE”
-
Purple (192…) “HUE_PURPLE”
-
Pink(224…) “HUE_PINK”
Often in other HSV color spaces, hue is represented as an angle from 0-360 degrees. But for compactness, efficiency, and speed, this library represents hue as a single-byte number from 0-255. There’s a full wiki page how FastLED deals with HSV colors here.
通常在其他 HSV 颜色空间中,色调表示为 0-360 度的角度。但为了紧凑、高效和速度,该库将色调表示为 0-255 之间的单字节数。这里有一个完整的 wiki 页面,介绍了 FastLED 如何处理 HSV 颜色。
“saturation” is a one-byte value ranging from 0-255, where 255 means “completely saturated, pure color”, 128 means “half-saturated, a light, pale color”, and 0 means “completely de-saturated: plain white”.
“饱和度”是一个介于 0-255 之间的单字节值,其中 255 表示“完全饱和,纯色”,128 表示“半饱和,浅色”,0 表示“完全不饱和:纯白色”。
“value” is a one-byte value ranging from 0-255 representing brightness, where 255 means “completely bright, fully lit”, 128 means “somewhat dimmed, only half-lit”, and zero means “completely dark: black.”
“value”是一个字节值,范围从 0-255 表示亮度,其中 255 表示“完全明亮,完全照亮”,128 表示“有点暗,只有半亮”,0 表示“完全黑暗:黑色”。
The CHSV Object【 CHSV 对象】
In the library, a CHSV object is used to represent a color in HSV color space. The CHSV object has the three one-byte data members that you might expect:
在库中,CHSV 对象用于表示 HSV 颜色空间中的颜色。CHSV 对象具有您可能期望的三个单字节数据成员:
- hue (or ‘h’) 色调(或“h”)
- saturation (or ‘sat’, or just ‘s’)
饱和度(或“sat”,或简称“s”) - value (or ‘val’, or just ‘v’) These can be directly manipulated in the same way that red, green, and blue can be on a CRGB object. CHSV objects are also “trivially copyable”.
value(或“val”,或简称“v”) 这些可以直接操作,就像红色、绿色和蓝色可以放在 CRGB 对象上一样。CHSV 对象也是“微不足道的可复制的”。
// Set up a CHSV color
CHSV paleBlue( 160, 128, 255);
// Now...
// paleBlue.hue == 160
// paleBlue.sat == 128
// paleBlue.val == 255
Automatic Color Conversion 自动颜色转换
The library provides fast, efficient methods for converting a CHSV color into a CRGB color. Many of these are automatic and require no explicit code.
该库提供了将 CHSV 颜色转换为 CRGB 颜色的快速、有效的方法。其中许多是自动的,不需要显式代码。
For example, to set an led to a color specified in HSV, you can simply assign a CHSV color to a CRGB color:
例如,要将 LED 设置为 HSV 中指定的颜色,您只需将 CHSV 颜色分配给 CRGB 颜色:
// Set color from Hue, Saturation, and Value.
// Conversion to RGB is automatic.
leds[i] = CHSV( 160, 255, 255);
// alternate syntax
leds[i].setHSV( 160, 255, 255);
// set color to a pure, bright, fully saturated, hue
leds[i].setHue( 160);
There is no conversion back from CRGB to CHSV provided with the library at this point.
此时,库中没有从 CRGB 转换回 CHSV。
Explicit Color Conversion 显式颜色转换
There are two different HSV color spaces: “spectrum” and “rainbow”, and they’re not exactly the same thing. Wikipedia has a good discussion here http://en.wikipedia.org/wiki/Rainbow#Number_of_colours_in_spectrum_or_rainbow but for purposes of the library, it can be summed up as follows:
有两种不同的 HSV 颜色空间:“光谱”和“彩虹”,它们并不完全相同。维基百科在这里有一个很好的讨论 http://en.wikipedia.org/wiki/Rainbow#Number_of_colours_in_spectrum_or_rainbow 但出于库的目的,它可以总结如下:
- “Spectra” have barely any real yellow in them; the yellow band is incredibly narrow.
“光谱”中几乎没有任何真正的黄色;黄色条带非常窄。 - “Rainbows” have a band of yellow approximately as wide as the ‘orange’ and ‘green’ bands around it; the yellow range is easy to see.
“彩虹”有一条黄色带,大约与它周围的“橙色”和“绿色”带一样宽;黄色范围很容易看到。
All of the automatic color conversions in the library use the “HSV Rainbow” color space, but through use of explicit color conversion routines, you can select to use the “HSV Spectrum” color space. There’s a full wiki page how FastLED deals with HSV colors here.
库中的所有自动颜色转换都使用“HSV Rainbow”色彩空间,但通过使用显式颜色转换例程,您可以选择使用“HSV Spectrum”色彩空间。这里有一个完整的 wiki 页面,介绍了 FastLED 如何处理 HSV 颜色。
The first explicit color conversion function is hsv2rgb_rainbow, which is used in the automatic color conversions:
第一个显式颜色转换函数是 hsv2rgb_rainbow,用于自动颜色转换:
// HSV (Rainbow) to RGB color conversion
CHSV hsv( 160, 255, 255); // pure blue in HSV Rainbow space
CRGB rgb;
hsv2rgb_rainbow( hsv, rgb);
// rgb will now be (0, 0, 255) -- pure blue as RGB
The HSV Spectrum color space has different cardinal points, and only six of them, which are correspondingly spread out further numerically. Here is the “Spectrum” color map that FastLED provides if you call hsv2rgb_spectrum explicitly:
HSV Spectrum 色彩空间具有不同的基点,其中只有六个基点,这些基点相应地在数字上进一步分布。以下是 FastLED 提供的“光谱”颜色图,如果您显式调用hsv2rgb_spectrum: Click here for full-size chart.
点击这里查看全尺寸图表。
- Red (0…) 红色 (0…)
- Yellow (42…) 黄色 (42…)
- Green (85…) 绿色 (85…)
- Aqua (128…) 水 (128…)
- Blue (171…) 蓝色 (171…)
- Purple (213…) 紫色 (213…)
The hsv2rgb_spectrum conversion function’s API is identical to hsv2rgb_rainbow:
hsv2rgb_spectrum转换函数的 API 与 hsv2rgb_rainbow 相同:
// HSV (Spectrum) to RGB color conversion
CHSV hsv( 171, 255, 255); // pure blue in HSV Spectrum space
CRGB rgb;
hsv2rgb_spectrum( hsv, rgb);
// rgb will now be (0, 0, 255) -- pure blue as RGB
Why use the Spectrum color space, instead of Rainbow? The HSV Spectrum color space can be converted to RGB a little faster than the HSV Rainbow color space can be – but the results are not as good visually; what little yellow there is appears dim, and at lower brightnesses, almost brownish. So there is a trade-off between a few clock cycles and visual quality. In general, start with the Rainbow functions (or better yet, the automatic conversions), and drop down to the Spectrum functions only if you completely run out of speed.
为什么使用 Spectrum 色彩空间而不是 Rainbow?HSV Spectrum 色彩空间转换为 RGB 的速度比 HSV Rainbow 色彩空间快一点——但结果在视觉上并不那么好;那里的黄色看起来很暗淡,在较低的亮度下,几乎是褐色的。因此,在几个时钟周期和视觉质量之间需要权衡。通常,从 Rainbow 函数(或者更好的是自动转换)开始,只有在完全耗尽速度时才使用Spectrum 函数。
Both color space conversion functions can also convert an array of CHSV colors to a corresponding array of CRGB colors:
这两个色彩空间转换函数还可以将 CHSV 颜色数组转换为相应的 CRGB 颜色数组:
// Convert ten CHSV rainbow values to ten CRGB values;
CHSV hsvs[10];
CRGB leds[10];
// (set hsv values here)
hsv2rgb_rainbow( hsvs, leds, 10); // convert all
The function “hsv2rgb_spectrum” can also be called this way for bulk conversions.
函数“hsv2rgb_spectrum”也可以以这种方式调用以进行批量转换。
Comparing Colors 比较颜色
CRGB colors can be compared for exact matches using ==
and !=
.
可以使用 ==
和 !=
比较 CRGB 颜色的完全匹配。
CRGB colors can be compared for relative light levels using <, >, <=, and =>. Note that this is a simple numeric comparison, and it will not always match the perceived brightness of the colors.
可以使用 <、>、<= 和 => 来比较相对光照水平的 CRGB 颜色。请注意,这是一个简单的数字比较,它并不总是与颜色的感知亮度相匹配。
Often it is useful to check if a color is completely ‘black’, or if it is ‘lit’ at all. You can do this by testing the color directly with ‘if’, or using it in any other boolean context.
通常,检查一种颜色是否完全“黑色”,或者它是否完全“点亮”是很有用的。您可以通过直接使用“if”测试颜色来做到这一点,或者在任何其他布尔上下文中使用它。
// Test if a color is lit at all (versus pure black)
if( leds[i] ) {
/* it is somewhat lit (not pure black) */
} else {
/* it is completely black */
}
Color Math 颜色的数学运算
The library supports a rich set of ‘color math’ operations that you can perform on one or more colors. For example, if you wanted to add a little bit of red to an existing LED color, you could do this:
该库支持一组丰富的“颜色数学”运算,您可以对一种或多种颜色执行这些运算。例如,如果您想在现有的 LED 颜色中添加一点红色,您可以这样做:
// Here's all that's needed to add "a little red" to an existing LED color:
leds[i] += CRGB( 20, 0, 0);
If you’ve ever done this sort of thing by hand before, you may notice something missing: the check for the red channel overflowing past 255. Traditionally, you’ve probably had to do something like this:
如果你以前曾经手动做过这种事情,你可能会注意到缺少一些东西:检查溢出超过 255 的红色通道。传统上,您可能不得不做这样的事情:
// Add a little red, the old way.
uint16_t newRed;
newRed = leds[i].r + 20;
if( newRed > 255) newRed = 255; // prevent wrap-around
leds[i].r = newRed;
This kind of add-and-then-check-and-then-adjust-if-needed logic is taken care of for you inside the library code for adding two CRGB colors, inside operator+ and operator+=. Furthermore, much of this logic is implemented directly in assembly language and is substantially smaller and faster than the corresponding C/C++ code. The net result is that you no longer have to do all the checking yourself, and your program runs faster, too.
这种 “添加–然后检查–然后调整–如果需要 ”的逻辑在添加两种 CRGB 颜色的库代码中、在操作符+
和操作符+=
中自动处理。 此外,这些逻辑大部分是直接用汇编语言实现的,比相应的 C/C++ 代码要小得多、快得多。最终结果是,你不再需要自己进行所有的检查,而且程序运行速度也更快了。
These ‘color math’ operations are part of what makes the library fast: it lets you develop your code faster, as well as executing it faster.
这些“颜色数学”操作是使库快速的部分原因:它可以让您更快地开发代码,并更快地执行代码。
All of the math operations defined on the CRGB colors are automatically protected from wrap-around, overflow, and underflow.
在 CRGB 颜色上定义的所有数学运算都会自动受到保护,防止环绕、溢出和下溢。
Adding and Subtracting Colors 增加和减去颜色
// Add one CRGB color to another.
leds[i] += CRGB( 20, 0, 0);
// Add a constant amount of brightness to all three (RGB) channels.
// 为所有三个(RGB)通道添加恒定亮度。
leds[i].addToRGB(20);
// Add a constant "1" to the brightness of all three (RGB) channels.
leds[i]++;
// Subtract one color from another.
leds[i] -= CRGB( 20, 0, 0);
// Subtract a contsant amount of brightness from all three (RGB) channels.
leds[i].subtractFromRGB(20);
// Subtract a constant "1" from the brightness of all three (RGB) channels.
leds[i]--;
Dimming and Brightening Colors 调暗和增亮颜色
There are two different methods for dimming a color: “video” style and “raw math” style. Video style is the default, and is explicitly designed to never accidentally dim any of the RGB channels down from a lit LED (no matter how dim) to an UNlit LED – because that often comes out looking wrong at low brightness levels. The “raw math” style will eventually fade to black.
有两种不同的调光方法:"视频 "风格和 "原始数学 "风格。视频样式是默认设置,其明确的设计目的是不会意外地将任何 RGB 通道从点亮的 LED(无论多暗)调暗到未点亮的 LED,因为在亮度较低的情况下,这样做往往会显得不妥。原始数学 "样式最终会褪色为黑色。
Colors are always dimmed down by a fraction. The dimming fraction is expressed in 256ths, so if you wanted to dim a color down by 25% of its current brightness, you first have to express that in 256ths. In this case, 25% = 64/256.
颜色总是按分数调暗。调光分数用 256 分之一表示,因此如果要将某个颜色的亮度调低当前亮度的 25%,首先必须用 256 分之一表示。在这种情况下,25% = 64/256。
// Dim a color by 25% (64/256ths)
// using "video" scaling, meaning: never fading to full black
leds[i].fadeLightBy( 64 );
You can also express this the other way: that you want to dim the pixel to 75% of its current brightness. 75% = 192/256. There are two ways to write this, both of which will do the same thing. The first uses the %= operator; the rationale here is that you’re setting the new color to “a percentage” of its previous value:
您也可以用另一种方式来表示:您希望将像素调暗至当前亮度的 75%。75% = 192/256. 有两种写法,都能达到同样的效果。第一种写法使用 %= 运算符;其原理是将新颜色设置为其先前值的 “一定百分比”:
// Reduce color to 75% (192/256ths) of its previous value
// using "video" scaling, meaning: never fading to full black
leds[i] %= 192;
The other way is to call the underlying scaling function directly. Note the “video” suffix.
另一种方法是直接调用底层缩放函数nscale8_video()
。请注意“video”后缀。
// Reduce color to 75% (192/256ths) of its previous value
// using "video" scaling, meaning: never fading to full black
leds[i].nscale8_video( 192);
If you want the color to eventually fade all the way to black, use one of these functions:
如果您希望颜色最终完全褪色为黑色,请使用以下功能之一:
// Dim a color by 25% (64/256ths)
// eventually fading to full black
leds[i].fadeToBlackBy( 64 );
// Reduce color to 75% (192/256ths) of its previous value eventually fading to full black
// 将颜色减至先前值的 75%(192/256 分之一),最终变为全黑
leds[i].nscale8( 192);
A function is also provided to boost a given color to maximum brightness while keeping the same hue:
还提供了一个功能,可以在保持相同色调的同时将给定颜色提升到最大亮度:
// Adjust brightness to maximum possible while keeping the same hue.
leds[i].maximizeBrightness();
Finally, colors can also be scaled up or down using multiplication and division.
最后,还可以使用乘法和除法放大或缩小颜色。
// Divide each channel by a single value
leds[i] /= 2;
// Multiply each channel by a single value
leds[i] *= 2;
Constraining Colors Within Limits 将颜色限制在限制范围内
`|=`运算符`](https://fastled.io/docs/class_c_pixel_view.html#a89e81df9b22cc4ebba8977b89795026b)
`PIXEL_TYPE |= `运算符应用于该集合中具有给定 PIXEL_TYPE 值的每个像素。
使用 CRGB 时,这将使每个通道的值达到两个值中的较高值
[`&=`运算符
"and"运算符使每个通道的值降至两个值中的较低值
The library provides a function that lets you ‘clamp’ each of the RGB channels to be within given minimums and maximums. You can force all of the color channels to be at least a given value, or at most a given value. These can then be combined to limit both minimum and maximum.
该库提供了一个函数,让您可以 “钳制 ”每个 RGB 通道,使其处于给定的最小值和最大值范围内。您可以强制所有颜色通道至少为给定值,或最多为给定值。然后可以将这些值组合起来,限制最小值和最大值。
// Bring each channel up to at least a minimum value.
// If any channel's value is lower than the given minimum for that channel,
// it is raised to the given minimum. The minimum can be specified separately
// for each channel (as a CRGB), or as a single value.
/*
使每个通道至少达到一个最小值。
如果任何通道的值低于给定的最小值,就会将其提升到给定的最小值。
可以为每个通道分别指定最小值(作为 CRGB),也可以指定为一个值。*/
leds[i] |= CRGB( 32, 48, 64);
leds[i] |= 96;
// Clamp each channel down to a maximum value.
// If any channel's value is higher than the given maximum for that channel,
// it is reduced to the given maximum.
// The minimum can be specified separately for each channel (as a CRGB), or as a single value.
/*
将每个通道的值限制在一个最大值。
如果任何通道的值高于给定的最大值,该通道就会被减小到给定的最大值。
每个通道的最小值可以单独指定(作为 CRGB),也可以指定为一个值。*/
leds[i] &= CRGB( 192, 128, 192);
leds[i] &= 160;
Misc Color Functions 其他颜色函数
The library provides a function that ‘inverts’ each RGB channel. Performing this operation twice results in the same color you started with.
该库提供了一个“反转”每个RGB通道的功能。执行此操作两次后,将生成与开始时相同的颜色。
// Invert each channel
leds[i] = -leds[i];
The library also provides functions for looking up the apparent (or mathematical) brightness of a color.
该库还提供了用于查询颜色表观亮度(或数学亮度)的函数。
// Get brightness, or luma (brightness, adjusted for eye's sensitivity to
// different light colors. See http://en.wikipedia.org/wiki/Luma_(video) )
uint8_t luma = leds[i].getLuma();
uint8_t avgLight = leds[i].getAverageLight();
Predefined colors list 预定义颜色列表
Please note - these predefined colors are defined using the W3C RGB definitions. These definitions are designed with RGB monitors in mind, not RGB leds, and so the colors that you get on LED strips may be a bit different than you’re expecting. In our experience, the colors are often too pale, or washed out (overly-desaturated).
请注意 - 这些预定义的颜色是使用 W3C RGB 定义定义的。这些定义在设计时考虑了 RGB 显示器,而不是 RGB LED,因此您在 LED 灯条上获得的颜色可能与您预期的略有不同。根据我们的经验,颜色通常太浅,或者褪色(过度饱和)。
Color name | Hex Value | Example |
---|---|---|
CRGB::AliceBlue | 0xF0F8FF | ![]() |
CRGB::Amethyst | 0x9966CC | ![]() |
CRGB::AntiqueWhite | 0xFAEBD7 | ![]() |
CRGB::Aqua | 0x00FFFF | ![]() |
CRGB::Aquamarine | 0x7FFFD4 | ![]() |
CRGB::Azure | 0xF0FFFF | ![]() |
CRGB::Beige | 0xF5F5DC | ![]() |
CRGB::Bisque | 0xFFE4C4 | ![]() |
CRGB::Black | 0x000000 | ![]() |
CRGB::BlanchedAlmond | 0xFFEBCD | ![]() |
CRGB::Blue | 0x0000FF | ![]() |
CRGB::BlueViolet | 0x8A2BE2 | ![]() |
CRGB::Brown | 0xA52A2A | ![]() |
CRGB::BurlyWood | 0xDEB887 | ![]() |
CRGB::CadetBlue | 0x5F9EA0 | ![]() |
CRGB::Chartreuse | 0x7FFF00 | ![]() |
CRGB::Chocolate | 0xD2691E | ![]() |
CRGB::Coral | 0xFF7F50 | ![]() |
CRGB::CornflowerBlue | 0x6495ED | ![]() |
CRGB::Cornsilk | 0xFFF8DC | ![]() |
CRGB::Crimson | 0xDC143C | ![]() |
CRGB::Cyan | 0x00FFFF | ![]() |
CRGB::DarkBlue | 0x00008B | ![]() |
CRGB::DarkCyan | 0x008B8B | ![]() |
CRGB::DarkGoldenrod | 0xB8860B | ![]() |
CRGB::DarkGray | 0xA9A9A9 | ![]() |
CRGB::DarkGreen | 0x006400 | ![]() |
CRGB::DarkKhaki | 0xBDB76B | ![]() |
CRGB::DarkMagenta | 0x8B008B | ![]() |
CRGB::DarkOliveGreen | 0x556B2F | ![]() |
CRGB::DarkOrange | 0xFF8C00 | ![]() |
CRGB::DarkOrchid | 0x9932CC | ![]() |
CRGB::DarkRed | 0x8B0000 | ![]() |
CRGB::DarkSalmon | 0xE9967A | ![]() |
CRGB::DarkSeaGreen | 0x8FBC8F | ![]() |
CRGB::DarkSlateBlue | 0x483D8B | ![]() |
CRGB::DarkSlateGray | 0x2F4F4F | ![]() |
CRGB::DarkTurquoise | 0x00CED1 | ![]() |
CRGB::DarkViolet | 0x9400D3 | ![]() |
CRGB::DeepPink | 0xFF1493 | ![]() |
CRGB::DeepSkyBlue | 0x00BFFF | ![]() |
CRGB::DimGray | 0x696969 | ![]() |
CRGB::DodgerBlue | 0x1E90FF | ![]() |
CRGB::FireBrick | 0xB22222 | ![]() |
CRGB::FloralWhite | 0xFFFAF0 | ![]() |
CRGB::ForestGreen | 0x228B22 | ![]() |
CRGB::Fuchsia | 0xFF00FF | ![]() |
CRGB::Gainsboro | 0xDCDCDC | ![]() |
CRGB::GhostWhite | 0xF8F8FF | ![]() |
CRGB::Gold | 0xFFD700 | ![]() |
CRGB::Goldenrod | 0xDAA520 | ![]() |
CRGB::Gray | 0x808080 | ![]() |
CRGB::Green | 0x008000 | ![]() |
CRGB::GreenYellow | 0xADFF2F | ![]() |
CRGB::Honeydew | 0xF0FFF0 | ![]() |
CRGB::HotPink | 0xFF69B4 | ![]() |
CRGB::IndianRed | 0xCD5C5C | ![]() |
CRGB::Indigo | 0x4B0082 | ![]() |
CRGB::Ivory | 0xFFFFF0 | ![]() |
CRGB::Khaki | 0xF0E68C | ![]() |
CRGB::Lavender | 0xE6E6FA | ![]() |
CRGB::LavenderBlush | 0xFFF0F5 | ![]() |
CRGB::LawnGreen | 0x7CFC00 | ![]() |
CRGB::LemonChiffon | 0xFFFACD | ![]() |
CRGB::LightBlue | 0xADD8E6 | ![]() |
CRGB::LightCoral | 0xF08080 | ![]() |
CRGB::LightCyan | 0xE0FFFF | ![]() |
CRGB::LightGoldenrodYellow | 0xFAFAD2 | ![]() |
CRGB::LightGreen | 0x90EE90 | ![]() |
CRGB::LightGrey | 0xD3D3D3 | ![]() |
CRGB::LightPink | 0xFFB6C1 | ![]() |
CRGB::LightSalmon | 0xFFA07A | ![]() |
CRGB::LightSeaGreen | 0x20B2AA | ![]() |
CRGB::LightSkyBlue | 0x87CEFA | ![]() |
CRGB::LightSlateGray | 0x778899 | ![]() |
CRGB::LightSteelBlue | 0xB0C4DE | ![]() |
CRGB::LightYellow | 0xFFFFE0 | ![]() |
CRGB::Lime | 0x00FF00 | ![]() |
CRGB::LimeGreen | 0x32CD32 | ![]() |
CRGB::Linen | 0xFAF0E6 | ![]() |
CRGB::Magenta | 0xFF00FF | ![]() |
CRGB::Maroon | 0x800000 | ![]() |
CRGB::MediumAquamarine | 0x66CDAA | ![]() |
CRGB::MediumBlue | 0x0000CD | ![]() |
CRGB::MediumOrchid | 0xBA55D3 | ![]() |
CRGB::MediumPurple | 0x9370DB | ![]() |
CRGB::MediumSeaGreen | 0x3CB371 | ![]() |
CRGB::MediumSlateBlue | 0x7B68EE | ![]() |
CRGB::MediumSpringGreen | 0x00FA9A | ![]() |
CRGB::MediumTurquoise | 0x48D1CC | ![]() |
CRGB::MediumVioletRed | 0xC71585 | ![]() |
CRGB::MidnightBlue | 0x191970 | ![]() |
CRGB::MintCream | 0xF5FFFA | ![]() |
CRGB::MistyRose | 0xFFE4E1 | ![]() |
CRGB::Moccasin | 0xFFE4B5 | ![]() |
CRGB::NavajoWhite | 0xFFDEAD | ![]() |
CRGB::Navy | 0x000080 | ![]() |
CRGB::OldLace | 0xFDF5E6 | ![]() |
CRGB::Olive | 0x808000 | ![]() |
CRGB::OliveDrab | 0x6B8E23 | ![]() |
CRGB::Orange | 0xFFA500 | ![]() |
CRGB::OrangeRed | 0xFF4500 | ![]() |
CRGB::Orchid | 0xDA70D6 | ![]() |
CRGB::PaleGoldenrod | 0xEEE8AA | ![]() |
CRGB::PaleGreen | 0x98FB98 | ![]() |
CRGB::PaleTurquoise | 0xAFEEEE | ![]() |
CRGB::PaleVioletRed | 0xDB7093 | ![]() |
CRGB::PapayaWhip | 0xFFEFD5 | ![]() |
CRGB::PeachPuff | 0xFFDAB9 | ![]() |
CRGB::Peru | 0xCD853F | ![]() |
CRGB::Pink | 0xFFC0CB | ![]() |
CRGB::Plaid | 0xCC5533 | ![]() |
CRGB::Plum | 0xDDA0DD | ![]() |
CRGB::PowderBlue | 0xB0E0E6 | ![]() |
CRGB::Purple | 0x800080 | ![]() |
CRGB::Red | 0xFF0000 | ![]() |
CRGB::RosyBrown | 0xBC8F8F | ![]() |
CRGB::RoyalBlue | 0x4169E1 | ![]() |
CRGB::SaddleBrown | 0x8B4513 | ![]() |
CRGB::Salmon | 0xFA8072 | ![]() |
CRGB::SandyBrown | 0xF4A460 | ![]() |
CRGB::SeaGreen | 0x2E8B57 | ![]() |
CRGB::Seashell | 0xFFF5EE | ![]() |
CRGB::Sienna | 0xA0522D | ![]() |
CRGB::Silver | 0xC0C0C0 | ![]() |
CRGB::SkyBlue | 0x87CEEB | ![]() |
CRGB::SlateBlue | 0x6A5ACD | ![]() |
CRGB::SlateGray | 0x708090 | ![]() |
CRGB::Snow | 0xFFFAFA | ![]() |
CRGB::SpringGreen | 0x00FF7F | ![]() |
CRGB::SteelBlue | 0x4682B4 | ![]() |
CRGB::Tan | 0xD2B48C | ![]() |
CRGB::Teal | 0x008080 | ![]() |
CRGB::Thistle | 0xD8BFD8 | ![]() |
CRGB::Tomato | 0xFF6347 | ![]() |
CRGB::Turquoise | 0x40E0D0 | ![]() |
CRGB::Violet | 0xEE82EE | ![]() |
CRGB::Wheat | 0xF5DEB3 | ![]() |
CRGB::White | 0xFFFFFF | ![]() |
CRGB::WhiteSmoke | 0xF5F5F5 | ![]() |
CRGB::Yellow | 0xFFFF00 | ![]() |
CRGB::YellowGreen | 0x9ACD32 | ![]() |
更多推荐
所有评论(0)