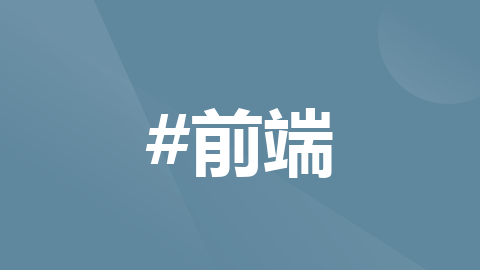
uniapp中使用vuex 及 存储用户 token 和 用户类型
vue
vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
项目地址:https://gitcode.com/gh_mirrors/vu/vue

·
1、创建
页面路径:store/index.js
import Vue from 'vue'
import Vuex from 'vuex'
import getters from './getters.js'
import user from './modules/user.js'
Vue.use(Vuex) // vue的插件机制
// Vue.Store 构造器选项
const store = new Vuex.Store({
state: {
"username": "张三",
"age": 18,
},
modules: {
user
},
getters
})
export default store
页面路径:store/getters.js
const getters = {
// age: state => state.user.age,
userInfo: state => 11,
}
export default getters
页面路径:store/modules/user.js
const user = {
state: {
name: '',
age: '11',
height: '',
weight: '',
userInfo: '',
},
// 相当于同步的操作 接收this.$store.commit('mutations方法名',值)
mutations: {
SET_NAME: (state, name) => {
state.name = name
},
SET_AGE: (state, age) => {
state.age = age
},
SET_USERINFO: (state, data) => {
state.userInfo = data
}
},
//相当于异步的操作,不能直接改变state的值,只能通过触发mutations的方法才能改变, 接收this.$store.dispatch('action方法名',值) 再触发mutations的方法
actions: {
setAge({commit}, age) {
commit('SET_AGE', age)
}
}
}
export default user
设置全局使用: main.js 文件
import App from './App'
// #ifndef VUE3
import Vue from 'vue'
import store from './store'
import './uni.promisify.adaptor'
Vue.prototype.$store = store
Vue.config.productionTip = false
App.mpType = 'app'
// 把store对象提供给 “store” 选项,这可以把 store 的实例注入所有的子组件
const app = new Vue({
store,
...App
})
app.$mount()
// #endif
// #ifdef VUE3
import { createSSRApp } from 'vue'
export function createApp() {
const app = createSSRApp(App)
return {
app
}
}
// #endif
2、state属性,主要功能是存储数据
通过 this.$store 访问到 state 里的数据
<template>
<view class="container">
<view>用户名:{{ username }}</view>
</view>
</template>
<script>
export default {
data() {
return {}
},
computed: {
username() {
return this.$store.state.username;
}
},
}
</script>
vue
vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
项目地址:https://gitcode.com/gh_mirrors/vu/vue
进阶方法:通过 mapState 辅助函数获取。当一个组件需要获取多个状态的时候,将这些状态都声明为计算属性会有些重复和冗余。 为了解决这个问题,我们可以使用 mapState 辅助函数 帮助我们生成计算属性,让你少按几次键,(说白了就是简写,不用一个一个去声明了,避免臃肿)
<template>
<view class="container">
<view>用户名:{{ username }}</view>
<view>年龄:{{ age }}</view>
</view>
</template>
<script>
import { mapState } from 'vuex'
export default {
data() {
return {}
},
computed: {
...mapState({
// 从state中拿到数据 箭头函数可使代码更简练
username: state => state.username,
age: state => state.age,
})
},
}
</script>
3、使用vuex, 操作数据
<template>
<view class="container">
<view>用户名:{{ username }}</view>
<view>年龄:{{ age }}</view>
<button @click="getBook">修改</button>
</view>
</template>
<script>
import { mapState } from 'vuex'
export default {
data() {
return {}
},
computed: {
...mapState({
username: state => state.username,
age: state => state.age,
})
},
mounted() {
console.log(this.$store.state);
},
methods: {
getBook() {
// 同步修改
this.$store.commit('SET_NAME', '圆圆');
// 异步修改
this.$store.dispatch('setAge', 22)
}
}
}
</script>
参考:uniapp中vuex的应用使用步骤_javascript技巧_脚本之家
4、存储用户Token和类型
页面路径:utils/auth.js
const TokenKey = 'Admin-Token' // 存储用户 token 名称
const UserType = 'User-Type' // 存储用户 类型 名称
// 存储用户 token
export function setToken(token) {
return uni.setStorageSync(TokenKey, token);
}
// 查看用户 token
export function getToken() {
return uni.getStorageSync(TokenKey);
}
// 删除用户 token
export function removeToken() {
return uni.removeStorageSync(TokenKey)
}
// 存储用户类型
export function setType(Type) {
return uni.setStorageSync(UserType, Type)
}
// 查看用户类型
export function getType() {
return uni.getStorageSync(UserType)
}
// 删除用户类型
export function removeType() {
return uni.removeStorageSync(UserType)
}
阅读全文
AI总结
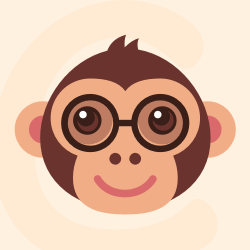



vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
最近提交(Master分支:6 个月前 )
9e887079
[skip ci] 4 个月前
73486cb5
* chore: fix link broken
Signed-off-by: snoppy <michaleli@foxmail.com>
* Update packages/template-compiler/README.md [skip ci]
---------
Signed-off-by: snoppy <michaleli@foxmail.com>
Co-authored-by: Eduardo San Martin Morote <posva@users.noreply.github.com> 8 个月前
更多推荐
相关推荐
查看更多
vue

vuejs/vue: 是一个用于构建用户界面的 JavaScript 框架,具有简洁的语法和丰富的组件库,可以用于开发单页面应用程序和多页面应用程序。
vue

Vue implementation of Geist
vue

A flexible icon family for Vue
所有评论(0)