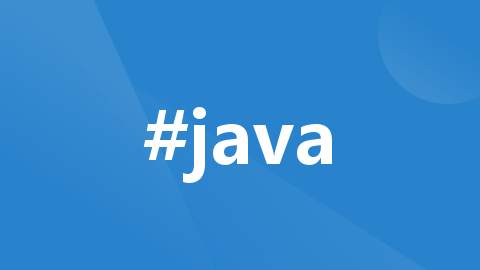
AES解密报错java.security.InvalidKeyException: Invalid AES key length: xx bytes
由于与其它系统接口对接,需要进行加密处理。对接系统采用AES加密方式,作为接收方获取加密内容,通过AES解密拿到数据。
·
一、前言
由于与其它系统接口对接,需要进行加密处理。
对接系统采用AES加密方式,作为接收方获取加密内容,通过AES解密拿到数据。
解密过程中遇到报错如下:
java.security.InvalidKeyException: Invalid AES key length: 20 bytes
二、Invalid AES key length: 20 bytes的解决方法
出现此错误,主要原因是秘钥长度不符合要求所导致的。
AES允许128位,192位或256位密钥长度。 这也就意味着秘钥只能是16,24或32个字节。
话不多说,代码呈现如下:
public class AESUtil {
private static final String AES = "AES";
private static final String UTF8 = "UTF-8";
private static final String CIPHERALGORITHM = "AES/ECB/PKCS5Padding";
private static final String Key = "9!#95hsup*&$1zq79$%a";
/**
* AES加密
*
* @param content
* @return
* @throws Exception
*/
public static String encrypt(String content) {
try {
byte[] encodeFormat = Key.getBytes();
SecretKeySpec key = new SecretKeySpec(encodeFormat, AES);
// Cipher对象实际完成加密操作
Cipher cipher = Cipher.getInstance(CIPHERALGORITHM);
// 加密内容进行编码
byte[] byteContent = content.getBytes(UTF8);
// 用密匙初始化Cipher对象
cipher.init(Cipher.ENCRYPT_MODE, key);
// 正式执行加密操作
byte[] result = cipher.doFinal(byteContent);
return Hex.encodeHexString(result);
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
/**
* AES解密
*
* @param contents
* @return
* @throws Exception
*/
public static String decrypt(String content) {
try {
// 密文使用Hex解码
byte[] byteContent = Hex.decodeHex(content.toCharArray());
byte[] encodeFormat = Key.getBytes();
SecretKeySpec key = new SecretKeySpec(encodeFormat, AES);
// Cipher对象实际完成加密操作
Cipher cipher = Cipher.getInstance(AES);
// 用密匙初始化Cipher对象
cipher.init(Cipher.DECRYPT_MODE, key);
// 正式执行解密操作
byte[] result = cipher.doFinal(byteContent);
return new String(result, UTF8);
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
}
执行报错,因为KEY
的值是9!#95hsup*&$1zq79$%a,长度为20,如果长度修改成16,KEY值为9!#95hsup*&$1zq7,执行就正常了。
更多推荐
所有评论(0)