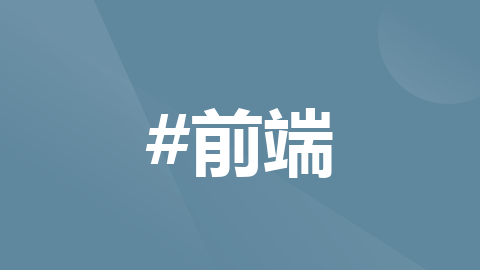
修改图片分辨率,python实现修改图片分辨率(大小)
将input.jpg和output.jpg改成自己的输入输出文件路径。2.将一个目录(文件夹)上的所有图片修改成相同的分辨率。1.修改一张图片分辨率。

一键AI生成摘要,助你高效阅读
问答
·
1.修改一张图片分辨率
将input.jpg和output.jpg改成自己的输入输出文件路径
from PIL import Image
def resize_image(input_image_path, output_image_path, new_width, new_height):
image = Image.open(input_image_path)
resized_image = image.resize((new_width, new_height))
resized_image.save(output_image_path)
# 使用示例
input_image_path = "input.jpg"
output_image_path = "output.jpg"
new_width = 800
new_height = 600
resize_image(input_image_path, output_image_path, new_width, new_height)
2.将一个目录(文件夹)上的所有图片修改成相同的分辨率
import os
from PIL import Image
def resize_images_in_folder(input_folder, output_folder, new_width, new_height):
# 遍历输入文件夹中的所有文件
for filename in os.listdir(input_folder):
if filename.endswith(".jpg") or filename.endswith(".png"):
# 构建输入和输出文件的路径
input_image_path = os.path.join(input_folder, filename)
output_image_path = os.path.join(output_folder, filename)
# 调用resize_image函数修改图片分辨率
resize_image(input_image_path, output_image_path, new_width, new_height)
def resize_image(input_image_path, output_image_path, new_width, new_height):
image = Image.open(input_image_path)
resized_image = image.resize((new_width, new_height))
resized_image.save(output_image_path)
# 使用示例
input_folder = "input_folder"
output_folder = "output_folder"
new_width = 800
new_height = 600
resize_images_in_folder(input_folder, output_folder, new_width, new_height)
3.等比例修改图片分辨率(大小)
from PIL import Image
def resize_image(input_path, output_path, new_width, new_height):
# 打开图片
image = Image.open(input_path)
# 计算缩放比例
width, height = image.size
aspect_ratio = width / height
if new_width is None:
new_width = int(new_height * aspect_ratio)
elif new_height is None:
new_height = int(new_width / aspect_ratio)
# 缩放图片
resized_image = image.resize((new_width, new_height), Image.ANTIALIAS)
# 保存缩放后的图片
resized_image.save(output_path)
if __name__ == "__main__":
input_path = "input.jpg" # 输入图片的文件路径
output_path = "output.jpg" # 输出图片的文件路径
new_width = 800 # 新的宽度,设置为None以根据高度自动计算
new_height = None # 新的高度,设置为None以根据宽度自动计算
resize_image(input_path, output_path, new_width, new_height)
4.递归实现等比例修改一个目录下所有目录的图片分辨率,并且覆盖之前图片
import os
from PIL import Image
def resize_image(input_path, new_width, new_height):
# 打开图片
image = Image.open(input_path)
# 计算缩放比例
width, height = image.size
aspect_ratio = width / height
if new_width is None:
new_width = int(new_height * aspect_ratio)
elif new_height is None:
new_height = int(new_width / aspect_ratio)
# 缩放图片
resized_image = image.resize((new_width, new_height), Image.ANTIALIAS)
# 覆盖原始图片
resized_image.save(input_path)
def resize_images_in_directory(directory, new_width, new_height):
for root, dirs, files in os.walk(directory):
for filename in files:
if filename.lower().endswith(('.jpg', '.jpeg', '.png', '.gif')):
input_path = os.path.join(root, filename)
resize_image(input_path, new_width, new_height)
if __name__ == "__main__":
target_directory = "your_directory_path" # 指定要处理的目录的路径
new_width = 800 # 新的宽度,设置为None以根据高度自动计算
new_height = None # 新的高度,设置为None以根据宽度自动计算
resize_images_in_directory(target_directory, new_width, new_height)
更多推荐
所有评论(0)