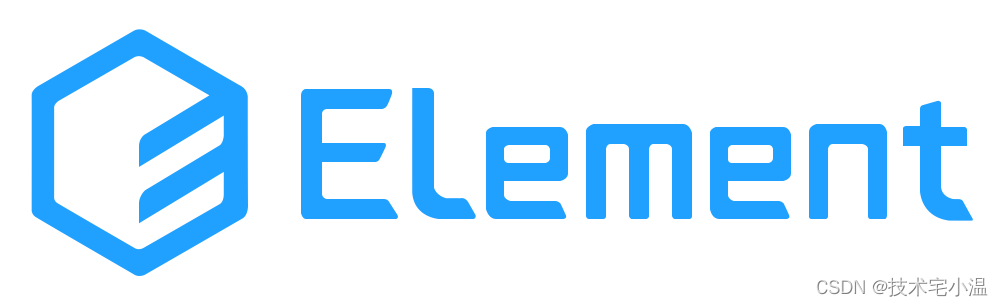
< vue + ElementUi 组件封装:实现弹窗展示富文本数据,允许全文搜索高亮显示搜索内容 >
在 Vue + elementUi 开发中,遇到需要实现一个富文本展示 且 需要实现富文本全文搜索,高亮对应搜索内容。显示关键词出现次数,允许上下按顺序切换,实现滚动条定位到对应关键词位置!接下来,简单阐述下,开发中使用方法!话不多说,先上效果图! 白嫖万岁!当然,如果有帮助,希望不要吝啬你的点赞呀!

👉 前言
在 Vue + elementUi 开发中,遇到需要实现一个富文本展示 且 需要实现富文本全文搜索,高亮对应搜索内容。显示关键词出现次数,允许上下按顺序切换,实现滚动条定位到对应关键词位置!
接下来,简单阐述下,开发中使用方法!
👉 一、效果演示
话不多说,先上效果图! 白嫖万岁!当然,如果有帮助,希望不要吝啬你的点赞呀!
以上数据来源于互联网记载的国内法律法规条例文献!
👉 二、实现思路
通过 v-html 将富文本解析到页面指定位置展示,并且预设的 class
名称,通过 document.querySelectorAll()
查询已渲染到页面的内容。
通过正则 htmlContent.replace(new RegExp(this.keyword, 'g'),
替换内容)。替换内容,通常为html样式的keyword
)
其实不难发现,在vue
和 JavaScript
中进行全文搜索有些许不同。由于其中搜索的HTML
是我们通过 v-html渲染
到页面上,它享有Vue数据双向绑定
的特性,我们其实只需要对v-html
绑定的变量参数进行修改,即可实时渲染到页面。当然有些标签属性变更,使用JavaScript来修改可能会更加高效一点。
稍微增加一点切换的滚动条挪动 及 样式清除细节逻辑!直接看代码吧! 简单易懂!
👉 三、实现案例
> 父组件中引用
<template>
<fullTextSearchDialog
v-if="lawDialogVisible"
ref="fullTextSearchDialog"
:curTitle="curTitle"
:content="lawFullText"
@close="closeLawFullTextDialog"
/>
</template>
<script>
import fullTextSearchDialog from "@/views/components/dialog/fullTextSearchDialog.vue";
data() {
return {
lawDialogVisible: false,
curTitle: '',
lawFullText: '',
}
}
methods: {
// 打开法律法规全文
openLawFullTextDialog(item) {
this.curTitle = item.title;
this.lawFullText = item.fullText;
this.lawDialogVisible = true
setTimeout(() => {
this.$refs.fullTextSearchDialog.openDialog()
}, 0)
},
// 关闭全文弹窗
closeLawFullTextDialog() {
this.curTitle = '';
this.lawFullText = ''
this.lawDialogVisible = false
},
}
</script>
> 子组件模板
<template>
<el-dialog
:title="curTitle + '-全文'"
:visible.sync="lawDialogVisible"
width="61.7%"
height="500"
:close-on-click-modal="true"
:modal="false"
custom-class="abolishDialog"
@close="handleClose"
>
<div class="lawContent">
<div class="searchBox">
全文内容搜索:
<el-input
placeholder="请输入关键词"
v-model="keyword"
class="input-with-select"
:clearable="false"
style="width: calc(100% - 240px);"
>
<div class="indexChange" slot="suffix">
<span class="index">{{ searchIndex + ' / ' + searchAllIndexs }}</span>
<div class="btnBox">
<i class="el-icon-arrow-up" @click="searchIndexDown"></i>
<i class="el-icon-arrow-down" @click="searchIndexUp"></i>
</div>
</div>
</el-input>
<el-button type="primary" size="mini" @click="lawFullTextSearch"
>查 询</el-button
>
<el-button size="mini" @click="resetSearch(true)"
>重 置</el-button
>
</div>
<div class="fullTextContent">
<p
v-if="refresh"
style="
width: 100%;
"
v-html="lawFullText || '暂无数据'"
class="el-tiptap-editor__content"
></p>
</div>
</div>
</el-dialog>
</template>
<script>
export default {
components: {},
props: {
curTitle: {
type: String,
default: () => {
return '提示';
},
},
content: {
type: String,
default: () => {
return '';
},
},
},
data() {
return {
lawDialogVisible: false, //弹框显隐
lawFullText: '',
searchIndex: 0,
searchAllIndexs: 0,
keyword: '',
refresh: true,
};
},
mounted() {
this.lawFullText = JSON.parse(JSON.stringify(this.content))
},
watch: {},
computed: {},
methods: {
/**
* @description:打开弹框回调
*/
openDialog() {
this.lawDialogVisible = true;
},
// 法律法规全文内容关键词搜索高亮
lawFullTextSearch() {
// window.console.log(this.keyword)
if (this.keyword && this.keyword !== '' && this.lawFullText.indexOf(this.keyword) != -1) {
this.searchAllIndexs = (this.lawFullText.split(this.keyword).length - 1) || 0
this.searchIndex = 1
this.lawFullText = this.lawFullText.replace(new RegExp(this.keyword, 'g'), `<em class='searchText'>${this.keyword}</em>`); // 通过正则全局匹配关键字,查出来的文字进行高亮替换
setTimeout(() => {
let allSearchIndex = document.querySelectorAll('em')
allSearchIndex[this.searchIndex - 1].className = 'curSearchText'
// 使滚动条滚动到指定位置
this.scrollChange()
}, 0)
} else {
this.resetSearch()
this.$message.info('无当前查询内容 或 未输入关键词!')
}
},
// 重置搜索内容
resetSearch(resetKey = false) {
if(resetKey) {
this.keyword = ''
}
this.searchAllIndexs = 0;
this.searchIndex = 0;
// 清除上次的查询记录
this.lawFullText = this.lawFullText.replace(new RegExp('</?em.*?>', 'gi'), ``);
// 刷新
this.refresh = false;
setTimeout(() => {
this.refresh = true;
}, 0)
},
// 查询内容上一个
searchIndexUp() {
if(this.searchIndex > 0 && this.searchIndex <= this.searchAllIndexs && this.searchAllIndexs > 0) {
this.searchIndex = (this.searchIndex + 1) > this.searchAllIndexs ? 1 : (this.searchIndex + 1)
setTimeout(() => {
let allSearchIndex = document.querySelectorAll('em')
// 清除上一个选中样式
allSearchIndex[this.searchIndex - 2 < 0 ? this.searchAllIndexs - this.searchIndex : this.searchIndex - 2].className = 'searchText'
allSearchIndex[this.searchIndex - 1].className = 'curSearchText'
// 使滚动条滚动到指定位置
this.scrollChange()
}, 0)
} else {
this.searchIndex = 0
}
},
// 查询内容下一个
searchIndexDown() {
if(this.searchIndex > 0 && this.searchIndex <= this.searchAllIndexs && this.searchAllIndexs > 0) {
this.searchIndex = (this.searchIndex - 1) <= 0 ? this.searchAllIndexs : (this.searchIndex - 1)
setTimeout(() => {
let allSearchIndex = document.querySelectorAll('em')
// 清除上一个选中样式
allSearchIndex[this.searchIndex > this.searchAllIndexs - 1 ? this.searchAllIndexs - this.searchIndex : this.searchIndex].className = 'searchText'
allSearchIndex[this.searchIndex - 1].className = 'curSearchText'
// 使滚动条滚动到指定位置
this.scrollChange()
}, 0)
} else {
this.searchIndex = 0
}
},
/**
* @description:关闭弹框回调
*/
handleClose() {
this.lawDialogVisible = false;
setTimeout(() => {
this.lawFullText = ''
this.keyword = ''
this.searchIndex = 0
this.searchAllIndexs = 0
this.$emit('close')
}, 0)
},
// 滚动条定位
scrollChange() {
let fullTextDom = document.querySelector('.fullTextContent')
let curDom = document.querySelector('.curSearchText').parentNode
// window.console.log(curDom, curDom.offsetTop)
fullTextDom.scrollTop = curDom.offsetTop - 137 || 0
},
},
};
</script>
<style lang="scss" scoped>
/deep/ {
.abolishDialog {
background: #ffffff !important;
border: 1px solid #cccccc !important;
box-shadow: 0 2px 10px rgba(0, 0, 0, 0.2);
border-radius: 4px;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
margin-top: 0 !important;
min-width: 480px;
.lawContent {
height: 50vh;
min-height: 500px;
display: flex;
align-content: space-around;
flex-wrap: wrap;
.searchBox {
width: 100%;
display: flex;
justify-content: space-around;
align-items: center;
.el-input__inner {
padding-right: 100px;
}
.el-input__suffix-inner {
width: 90px;
display: flex;
justify-content: space-between;
.indexChange {
width: 100%;
display: flex;
justify-content: space-around;
align-items: center;
.btnBox {
display: flex;
flex-direction: column;
margin: 0 5px;
}
}
}
}
.fullTextContent {
width: 100%;
height: calc(100% - 70px);
padding-top: 20px;
overflow-y: auto;
.searchText {
background-color: yellow;
color: #333;
font-weight: bold;
margin: 0 3px;
padding: 2px;
border-radius: 5px;
}
.curSearchText {
background-color: red;
color:white;
padding: 3px;
font-weight: bold;
margin: 0 3px;
padding: 2px;
border-radius: 5px;
}
}
}
.el-dialog__header {
border-bottom: 0.5px solid #cccccc !important;
padding: 0 15px;
font-weight: bold;
display: flex;
align-items: center;
justify-content: space-between;
.el-dialog__headerbtn {
width: 20px;
position: relative;
top: 0;
right: 0;
}
// height: 48px !important;
}
.el-dialog__body {
// min-height: 100px;
padding: 15px;
.title {
font-size: 10.5px;
color: #f56c6c;
text-align: right;
margin-top: 15px;
}
}
.el-dialog__footer {
border-top: 0.5px solid #cccccc !important;
padding: 10px;
}
}
}
</style>
案例较为粗浅,仅供参考!
👍 卷王必胜!
如果本篇文章对您有所帮助! 请不要吝惜您的小手,给小温来个小小的点赞!您的支持是对小温无比的认同!
往期内容 💨
🔥 < 每日小技巧: 基于Vue状态的过渡动画 - Transition 和 TransitionGroup>
🔥 < JavaScript技术分享: 大文件切片上传 及 断点续传思路 >
更多推荐
所有评论(0)