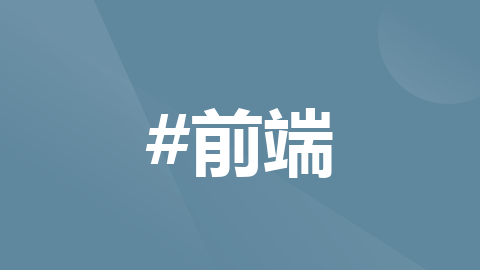
vue 轮播图的实现方法
以上是一个简单的Vue轮播图实现的示例。注意:上面的示例使用了默认的样式和配置。你可以根据需要自定义样式和配置,例如设置轮播图的宽度、高度、自动播放等。注意:上面的示例使用了默认的样式和配置。你可以根据需要自定义样式和配置,例如设置轮播图的宽度、高度、动画效果等。:在你的 Vue 项目中,通过 npm 或 yarn 安装。:在你的 Vue 项目中,通过 npm 或 yarn 安装。中定义了正确的图
1. Vue 轮播图的实现
- 创建Vue组件:创建一个名为"Carousel"的Vue组件。可以使用单文件组件(.vue文件)或在Vue实例中定义组件。
- 定义数据:在Carousel组件中定义一个数据属性,用于存储轮播图的图片列表和当前显示图片的索引。例如:
javascriptCopy code
data() {
return {
images: [
'image1.jpg',
'image2.jpg',
'image3.jpg'
],
currentIndex: 0
};
}
- 实现轮播逻辑:在Carousel组件中编写方法来切换当前显示图片的索引。可以使用计时器来定期更新索引值,实现自动轮播。例如:
methods: {
nextImage() {
this.currentIndex = (this.currentIndex + 1) % this.images.length;
},
prevImage() {
this.currentIndex = (this.currentIndex - 1 + this.images.length) % this.images.length;
}
},
mounted() {
// 自动轮播
setInterval(this.nextImage, 3000);
}
- 模板绑定:在Carousel组件的模板中使用动态绑定来显示当前索引对应的图片。可以使用
v-bind
指令将src
属性绑定到当前图片的路径。例如:
<template>
<div>
<img :src="images[currentIndex]" alt="Carousel Image">
<button @click="prevImage">Previous</button>
<button @click="nextImage">Next</button>
</div>
</template>
- 使用组件:在Vue根实例或其他组件中使用Carousel组件。例如:
phpCopy code
<template>
<div>
<h1>My Carousel</h1>
<carousel></carousel>
</div>
</template>
<script>
import Carousel from './Carousel.vue';
export default {
components: {
Carousel
}
};
</script>
以上是一个简单的Vue轮播图实现的示例。你可以根据具体的需求进行更复杂的样式和功能定制。还可以使用第三方的Vue轮播图库,如vue-carousel
或vue-awesome-swiper
,它们提供了更多的配置选项和交互效果。
2. vue-carousel 实现轮播图
-
安装
vue-carousel
:在你的 Vue 项目中,通过 npm 或 yarn 安装vue-carousel
。运行以下命令之一:bashCopy code npm install vue-carousel
bashCopy code yarn add vue-carousel
-
引入
vue-carousel
:在需要使用轮播图的组件中,导入vue-carousel
。javascriptCopy code import VueCarousel from 'vue-carousel';
-
注册
vue-carousel
组件:在组件的components
属性中注册VueCarousel
组件。javascriptCopy code export default { components: { VueCarousel, }, // ... }
-
使用
vue-carousel
:在组件的模板中使用vue-carousel
。可以使用v-for
循环指令来动态生成轮播项。htmlCopy code <template> <div> <vue-carousel> <slide v-for="(image, index) in images" :key="index"> <img :src="image" alt="Slide Image" /> </slide> </vue-carousel> </div> </template>
在上面的示例中,
images
是包含轮播图图片路径的数组。通过v-for
循环,生成对应数量的slide
组件,并在其中插入图片。注意:上面的示例使用了默认的样式和配置。你可以根据需要自定义样式和配置,例如设置轮播图的宽度、高度、自动播放等。
这样,你就可以使用 vue-carousel
实现轮播图了。确保在 data
中定义了正确的图片路径数组,并根据需要自定义样式和配置。
3. vue-awesome-swiper 实现轮播图
-
安装
vue-awesome-swiper
:在你的 Vue 项目中,通过 npm 或 yarn 安装vue-awesome-swiper
。运行以下命令之一:bashCopy code npm install vue-awesome-swiper
bashCopy code yarn add vue-awesome-swiper
-
引入
vue-awesome-swiper
:在需要使用轮播图的组件中,导入vue-awesome-swiper
。javascriptCopy code import 'swiper/css/swiper.css'; import { swiper, swiperSlide } from 'vue-awesome-swiper';
上面的示例代码中,我们导入了
swiper
和swiperSlide
组件,并引入了swiper.css
文件以应用默认的样式。 -
注册
vue-awesome-swiper
组件:在组件的components
属性中注册swiper
和swiperSlide
组件。javascriptCopy code export default { components: { swiper, swiperSlide, }, // ... }
-
使用
vue-awesome-swiper
:在组件的模板中使用vue-awesome-swiper
。htmlCopy code <template> <div> <swiper :options="swiperOptions"> <swiper-slide v-for="(image, index) in images" :key="index"> <img :src="image" alt="Slide Image" /> </swiper-slide> </swiper> </div> </template>
在上面的示例中,
images
是包含轮播图图片路径的数组。通过v-for
循环,生成对应数量的swiper-slide
组件,并在其中插入图片。swiperOptions
是一个对象,用于配置 Swiper 实例的选项。你可以在其中设置自动播放、导航按钮、分页器等。注意:上面的示例使用了默认的样式和配置。你可以根据需要自定义样式和配置,例如设置轮播图的宽度、高度、动画效果等。
这样,你就可以使用 vue-awesome-swiper
实现轮播图了。确保在 data
中定义了正确的图片路径数组,并根据需要自定义样式和配置。
更多推荐
所有评论(0)