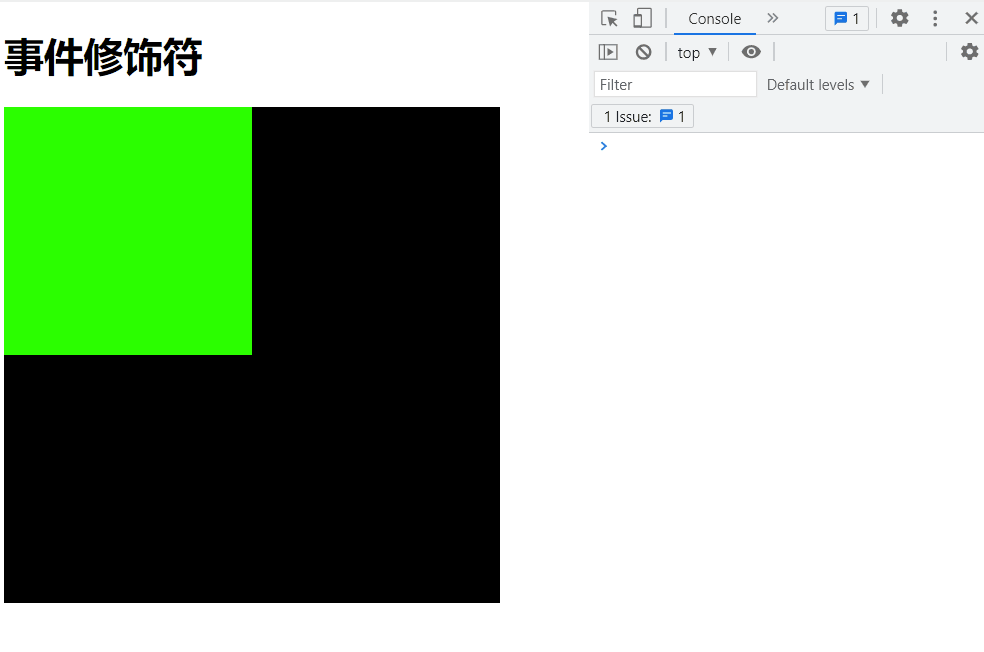
Vue.js中的两大指令:v-on和v-bind,实现页面动态渲染和事件响应
v-bind是Vue.js框架中的一个指令,用于动态绑定数据到HTML元素的特定属性上。它的作用是将Vue实例中的数据模型绑定到HTML中,从而实现页面的动态渲染和数据驱动。v-on用于绑定DOM事件监听器到HTML元素上,以便在用户触发这些事件时执行特定的逻辑。它的作用是将Vue实例中定义的方法与指定的HTML事件关联起来,从而实现事件处理和响应。
·
Vue.js中的两大指令:v-on和v-bind,实现页面动态渲染和事件响应
一、Vue指令
- vue指令, 实质上就是特殊的 html 标签属性, 特点: v- 开头
- 每个 v- 开头的指令, 都有着自己独立的功能, 将来vue解析时, 会根据不同的指令提供不同的功能
(一)v-bind指令
- 描述:插值表达式不能用在html的属性上,如果想要动态的设置html元素的属性,需要使用v-bind指令
- 作用:动态的设置html的属性,数据绑定操作
- 语法:
v-bind:title="msg"
- 简写:
:title="msg"
<!-- 完整语法 -->
<a v-bind:href="url"></a>
<!-- 缩写 -->
<a :href="url"></a>
<template>
<div>
<h1>v-bing</h1>
<p>作用:插值表达式适用于行外数据绑定! v-bind用于行内</p>
<a v-bind:href="msg">你好啊 Vue</a>
<a :href="msg">你好啊 Vue</a>
</div>
</template>
<script>
export default {
data(){
return{
msg:"www.baidu.com"
}
}
}
</script>
<style>
</style>
(二)v-on指令
1. 基本使用
- 作用:注册事件
- 语法:
①、 v-on:事件名=“要执行的少量代码"
②、v-on:事件名=“methods中的函数名"
③、v-on:事件名=“methods中的函数名(实参)" - 注意:事件处理函数在methods中提供
(1)最基本的语法
<button v-on:事件名="事件函数">按钮</button>
,需要在methods中提供事件处理函数
<button v-on:click="fn">搬砖</button>
<button v-on:click="fn1">卖房</button>
// 提供方法
methods: {
fn () {
console.log('你好啊')
// console.log(this)
//methods中的所有函数都可以通过this访问到当前组件
this.money++
},
fn1 () {
this.money += 10000
},
}
- 需要传递参数
<button v-on:事件名="事件函数(参数)">按钮</button>
,需要在methods中提供事件函数,接受参数
<template>
<div>
<h1>v-on</h1>
<p>商品数量:{{ num }}</p>
<p>
<!-- add(形参) -->
<button @click="add(7)">+几件</button>
</p>
</div>
</template>
<script>
export default {
data(){
return{
num:10
}
},methods:{
add(a){
//add(实参)
this.num+=a;
}
}
}
</script>
- 简写:v-on 可以 简写 成 @
<button @click="money = money+10">加十</button>
<button @click="addMoney(10000)">卖房</button>
2. Vue中获取事件对象(了解)
- 需求: 默认a标签点击会跳走, 希望阻止默认的跳转, 阻止默认行为 e.preventDefault()
- vue中获取事件对象
(1) 没有传参, 通过形参接收 e
(2) 传参了, 通过$event指代事件对象 e
<template>
<div>
<h1>获取事件对象</h1>
<button @click="fn">btn</button >
</div>
</template>
<script>
export default {
methods:{
fn(e){
// 执行函数,没有传任何实参,此时形参:打印的就是事件对象!
console.log(e)
}
}
}
</script>
- 在下面的代码中,我们通过点击百度一下按钮,按钮不会进行跳转,因为我们在fn方法里面添加了
e.preventDefault();
<template>
<div>
<h1>获取事件对象</h1>
<a @click="fn" href="https://www.baidu.com/">百度一下</a>
</div>
</template>
<script>
export default {
methods:{
fn(e){
e.preventDefault();
}
}
}
</script>
- 在fn1()方法里面传递的第一个值为形参,然后方法a实参传递进去;我们此时还想要一个对象,那么第二个值就必须写$event,这样我们在fn1()方法获取的才是对象。
<template>
<div>
<h1>获取事件对象</h1>
<!-- 问题:又要传实参,又要拿事件对象?? -->
<div @click="fn1(10,$event)" href="https://www.baidu.com/">百度一下</div>
</div>
</template>
<script>
export default {
methods:{
fn1(a,b){
//形参:拿实参!还要拿事件对象!
// 多个值,形参个数也是多个,a,b
// 实参:值,事件对象必须是$event
console.log(a,b);
}
}
}
</script>
3. v-on 事件修饰符
- 事件修饰符:vue提供事件修饰符,可以快速阻止默认行为或阻止冒泡
- vue中提供的事件修饰符
.prevent 阻止默认行为(下面代码阻止页面跳转)
.stop 阻止冒泡
<template>
<div>
<h1>事件修饰符</h1>
<!-- 事件后面加修饰符 .prevent组织阻止默认行为-->
<a href="https://www.baidu.com" @click.prevent="fn">百度一下</a>
</div>
</template>
<script>
export default {
methods:{
fn(){
console.log(1);
}
}
}
</script>
- 冒泡:从目标元素,往根元素一层一层的找,如果在它尽力的父辈元素们也注册了同样的事件类型,那么父辈会按照冒泡的顺序也执行了。
<template>
<div>
<h1>事件修饰符</h1>
<div class="father" @click="fn2">
<div class="son" @click="fn1"></div>
</div>
</div>
</template>
<script>
export default {
methods:{
fn1(){
console.log("儿子");
},
fn2(){
console.log("父亲");
}
}
}
</script>
<style>
.father{
width: 400px;
height: 400px;
background-color: rgb(0, 0, 0);
}
.son{
width: 200px;
height: 200px;
background-color: #2bff00;
}
</style>
- 那如何阻止上面冒泡事件的发声呢? 直接
.stop 阻止冒泡
即可。
<template>
<div>
<h1>事件修饰符</h1>
<div class="father" @click.stop="fn2">
<div class="son" @click.stop="fn1"></div>
</div>
</div>
</template>
<script>
export default {
methods:{
fn1(){
console.log("儿子");
},
fn2(){
console.log("父亲");
}
}
}
</script>
<style>
.father{
width: 400px;
height: 400px;
background-color: rgb(0, 0, 0);
}
.son{
width: 200px;
height: 200px;
background-color: #2bff00;
}
</style>
4. 按键修饰符
- 需求: 用户输入内容, 回车, 打印输入的内容
- 在监听键盘事件时,我们经常需要判断详细的按键。此时,可以为键盘相关的事件添加按键修饰符
- @keyup.enter 回车 — 只要按下回车才能触发这个键盘事件函数
- @keyup.esc 返回
<div id="app">
<input type="text" @keyup="fn"> <hr>
<input type="text" @keyup.enter="fn2">
</div>
5. 案例:反转字符串
<template>
<div>
<h1>反转世界</h1>
<h3>{{ msg }}</h3>
<button @click="fn">反转</button>
</div>
</template>
<script>
export default {
// 思路:
// 1.点击:肯定注册点击事件
// 2.字反转,点击后控制字!字符串!
// 3.字符串一定是个初始化数据变量,控制这个变量,用相应的方法!
data(){
return{
msg:"一大堆文字,你要找麻烦??"
}
},
methods:{
fn(){
// 数组
let arr = this.msg.split("");
// 反转数组
let res = arr.reverse();
// 变为字符串
let str = res.join("");
// 重新赋值
this.msg = str;
}
}
}
</script>
更多推荐
所有评论(0)